Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Data / System / Data / OleDb / PropertyIDSet.cs / 1 / PropertyIDSet.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- using System; using System.Data; using System.Data.Common; using System.Data.ProviderBase; using System.Diagnostics; using System.Runtime.InteropServices; using System.Runtime.CompilerServices; using System.Security; using System.Security.Permissions; namespace System.Data.OleDb { internal sealed class PropertyIDSet : DbBuffer { static private readonly int PropertyIDSetAndValueSize = ODB.SizeOf_tagDBPROPIDSET + ADP.PtrSize; // sizeof(tagDBPROPIDSET) + sizeof(int) static private readonly int PropertyIDSetSize = ODB.SizeOf_tagDBPROPIDSET; private int _count; // the PropertyID is stored at the end of the tagDBPROPIDSET structure // this way only a single memory allocation is required instead of two internal PropertyIDSet(Guid propertySet, int propertyID) : base(PropertyIDSetAndValueSize) { _count = 1; // rgPropertyIDs references where that PropertyID is stored // depending on IntPtr.Size, tagDBPROPIDSET is either 24 or 28 bytes long IntPtr ptr = ADP.IntPtrOffset(base.handle, PropertyIDSetSize); Marshal.WriteIntPtr(base.handle, 0, ptr); Marshal.WriteInt32(base.handle, ADP.PtrSize, /*propertyid count*/1); ptr = ADP.IntPtrOffset(base.handle, ODB.OffsetOf_tagDBPROPIDSET_PropertySet); Marshal.StructureToPtr(propertySet, ptr, false/*deleteold*/); // write the propertyID at the same offset Marshal.WriteInt32(base.handle, PropertyIDSetSize, propertyID); } // no propertyIDs, just the propertyset guids internal PropertyIDSet(Guid[] propertySets) : base(PropertyIDSetSize * propertySets.Length) { _count = propertySets.Length; for(int i = 0; i < propertySets.Length; ++i) { IntPtr ptr = ADP.IntPtrOffset(base.handle, (i * PropertyIDSetSize) + ODB.OffsetOf_tagDBPROPIDSET_PropertySet); Marshal.StructureToPtr(propertySets[i], ptr, false/*deleteold*/); } } internal int Count { get { return _count; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- using System; using System.Data; using System.Data.Common; using System.Data.ProviderBase; using System.Diagnostics; using System.Runtime.InteropServices; using System.Runtime.CompilerServices; using System.Security; using System.Security.Permissions; namespace System.Data.OleDb { internal sealed class PropertyIDSet : DbBuffer { static private readonly int PropertyIDSetAndValueSize = ODB.SizeOf_tagDBPROPIDSET + ADP.PtrSize; // sizeof(tagDBPROPIDSET) + sizeof(int) static private readonly int PropertyIDSetSize = ODB.SizeOf_tagDBPROPIDSET; private int _count; // the PropertyID is stored at the end of the tagDBPROPIDSET structure // this way only a single memory allocation is required instead of two internal PropertyIDSet(Guid propertySet, int propertyID) : base(PropertyIDSetAndValueSize) { _count = 1; // rgPropertyIDs references where that PropertyID is stored // depending on IntPtr.Size, tagDBPROPIDSET is either 24 or 28 bytes long IntPtr ptr = ADP.IntPtrOffset(base.handle, PropertyIDSetSize); Marshal.WriteIntPtr(base.handle, 0, ptr); Marshal.WriteInt32(base.handle, ADP.PtrSize, /*propertyid count*/1); ptr = ADP.IntPtrOffset(base.handle, ODB.OffsetOf_tagDBPROPIDSET_PropertySet); Marshal.StructureToPtr(propertySet, ptr, false/*deleteold*/); // write the propertyID at the same offset Marshal.WriteInt32(base.handle, PropertyIDSetSize, propertyID); } // no propertyIDs, just the propertyset guids internal PropertyIDSet(Guid[] propertySets) : base(PropertyIDSetSize * propertySets.Length) { _count = propertySets.Length; for(int i = 0; i < propertySets.Length; ++i) { IntPtr ptr = ADP.IntPtrOffset(base.handle, (i * PropertyIDSetSize) + ODB.OffsetOf_tagDBPROPIDSET_PropertySet); Marshal.StructureToPtr(propertySets[i], ptr, false/*deleteold*/); } } internal int Count { get { return _count; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
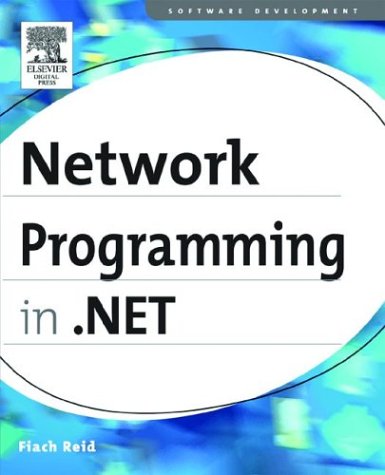
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InfoCardRSAPKCS1SignatureDeformatter.cs
- HuffModule.cs
- ClientTargetSection.cs
- Roles.cs
- EventRoute.cs
- DetailsViewUpdateEventArgs.cs
- ConcurrentQueue.cs
- UriWriter.cs
- XmlWrappingReader.cs
- ListMarkerSourceInfo.cs
- BitArray.cs
- MetadataArtifactLoaderXmlReaderWrapper.cs
- ListBox.cs
- TextPattern.cs
- OpenTypeCommon.cs
- RepeaterItem.cs
- BitmapEffectDrawingContent.cs
- OperationAbortedException.cs
- WindowsIdentity.cs
- DataKeyCollection.cs
- XmlDataSource.cs
- QueueProcessor.cs
- FlowDocumentPageViewerAutomationPeer.cs
- SecurityTokenRequirement.cs
- HttpResponseHeader.cs
- NamedObjectList.cs
- EntityEntry.cs
- MetadataItemCollectionFactory.cs
- Manipulation.cs
- EncryptedKey.cs
- XmlAutoDetectWriter.cs
- SafeLocalAllocation.cs
- AspCompat.cs
- QuaternionConverter.cs
- IERequestCache.cs
- WsatTransactionHeader.cs
- XmlSchemaSubstitutionGroup.cs
- MaterialGroup.cs
- ProcessInputEventArgs.cs
- SortedList.cs
- AssemblyName.cs
- SessionStateItemCollection.cs
- StreamInfo.cs
- Canvas.cs
- EventLogPermissionAttribute.cs
- CountdownEvent.cs
- AccessDataSourceView.cs
- ObjectCache.cs
- EventLogPermissionAttribute.cs
- ContentElement.cs
- ToolStripGripRenderEventArgs.cs
- IWorkflowDebuggerService.cs
- MethodBuilder.cs
- RectConverter.cs
- SchemaMerger.cs
- _SingleItemRequestCache.cs
- WindowProviderWrapper.cs
- DataSvcMapFile.cs
- WebScriptMetadataInstanceContextProvider.cs
- CssClassPropertyAttribute.cs
- WindowProviderWrapper.cs
- InheritedPropertyChangedEventArgs.cs
- HtmlMobileTextWriter.cs
- RunWorkerCompletedEventArgs.cs
- HMACSHA256.cs
- UrlPath.cs
- BindingContext.cs
- XmlAttributes.cs
- SessionEndedEventArgs.cs
- QilTernary.cs
- ReliableMessagingHelpers.cs
- TransportContext.cs
- LineMetrics.cs
- DataBindingList.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- PointValueSerializer.cs
- StrokeCollectionConverter.cs
- UrlAuthFailedErrorFormatter.cs
- TextEditorThreadLocalStore.cs
- DataGridViewCellCancelEventArgs.cs
- WorkflowMarkupElementEventArgs.cs
- CompilerGlobalScopeAttribute.cs
- SelectorItemAutomationPeer.cs
- TableHeaderCell.cs
- PseudoWebRequest.cs
- ZoomPercentageConverter.cs
- GetPageNumberCompletedEventArgs.cs
- TransformedBitmap.cs
- RegexGroup.cs
- RootBuilder.cs
- BaseParser.cs
- ObjectListFieldCollection.cs
- NumberSubstitution.cs
- TextComposition.cs
- TypeDescriptionProviderAttribute.cs
- AttributeTable.cs
- RawAppCommandInputReport.cs
- PropertyValue.cs
- SqlExpander.cs
- XmlILCommand.cs