Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Server / System / Data / Services / Providers / DataServiceProviderMethods.cs / 1607349 / DataServiceProviderMethods.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// DataServiceProvider methods used for queries over IDSP providers. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Providers { #region Namespaces using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Reflection; #endregion ///Use this class to perform late-bound operations on data service resource sets. public static class DataServiceProviderMethods { #region Internal MethodInfos ///MethodInfo for object DataServiceProviderMethods.GetValue(this object value, string propertyName). internal static readonly MethodInfo GetValueMethodInfo = typeof(DataServiceProviderMethods).GetMethod( "GetValue", BindingFlags.Static | BindingFlags.Public, null, new Type[] { typeof(object), typeof(ResourceProperty) }, null); ///MethodInfo for IEnumerable<T> DataServiceProviderMethods.GetSequenceValue(this object value, string propertyName). internal static readonly MethodInfo GetSequenceValueMethodInfo = typeof(DataServiceProviderMethods).GetMethod( "GetSequenceValue", BindingFlags.Static | BindingFlags.Public, null, new Type[] { typeof(object), typeof(ResourceProperty) }, null); ///MethodInfo for Convert. internal static readonly MethodInfo ConvertMethodInfo = typeof(DataServiceProviderMethods).GetMethod( "Convert", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for TypeIs. internal static readonly MethodInfo TypeIsMethodInfo = typeof(DataServiceProviderMethods).GetMethod( "TypeIs", BindingFlags.Static | BindingFlags.Public); #endregion #region GetValue, GetSequenceValue ///Gets a named value from the specified object. /// Object to get value from. /// ResourceProperty describing the property whose value needs to be fetched. ///The requested value. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object GetValue(object value, ResourceProperty property) { throw new NotImplementedException(); } ///Gets a named value from the specified object as a sequence. /// Object to get value from. /// ResourceProperty describing the property whose value needs to be fetched. ///expected result type ///The requested value as a sequence; null if not found. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1004:GenericMethodsShouldProvideTypeParameter", Justification = "need T for proper binding to collections")] public static IEnumerableGetSequenceValue (object value, ResourceProperty property) { throw new NotImplementedException(); } #endregion #region Type Conversions /// Performs an type cast on the specified value. /// Value. /// Resource type to check for. ///Casted value. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Convert(object value, ResourceType type) { throw new NotImplementedException(); } ///Performs an type check on the specified value. /// Value. /// Resource type to check for. ///True if value is-a type; false otherwise. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static bool TypeIs(object value, ResourceType type) { throw new NotImplementedException(); } #endregion #region Type Comparers ////// Compares 2 strings by ordinal, used to obtain MethodInfo for comparison operator expression parameter /// /// Left Parameter /// Right Parameter ///0 for equality, -1 for left less than right, 1 for left greater than right ////// Do not change the name of this function because LINQ to SQL is sensitive about the /// method name, so is EF probably. /// public static int Compare(String left, String right) { return Comparer.Default.Compare(left, right); } /// /// Compares 2 booleans with true greater than false, used to obtain MethodInfo for comparison operator expression parameter /// /// Left Parameter /// Right Parameter ///0 for equality, -1 for left less than right, 1 for left greater than right ////// Do not change the name of this function because LINQ to SQL is sensitive about the /// method name, so is EF probably. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.MSInternal", "CA908:AvoidTypesThatRequireJitCompilationInPrecompiledAssemblies", Justification = "Need implementation")] public static int Compare(bool left, bool right) { return Comparer.Default.Compare(left, right); } /// /// Compares 2 nullable booleans with true greater than false, used to obtain MethodInfo for comparison operator expression parameter /// /// Left Parameter /// Right Parameter ///0 for equality, -1 for left less than right, 1 for left greater than right ////// Do not change the name of this function because LINQ to SQL is sensitive about the /// method name, so is EF probably. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.MSInternal", "CA908:AvoidTypesThatRequireJitCompilationInPrecompiledAssemblies", Justification = "Need implementation")] public static int Compare(bool? left, bool? right) { return Comparer.Default.Compare(left, right); } /// /// Compares 2 guids by byte order, used to obtain MethodInfo for comparison operator expression parameter /// /// Left Parameter /// Right Parameter ///0 for equality, -1 for left less than right, 1 for left greater than right ////// Do not change the name of this function because LINQ to SQL is sensitive about the /// method name, so is EF probably. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.MSInternal", "CA908:AvoidTypesThatRequireJitCompilationInPrecompiledAssemblies", Justification = "Need implementation")] public static int Compare(Guid left, Guid right) { return Comparer.Default.Compare(left, right); } /// /// Compares 2 nullable guids by byte order, used to obtain MethodInfo for comparison operator expression parameter /// /// Left Parameter /// Right Parameter ///0 for equality, -1 for left less than right, 1 for left greater than right ////// Do not change the name of this function because LINQ to SQL is sensitive about the /// method name, so is EF probably. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.MSInternal", "CA908:AvoidTypesThatRequireJitCompilationInPrecompiledAssemblies", Justification = "Need implementation")] public static int Compare(Guid? left, Guid? right) { return Comparer.Default.Compare(left, right); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
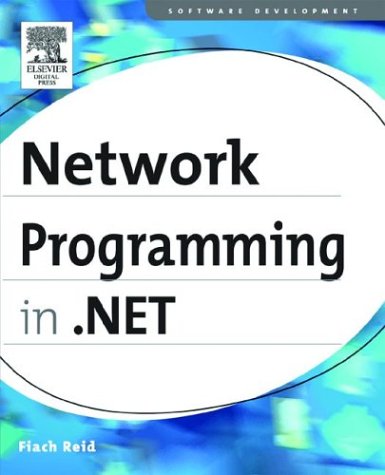
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- shaperfactoryquerycacheentry.cs
- ColorTransform.cs
- AuthorizationRuleCollection.cs
- DbCommandTree.cs
- ItemList.cs
- TextElement.cs
- TypeRestriction.cs
- ValueSerializerAttribute.cs
- HelpKeywordAttribute.cs
- IndexedSelectQueryOperator.cs
- AspNetSynchronizationContext.cs
- CancelEventArgs.cs
- NativeMethodsOther.cs
- PackagePartCollection.cs
- SettingsAttributes.cs
- SqlTypeSystemProvider.cs
- InvalidPrinterException.cs
- DataViewListener.cs
- Random.cs
- XmlRawWriter.cs
- RequestCache.cs
- BaseCodePageEncoding.cs
- CellIdBoolean.cs
- ThemeInfoAttribute.cs
- AutoResetEvent.cs
- ActivityExecutorOperation.cs
- Group.cs
- ReferencedCollectionType.cs
- Mouse.cs
- DefaultPropertyAttribute.cs
- XmlILIndex.cs
- ParameterCollection.cs
- CommandEventArgs.cs
- NonVisualControlAttribute.cs
- ListChangedEventArgs.cs
- ToolStripContainer.cs
- Ipv6Element.cs
- JsonFormatGeneratorStatics.cs
- StoreContentChangedEventArgs.cs
- HMACMD5.cs
- ConstrainedDataObject.cs
- ContractsBCL.cs
- DoubleStorage.cs
- OpenFileDialog.cs
- ObjectQueryProvider.cs
- HorizontalAlignConverter.cs
- ClientSettingsSection.cs
- UpdateTracker.cs
- ConfigWriter.cs
- LoginDesignerUtil.cs
- ControlBuilder.cs
- DataControlReference.cs
- PageThemeBuildProvider.cs
- SQLBytes.cs
- UnknownExceptionActionHelper.cs
- GlobalizationAssembly.cs
- OnOperation.cs
- StreamReader.cs
- WindowsComboBox.cs
- DetailsViewRowCollection.cs
- ItemsPanelTemplate.cs
- DurableMessageDispatchInspector.cs
- SoundPlayer.cs
- HttpInputStream.cs
- LinkConverter.cs
- WebProxyScriptElement.cs
- TextElementEnumerator.cs
- ProfileManager.cs
- CodeCommentStatement.cs
- AuthenticationSection.cs
- BitmapEffectState.cs
- OleStrCAMarshaler.cs
- StylusSystemGestureEventArgs.cs
- RegistrationContext.cs
- PassportAuthenticationModule.cs
- StreamAsIStream.cs
- KeyValueSerializer.cs
- ProcessModule.cs
- TargetPerspective.cs
- InitializationEventAttribute.cs
- OpacityConverter.cs
- PopupEventArgs.cs
- DetailsViewPageEventArgs.cs
- _HelperAsyncResults.cs
- CrossSiteScriptingValidation.cs
- ToolZone.cs
- XmlQueryCardinality.cs
- DataGridViewCellStyleChangedEventArgs.cs
- SimpleApplicationHost.cs
- ClientRolePrincipal.cs
- IdentitySection.cs
- MatrixAnimationUsingPath.cs
- SevenBitStream.cs
- IdentifierElement.cs
- TextEffect.cs
- HMACSHA256.cs
- EventLogPermissionAttribute.cs
- ConnectionStringSettingsCollection.cs
- TextDecorationCollectionConverter.cs
- UnsafeNativeMethodsPenimc.cs