Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / tx / System / Transactions / EnlistmentState.cs / 1305376 / EnlistmentState.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Transactions { using System; using System.Diagnostics; using System.Globalization; using System.Threading; using System.Transactions.Diagnostics; // Base class for all enlistment states abstract class EnlistmentState { internal abstract void EnterState( InternalEnlistment enlistment ); internal static EnlistmentStatePromoted _enlistmentStatePromoted; // Object for synchronizing access to the entire class( avoiding lock( typeof( ... )) ) private static object classSyncObject; // Helper object for static synchronization private static object ClassSyncObject { get { if( classSyncObject == null ) { object o = new object(); Interlocked.CompareExchange( ref classSyncObject, o, null ); } return classSyncObject; } } internal static EnlistmentStatePromoted _EnlistmentStatePromoted { get { if (_enlistmentStatePromoted == null) { lock (ClassSyncObject) { if (_enlistmentStatePromoted == null) { EnlistmentStatePromoted temp = new EnlistmentStatePromoted(); Thread.MemoryBarrier(); _enlistmentStatePromoted = temp; } } } return _enlistmentStatePromoted; } } internal virtual void EnlistmentDone( InternalEnlistment enlistment ) { throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void Prepared( InternalEnlistment enlistment ) { throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void ForceRollback( InternalEnlistment enlistment, Exception e ) { throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void Committed( InternalEnlistment enlistment ) { throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void Aborted( InternalEnlistment enlistment, Exception e ) { throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void InDoubt( InternalEnlistment enlistment, Exception e ) { throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual byte[] RecoveryInformation( InternalEnlistment enlistment ) { throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void InternalAborted( InternalEnlistment enlistment ) { Debug.Assert( false, string.Format( null, "Invalid Event for InternalEnlistment State; Current State: {0}", this.GetType() )); throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void InternalCommitted( InternalEnlistment enlistment ) { Debug.Assert( false, string.Format( null, "Invalid Event for InternalEnlistment State; Current State: {0}", this.GetType() )); throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void InternalIndoubt( InternalEnlistment enlistment ) { Debug.Assert( false, string.Format( null, "Invalid Event for InternalEnlistment State; Current State: {0}", this.GetType() )); throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void ChangeStateCommitting( InternalEnlistment enlistment ) { Debug.Assert( false, string.Format( null, "Invalid Event for InternalEnlistment State; Current State: {0}", this.GetType() )); throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void ChangeStatePromoted( InternalEnlistment enlistment, IPromotedEnlistment promotedEnlistment ) { Debug.Assert( false, string.Format( null, "Invalid Event for InternalEnlistment State; Current State: {0}", this.GetType() )); throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void ChangeStateDelegated( InternalEnlistment enlistment ) { Debug.Assert( false, string.Format( null, "Invalid Event for InternalEnlistment State; Current State: {0}", this.GetType() )); throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void ChangeStatePreparing( InternalEnlistment enlistment ) { Debug.Assert( false, string.Format( null, "Invalid Event for InternalEnlistment State; Current State: {0}", this.GetType() )); throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void ChangeStateSinglePhaseCommit( InternalEnlistment enlistment ) { Debug.Assert( false, string.Format( null, "Invalid Event for InternalEnlistment State; Current State: {0}", this.GetType() )); throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } } internal class EnlistmentStatePromoted : EnlistmentState { internal override void EnterState( InternalEnlistment enlistment ) { enlistment.State = this; } internal override void EnlistmentDone( InternalEnlistment enlistment ) { Monitor.Exit( enlistment.SyncRoot ); try { enlistment.PromotedEnlistment.EnlistmentDone(); } finally { #pragma warning disable 0618 //@ Monitor.Enter(enlistment.SyncRoot); #pragma warning restore 0618 } } internal override void Prepared( InternalEnlistment enlistment ) { Monitor.Exit( enlistment.SyncRoot ); try { enlistment.PromotedEnlistment.Prepared(); } finally { #pragma warning disable 0618 //@ Monitor.Enter(enlistment.SyncRoot); #pragma warning restore 0618 } } internal override void ForceRollback( InternalEnlistment enlistment, Exception e ) { Monitor.Exit( enlistment.SyncRoot ); try { enlistment.PromotedEnlistment.ForceRollback( e ); } finally { #pragma warning disable 0618 //@ Monitor.Enter(enlistment.SyncRoot); #pragma warning restore 0618 } } internal override void Committed( InternalEnlistment enlistment ) { Monitor.Exit( enlistment.SyncRoot ); try { enlistment.PromotedEnlistment.Committed(); } finally { #pragma warning disable 0618 //@ Monitor.Enter(enlistment.SyncRoot); #pragma warning restore 0618 } } internal override void Aborted( InternalEnlistment enlistment, Exception e ) { Monitor.Exit( enlistment.SyncRoot ); try { enlistment.PromotedEnlistment.Aborted( e ); } finally { #pragma warning disable 0618 //@ Monitor.Enter(enlistment.SyncRoot); #pragma warning restore 0618 } } internal override void InDoubt( InternalEnlistment enlistment, Exception e ) { Monitor.Exit( enlistment.SyncRoot ); try { enlistment.PromotedEnlistment.InDoubt( e ); } finally { #pragma warning disable 0618 //@ Monitor.Enter(enlistment.SyncRoot); #pragma warning restore 0618 } } internal override byte[] RecoveryInformation( InternalEnlistment enlistment ) { Monitor.Exit( enlistment.SyncRoot ); try { return enlistment.PromotedEnlistment.GetRecoveryInformation(); } finally { #pragma warning disable 0618 //@ Monitor.Enter(enlistment.SyncRoot); #pragma warning restore 0618 } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Transactions { using System; using System.Diagnostics; using System.Globalization; using System.Threading; using System.Transactions.Diagnostics; // Base class for all enlistment states abstract class EnlistmentState { internal abstract void EnterState( InternalEnlistment enlistment ); internal static EnlistmentStatePromoted _enlistmentStatePromoted; // Object for synchronizing access to the entire class( avoiding lock( typeof( ... )) ) private static object classSyncObject; // Helper object for static synchronization private static object ClassSyncObject { get { if( classSyncObject == null ) { object o = new object(); Interlocked.CompareExchange( ref classSyncObject, o, null ); } return classSyncObject; } } internal static EnlistmentStatePromoted _EnlistmentStatePromoted { get { if (_enlistmentStatePromoted == null) { lock (ClassSyncObject) { if (_enlistmentStatePromoted == null) { EnlistmentStatePromoted temp = new EnlistmentStatePromoted(); Thread.MemoryBarrier(); _enlistmentStatePromoted = temp; } } } return _enlistmentStatePromoted; } } internal virtual void EnlistmentDone( InternalEnlistment enlistment ) { throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void Prepared( InternalEnlistment enlistment ) { throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void ForceRollback( InternalEnlistment enlistment, Exception e ) { throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void Committed( InternalEnlistment enlistment ) { throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void Aborted( InternalEnlistment enlistment, Exception e ) { throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void InDoubt( InternalEnlistment enlistment, Exception e ) { throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual byte[] RecoveryInformation( InternalEnlistment enlistment ) { throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void InternalAborted( InternalEnlistment enlistment ) { Debug.Assert( false, string.Format( null, "Invalid Event for InternalEnlistment State; Current State: {0}", this.GetType() )); throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void InternalCommitted( InternalEnlistment enlistment ) { Debug.Assert( false, string.Format( null, "Invalid Event for InternalEnlistment State; Current State: {0}", this.GetType() )); throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void InternalIndoubt( InternalEnlistment enlistment ) { Debug.Assert( false, string.Format( null, "Invalid Event for InternalEnlistment State; Current State: {0}", this.GetType() )); throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void ChangeStateCommitting( InternalEnlistment enlistment ) { Debug.Assert( false, string.Format( null, "Invalid Event for InternalEnlistment State; Current State: {0}", this.GetType() )); throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void ChangeStatePromoted( InternalEnlistment enlistment, IPromotedEnlistment promotedEnlistment ) { Debug.Assert( false, string.Format( null, "Invalid Event for InternalEnlistment State; Current State: {0}", this.GetType() )); throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void ChangeStateDelegated( InternalEnlistment enlistment ) { Debug.Assert( false, string.Format( null, "Invalid Event for InternalEnlistment State; Current State: {0}", this.GetType() )); throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void ChangeStatePreparing( InternalEnlistment enlistment ) { Debug.Assert( false, string.Format( null, "Invalid Event for InternalEnlistment State; Current State: {0}", this.GetType() )); throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void ChangeStateSinglePhaseCommit( InternalEnlistment enlistment ) { Debug.Assert( false, string.Format( null, "Invalid Event for InternalEnlistment State; Current State: {0}", this.GetType() )); throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } } internal class EnlistmentStatePromoted : EnlistmentState { internal override void EnterState( InternalEnlistment enlistment ) { enlistment.State = this; } internal override void EnlistmentDone( InternalEnlistment enlistment ) { Monitor.Exit( enlistment.SyncRoot ); try { enlistment.PromotedEnlistment.EnlistmentDone(); } finally { #pragma warning disable 0618 //@ Monitor.Enter(enlistment.SyncRoot); #pragma warning restore 0618 } } internal override void Prepared( InternalEnlistment enlistment ) { Monitor.Exit( enlistment.SyncRoot ); try { enlistment.PromotedEnlistment.Prepared(); } finally { #pragma warning disable 0618 //@ Monitor.Enter(enlistment.SyncRoot); #pragma warning restore 0618 } } internal override void ForceRollback( InternalEnlistment enlistment, Exception e ) { Monitor.Exit( enlistment.SyncRoot ); try { enlistment.PromotedEnlistment.ForceRollback( e ); } finally { #pragma warning disable 0618 //@ Monitor.Enter(enlistment.SyncRoot); #pragma warning restore 0618 } } internal override void Committed( InternalEnlistment enlistment ) { Monitor.Exit( enlistment.SyncRoot ); try { enlistment.PromotedEnlistment.Committed(); } finally { #pragma warning disable 0618 //@ Monitor.Enter(enlistment.SyncRoot); #pragma warning restore 0618 } } internal override void Aborted( InternalEnlistment enlistment, Exception e ) { Monitor.Exit( enlistment.SyncRoot ); try { enlistment.PromotedEnlistment.Aborted( e ); } finally { #pragma warning disable 0618 //@ Monitor.Enter(enlistment.SyncRoot); #pragma warning restore 0618 } } internal override void InDoubt( InternalEnlistment enlistment, Exception e ) { Monitor.Exit( enlistment.SyncRoot ); try { enlistment.PromotedEnlistment.InDoubt( e ); } finally { #pragma warning disable 0618 //@ Monitor.Enter(enlistment.SyncRoot); #pragma warning restore 0618 } } internal override byte[] RecoveryInformation( InternalEnlistment enlistment ) { Monitor.Exit( enlistment.SyncRoot ); try { return enlistment.PromotedEnlistment.GetRecoveryInformation(); } finally { #pragma warning disable 0618 //@ Monitor.Enter(enlistment.SyncRoot); #pragma warning restore 0618 } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
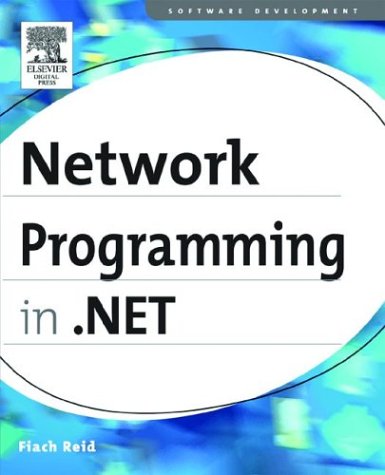
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeGenerator.cs
- ShaderEffect.cs
- ToolBarButtonDesigner.cs
- FrameworkContextData.cs
- SmtpSection.cs
- PKCS1MaskGenerationMethod.cs
- TimeSpanValidator.cs
- WebServicesInteroperability.cs
- PauseStoryboard.cs
- GridViewUpdatedEventArgs.cs
- srgsitem.cs
- PowerStatus.cs
- XmlParserContext.cs
- thaishape.cs
- PermissionToken.cs
- TextServicesManager.cs
- XhtmlBasicPageAdapter.cs
- WebPartDisplayModeCancelEventArgs.cs
- MultipleViewPatternIdentifiers.cs
- SchemaSetCompiler.cs
- EventPropertyMap.cs
- KnownBoxes.cs
- ProcessModuleCollection.cs
- NetNamedPipeBindingElement.cs
- ToolStripTextBox.cs
- VirtualPathProvider.cs
- RadialGradientBrush.cs
- CellTreeNodeVisitors.cs
- KeyPressEvent.cs
- MetadataArtifactLoaderCompositeResource.cs
- Utilities.cs
- IntSecurity.cs
- RawStylusSystemGestureInputReport.cs
- ResourceProviderFactory.cs
- TypeLoadException.cs
- RectangleGeometry.cs
- XsltException.cs
- EditorAttribute.cs
- EventEntry.cs
- CompilationUtil.cs
- NumericExpr.cs
- UnsafeNativeMethodsTablet.cs
- PackWebRequestFactory.cs
- PersonalizationAdministration.cs
- TextElementEnumerator.cs
- DesignerActionListCollection.cs
- XPathExpr.cs
- ContextQuery.cs
- RawMouseInputReport.cs
- EllipseGeometry.cs
- BinHexEncoder.cs
- TextFormatterImp.cs
- StoreItemCollection.Loader.cs
- DataSvcMapFileSerializer.cs
- StackBuilderSink.cs
- ButtonAutomationPeer.cs
- ManifestResourceInfo.cs
- CollectionConverter.cs
- Label.cs
- FtpWebResponse.cs
- XmlEnumAttribute.cs
- ScrollEvent.cs
- CustomError.cs
- AtomicFile.cs
- WebBrowserSiteBase.cs
- AnnotationMap.cs
- SqlTypesSchemaImporter.cs
- FileDialog.cs
- Rectangle.cs
- HyperlinkAutomationPeer.cs
- PageAdapter.cs
- HwndStylusInputProvider.cs
- SaveFileDialog.cs
- __TransparentProxy.cs
- TextDecorationCollection.cs
- CatalogZone.cs
- ResetableIterator.cs
- FrameworkTemplate.cs
- UIElement3DAutomationPeer.cs
- BinaryObjectWriter.cs
- TimeSpanValidator.cs
- SynchronizedInputProviderWrapper.cs
- TableRow.cs
- ProfileEventArgs.cs
- AsyncPostBackErrorEventArgs.cs
- DecoratedNameAttribute.cs
- StackBuilderSink.cs
- LockCookie.cs
- SchemaLookupTable.cs
- Quaternion.cs
- TextDpi.cs
- ChannelManagerHelpers.cs
- RemoteWebConfigurationHost.cs
- XmlSchemaAttribute.cs
- BasicCellRelation.cs
- ResolvePPIDRequest.cs
- ScriptingProfileServiceSection.cs
- ComponentManagerBroker.cs
- StringPropertyBuilder.cs
- DataGridLength.cs