Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / MS / Internal / Utility / MonitorWrapper.cs / 1305600 / MonitorWrapper.cs
//---------------------------------------------------------------------------- // //// Copyright (C) 2005 by Microsoft Corporation. All rights reserved. // // // // Description: Wraps System.Threading.Monitor and adds a busy flag // //--------------------------------------------------------------------------- using System; using System.Threading; using System.Windows; using MS.Internal; namespace MS.Internal.Utility { ////// Monitor with Busy flag while it is entered. /// internal class MonitorWrapper { public IDisposable Enter() { Monitor.Enter(_syncRoot); Interlocked.Increment(ref _enterCount); return new MonitorHelper(this); } public void Exit() { int count = Interlocked.Decrement(ref _enterCount); Invariant.Assert(count >= 0, "unmatched call to MonitorWrapper.Exit"); Monitor.Exit(_syncRoot); } public bool Busy { get { return (_enterCount > 0); } } int _enterCount; object _syncRoot = new object(); private class MonitorHelper : IDisposable { public MonitorHelper(MonitorWrapper monitorWrapper) { _monitorWrapper = monitorWrapper; } public void Dispose() { if (_monitorWrapper != null) { _monitorWrapper.Exit(); _monitorWrapper = null; } GC.SuppressFinalize(this); } private MonitorWrapper _monitorWrapper; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) 2005 by Microsoft Corporation. All rights reserved. // // // // Description: Wraps System.Threading.Monitor and adds a busy flag // //--------------------------------------------------------------------------- using System; using System.Threading; using System.Windows; using MS.Internal; namespace MS.Internal.Utility { ////// Monitor with Busy flag while it is entered. /// internal class MonitorWrapper { public IDisposable Enter() { Monitor.Enter(_syncRoot); Interlocked.Increment(ref _enterCount); return new MonitorHelper(this); } public void Exit() { int count = Interlocked.Decrement(ref _enterCount); Invariant.Assert(count >= 0, "unmatched call to MonitorWrapper.Exit"); Monitor.Exit(_syncRoot); } public bool Busy { get { return (_enterCount > 0); } } int _enterCount; object _syncRoot = new object(); private class MonitorHelper : IDisposable { public MonitorHelper(MonitorWrapper monitorWrapper) { _monitorWrapper = monitorWrapper; } public void Dispose() { if (_monitorWrapper != null) { _monitorWrapper.Exit(); _monitorWrapper = null; } GC.SuppressFinalize(this); } private MonitorWrapper _monitorWrapper; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
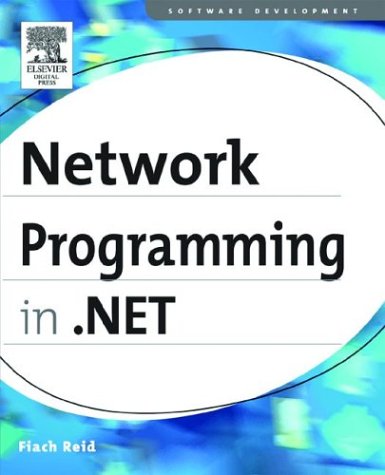
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CreatingCookieEventArgs.cs
- NetTcpBindingCollectionElement.cs
- RecognizerStateChangedEventArgs.cs
- RegexCapture.cs
- Processor.cs
- NetworkCredential.cs
- FrameDimension.cs
- CodeDomConfigurationHandler.cs
- SafeLibraryHandle.cs
- BufferedReadStream.cs
- DocumentGrid.cs
- WebDescriptionAttribute.cs
- ButtonRenderer.cs
- CodeGotoStatement.cs
- UnknownWrapper.cs
- MappingException.cs
- Journaling.cs
- MSAAEventDispatcher.cs
- SigningProgress.cs
- MetadataPropertyvalue.cs
- Expressions.cs
- ReadOnlyCollectionBase.cs
- WCFBuildProvider.cs
- ITextView.cs
- DockingAttribute.cs
- TransactionTable.cs
- Listbox.cs
- ExtendedPropertyDescriptor.cs
- SqlServices.cs
- PasswordTextNavigator.cs
- TriState.cs
- __Filters.cs
- InvokeWebServiceDesigner.cs
- ProviderMetadataCachedInformation.cs
- RangeValidator.cs
- OciEnlistContext.cs
- TabControlCancelEvent.cs
- UnmanagedMemoryStream.cs
- CollectionViewGroup.cs
- SelfIssuedAuthRSAPKCS1SignatureDeformatter.cs
- MimeWriter.cs
- ProcessModelSection.cs
- XmlObjectSerializerWriteContextComplexJson.cs
- DataGridViewEditingControlShowingEventArgs.cs
- SchemaNamespaceManager.cs
- DesignerAutoFormatCollection.cs
- KnowledgeBase.cs
- PenContexts.cs
- CompareInfo.cs
- ImageFormat.cs
- GridViewAutomationPeer.cs
- DelegateHelpers.Generated.cs
- FontUnitConverter.cs
- XmlSchemaElement.cs
- DBSchemaRow.cs
- ExceptionUtil.cs
- SoapWriter.cs
- ConfigurationElementProperty.cs
- RoleManagerSection.cs
- BaseAddressElementCollection.cs
- FixedSOMPage.cs
- SiteMapProvider.cs
- XmlUtf8RawTextWriter.cs
- DynamicValidatorEventArgs.cs
- ZipFileInfoCollection.cs
- _ContextAwareResult.cs
- NoClickablePointException.cs
- WindowsIPAddress.cs
- ModifiableIteratorCollection.cs
- XmlReaderSettings.cs
- WebPartPersonalization.cs
- SchemaObjectWriter.cs
- DataGridViewRowErrorTextNeededEventArgs.cs
- StaticResourceExtension.cs
- validationstate.cs
- FactoryMaker.cs
- NamespaceMapping.cs
- ProfileServiceManager.cs
- AssemblyInfo.cs
- IISUnsafeMethods.cs
- SecurityRequiresReviewAttribute.cs
- SafeEventLogReadHandle.cs
- SpellerInterop.cs
- TemplateField.cs
- HierarchicalDataBoundControl.cs
- QueryStringHandler.cs
- ApplicationException.cs
- VirtualizingStackPanel.cs
- Button.cs
- SerializationSectionGroup.cs
- AsmxEndpointPickerExtension.cs
- SchemaNamespaceManager.cs
- WmlLiteralTextAdapter.cs
- QuaternionAnimationUsingKeyFrames.cs
- DataTableTypeConverter.cs
- EntityDataSourceUtil.cs
- Int32EqualityComparer.cs
- basecomparevalidator.cs
- VisualTreeHelper.cs
- HMACSHA384.cs