Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Configuration / System / Configuration / ConnectionStringSettingsCollection.cs / 1305376 / ConnectionStringSettingsCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.IO; using System.Text; // class ConnectionStringsSection [ConfigurationCollection(typeof(ConnectionStringSettings))] public sealed class ConnectionStringSettingsCollection : ConfigurationElementCollection { private static ConfigurationPropertyCollection _properties; static ConnectionStringSettingsCollection() { // Property initialization _properties = new ConfigurationPropertyCollection(); } protected internal override ConfigurationPropertyCollection Properties { get { return _properties; } } public ConnectionStringSettingsCollection() : base(StringComparer.OrdinalIgnoreCase) { } public ConnectionStringSettings this[int index] { get { return (ConnectionStringSettings)BaseGet(index); } set { if (BaseGet(index) != null) { BaseRemoveAt(index); } BaseAdd(index, value); } } new public ConnectionStringSettings this[string name] { get { return (ConnectionStringSettings)BaseGet(name); } } public int IndexOf(ConnectionStringSettings settings) { return BaseIndexOf(settings); } // the connection string behavior is strange in that is acts kind of like a // basic map and partially like a add remove clear collection // Overriding these methods allows for the specific behaviors to be // patterened protected override void BaseAdd(int index, ConfigurationElement element) { if (index == -1) { BaseAdd(element, false); } else { base.BaseAdd(index, element); } } public void Add(ConnectionStringSettings settings) { BaseAdd(settings); } public void Remove(ConnectionStringSettings settings) { if (BaseIndexOf(settings) >= 0) { BaseRemove(settings.Key); } } public void RemoveAt(int index) { BaseRemoveAt(index); } public void Remove(string name) { BaseRemove(name); } protected override ConfigurationElement CreateNewElement() { return new ConnectionStringSettings(); } protected override Object GetElementKey(ConfigurationElement element) { return ((ConnectionStringSettings)element).Key; } public void Clear() { BaseClear(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.IO; using System.Text; // class ConnectionStringsSection [ConfigurationCollection(typeof(ConnectionStringSettings))] public sealed class ConnectionStringSettingsCollection : ConfigurationElementCollection { private static ConfigurationPropertyCollection _properties; static ConnectionStringSettingsCollection() { // Property initialization _properties = new ConfigurationPropertyCollection(); } protected internal override ConfigurationPropertyCollection Properties { get { return _properties; } } public ConnectionStringSettingsCollection() : base(StringComparer.OrdinalIgnoreCase) { } public ConnectionStringSettings this[int index] { get { return (ConnectionStringSettings)BaseGet(index); } set { if (BaseGet(index) != null) { BaseRemoveAt(index); } BaseAdd(index, value); } } new public ConnectionStringSettings this[string name] { get { return (ConnectionStringSettings)BaseGet(name); } } public int IndexOf(ConnectionStringSettings settings) { return BaseIndexOf(settings); } // the connection string behavior is strange in that is acts kind of like a // basic map and partially like a add remove clear collection // Overriding these methods allows for the specific behaviors to be // patterened protected override void BaseAdd(int index, ConfigurationElement element) { if (index == -1) { BaseAdd(element, false); } else { base.BaseAdd(index, element); } } public void Add(ConnectionStringSettings settings) { BaseAdd(settings); } public void Remove(ConnectionStringSettings settings) { if (BaseIndexOf(settings) >= 0) { BaseRemove(settings.Key); } } public void RemoveAt(int index) { BaseRemoveAt(index); } public void Remove(string name) { BaseRemove(name); } protected override ConfigurationElement CreateNewElement() { return new ConnectionStringSettings(); } protected override Object GetElementKey(ConfigurationElement element) { return ((ConnectionStringSettings)element).Key; } public void Clear() { BaseClear(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
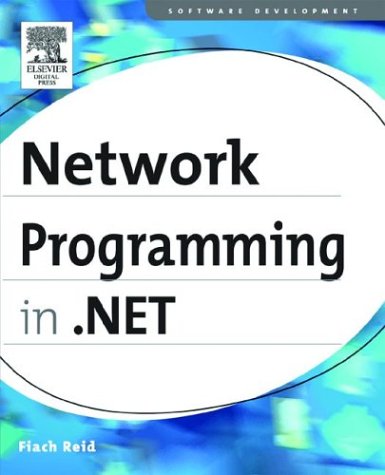
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BitmapEffectrendercontext.cs
- GraphicsContainer.cs
- OdbcConnectionOpen.cs
- FileRecordSequence.cs
- MasterPage.cs
- StateManagedCollection.cs
- WebScriptServiceHost.cs
- ByteAnimation.cs
- XamlFxTrace.cs
- Glyph.cs
- TreeNodeCollection.cs
- AppDomainCompilerProxy.cs
- Command.cs
- WindowsAuthenticationModule.cs
- WebPartManagerInternals.cs
- RelationshipEndMember.cs
- ComponentDispatcher.cs
- UdpChannelFactory.cs
- MailDefinition.cs
- FilteredReadOnlyMetadataCollection.cs
- ResourceContainer.cs
- Application.cs
- SamlDoNotCacheCondition.cs
- XPathConvert.cs
- DesignerTransaction.cs
- CodeIdentifiers.cs
- ParameterModifier.cs
- ReadOnlyKeyedCollection.cs
- _LocalDataStoreMgr.cs
- ConnectionPointCookie.cs
- WeakReferenceEnumerator.cs
- VisualBrush.cs
- DataServiceQueryProvider.cs
- BlockCollection.cs
- EntitySetDataBindingList.cs
- PageFunction.cs
- HtmlButton.cs
- ToolStripProgressBar.cs
- SqlDataSourceFilteringEventArgs.cs
- LocalFileSettingsProvider.cs
- SqlDependencyListener.cs
- TaiwanCalendar.cs
- EntitySqlQueryBuilder.cs
- ArgumentValue.cs
- ThicknessAnimationBase.cs
- ImportContext.cs
- Compress.cs
- Frame.cs
- PartialToken.cs
- ClientFormsAuthenticationCredentials.cs
- DiagnosticsConfiguration.cs
- PenThreadWorker.cs
- GetWinFXPath.cs
- ReadOnlyHierarchicalDataSourceView.cs
- TripleDES.cs
- CustomAttributeSerializer.cs
- RequestResizeEvent.cs
- EntityDescriptor.cs
- DataGrid.cs
- CodeSnippetStatement.cs
- Debug.cs
- AnimatedTypeHelpers.cs
- UpdateManifestForBrowserApplication.cs
- WaitingCursor.cs
- WmlFormAdapter.cs
- PageMediaSize.cs
- WebControlsSection.cs
- BitmapEffectCollection.cs
- HttpConfigurationSystem.cs
- WindowsFormsSectionHandler.cs
- ToolBarOverflowPanel.cs
- TemplatedWizardStep.cs
- GlobalizationAssembly.cs
- RenderingEventArgs.cs
- ExtensionDataReader.cs
- StateMachineDesignerPaint.cs
- Run.cs
- PageAsyncTask.cs
- ProgressBarHighlightConverter.cs
- Transactions.cs
- ResXResourceWriter.cs
- Wildcard.cs
- Help.cs
- PageHandlerFactory.cs
- SqlCommandSet.cs
- WebPartsSection.cs
- UInt16Storage.cs
- PermissionToken.cs
- SmtpMail.cs
- SelectionProcessor.cs
- CodeNamespaceCollection.cs
- PropertySegmentSerializer.cs
- ObjectSet.cs
- WebPartConnectionsDisconnectVerb.cs
- COM2IDispatchConverter.cs
- SafeSecurityHandles.cs
- _LazyAsyncResult.cs
- KeySpline.cs
- Canvas.cs
- ControlBindingsCollection.cs