Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / CompMod / System / ComponentModel / ExtendedPropertyDescriptor.cs / 1 / ExtendedPropertyDescriptor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel { using Microsoft.Win32; using System; using System.Collections; using System.ComponentModel.Design; using System.Diagnostics; using System.Security.Permissions; ////// /// [HostProtection(SharedState = true)] internal sealed class ExtendedPropertyDescriptor : PropertyDescriptor { private readonly ReflectPropertyDescriptor extenderInfo; // the extender property private readonly IExtenderProvider provider; // the guy providing it ////// This class wraps an PropertyDescriptor with something that looks like a property. It /// allows you to treat extended properties the same as regular properties. /// ////// Creates a new extended property info. Callers can then treat this as /// a standard property. /// public ExtendedPropertyDescriptor(ReflectPropertyDescriptor extenderInfo, Type receiverType, IExtenderProvider provider, Attribute[] attributes) : base(extenderInfo, attributes) { Debug.Assert(extenderInfo != null, "ExtendedPropertyDescriptor must have extenderInfo"); Debug.Assert(provider != null, "ExtendedPropertyDescriptor must have provider"); ArrayList attrList = new ArrayList(AttributeArray); attrList.Add(ExtenderProvidedPropertyAttribute.Create(extenderInfo, receiverType, provider)); if (extenderInfo.IsReadOnly) { attrList.Add(ReadOnlyAttribute.Yes); } Attribute[] temp = new Attribute[attrList.Count]; attrList.CopyTo(temp, 0); AttributeArray = temp; this.extenderInfo = extenderInfo; this.provider = provider; } public ExtendedPropertyDescriptor(PropertyDescriptor extender, Attribute[] attributes) : base(extender, attributes) { Debug.Assert(extender != null, "The original PropertyDescriptor must be non-null"); ExtenderProvidedPropertyAttribute attr = extender.Attributes[typeof(ExtenderProvidedPropertyAttribute)] as ExtenderProvidedPropertyAttribute; Debug.Assert(attr != null, "The original PropertyDescriptor does not have an ExtenderProvidedPropertyAttribute"); ReflectPropertyDescriptor reflectDesc = attr.ExtenderProperty as ReflectPropertyDescriptor; Debug.Assert(reflectDesc != null, "The original PropertyDescriptor has an invalid ExtenderProperty"); this.extenderInfo = reflectDesc; this.provider = attr.Provider; } ////// Determines if the the component will allow its value to be reset. /// public override bool CanResetValue(object comp) { return extenderInfo.ExtenderCanResetValue(provider, comp); } ////// Retrieves the type of the component this PropertyDescriptor is bound to. /// public override Type ComponentType { get { return extenderInfo.ComponentType; } } ////// Determines if the property can be written to. /// public override bool IsReadOnly { get { return Attributes[typeof(ReadOnlyAttribute)].Equals(ReadOnlyAttribute.Yes); } } ////// Retrieves the data type of the property. /// public override Type PropertyType { get { return extenderInfo.ExtenderGetType(provider); } } ////// Retrieves the display name of the property. This is the name that will /// be displayed in a properties window. This will be the same as the property /// name for most properties. /// public override string DisplayName { get { string name = base.DisplayName; DisplayNameAttribute displayNameAttr = Attributes[typeof(DisplayNameAttribute)] as DisplayNameAttribute; if (displayNameAttr == null || displayNameAttr.IsDefaultAttribute()) { ISite site = GetSite(provider); if (site != null) { string providerName = site.Name; if (providerName != null && providerName.Length > 0) { name = SR.GetString(SR.MetaExtenderName, name, providerName); } } } return name; } } ////// Retrieves the value of the property for the given component. This will /// throw an exception if the component does not have this property. /// public override object GetValue(object comp) { return extenderInfo.ExtenderGetValue(provider, comp); } ////// Resets the value of this property on comp to the default value. /// public override void ResetValue(object comp) { extenderInfo.ExtenderResetValue(provider, comp, this); } ////// Sets the value of this property on the given component. /// public override void SetValue(object component, object value) { extenderInfo.ExtenderSetValue(provider, component, value, this); } ////// Determines if this property should be persisted. A property is /// to be persisted if it is marked as persistable through a /// PersistableAttribute, and if the property contains something other /// than the default value. Note, however, that this method will /// return true for design time properties as well, so callers /// should also check to see if a property is design time only before /// persisting to runtime storage. /// public override bool ShouldSerializeValue(object comp) { return extenderInfo.ExtenderShouldSerializeValue(provider, comp); } /* The following code has been removed to fix FXCOP violations. The code is left here incase it needs to be resurrected in the future. ////// Creates a new extended property info. Callers can then treat this as /// a standard property. /// public ExtendedPropertyDescriptor(ReflectPropertyDescriptor extenderInfo, Type receiverType, IExtenderProvider provider) : this(extenderInfo, receiverType, provider, null) { } ////// Retrieves the object that is providing this extending property. /// public IExtenderProvider Provider { get { return provider; } } */ } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel { using Microsoft.Win32; using System; using System.Collections; using System.ComponentModel.Design; using System.Diagnostics; using System.Security.Permissions; ////// /// [HostProtection(SharedState = true)] internal sealed class ExtendedPropertyDescriptor : PropertyDescriptor { private readonly ReflectPropertyDescriptor extenderInfo; // the extender property private readonly IExtenderProvider provider; // the guy providing it ////// This class wraps an PropertyDescriptor with something that looks like a property. It /// allows you to treat extended properties the same as regular properties. /// ////// Creates a new extended property info. Callers can then treat this as /// a standard property. /// public ExtendedPropertyDescriptor(ReflectPropertyDescriptor extenderInfo, Type receiverType, IExtenderProvider provider, Attribute[] attributes) : base(extenderInfo, attributes) { Debug.Assert(extenderInfo != null, "ExtendedPropertyDescriptor must have extenderInfo"); Debug.Assert(provider != null, "ExtendedPropertyDescriptor must have provider"); ArrayList attrList = new ArrayList(AttributeArray); attrList.Add(ExtenderProvidedPropertyAttribute.Create(extenderInfo, receiverType, provider)); if (extenderInfo.IsReadOnly) { attrList.Add(ReadOnlyAttribute.Yes); } Attribute[] temp = new Attribute[attrList.Count]; attrList.CopyTo(temp, 0); AttributeArray = temp; this.extenderInfo = extenderInfo; this.provider = provider; } public ExtendedPropertyDescriptor(PropertyDescriptor extender, Attribute[] attributes) : base(extender, attributes) { Debug.Assert(extender != null, "The original PropertyDescriptor must be non-null"); ExtenderProvidedPropertyAttribute attr = extender.Attributes[typeof(ExtenderProvidedPropertyAttribute)] as ExtenderProvidedPropertyAttribute; Debug.Assert(attr != null, "The original PropertyDescriptor does not have an ExtenderProvidedPropertyAttribute"); ReflectPropertyDescriptor reflectDesc = attr.ExtenderProperty as ReflectPropertyDescriptor; Debug.Assert(reflectDesc != null, "The original PropertyDescriptor has an invalid ExtenderProperty"); this.extenderInfo = reflectDesc; this.provider = attr.Provider; } ////// Determines if the the component will allow its value to be reset. /// public override bool CanResetValue(object comp) { return extenderInfo.ExtenderCanResetValue(provider, comp); } ////// Retrieves the type of the component this PropertyDescriptor is bound to. /// public override Type ComponentType { get { return extenderInfo.ComponentType; } } ////// Determines if the property can be written to. /// public override bool IsReadOnly { get { return Attributes[typeof(ReadOnlyAttribute)].Equals(ReadOnlyAttribute.Yes); } } ////// Retrieves the data type of the property. /// public override Type PropertyType { get { return extenderInfo.ExtenderGetType(provider); } } ////// Retrieves the display name of the property. This is the name that will /// be displayed in a properties window. This will be the same as the property /// name for most properties. /// public override string DisplayName { get { string name = base.DisplayName; DisplayNameAttribute displayNameAttr = Attributes[typeof(DisplayNameAttribute)] as DisplayNameAttribute; if (displayNameAttr == null || displayNameAttr.IsDefaultAttribute()) { ISite site = GetSite(provider); if (site != null) { string providerName = site.Name; if (providerName != null && providerName.Length > 0) { name = SR.GetString(SR.MetaExtenderName, name, providerName); } } } return name; } } ////// Retrieves the value of the property for the given component. This will /// throw an exception if the component does not have this property. /// public override object GetValue(object comp) { return extenderInfo.ExtenderGetValue(provider, comp); } ////// Resets the value of this property on comp to the default value. /// public override void ResetValue(object comp) { extenderInfo.ExtenderResetValue(provider, comp, this); } ////// Sets the value of this property on the given component. /// public override void SetValue(object component, object value) { extenderInfo.ExtenderSetValue(provider, component, value, this); } ////// Determines if this property should be persisted. A property is /// to be persisted if it is marked as persistable through a /// PersistableAttribute, and if the property contains something other /// than the default value. Note, however, that this method will /// return true for design time properties as well, so callers /// should also check to see if a property is design time only before /// persisting to runtime storage. /// public override bool ShouldSerializeValue(object comp) { return extenderInfo.ExtenderShouldSerializeValue(provider, comp); } /* The following code has been removed to fix FXCOP violations. The code is left here incase it needs to be resurrected in the future. ////// Creates a new extended property info. Callers can then treat this as /// a standard property. /// public ExtendedPropertyDescriptor(ReflectPropertyDescriptor extenderInfo, Type receiverType, IExtenderProvider provider) : this(extenderInfo, receiverType, provider, null) { } ////// Retrieves the object that is providing this extending property. /// public IExtenderProvider Provider { get { return provider; } } */ } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
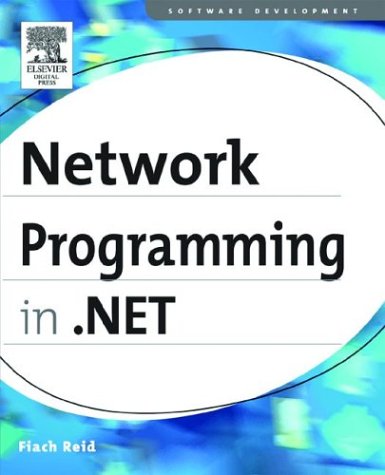
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GeneratedContractType.cs
- NamespaceList.cs
- ListenerConnectionModeReader.cs
- CodeTypeDeclarationCollection.cs
- RefreshEventArgs.cs
- PerformanceCounters.cs
- DecoderExceptionFallback.cs
- WebEncodingValidator.cs
- VersionedStreamOwner.cs
- WindowsSpinner.cs
- PerformanceCounterTraceRecord.cs
- SystemIPInterfaceStatistics.cs
- DataGridItemEventArgs.cs
- ItemDragEvent.cs
- KeySpline.cs
- WebServiceReceiveDesigner.cs
- Util.cs
- ApplicationFileCodeDomTreeGenerator.cs
- UnknownBitmapDecoder.cs
- NotImplementedException.cs
- Regex.cs
- HtmlTableCell.cs
- AnimationClock.cs
- LoadedOrUnloadedOperation.cs
- TracedNativeMethods.cs
- XPathAncestorQuery.cs
- ProvidePropertyAttribute.cs
- MetafileHeaderWmf.cs
- StylusTip.cs
- KoreanCalendar.cs
- BrowserCapabilitiesCodeGenerator.cs
- PeerEndPoint.cs
- WebControl.cs
- XslNumber.cs
- CoreSwitches.cs
- RowBinding.cs
- PriorityItem.cs
- ipaddressinformationcollection.cs
- ModelChangedEventArgsImpl.cs
- DiscoveryMessageSequenceGenerator.cs
- WebUtil.cs
- FunctionQuery.cs
- MethodAccessException.cs
- ExpressionTextBoxAutomationPeer.cs
- QuotedPrintableStream.cs
- ToolStripItemTextRenderEventArgs.cs
- UriTemplateCompoundPathSegment.cs
- OperationCanceledException.cs
- JsonFormatGeneratorStatics.cs
- UrlEncodedParameterWriter.cs
- RuntimeEnvironment.cs
- Preprocessor.cs
- UnsafeNativeMethods.cs
- rsa.cs
- CompositeCollection.cs
- SequenceDesigner.cs
- ClickablePoint.cs
- SchemaType.cs
- RuleInfoComparer.cs
- DependencyPropertyDescriptor.cs
- RegionIterator.cs
- WebPartAuthorizationEventArgs.cs
- VectorAnimationUsingKeyFrames.cs
- ContentElementAutomationPeer.cs
- SqlAliaser.cs
- ViewLoader.cs
- ManipulationStartedEventArgs.cs
- DoubleAnimationUsingKeyFrames.cs
- ReadOnlyNameValueCollection.cs
- TextTreeInsertElementUndoUnit.cs
- Renderer.cs
- ContractCodeDomInfo.cs
- TextBoxDesigner.cs
- HtmlFormWrapper.cs
- ApplicationCommands.cs
- ComboBoxItem.cs
- EntryIndex.cs
- XmlComplianceUtil.cs
- DesignerDataSourceView.cs
- InvalidAsynchronousStateException.cs
- PrimitiveXmlSerializers.cs
- DbgCompiler.cs
- BevelBitmapEffect.cs
- WebServiceResponseDesigner.cs
- ListViewInsertionMark.cs
- SegmentInfo.cs
- XmlEncodedRawTextWriter.cs
- log.cs
- BamlRecordWriter.cs
- DeploymentExceptionMapper.cs
- ReliableSession.cs
- TransactionChannelListener.cs
- WebBrowserNavigatedEventHandler.cs
- URLAttribute.cs
- basenumberconverter.cs
- ResponseBodyWriter.cs
- XmlAnyElementAttribute.cs
- ToolStripCodeDomSerializer.cs
- WindowsToolbarItemAsMenuItem.cs
- XmlSerializationWriter.cs