Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / xsp / System / Web / UI / WebControls / ParameterCollection.cs / 1 / ParameterCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.ComponentModel.Design; using System.Data; using System.Drawing.Design; using System.Globalization; using System.Reflection; using System.Web.UI; using System.Web.UI.WebControls; using System.Web.Util; using System.Security.Permissions; ////// A state managed collection of Parameter objects. /// These are used in many DataSourceControls to filter queries. /// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] [ Editor("System.Web.UI.Design.WebControls.ParameterCollectionEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)), ] public class ParameterCollection : StateManagedCollection { private EventHandler _parametersChangedHandler; private static readonly Type[] knownTypes = new Type[] { typeof(ControlParameter), typeof(CookieParameter), typeof(FormParameter), typeof(Parameter), typeof(QueryStringParameter), typeof(SessionParameter), typeof(ProfileParameter), }; ////// Returns the Parameter at a given index. /// public Parameter this[int index] { get { return (Parameter)((IList)this)[index]; } set { ((IList)this)[index] = value; } } ////// Returns the Parameter with a given name. /// public Parameter this[string name] { get { int parameterIndex = GetParameterIndex(name); if (parameterIndex == -1) { return null; } return this[parameterIndex]; } set { int parameterIndex = GetParameterIndex(name); if (parameterIndex == -1) { Add(value); } else { this[parameterIndex] = value; } } } ////// Occurs when any of the Parameter objects in the collection change or when the collection itself changes. /// public event EventHandler ParametersChanged { add { _parametersChangedHandler = (EventHandler)Delegate.Combine(_parametersChangedHandler, value); } remove { _parametersChangedHandler = (EventHandler)Delegate.Remove(_parametersChangedHandler, value); } } ////// Adds a Parameter to the collection. /// public int Add(Parameter parameter) { return ((IList)this).Add(parameter); } ////// Adds a Parameter to the collection with a specified name and value. /// public int Add(string name, string value) { return ((IList)this).Add(new Parameter(name, TypeCode.Empty, value)); } ////// Adds a Parameter to the collection with a specified name, type, and value. /// public int Add(string name, TypeCode type, string value) { return ((IList)this).Add(new Parameter(name, type, value)); } ////// Adds a Parameter to the collection with a specified name, database type, and value. /// public int Add(string name, DbType dbType, string value) { return ((IList)this).Add(new Parameter(name, dbType, value)); } ////// Used by Parameters to raise the ParametersChanged event. /// internal void CallOnParametersChanged() { OnParametersChanged(EventArgs.Empty); } public bool Contains(Parameter parameter) { return ((IList)this).Contains(parameter); } public void CopyTo(Parameter[] parameterArray, int index) { base.CopyTo(parameterArray, index); } ////// Creates a known type of Parameter. /// protected override object CreateKnownType(int index) { switch (index) { case 0: return new ControlParameter(); case 1: return new CookieParameter(); case 2: return new FormParameter(); case 3: return new Parameter(); case 4: return new QueryStringParameter(); case 5: return new SessionParameter(); case 6: return new ProfileParameter(); default: throw new ArgumentOutOfRangeException("index"); } } ////// Returns an ArrayList of known Parameter types. /// protected override Type[] GetKnownTypes() { return knownTypes; } ////// Returns the index of a parameter by name. /// private int GetParameterIndex(string name) { for (int i = 0; i < Count; i++) { if (String.Equals(this[i].Name, name, StringComparison.OrdinalIgnoreCase)) { return i; } } return -1; } ////// Returns an IDictionary containing Name / Value pairs of all the parameters. /// public IOrderedDictionary GetValues(HttpContext context, Control control) { UpdateValues(context, control); // Create dictionary IOrderedDictionary valueDictionary = new OrderedDictionary(); // Add Parameters foreach (Parameter param in this) { // For the OrderedDictionary, every parameter must have a unique name, so in some cases we have to alter them. string uniqueName = param.Name; int count = 1; while (valueDictionary.Contains(uniqueName)) { uniqueName = param.Name + count.ToString(CultureInfo.InvariantCulture); count++; } valueDictionary.Add(uniqueName, param.ParameterValue); } return valueDictionary; } public int IndexOf(Parameter parameter) { return ((IList)this).IndexOf(parameter); } ////// Inserts a Parameter into the collection. /// public void Insert(int index, Parameter parameter) { ((IList)this).Insert(index, parameter); } ////// Called when the Clear() method is complete. /// protected override void OnClearComplete() { base.OnClearComplete(); OnParametersChanged(EventArgs.Empty); } ////// Called when the Insert() method is starting. /// Adds an event handler to listen to the Parameter's ParameterChanged event. /// protected override void OnInsert(int index, object value) { base.OnInsert(index, value); // Set owner (we are guaranteed that it is a Parameter // in OnValidate). ((Parameter)value).SetOwner(this); } ////// Called when the Insert() method is complete. /// protected override void OnInsertComplete(int index, object value) { base.OnInsertComplete(index, value); OnParametersChanged(EventArgs.Empty); } ////// Raises the ParametersChanged event. /// protected virtual void OnParametersChanged(EventArgs e) { if (_parametersChangedHandler != null) { _parametersChangedHandler(this, e); } } ////// Called when the Remove() method is complete. /// protected override void OnRemoveComplete(int index, object value) { base.OnRemoveComplete(index, value); // Clear owner ((Parameter)value).SetOwner(null); OnParametersChanged(EventArgs.Empty); } ////// Validates that an object is a Parameter. /// protected override void OnValidate(object o) { base.OnValidate(o); if (!(o is Parameter)) throw new ArgumentException(SR.GetString(SR.ParameterCollection_NotParameter), "o"); } ////// Removes a Parameter from the collection. /// public void Remove(Parameter parameter) { ((IList)this).Remove(parameter); } ////// Removes a Parameter from the collection at a given index. /// public void RemoveAt(int index) { ((IList)this).RemoveAt(index); } ////// Marks a Parameter as dirty so that it will record its entire state into view state. /// protected override void SetDirtyObject(object o) { ((Parameter)o).SetDirty(); } ////// Updates all parameter values to possibly raise a ParametersChanged event. /// public void UpdateValues(HttpContext context, Control control) { foreach (Parameter param in this) { param.UpdateValue(context, control); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.ComponentModel.Design; using System.Data; using System.Drawing.Design; using System.Globalization; using System.Reflection; using System.Web.UI; using System.Web.UI.WebControls; using System.Web.Util; using System.Security.Permissions; ////// A state managed collection of Parameter objects. /// These are used in many DataSourceControls to filter queries. /// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] [ Editor("System.Web.UI.Design.WebControls.ParameterCollectionEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)), ] public class ParameterCollection : StateManagedCollection { private EventHandler _parametersChangedHandler; private static readonly Type[] knownTypes = new Type[] { typeof(ControlParameter), typeof(CookieParameter), typeof(FormParameter), typeof(Parameter), typeof(QueryStringParameter), typeof(SessionParameter), typeof(ProfileParameter), }; ////// Returns the Parameter at a given index. /// public Parameter this[int index] { get { return (Parameter)((IList)this)[index]; } set { ((IList)this)[index] = value; } } ////// Returns the Parameter with a given name. /// public Parameter this[string name] { get { int parameterIndex = GetParameterIndex(name); if (parameterIndex == -1) { return null; } return this[parameterIndex]; } set { int parameterIndex = GetParameterIndex(name); if (parameterIndex == -1) { Add(value); } else { this[parameterIndex] = value; } } } ////// Occurs when any of the Parameter objects in the collection change or when the collection itself changes. /// public event EventHandler ParametersChanged { add { _parametersChangedHandler = (EventHandler)Delegate.Combine(_parametersChangedHandler, value); } remove { _parametersChangedHandler = (EventHandler)Delegate.Remove(_parametersChangedHandler, value); } } ////// Adds a Parameter to the collection. /// public int Add(Parameter parameter) { return ((IList)this).Add(parameter); } ////// Adds a Parameter to the collection with a specified name and value. /// public int Add(string name, string value) { return ((IList)this).Add(new Parameter(name, TypeCode.Empty, value)); } ////// Adds a Parameter to the collection with a specified name, type, and value. /// public int Add(string name, TypeCode type, string value) { return ((IList)this).Add(new Parameter(name, type, value)); } ////// Adds a Parameter to the collection with a specified name, database type, and value. /// public int Add(string name, DbType dbType, string value) { return ((IList)this).Add(new Parameter(name, dbType, value)); } ////// Used by Parameters to raise the ParametersChanged event. /// internal void CallOnParametersChanged() { OnParametersChanged(EventArgs.Empty); } public bool Contains(Parameter parameter) { return ((IList)this).Contains(parameter); } public void CopyTo(Parameter[] parameterArray, int index) { base.CopyTo(parameterArray, index); } ////// Creates a known type of Parameter. /// protected override object CreateKnownType(int index) { switch (index) { case 0: return new ControlParameter(); case 1: return new CookieParameter(); case 2: return new FormParameter(); case 3: return new Parameter(); case 4: return new QueryStringParameter(); case 5: return new SessionParameter(); case 6: return new ProfileParameter(); default: throw new ArgumentOutOfRangeException("index"); } } ////// Returns an ArrayList of known Parameter types. /// protected override Type[] GetKnownTypes() { return knownTypes; } ////// Returns the index of a parameter by name. /// private int GetParameterIndex(string name) { for (int i = 0; i < Count; i++) { if (String.Equals(this[i].Name, name, StringComparison.OrdinalIgnoreCase)) { return i; } } return -1; } ////// Returns an IDictionary containing Name / Value pairs of all the parameters. /// public IOrderedDictionary GetValues(HttpContext context, Control control) { UpdateValues(context, control); // Create dictionary IOrderedDictionary valueDictionary = new OrderedDictionary(); // Add Parameters foreach (Parameter param in this) { // For the OrderedDictionary, every parameter must have a unique name, so in some cases we have to alter them. string uniqueName = param.Name; int count = 1; while (valueDictionary.Contains(uniqueName)) { uniqueName = param.Name + count.ToString(CultureInfo.InvariantCulture); count++; } valueDictionary.Add(uniqueName, param.ParameterValue); } return valueDictionary; } public int IndexOf(Parameter parameter) { return ((IList)this).IndexOf(parameter); } ////// Inserts a Parameter into the collection. /// public void Insert(int index, Parameter parameter) { ((IList)this).Insert(index, parameter); } ////// Called when the Clear() method is complete. /// protected override void OnClearComplete() { base.OnClearComplete(); OnParametersChanged(EventArgs.Empty); } ////// Called when the Insert() method is starting. /// Adds an event handler to listen to the Parameter's ParameterChanged event. /// protected override void OnInsert(int index, object value) { base.OnInsert(index, value); // Set owner (we are guaranteed that it is a Parameter // in OnValidate). ((Parameter)value).SetOwner(this); } ////// Called when the Insert() method is complete. /// protected override void OnInsertComplete(int index, object value) { base.OnInsertComplete(index, value); OnParametersChanged(EventArgs.Empty); } ////// Raises the ParametersChanged event. /// protected virtual void OnParametersChanged(EventArgs e) { if (_parametersChangedHandler != null) { _parametersChangedHandler(this, e); } } ////// Called when the Remove() method is complete. /// protected override void OnRemoveComplete(int index, object value) { base.OnRemoveComplete(index, value); // Clear owner ((Parameter)value).SetOwner(null); OnParametersChanged(EventArgs.Empty); } ////// Validates that an object is a Parameter. /// protected override void OnValidate(object o) { base.OnValidate(o); if (!(o is Parameter)) throw new ArgumentException(SR.GetString(SR.ParameterCollection_NotParameter), "o"); } ////// Removes a Parameter from the collection. /// public void Remove(Parameter parameter) { ((IList)this).Remove(parameter); } ////// Removes a Parameter from the collection at a given index. /// public void RemoveAt(int index) { ((IList)this).RemoveAt(index); } ////// Marks a Parameter as dirty so that it will record its entire state into view state. /// protected override void SetDirtyObject(object o) { ((Parameter)o).SetDirty(); } ////// Updates all parameter values to possibly raise a ParametersChanged event. /// public void UpdateValues(HttpContext context, Control control) { foreach (Parameter param in this) { param.UpdateValue(context, control); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
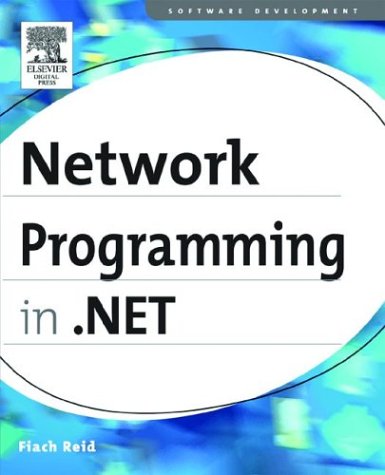
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridColumn.cs
- DispatchChannelSink.cs
- DateTimeFormatInfoScanner.cs
- UnsafeNativeMethodsMilCoreApi.cs
- Condition.cs
- ImageIndexConverter.cs
- DataGridViewCellCollection.cs
- ToolboxItemWrapper.cs
- TypeUtils.cs
- SchemaContext.cs
- BitmapMetadataEnumerator.cs
- QilList.cs
- FontUnit.cs
- WSHttpTransportSecurityElement.cs
- TypeGeneratedEventArgs.cs
- TrackBar.cs
- QueryGenerator.cs
- PseudoWebRequest.cs
- PowerStatus.cs
- BindingContext.cs
- JsonSerializer.cs
- DataException.cs
- SequenceDesigner.cs
- XmlILAnnotation.cs
- oledbmetadatacolumnnames.cs
- SqlParameterizer.cs
- CustomAttributeSerializer.cs
- Cursor.cs
- GestureRecognizer.cs
- PreservationFileWriter.cs
- CellCreator.cs
- SettingsPropertyWrongTypeException.cs
- SystemInfo.cs
- SerialPinChanges.cs
- QueueProcessor.cs
- FunctionNode.cs
- _BaseOverlappedAsyncResult.cs
- InvalidProgramException.cs
- HtmlHead.cs
- HttpHeaderCollection.cs
- GridSplitter.cs
- DrawingServices.cs
- SchemaAttDef.cs
- DateTimeOffsetStorage.cs
- HtmlInputButton.cs
- DataGridRow.cs
- PermissionAttributes.cs
- RemoteWebConfigurationHost.cs
- LogSwitch.cs
- BinaryReader.cs
- SqlParameter.cs
- ReferenceSchema.cs
- ListViewUpdatedEventArgs.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- ClipboardProcessor.cs
- MetaForeignKeyColumn.cs
- CustomErrorCollection.cs
- _ProxyChain.cs
- CacheVirtualItemsEvent.cs
- FilteredDataSetHelper.cs
- KnownTypes.cs
- HtmlToClrEventProxy.cs
- FacetValueContainer.cs
- StructureChangedEventArgs.cs
- ListControlConvertEventArgs.cs
- DefaultWorkflowLoaderService.cs
- SqlHelper.cs
- Roles.cs
- Point.cs
- VerificationAttribute.cs
- UnrecognizedAssertionsBindingElement.cs
- TextParaClient.cs
- Models.cs
- XmlSchemaAny.cs
- Transform3DGroup.cs
- SimpleRecyclingCache.cs
- AutoResetEvent.cs
- SerialErrors.cs
- ScriptComponentDescriptor.cs
- ResolveMatchesCD1.cs
- HttpCapabilitiesSectionHandler.cs
- WCFServiceClientProxyGenerator.cs
- DataComponentGenerator.cs
- SqlPersonalizationProvider.cs
- Columns.cs
- Literal.cs
- LineSegment.cs
- TreeIterators.cs
- PointHitTestResult.cs
- PageCatalogPartDesigner.cs
- SchemaNames.cs
- DetailsViewPageEventArgs.cs
- AbstractSvcMapFileLoader.cs
- VBCodeProvider.cs
- ListChangedEventArgs.cs
- DelegateSerializationHolder.cs
- SQLRoleProvider.cs
- ActivityDesignerResources.cs
- ValueOfAction.cs
- ConvertersCollection.cs