Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / CommonUI / System / Drawing / Advanced / ImageFormat.cs / 1 / ImageFormat.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Imaging { using System; using System.Diagnostics; using System.Drawing; using System.ComponentModel; /** * Image format constants */ ////// /// Specifies the format of the image. /// [TypeConverterAttribute(typeof(ImageFormatConverter))] public sealed class ImageFormat { // Format IDs // private static ImageFormat undefined = new ImageFormat(new Guid("{b96b3ca9-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat memoryBMP = new ImageFormat(new Guid("{b96b3caa-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat bmp = new ImageFormat(new Guid("{b96b3cab-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat emf = new ImageFormat(new Guid("{b96b3cac-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat wmf = new ImageFormat(new Guid("{b96b3cad-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat jpeg = new ImageFormat(new Guid("{b96b3cae-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat png = new ImageFormat(new Guid("{b96b3caf-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat gif = new ImageFormat(new Guid("{b96b3cb0-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat tiff = new ImageFormat(new Guid("{b96b3cb1-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat exif = new ImageFormat(new Guid("{b96b3cb2-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat photoCD = new ImageFormat(new Guid("{b96b3cb3-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat flashPIX = new ImageFormat(new Guid("{b96b3cb4-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat icon = new ImageFormat(new Guid("{b96b3cb5-0728-11d3-9d7b-0000f81ef32e}")); private Guid guid; ////// /// Initializes a new instance of the public ImageFormat(Guid guid) { this.guid = guid; } ///class with the specified GUID. /// /// /// Specifies a global unique identifier (GUID) /// that represents this public Guid Guid { get { return guid;} } ///. /// /// /// Specifies a memory bitmap image format. /// public static ImageFormat MemoryBmp { get { return memoryBMP;} } ////// /// Specifies the bitmap image format. /// public static ImageFormat Bmp { get { return bmp;} } ////// /// Specifies the enhanced Windows metafile /// image format. /// public static ImageFormat Emf { get { return emf;} } ////// /// Specifies the Windows metafile image /// format. /// public static ImageFormat Wmf { get { return wmf;} } ////// /// Specifies the GIF image format. /// public static ImageFormat Gif { get { return gif;} } ////// /// Specifies the JPEG image format. /// public static ImageFormat Jpeg { get { return jpeg;} } ////// /// public static ImageFormat Png { get { return png;} } ////// Specifies the W3C PNG image format. /// ////// /// Specifies the Tag Image File /// Format (TIFF) image format. /// public static ImageFormat Tiff { get { return tiff;} } ////// /// Specifies the Exchangable Image Format /// (EXIF). /// public static ImageFormat Exif { get { return exif;} } ////// /// public static ImageFormat Icon { get { return icon;} } ////// Specifies the Windows icon image format. /// ////// /// Returns a value indicating whether the /// specified object is an public override bool Equals(object o) { ImageFormat format = o as ImageFormat; if (format == null) return false; return this.guid == format.guid; } ///equivalent to this . /// /// /// public override int GetHashCode() { return this.guid.GetHashCode(); } #if !FEATURE_PAL // Find any random encoder which supports this format internal ImageCodecInfo FindEncoder() { ImageCodecInfo[] codecs = ImageCodecInfo.GetImageEncoders(); foreach (ImageCodecInfo codec in codecs) { if (codec.FormatID.Equals(this.guid)) return codec; } return null; } #endif ////// Returns a hash code. /// ////// /// Converts this public override string ToString() { if (this == memoryBMP) return "MemoryBMP"; if (this == bmp) return "Bmp"; if (this == emf) return "Emf"; if (this == wmf) return "Wmf"; if (this == gif) return "Gif"; if (this == jpeg) return "Jpeg"; if (this == png) return "Png"; if (this == tiff) return "Tiff"; if (this == exif) return "Exif"; if (this == icon) return "Icon"; return "[ImageFormat: " + guid + "]"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //to a human-readable string. /// // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Imaging { using System; using System.Diagnostics; using System.Drawing; using System.ComponentModel; /** * Image format constants */ ////// /// Specifies the format of the image. /// [TypeConverterAttribute(typeof(ImageFormatConverter))] public sealed class ImageFormat { // Format IDs // private static ImageFormat undefined = new ImageFormat(new Guid("{b96b3ca9-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat memoryBMP = new ImageFormat(new Guid("{b96b3caa-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat bmp = new ImageFormat(new Guid("{b96b3cab-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat emf = new ImageFormat(new Guid("{b96b3cac-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat wmf = new ImageFormat(new Guid("{b96b3cad-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat jpeg = new ImageFormat(new Guid("{b96b3cae-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat png = new ImageFormat(new Guid("{b96b3caf-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat gif = new ImageFormat(new Guid("{b96b3cb0-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat tiff = new ImageFormat(new Guid("{b96b3cb1-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat exif = new ImageFormat(new Guid("{b96b3cb2-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat photoCD = new ImageFormat(new Guid("{b96b3cb3-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat flashPIX = new ImageFormat(new Guid("{b96b3cb4-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat icon = new ImageFormat(new Guid("{b96b3cb5-0728-11d3-9d7b-0000f81ef32e}")); private Guid guid; ////// /// Initializes a new instance of the public ImageFormat(Guid guid) { this.guid = guid; } ///class with the specified GUID. /// /// /// Specifies a global unique identifier (GUID) /// that represents this public Guid Guid { get { return guid;} } ///. /// /// /// Specifies a memory bitmap image format. /// public static ImageFormat MemoryBmp { get { return memoryBMP;} } ////// /// Specifies the bitmap image format. /// public static ImageFormat Bmp { get { return bmp;} } ////// /// Specifies the enhanced Windows metafile /// image format. /// public static ImageFormat Emf { get { return emf;} } ////// /// Specifies the Windows metafile image /// format. /// public static ImageFormat Wmf { get { return wmf;} } ////// /// Specifies the GIF image format. /// public static ImageFormat Gif { get { return gif;} } ////// /// Specifies the JPEG image format. /// public static ImageFormat Jpeg { get { return jpeg;} } ////// /// public static ImageFormat Png { get { return png;} } ////// Specifies the W3C PNG image format. /// ////// /// Specifies the Tag Image File /// Format (TIFF) image format. /// public static ImageFormat Tiff { get { return tiff;} } ////// /// Specifies the Exchangable Image Format /// (EXIF). /// public static ImageFormat Exif { get { return exif;} } ////// /// public static ImageFormat Icon { get { return icon;} } ////// Specifies the Windows icon image format. /// ////// /// Returns a value indicating whether the /// specified object is an public override bool Equals(object o) { ImageFormat format = o as ImageFormat; if (format == null) return false; return this.guid == format.guid; } ///equivalent to this . /// /// /// public override int GetHashCode() { return this.guid.GetHashCode(); } #if !FEATURE_PAL // Find any random encoder which supports this format internal ImageCodecInfo FindEncoder() { ImageCodecInfo[] codecs = ImageCodecInfo.GetImageEncoders(); foreach (ImageCodecInfo codec in codecs) { if (codec.FormatID.Equals(this.guid)) return codec; } return null; } #endif ////// Returns a hash code. /// ////// /// Converts this public override string ToString() { if (this == memoryBMP) return "MemoryBMP"; if (this == bmp) return "Bmp"; if (this == emf) return "Emf"; if (this == wmf) return "Wmf"; if (this == gif) return "Gif"; if (this == jpeg) return "Jpeg"; if (this == png) return "Png"; if (this == tiff) return "Tiff"; if (this == exif) return "Exif"; if (this == icon) return "Icon"; return "[ImageFormat: " + guid + "]"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.to a human-readable string. ///
Link Menu
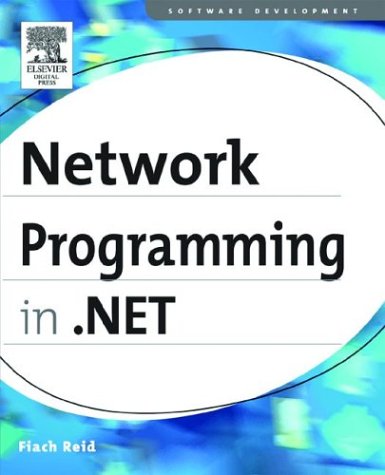
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Identity.cs
- SerializationInfo.cs
- WeakReference.cs
- SqlParameter.cs
- DataServiceProcessingPipelineEventArgs.cs
- DataGridViewAutoSizeColumnsModeEventArgs.cs
- XsdDuration.cs
- NameService.cs
- LogLogRecord.cs
- XmlSchema.cs
- EntityDataSourceReferenceGroup.cs
- TextParagraphCache.cs
- __Filters.cs
- Header.cs
- DriveInfo.cs
- ScriptManagerProxy.cs
- WebPartUserCapability.cs
- ProgressChangedEventArgs.cs
- SHA512.cs
- QueryCreatedEventArgs.cs
- MatrixTransform.cs
- DynamicResourceExtension.cs
- RelatedImageListAttribute.cs
- DBConnectionString.cs
- CategoryAttribute.cs
- StretchValidation.cs
- PropertyOverridesDialog.cs
- PartBasedPackageProperties.cs
- DesignConnection.cs
- ConnectionPoolManager.cs
- StdValidatorsAndConverters.cs
- DateTimeParse.cs
- PropertyEmitterBase.cs
- AccessDataSourceView.cs
- CompModSwitches.cs
- EraserBehavior.cs
- __Filters.cs
- KeyFrames.cs
- TreeIterator.cs
- SrgsGrammarCompiler.cs
- CodeDomSerializer.cs
- COM2ExtendedBrowsingHandler.cs
- UseManagedPresentationElement.cs
- PeerNameResolver.cs
- ExceptionUtil.cs
- Rect.cs
- RepeaterItemCollection.cs
- Timer.cs
- ImageListStreamer.cs
- TypeConverterHelper.cs
- DataGrid.cs
- HttpHandlersSection.cs
- FacetChecker.cs
- HostProtectionPermission.cs
- WindowsIPAddress.cs
- Helpers.cs
- WebPart.cs
- SafeNativeMethods.cs
- WindowsRichEdit.cs
- TimelineGroup.cs
- __FastResourceComparer.cs
- PolicyManager.cs
- TreeViewAutomationPeer.cs
- ModelItemKeyValuePair.cs
- GenericTextProperties.cs
- UniqueIdentifierService.cs
- DnsEndpointIdentity.cs
- XmlDocumentType.cs
- SplashScreen.cs
- Transform3D.cs
- CodeSnippetStatement.cs
- EntityDataSourceStatementEditorForm.cs
- StaticSiteMapProvider.cs
- EdmRelationshipNavigationPropertyAttribute.cs
- ObservableDictionary.cs
- ObjectHandle.cs
- OuterGlowBitmapEffect.cs
- PropertyIDSet.cs
- NameObjectCollectionBase.cs
- StyleTypedPropertyAttribute.cs
- ControlAdapter.cs
- XsdCachingReader.cs
- DiffuseMaterial.cs
- PipelineComponent.cs
- DrawTreeNodeEventArgs.cs
- HttpUnhandledOperationInvoker.cs
- IssuedTokensHeader.cs
- ListViewDesigner.cs
- MetadataPropertyCollection.cs
- ObjectRef.cs
- MsmqIntegrationChannelListener.cs
- ContractHandle.cs
- DataGridViewLayoutData.cs
- BitmapSource.cs
- URLString.cs
- _AcceptOverlappedAsyncResult.cs
- SendKeys.cs
- RichTextBox.cs
- Message.cs
- CodeComment.cs