Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / CompMod / System / CodeDOM / Compiler / CompilerResults.cs / 1 / CompilerResults.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.CodeDom.Compiler { using System; using System.CodeDom; using System.Reflection; using System.Collections; using System.Collections.Specialized; using System.Security; using System.Security.Permissions; using System.Security.Policy; using System.Runtime.Serialization; using System.Runtime.Serialization.Formatters.Binary; using System.IO; ////// [Serializable()] [PermissionSet(SecurityAction.InheritanceDemand, Name="FullTrust")] public class CompilerResults { private CompilerErrorCollection errors = new CompilerErrorCollection(); private StringCollection output = new StringCollection(); private Assembly compiledAssembly; private string pathToAssembly; private int nativeCompilerReturnValue; private TempFileCollection tempFiles; private Evidence evidence; ////// Represents the results /// of compilation from the compiler. /// ////// [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] public CompilerResults(TempFileCollection tempFiles) { this.tempFiles = tempFiles; } ////// Initializes a new instance of ////// that uses the specified /// temporary files. /// /// public TempFileCollection TempFiles { [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] get { return tempFiles; } [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] set { tempFiles = value; } } ////// Gets or sets the temporary files to use. /// ////// public Evidence Evidence { [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] get { Evidence e = null; if (evidence != null) e = CloneEvidence(evidence); return e; } [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] [SecurityPermissionAttribute( SecurityAction.Demand, ControlEvidence = true )] set { if (value != null) evidence = CloneEvidence(value); else evidence = null; } } ////// Set the evidence for partially trusted scenarios. /// ////// public Assembly CompiledAssembly { [SecurityPermissionAttribute(SecurityAction.Assert, Flags=SecurityPermissionFlag.ControlEvidence)] get { if (compiledAssembly == null && pathToAssembly != null) { AssemblyName assemName = new AssemblyName(); assemName.CodeBase = pathToAssembly; compiledAssembly = Assembly.Load(assemName,evidence); } return compiledAssembly; } [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] set { compiledAssembly = value; } } ////// The compiled assembly. /// ////// public CompilerErrorCollection Errors { get { return errors; } } ////// Gets or sets the collection of compiler errors. /// ////// public StringCollection Output { [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] get { return output; } } ////// Gets or sets the compiler output messages. /// ////// public string PathToAssembly { [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] get { return pathToAssembly; } [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] set { pathToAssembly = value; } } ////// Gets or sets the path to the assembly. /// ////// public int NativeCompilerReturnValue { get { return nativeCompilerReturnValue; } [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] set { nativeCompilerReturnValue = value; } } internal static Evidence CloneEvidence(Evidence ev) { new PermissionSet( PermissionState.Unrestricted ).Assert(); MemoryStream stream = new MemoryStream(); BinaryFormatter formatter = new BinaryFormatter(); formatter.Serialize( stream, ev ); stream.Position = 0; return (Evidence)formatter.Deserialize( stream ); } } }/// Gets or sets the compiler's return value. /// ///
Link Menu
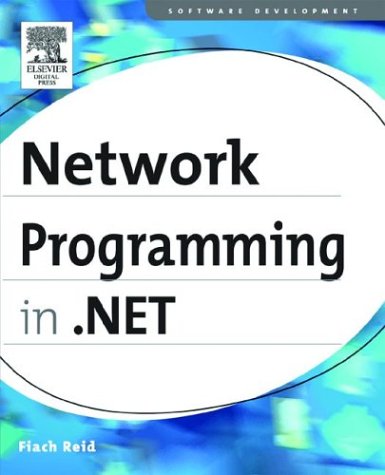
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BezierSegment.cs
- NameValueSectionHandler.cs
- ConfigurationLocation.cs
- PropertyPushdownHelper.cs
- UIntPtr.cs
- XmlNodeComparer.cs
- HwndSourceKeyboardInputSite.cs
- OSFeature.cs
- TemplateInstanceAttribute.cs
- oledbmetadatacolumnnames.cs
- XPathItem.cs
- SqlRecordBuffer.cs
- ObjectDataSource.cs
- CacheMemory.cs
- X509Certificate2Collection.cs
- RtfToXamlLexer.cs
- SqlGatherConsumedAliases.cs
- EntityDataSourceWrapperCollection.cs
- Converter.cs
- StateManagedCollection.cs
- BaseResourcesBuildProvider.cs
- Vector3DValueSerializer.cs
- ScrollContentPresenter.cs
- FilteredSchemaElementLookUpTable.cs
- WebPartAuthorizationEventArgs.cs
- ArrayListCollectionBase.cs
- XmlSchemaComplexContent.cs
- FileLoadException.cs
- Item.cs
- CommentAction.cs
- DisplayInformation.cs
- DataGridViewCellConverter.cs
- GeometryGroup.cs
- AnimationStorage.cs
- SuppressMergeCheckAttribute.cs
- DialogResultConverter.cs
- TypeToken.cs
- QueryableDataSourceView.cs
- _KerberosClient.cs
- GenericsNotImplementedException.cs
- Int32Rect.cs
- QilTypeChecker.cs
- TableProvider.cs
- RemoveStoryboard.cs
- RenderingBiasValidation.cs
- Drawing.cs
- Executor.cs
- SqlStatistics.cs
- MultiAsyncResult.cs
- String.cs
- DataGridViewCellStyleConverter.cs
- ConnectionPoolRegistry.cs
- ParameterCollection.cs
- PropertyMap.cs
- InfoCardHelper.cs
- ChangeNode.cs
- Vertex.cs
- SafeNativeMemoryHandle.cs
- TimeSpanValidator.cs
- DropShadowEffect.cs
- CheckPair.cs
- Registry.cs
- DictionarySectionHandler.cs
- BitVec.cs
- HostingEnvironmentSection.cs
- InsufficientMemoryException.cs
- CodeDesigner.cs
- TypeReference.cs
- MessagePropertyVariants.cs
- StringToken.cs
- AttributedMetaModel.cs
- DecoderFallbackWithFailureFlag.cs
- FixedLineResult.cs
- Subset.cs
- WindowsRegion.cs
- InternalSafeNativeMethods.cs
- ErrorWebPart.cs
- CompleteWizardStep.cs
- PageCanvasSize.cs
- WaitForChangedResult.cs
- MiniMapControl.xaml.cs
- PrintingPermission.cs
- DesignerAttribute.cs
- ErasingStroke.cs
- ColorMatrix.cs
- SqlConnectionPoolProviderInfo.cs
- SelectionRangeConverter.cs
- PropertyGeneratedEventArgs.cs
- CodeDirectoryCompiler.cs
- DataGridViewColumnEventArgs.cs
- UrlAuthFailedErrorFormatter.cs
- _Events.cs
- OperationResponse.cs
- DataGridViewSelectedRowCollection.cs
- HtmlUtf8RawTextWriter.cs
- WsdlInspector.cs
- StreamSecurityUpgradeAcceptorBase.cs
- XmlNodeReader.cs
- DSASignatureDeformatter.cs
- ProfilePropertySettings.cs