Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Print / Reach / PrintConfig / PageCanvasSize.cs / 1 / PageCanvasSize.cs
/*++ Copyright (C) 2003 Microsoft Corporation All rights reserved. Module Name: PageCanvasSize.cs Abstract: Definition and implementation of this public property type. Author: [....] ([....]) 8/22/2003 --*/ using System; using System.IO; using System.Xml; using System.Collections; using System.Diagnostics; using System.Printing; using MS.Internal.Printing.Configuration; #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages namespace MS.Internal.Printing.Configuration { ////// Specifies the imageable area of the canvas. /// internal class CanvasImageableArea { #region Constructors internal CanvasImageableArea(ImageableSizeCapability ownerProperty) { this._ownerProperty = ownerProperty; _originWidth = _originHeight = _extentWidth = _extentHeight = PrintSchema.UnspecifiedIntValue; } #endregion Constructors #region Public Properties ////// Specifies the horizontal origin of the imageable area relative to the application media size, in 1/96 inch unit. /// public double OriginWidth { get { return UnitConverter.LengthValueFromMicronToDIP(_originWidth); } } ////// Specifies the vertical origin of the imageable area relative to the application media size, in 1/96 inch unit. /// public double OriginHeight { get { return UnitConverter.LengthValueFromMicronToDIP(_originHeight); } } ////// Specifies the horizontal distance between the origin and /// the bounding limit of the application media size, in 1/96 inch unit. /// public double ExtentWidth { get { return UnitConverter.LengthValueFromMicronToDIP(_extentWidth); } } ////// Specifies the vertical distance between the origin and /// the bounding limit of the application media size, in 1/96 inch unit. /// public double ExtentHeight { get { return UnitConverter.LengthValueFromMicronToDIP(_extentHeight); } } #endregion Public Properties #region Public Methods ////// Converts the imageable area to human-readable string. /// ///A string that represents this imageable area. public override string ToString() { return "[ImageableArea: Origin (" + OriginWidth + "," + OriginHeight + "), Extent (" + ExtentWidth + "x" + ExtentHeight + ")]"; } #endregion Public Methods #region Internal Fields internal ImageableSizeCapability _ownerProperty; // Integer values are always in micron unit internal int _originWidth; internal int _originHeight; internal int _extentWidth; internal int _extentHeight; #endregion Internal Fields } ////// Represents imageable size capability. /// internal class ImageableSizeCapability : PrintCapabilityRootProperty { #region Constructors internal ImageableSizeCapability() : base() { _imageableSizeWidth = _imageableSizeHeight = PrintSchema.UnspecifiedIntValue; } #endregion Constructors #region Public Properties ////// Specifies the horizontal dimension of application media size relative to the PageOrientation, in 1/96 inch unit. /// public double ImageableSizeWidth { get { return UnitConverter.LengthValueFromMicronToDIP(_imageableSizeWidth); } } ////// Specifies the vertical dimension of the application media size relative to the PageOrientation, in 1/96 inch unit. /// public double ImageableSizeHeight { get { return UnitConverter.LengthValueFromMicronToDIP(_imageableSizeHeight); } } ////// Specifies the imageable area of the canvas. /// public CanvasImageableArea ImageableArea { get { return _imageableArea; } } #endregion Public Properties #region Public Methods ////// Converts the imageable size capability object to human-readable string. /// ///A string that represents this imageable size capability object. public override string ToString() { return "ImageableSizeWidth=" + ImageableSizeWidth + ", ImageableSizeHeight=" + ImageableSizeHeight + " " + ((ImageableArea != null) ? ImageableArea.ToString() : "[ImageableArea: null]"); } #endregion Public Methods #region Internal Methods ///XML parser finds non-well-formness of XML internal override sealed bool BuildProperty(XmlPrintCapReader reader) { #if _DEBUG Trace.Assert(reader.CurrentElementNodeType == PrintSchemaNodeTypes.Property, "THIS SHOULD NOT HAPPEN: RootPropertyPropCallback gets non-Property node"); #endif int subPropDepth = reader.CurrentElementDepth + 1; // Loops over immediate property children of the root-level property while (reader.MoveToNextSchemaElement(subPropDepth, PrintSchemaNodeTypes.Property)) { if (reader.CurrentElementNameAttrValue == PrintSchemaTags.Keywords.PageImageableSizeKeys.ImageableSizeWidth) { try { this._imageableSizeWidth = reader.GetCurrentPropertyIntValueWithException(); } // We want to catch internal FormatException to skip recoverable XML content syntax error #pragma warning suppress 56502 #if _DEBUG catch (FormatException e) #else catch (FormatException) #endif { #if _DEBUG Trace.WriteLine("-Error- " + e.Message); #endif } } else if (reader.CurrentElementNameAttrValue == PrintSchemaTags.Keywords.PageImageableSizeKeys.ImageableSizeHeight) { try { this._imageableSizeHeight = reader.GetCurrentPropertyIntValueWithException(); } // We want to catch internal FormatException to skip recoverable XML content syntax error #pragma warning suppress 56502 #if _DEBUG catch (FormatException e) #else catch (FormatException) #endif { #if _DEBUG Trace.WriteLine("-Error- " + e.Message); #endif } } else if (reader.CurrentElementNameAttrValue == PrintSchemaTags.Keywords.PageImageableSizeKeys.ImageableArea) { this._imageableArea = new CanvasImageableArea(this); // When need to loop at a deeper depth, the code should cache the desired depth // value and use the cached value in the while-loop. Using CurrentElementDepth // in while-loop won't work correctly since the CurrentElementDepth value is changing. int iaPropDepth = reader.CurrentElementDepth + 1; // loop over one level down to read "ImageableArea" child-element properties while (reader.MoveToNextSchemaElement(iaPropDepth, PrintSchemaNodeTypes.Property)) { if (reader.CurrentElementNameAttrValue == PrintSchemaTags.Keywords.PageImageableSizeKeys.OriginWidth) { try { this._imageableArea._originWidth = reader.GetCurrentPropertyIntValueWithException(); } // We want to catch internal FormatException to skip recoverable XML content syntax error #pragma warning suppress 56502 #if _DEBUG catch (FormatException e) #else catch (FormatException) #endif { #if _DEBUG Trace.WriteLine("-Error- " + e.Message); #endif } } else if (reader.CurrentElementNameAttrValue == PrintSchemaTags.Keywords.PageImageableSizeKeys.OriginHeight) { try { this._imageableArea._originHeight = reader.GetCurrentPropertyIntValueWithException(); } // We want to catch internal FormatException to skip recoverable XML content syntax error #pragma warning suppress 56502 #if _DEBUG catch (FormatException e) #else catch (FormatException) #endif { #if _DEBUG Trace.WriteLine("-Error- " + e.Message); #endif } } else if (reader.CurrentElementNameAttrValue == PrintSchemaTags.Keywords.PageImageableSizeKeys.ExtentWidth) { try { this._imageableArea._extentWidth = reader.GetCurrentPropertyIntValueWithException(); } // We want to catch internal FormatException to skip recoverable XML content syntax error #pragma warning suppress 56502 #if _DEBUG catch (FormatException e) #else catch (FormatException) #endif { #if _DEBUG Trace.WriteLine ("-Error- " + e.Message); #endif } } else if (reader.CurrentElementNameAttrValue == PrintSchemaTags.Keywords.PageImageableSizeKeys.ExtentHeight) { try { this._imageableArea._extentHeight = reader.GetCurrentPropertyIntValueWithException(); } // We want to catch internal FormatException to skip recoverable XML content syntax error #pragma warning suppress 56502 #if _DEBUG catch (FormatException e) #else catch (FormatException) #endif { #if _DEBUG Trace.WriteLine ("-Error- " + e.Message); #endif } } else { #if _DEBUG Trace.WriteLine("-Warning- skip unknown ImageableArea sub-property '" + reader.CurrentElementNameAttrValue + "' at line " + reader._xmlReader.LineNumber + ", position " + reader._xmlReader.LinePosition); #endif } } } else { #if _DEBUG Trace.WriteLine("-Warning- skip unknown PageImageableSize sub-Property '" + reader.CurrentElementNameAttrValue + "' at line " + reader._xmlReader.LineNumber + ", position " + reader._xmlReader.LinePosition); #endif } } bool isValid = false; // We require ImageableSizeWidth/Height and ExtentWidth/Height values must be non-negative if ((this._imageableSizeWidth >= 0) && (this._imageableSizeHeight >= 0)) { isValid = true; // If ImageableArea is present, then its ExtentWidth/Height values must be non-negative. if (this.ImageableArea != null) { isValid = false; if ((this.ImageableArea._extentWidth >= 0) && (this.ImageableArea._extentHeight >= 0)) { isValid = true; } } } else { #if _DEBUG Trace.WriteLine("-Error- invalid PageImageableSize size values: " + this.ToString()); #endif } return isValid; } #endregion Internal Methods #region Internal Fields internal int _imageableSizeWidth; internal int _imageableSizeHeight; internal CanvasImageableArea _imageableArea; #endregion Internal Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
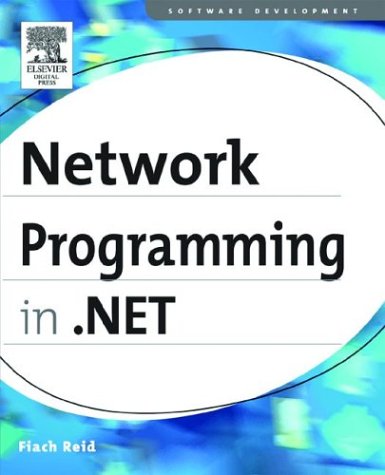
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BuildProviderAppliesToAttribute.cs
- UIElementParagraph.cs
- JournalEntryStack.cs
- ParameterBuilder.cs
- BezierSegment.cs
- DictionaryMarkupSerializer.cs
- XPathPatternParser.cs
- StringConverter.cs
- DataGridView.cs
- LayoutTable.cs
- LayoutTable.cs
- PlanCompiler.cs
- RepeaterCommandEventArgs.cs
- FixedPageProcessor.cs
- SecurityChannelFaultConverter.cs
- DataRowExtensions.cs
- Attributes.cs
- EventMappingSettings.cs
- PerformanceCounterPermission.cs
- SourceSwitch.cs
- TextDocumentView.cs
- PageThemeCodeDomTreeGenerator.cs
- NavigationProgressEventArgs.cs
- prompt.cs
- PersonalizableAttribute.cs
- Style.cs
- RegexStringValidatorAttribute.cs
- WebPartsPersonalizationAuthorization.cs
- Path.cs
- XmlNamespaceMapping.cs
- LinqDataSourceContextEventArgs.cs
- BamlWriter.cs
- CodeGeneratorOptions.cs
- ListViewCancelEventArgs.cs
- EdgeModeValidation.cs
- SqlOuterApplyReducer.cs
- _RequestCacheProtocol.cs
- Transform.cs
- UnsafeNativeMethods.cs
- CodeLabeledStatement.cs
- WebPartManagerInternals.cs
- PtsCache.cs
- ToolStripTextBox.cs
- DbException.cs
- CollectionViewGroupInternal.cs
- NavigateEvent.cs
- PolicyException.cs
- HandlerFactoryWrapper.cs
- ProfileGroupSettings.cs
- SoapObjectInfo.cs
- HttpWriter.cs
- StorageSetMapping.cs
- PeerApplicationLaunchInfo.cs
- RelationshipDetailsRow.cs
- TypeForwardedToAttribute.cs
- ReadWriteSpinLock.cs
- Codec.cs
- PageThemeCodeDomTreeGenerator.cs
- InputLanguageCollection.cs
- ResXResourceSet.cs
- Vars.cs
- HyperLinkStyle.cs
- WindowsFormsHelpers.cs
- TimelineClockCollection.cs
- Ref.cs
- CustomPopupPlacement.cs
- TraceUtils.cs
- XmlSchemas.cs
- TypeConverters.cs
- FixedSOMElement.cs
- InputScope.cs
- CapabilitiesPattern.cs
- SchemaImporterExtensionsSection.cs
- DrawingContextWalker.cs
- BindingContext.cs
- sitestring.cs
- documentsequencetextcontainer.cs
- HttpWebRequest.cs
- WebPartEditVerb.cs
- VectorAnimationBase.cs
- ChtmlTextWriter.cs
- SettingsPropertyCollection.cs
- NoneExcludedImageIndexConverter.cs
- LiteralControl.cs
- _IPv4Address.cs
- ProxyHelper.cs
- ConfigurationElementCollection.cs
- ButtonBase.cs
- LocalizabilityAttribute.cs
- ConfigurationStrings.cs
- WebExceptionStatus.cs
- FocusManager.cs
- GridItemProviderWrapper.cs
- ResourceProperty.cs
- ProgressBarAutomationPeer.cs
- UnaryNode.cs
- WindowsGraphics2.cs
- FirstQueryOperator.cs
- MoveSizeWinEventHandler.cs
- DirtyTextRange.cs