Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media3D / Generated / Scene3D.cs / 1 / Scene3D.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Text; using System.Windows.Markup; using System.Windows.Media.Media3D.Converters; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Windows.Media.Imaging; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media.Media3D { sealed partial class Scene3D : Animatable, DUCE.IResource { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new Scene3D Clone() { return (Scene3D)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new Scene3D CloneCurrentValue() { return (Scene3D)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- private static void ModelsPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { // The first change to the default value of a mutable collection property (e.g. GeometryGroup.Children) // will promote the property value from a default value to a local value. This is technically a sub-property // change because the collection was changed and not a new collection set (GeometryGroup.Children. // Add versus GeometryGroup.Children = myNewChildrenCollection). However, we never marshalled // the default value to the compositor. If the property changes from a default value, the new local value // needs to be marshalled to the compositor. We detect this scenario with the second condition // e.OldValueSource != e.NewValueSource. Specifically in this scenario the OldValueSource will be // Default and the NewValueSource will be Local. if (e.IsASubPropertyChange && (e.OldValueSource == e.NewValueSource)) { return; } Scene3D target = ((Scene3D) d); Model3DGroup oldV = (Model3DGroup) e.OldValue; Model3DGroup newV = (Model3DGroup) e.NewValue; System.Windows.Threading.Dispatcher dispatcher = target.Dispatcher; if (dispatcher != null) { DUCE.IResource targetResource = (DUCE.IResource)target; using (CompositionEngineLock.Acquire()) { int channelCount = targetResource.GetChannelCount(); for (int channelIndex = 0; channelIndex < channelCount; channelIndex++) { DUCE.Channel channel = targetResource.GetChannel(channelIndex); Debug.Assert(!channel.IsOutOfBandChannel); Debug.Assert(!targetResource.GetHandle(channel).IsNull); target.ReleaseResource(oldV,channel); target.AddRefResource(newV,channel); } } } target.PropertyChanged(ModelsProperty); } private static void CameraPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { // The first change to the default value of a mutable collection property (e.g. GeometryGroup.Children) // will promote the property value from a default value to a local value. This is technically a sub-property // change because the collection was changed and not a new collection set (GeometryGroup.Children. // Add versus GeometryGroup.Children = myNewChildrenCollection). However, we never marshalled // the default value to the compositor. If the property changes from a default value, the new local value // needs to be marshalled to the compositor. We detect this scenario with the second condition // e.OldValueSource != e.NewValueSource. Specifically in this scenario the OldValueSource will be // Default and the NewValueSource will be Local. if (e.IsASubPropertyChange && (e.OldValueSource == e.NewValueSource)) { return; } Scene3D target = ((Scene3D) d); Camera oldV = (Camera) e.OldValue; Camera newV = (Camera) e.NewValue; System.Windows.Threading.Dispatcher dispatcher = target.Dispatcher; if (dispatcher != null) { DUCE.IResource targetResource = (DUCE.IResource)target; using (CompositionEngineLock.Acquire()) { int channelCount = targetResource.GetChannelCount(); for (int channelIndex = 0; channelIndex < channelCount; channelIndex++) { DUCE.Channel channel = targetResource.GetChannel(channelIndex); Debug.Assert(!channel.IsOutOfBandChannel); Debug.Assert(!targetResource.GetHandle(channel).IsNull); target.ReleaseResource(oldV,channel); target.AddRefResource(newV,channel); } } } target.PropertyChanged(CameraProperty); } private static void ViewportPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { Scene3D target = ((Scene3D) d); target.PropertyChanged(ViewportProperty); } #region Public Properties ////// Models - Model3DGroup. Default value is Model3DGroup.EmptyGroup. /// public Model3DGroup Models { get { return (Model3DGroup) GetValue(ModelsProperty); } set { SetValueInternal(ModelsProperty, value); } } ////// Camera - Camera. Default value is null. /// public Camera Camera { get { return (Camera) GetValue(CameraProperty); } set { SetValueInternal(CameraProperty, value); } } ////// Viewport - Rect. Default value is new Rect(0,0,1,1). /// public Rect Viewport { get { return (Rect) GetValue(ViewportProperty); } set { SetValueInternal(ViewportProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new Scene3D(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Critical: This code calls into an unsafe code block /// TreatAsSafe: This code does not return any critical data.It is ok to expose /// Channels are safe to call into and do not go cross domain and cross process /// [SecurityCritical,SecurityTreatAsSafe] internal override void UpdateResource(DUCE.Channel channel, bool skipOnChannelCheck) { // If we're told we can skip the channel check, then we must be on channel Debug.Assert(!skipOnChannelCheck || _duceResource.IsOnChannel(channel)); if (skipOnChannelCheck || _duceResource.IsOnChannel(channel)) { base.UpdateResource(channel, skipOnChannelCheck); // Read values of properties into local variables Model3DGroup vModels = Models; Camera vCamera = Camera; // Obtain handles for properties that implement DUCE.IResource DUCE.ResourceHandle hModels = vModels != null ? ((DUCE.IResource)vModels).GetHandle(channel) : DUCE.ResourceHandle.Null; DUCE.ResourceHandle hCamera = vCamera != null ? ((DUCE.IResource)vCamera).GetHandle(channel) : DUCE.ResourceHandle.Null; // Obtain handles for animated properties DUCE.ResourceHandle hViewportAnimations = GetAnimationResourceHandle(ViewportProperty, channel); // Pack & send command packet DUCE.MILCMD_SCENE3D data; unsafe { data.Type = MILCMD.MilCmdScene3D; data.Handle = _duceResource.GetHandle(channel); data.hmodels = hModels; data.hcamera = hCamera; if (hViewportAnimations.IsNull) { data.viewport = Viewport; } data.hViewportAnimations = hViewportAnimations; // Send packed command structure channel.SendCommand( (byte*)&data, sizeof(DUCE.MILCMD_SCENE3D)); } } } DUCE.ResourceHandle DUCE.IResource.AddRefOnChannel(DUCE.Channel channel) { using (CompositionEngineLock.Acquire()) { if (_duceResource.CreateOrAddRefOnChannel(channel, System.Windows.Media.Composition.DUCE.ResourceType.TYPE_SCENE3D)) { Model3DGroup vModels = Models; if (vModels != null) ((DUCE.IResource)vModels).AddRefOnChannel(channel); Camera vCamera = Camera; if (vCamera != null) ((DUCE.IResource)vCamera).AddRefOnChannel(channel); AddRefOnChannelAnimations(channel); UpdateResource(channel, true /* skip "on channel" check - we already know that we're on channel */ ); } return _duceResource.GetHandle(channel); } } void DUCE.IResource.ReleaseOnChannel(DUCE.Channel channel) { using (CompositionEngineLock.Acquire()) { Debug.Assert(_duceResource.IsOnChannel(channel)); if (_duceResource.ReleaseOnChannel(channel)) { Model3DGroup vModels = Models; if (vModels != null) ((DUCE.IResource)vModels).ReleaseOnChannel(channel); Camera vCamera = Camera; if (vCamera != null) ((DUCE.IResource)vCamera).ReleaseOnChannel(channel); ReleaseOnChannelAnimations(channel); } } } DUCE.ResourceHandle DUCE.IResource.GetHandle(DUCE.Channel channel) { DUCE.ResourceHandle h; // Reconsider the need for this lock when removing the MultiChannelResource. using (CompositionEngineLock.Acquire()) { h = _duceResource.GetHandle(channel); } return h; } int DUCE.IResource.GetChannelCount() { // must already be in composition lock here return _duceResource.GetChannelCount(); } DUCE.Channel DUCE.IResource.GetChannel(int index) { // in a lock already return _duceResource.GetChannel(index); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties // // This property finds the correct initial size for the _effectiveValues store on the // current DependencyObject as a performance optimization // // This includes: // Models // Camera // Viewport // internal override int EffectiveValuesInitialSize { get { return 3; } } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the Scene3D.Models property. /// public static readonly DependencyProperty ModelsProperty; ////// The DependencyProperty for the Scene3D.Camera property. /// public static readonly DependencyProperty CameraProperty; ////// The DependencyProperty for the Scene3D.Viewport property. /// public static readonly DependencyProperty ViewportProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal System.Windows.Media.Composition.DUCE.MultiChannelResource _duceResource = new System.Windows.Media.Composition.DUCE.MultiChannelResource(); internal static Model3DGroup s_Models = Model3DGroup.EmptyGroup; internal static Camera s_Camera = null; internal static Rect s_Viewport = new Rect(0,0,1,1); #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static Scene3D() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // Debug.Assert(s_Models == null || s_Models.IsFrozen, "Detected context bound default value Scene3D.s_Models (See OS Bug #947272)."); Debug.Assert(s_Camera == null || s_Camera.IsFrozen, "Detected context bound default value Scene3D.s_Camera (See OS Bug #947272)."); // Initializations Type typeofThis = typeof(Scene3D); ModelsProperty = RegisterProperty("Models", typeof(Model3DGroup), typeofThis, Model3DGroup.EmptyGroup, new PropertyChangedCallback(ModelsPropertyChanged), null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); CameraProperty = RegisterProperty("Camera", typeof(Camera), typeofThis, null, new PropertyChangedCallback(CameraPropertyChanged), null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); ViewportProperty = RegisterProperty("Viewport", typeof(Rect), typeofThis, new Rect(0,0,1,1), new PropertyChangedCallback(ViewportPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Text; using System.Windows.Markup; using System.Windows.Media.Media3D.Converters; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Windows.Media.Imaging; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media.Media3D { sealed partial class Scene3D : Animatable, DUCE.IResource { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new Scene3D Clone() { return (Scene3D)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new Scene3D CloneCurrentValue() { return (Scene3D)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- private static void ModelsPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { // The first change to the default value of a mutable collection property (e.g. GeometryGroup.Children) // will promote the property value from a default value to a local value. This is technically a sub-property // change because the collection was changed and not a new collection set (GeometryGroup.Children. // Add versus GeometryGroup.Children = myNewChildrenCollection). However, we never marshalled // the default value to the compositor. If the property changes from a default value, the new local value // needs to be marshalled to the compositor. We detect this scenario with the second condition // e.OldValueSource != e.NewValueSource. Specifically in this scenario the OldValueSource will be // Default and the NewValueSource will be Local. if (e.IsASubPropertyChange && (e.OldValueSource == e.NewValueSource)) { return; } Scene3D target = ((Scene3D) d); Model3DGroup oldV = (Model3DGroup) e.OldValue; Model3DGroup newV = (Model3DGroup) e.NewValue; System.Windows.Threading.Dispatcher dispatcher = target.Dispatcher; if (dispatcher != null) { DUCE.IResource targetResource = (DUCE.IResource)target; using (CompositionEngineLock.Acquire()) { int channelCount = targetResource.GetChannelCount(); for (int channelIndex = 0; channelIndex < channelCount; channelIndex++) { DUCE.Channel channel = targetResource.GetChannel(channelIndex); Debug.Assert(!channel.IsOutOfBandChannel); Debug.Assert(!targetResource.GetHandle(channel).IsNull); target.ReleaseResource(oldV,channel); target.AddRefResource(newV,channel); } } } target.PropertyChanged(ModelsProperty); } private static void CameraPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { // The first change to the default value of a mutable collection property (e.g. GeometryGroup.Children) // will promote the property value from a default value to a local value. This is technically a sub-property // change because the collection was changed and not a new collection set (GeometryGroup.Children. // Add versus GeometryGroup.Children = myNewChildrenCollection). However, we never marshalled // the default value to the compositor. If the property changes from a default value, the new local value // needs to be marshalled to the compositor. We detect this scenario with the second condition // e.OldValueSource != e.NewValueSource. Specifically in this scenario the OldValueSource will be // Default and the NewValueSource will be Local. if (e.IsASubPropertyChange && (e.OldValueSource == e.NewValueSource)) { return; } Scene3D target = ((Scene3D) d); Camera oldV = (Camera) e.OldValue; Camera newV = (Camera) e.NewValue; System.Windows.Threading.Dispatcher dispatcher = target.Dispatcher; if (dispatcher != null) { DUCE.IResource targetResource = (DUCE.IResource)target; using (CompositionEngineLock.Acquire()) { int channelCount = targetResource.GetChannelCount(); for (int channelIndex = 0; channelIndex < channelCount; channelIndex++) { DUCE.Channel channel = targetResource.GetChannel(channelIndex); Debug.Assert(!channel.IsOutOfBandChannel); Debug.Assert(!targetResource.GetHandle(channel).IsNull); target.ReleaseResource(oldV,channel); target.AddRefResource(newV,channel); } } } target.PropertyChanged(CameraProperty); } private static void ViewportPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { Scene3D target = ((Scene3D) d); target.PropertyChanged(ViewportProperty); } #region Public Properties ////// Models - Model3DGroup. Default value is Model3DGroup.EmptyGroup. /// public Model3DGroup Models { get { return (Model3DGroup) GetValue(ModelsProperty); } set { SetValueInternal(ModelsProperty, value); } } ////// Camera - Camera. Default value is null. /// public Camera Camera { get { return (Camera) GetValue(CameraProperty); } set { SetValueInternal(CameraProperty, value); } } ////// Viewport - Rect. Default value is new Rect(0,0,1,1). /// public Rect Viewport { get { return (Rect) GetValue(ViewportProperty); } set { SetValueInternal(ViewportProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new Scene3D(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Critical: This code calls into an unsafe code block /// TreatAsSafe: This code does not return any critical data.It is ok to expose /// Channels are safe to call into and do not go cross domain and cross process /// [SecurityCritical,SecurityTreatAsSafe] internal override void UpdateResource(DUCE.Channel channel, bool skipOnChannelCheck) { // If we're told we can skip the channel check, then we must be on channel Debug.Assert(!skipOnChannelCheck || _duceResource.IsOnChannel(channel)); if (skipOnChannelCheck || _duceResource.IsOnChannel(channel)) { base.UpdateResource(channel, skipOnChannelCheck); // Read values of properties into local variables Model3DGroup vModels = Models; Camera vCamera = Camera; // Obtain handles for properties that implement DUCE.IResource DUCE.ResourceHandle hModels = vModels != null ? ((DUCE.IResource)vModels).GetHandle(channel) : DUCE.ResourceHandle.Null; DUCE.ResourceHandle hCamera = vCamera != null ? ((DUCE.IResource)vCamera).GetHandle(channel) : DUCE.ResourceHandle.Null; // Obtain handles for animated properties DUCE.ResourceHandle hViewportAnimations = GetAnimationResourceHandle(ViewportProperty, channel); // Pack & send command packet DUCE.MILCMD_SCENE3D data; unsafe { data.Type = MILCMD.MilCmdScene3D; data.Handle = _duceResource.GetHandle(channel); data.hmodels = hModels; data.hcamera = hCamera; if (hViewportAnimations.IsNull) { data.viewport = Viewport; } data.hViewportAnimations = hViewportAnimations; // Send packed command structure channel.SendCommand( (byte*)&data, sizeof(DUCE.MILCMD_SCENE3D)); } } } DUCE.ResourceHandle DUCE.IResource.AddRefOnChannel(DUCE.Channel channel) { using (CompositionEngineLock.Acquire()) { if (_duceResource.CreateOrAddRefOnChannel(channel, System.Windows.Media.Composition.DUCE.ResourceType.TYPE_SCENE3D)) { Model3DGroup vModels = Models; if (vModels != null) ((DUCE.IResource)vModels).AddRefOnChannel(channel); Camera vCamera = Camera; if (vCamera != null) ((DUCE.IResource)vCamera).AddRefOnChannel(channel); AddRefOnChannelAnimations(channel); UpdateResource(channel, true /* skip "on channel" check - we already know that we're on channel */ ); } return _duceResource.GetHandle(channel); } } void DUCE.IResource.ReleaseOnChannel(DUCE.Channel channel) { using (CompositionEngineLock.Acquire()) { Debug.Assert(_duceResource.IsOnChannel(channel)); if (_duceResource.ReleaseOnChannel(channel)) { Model3DGroup vModels = Models; if (vModels != null) ((DUCE.IResource)vModels).ReleaseOnChannel(channel); Camera vCamera = Camera; if (vCamera != null) ((DUCE.IResource)vCamera).ReleaseOnChannel(channel); ReleaseOnChannelAnimations(channel); } } } DUCE.ResourceHandle DUCE.IResource.GetHandle(DUCE.Channel channel) { DUCE.ResourceHandle h; // Reconsider the need for this lock when removing the MultiChannelResource. using (CompositionEngineLock.Acquire()) { h = _duceResource.GetHandle(channel); } return h; } int DUCE.IResource.GetChannelCount() { // must already be in composition lock here return _duceResource.GetChannelCount(); } DUCE.Channel DUCE.IResource.GetChannel(int index) { // in a lock already return _duceResource.GetChannel(index); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties // // This property finds the correct initial size for the _effectiveValues store on the // current DependencyObject as a performance optimization // // This includes: // Models // Camera // Viewport // internal override int EffectiveValuesInitialSize { get { return 3; } } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the Scene3D.Models property. /// public static readonly DependencyProperty ModelsProperty; ////// The DependencyProperty for the Scene3D.Camera property. /// public static readonly DependencyProperty CameraProperty; ////// The DependencyProperty for the Scene3D.Viewport property. /// public static readonly DependencyProperty ViewportProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal System.Windows.Media.Composition.DUCE.MultiChannelResource _duceResource = new System.Windows.Media.Composition.DUCE.MultiChannelResource(); internal static Model3DGroup s_Models = Model3DGroup.EmptyGroup; internal static Camera s_Camera = null; internal static Rect s_Viewport = new Rect(0,0,1,1); #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static Scene3D() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // Debug.Assert(s_Models == null || s_Models.IsFrozen, "Detected context bound default value Scene3D.s_Models (See OS Bug #947272)."); Debug.Assert(s_Camera == null || s_Camera.IsFrozen, "Detected context bound default value Scene3D.s_Camera (See OS Bug #947272)."); // Initializations Type typeofThis = typeof(Scene3D); ModelsProperty = RegisterProperty("Models", typeof(Model3DGroup), typeofThis, Model3DGroup.EmptyGroup, new PropertyChangedCallback(ModelsPropertyChanged), null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); CameraProperty = RegisterProperty("Camera", typeof(Camera), typeofThis, null, new PropertyChangedCallback(CameraPropertyChanged), null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); ViewportProperty = RegisterProperty("Viewport", typeof(Rect), typeofThis, new Rect(0,0,1,1), new PropertyChangedCallback(ViewportPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
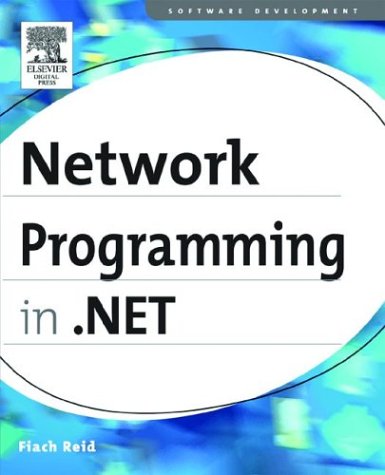
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EntityConnectionStringBuilder.cs
- TextBox.cs
- WorkflowDesignerMessageFilter.cs
- SecureEnvironment.cs
- WrapPanel.cs
- DataSourceGroupCollection.cs
- DebugView.cs
- DataSourceSelectArguments.cs
- SiteMapNodeItemEventArgs.cs
- InvalidCastException.cs
- Certificate.cs
- glyphs.cs
- WebReferencesBuildProvider.cs
- JsonQueryStringConverter.cs
- SolidColorBrush.cs
- CodeDOMProvider.cs
- VectorCollectionValueSerializer.cs
- QilPatternFactory.cs
- EndEvent.cs
- ApplicationServicesHostFactory.cs
- CompositionTarget.cs
- IteratorDescriptor.cs
- StorageTypeMapping.cs
- datacache.cs
- GenericEnumConverter.cs
- JoinTreeSlot.cs
- AffineTransform3D.cs
- ResXDataNode.cs
- InputLanguageSource.cs
- DeferredReference.cs
- QuaternionKeyFrameCollection.cs
- Group.cs
- DataGridViewCellParsingEventArgs.cs
- UnknownBitmapDecoder.cs
- XamlBuildTaskServices.cs
- SecurityToken.cs
- PropertyFilterAttribute.cs
- HyperLink.cs
- CanonicalXml.cs
- FileUpload.cs
- XmlNamedNodeMap.cs
- DataGridViewBindingCompleteEventArgs.cs
- VectorKeyFrameCollection.cs
- Timeline.cs
- DigitShape.cs
- SmtpMail.cs
- ConstraintConverter.cs
- CodeIdentifier.cs
- SupportsEventValidationAttribute.cs
- ThicknessAnimationUsingKeyFrames.cs
- ObjectDataSourceFilteringEventArgs.cs
- TimeSpan.cs
- Identity.cs
- FormatterConverter.cs
- DataKeyArray.cs
- OrthographicCamera.cs
- ApplicationSecurityInfo.cs
- Helper.cs
- EntityModelSchemaGenerator.cs
- PerformanceCounterPermissionEntryCollection.cs
- StreamMarshaler.cs
- StateDesigner.Layouts.cs
- DesignerListAdapter.cs
- AtomMaterializer.cs
- HostingEnvironmentWrapper.cs
- ProjectionPruner.cs
- Soap.cs
- Viewport3DAutomationPeer.cs
- CustomCategoryAttribute.cs
- SoapSchemaMember.cs
- Attribute.cs
- TextParagraphProperties.cs
- DirectionalLight.cs
- ProcessHostConfigUtils.cs
- DelegatingTypeDescriptionProvider.cs
- MappingItemCollection.cs
- LicenseManager.cs
- VisualStyleRenderer.cs
- ImageList.cs
- ColumnMapCopier.cs
- EntityDataSourceStatementEditor.cs
- HwndTarget.cs
- ErrorHandler.cs
- ApplicationFileParser.cs
- FullTextState.cs
- WebControlAdapter.cs
- TraceLevelStore.cs
- SQLBinaryStorage.cs
- PrintEvent.cs
- DependencyPropertyHelper.cs
- WebPartEditorOkVerb.cs
- CalendarDayButton.cs
- ColorConvertedBitmap.cs
- ProcessInputEventArgs.cs
- InputLanguageProfileNotifySink.cs
- DataViewSettingCollection.cs
- NavigateEvent.cs
- TreeNodeConverter.cs
- EnumValAlphaComparer.cs
- DataViewSetting.cs