Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Metadata / MetadataArtifactLoaderXmlReaderWrapper.cs / 1305376 / MetadataArtifactLoaderXmlReaderWrapper.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Collections; using System.Diagnostics; using System.Text; using System.Xml; using System.Security.Permissions; namespace System.Data.Metadata.Edm { ////// This class represents a wrapper around an XmlReader to be used to load metadata. /// Note that the XmlReader object isn't created here -- the wrapper simply stores /// a reference to it -- therefore we do not Close() the reader when we Dispose() /// the wrapper, i.e., Dispose() is a no-op. /// internal class MetadataArtifactLoaderXmlReaderWrapper : MetadataArtifactLoader, IComparable { private readonly XmlReader _reader = null; private readonly string _resourceUri = null; ////// Constructor - saves off the XmlReader in a private data field /// /// The path to the resource to load public MetadataArtifactLoaderXmlReaderWrapper(XmlReader xmlReader) { _reader = xmlReader; _resourceUri = xmlReader.BaseURI; } public override string Path { get { if (string.IsNullOrEmpty(this._resourceUri)) { return string.Empty; } else { return this._resourceUri; } } } ////// Implementation of IComparable.CompareTo() /// /// The object to compare to ///0 if the loaders are "equal" (i.e., have the same _path value) public int CompareTo(object obj) { MetadataArtifactLoaderXmlReaderWrapper loader = obj as MetadataArtifactLoaderXmlReaderWrapper; if (loader != null) { if (Object.ReferenceEquals(this._reader, loader._reader)) { return 0; } else { return -1; } } Debug.Assert(false, "object is not a MetadataArtifactLoaderXmlReaderWrapper"); return -1; } ////// Equals() returns true if the objects have the same _path value /// /// The object to compare to ///true if the objects have the same _path value public override bool Equals(object obj) { return this.CompareTo(obj) == 0; } ////// GetHashCode override that defers the result to the _path member variable. /// ///public override int GetHashCode() { return _reader.GetHashCode(); } public override void CollectFilePermissionPaths(List paths, DataSpace spaceToGet) { // no op } /// /// Get paths to artifacts for a specific DataSpace. /// /// The DataSpace for the artifacts of interest ///A List of strings identifying paths to all artifacts for a specific DataSpace public override ListGetPaths(DataSpace spaceToGet) { List list = new List (); if (MetadataArtifactLoader.IsArtifactOfDataSpace(Path, spaceToGet)) { list.Add(Path); } return list; } /// /// Get paths to all artifacts /// ///A List of strings identifying paths to all resources public override ListGetPaths() { return new List (new string[] { Path }); } /// /// Get XmlReaders for all resources /// ///A List of XmlReaders for all resources public override ListGetReaders(Dictionary sourceDictionary) { List list = new List (); list.Add(this._reader); if (sourceDictionary != null) { sourceDictionary.Add(this, _reader); } return list; } /// /// Create and return an XmlReader around the resource represented by this instance /// if it is of the requested DataSpace type. /// /// The DataSpace corresponding to the requested artifacts ///A List of XmlReader objects public override ListCreateReaders(DataSpace spaceToGet) { List list = new List (); if (MetadataArtifactLoader.IsArtifactOfDataSpace(Path, spaceToGet)) { list.Add(_reader); } return list; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Collections; using System.Diagnostics; using System.Text; using System.Xml; using System.Security.Permissions; namespace System.Data.Metadata.Edm { ////// This class represents a wrapper around an XmlReader to be used to load metadata. /// Note that the XmlReader object isn't created here -- the wrapper simply stores /// a reference to it -- therefore we do not Close() the reader when we Dispose() /// the wrapper, i.e., Dispose() is a no-op. /// internal class MetadataArtifactLoaderXmlReaderWrapper : MetadataArtifactLoader, IComparable { private readonly XmlReader _reader = null; private readonly string _resourceUri = null; ////// Constructor - saves off the XmlReader in a private data field /// /// The path to the resource to load public MetadataArtifactLoaderXmlReaderWrapper(XmlReader xmlReader) { _reader = xmlReader; _resourceUri = xmlReader.BaseURI; } public override string Path { get { if (string.IsNullOrEmpty(this._resourceUri)) { return string.Empty; } else { return this._resourceUri; } } } ////// Implementation of IComparable.CompareTo() /// /// The object to compare to ///0 if the loaders are "equal" (i.e., have the same _path value) public int CompareTo(object obj) { MetadataArtifactLoaderXmlReaderWrapper loader = obj as MetadataArtifactLoaderXmlReaderWrapper; if (loader != null) { if (Object.ReferenceEquals(this._reader, loader._reader)) { return 0; } else { return -1; } } Debug.Assert(false, "object is not a MetadataArtifactLoaderXmlReaderWrapper"); return -1; } ////// Equals() returns true if the objects have the same _path value /// /// The object to compare to ///true if the objects have the same _path value public override bool Equals(object obj) { return this.CompareTo(obj) == 0; } ////// GetHashCode override that defers the result to the _path member variable. /// ///public override int GetHashCode() { return _reader.GetHashCode(); } public override void CollectFilePermissionPaths(List paths, DataSpace spaceToGet) { // no op } /// /// Get paths to artifacts for a specific DataSpace. /// /// The DataSpace for the artifacts of interest ///A List of strings identifying paths to all artifacts for a specific DataSpace public override ListGetPaths(DataSpace spaceToGet) { List list = new List (); if (MetadataArtifactLoader.IsArtifactOfDataSpace(Path, spaceToGet)) { list.Add(Path); } return list; } /// /// Get paths to all artifacts /// ///A List of strings identifying paths to all resources public override ListGetPaths() { return new List (new string[] { Path }); } /// /// Get XmlReaders for all resources /// ///A List of XmlReaders for all resources public override ListGetReaders(Dictionary sourceDictionary) { List list = new List (); list.Add(this._reader); if (sourceDictionary != null) { sourceDictionary.Add(this, _reader); } return list; } /// /// Create and return an XmlReader around the resource represented by this instance /// if it is of the requested DataSpace type. /// /// The DataSpace corresponding to the requested artifacts ///A List of XmlReader objects public override ListCreateReaders(DataSpace spaceToGet) { List list = new List (); if (MetadataArtifactLoader.IsArtifactOfDataSpace(Path, spaceToGet)) { list.Add(_reader); } return list; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
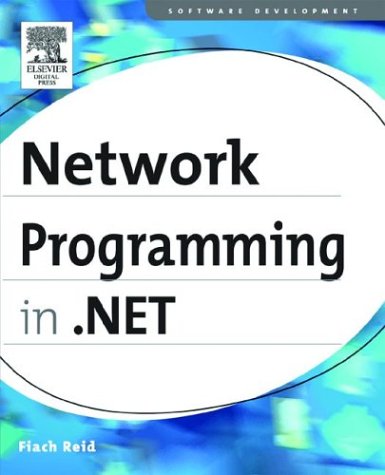
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProfileManager.cs
- Translator.cs
- SynchronizedDispatch.cs
- WebDisplayNameAttribute.cs
- CornerRadius.cs
- ControlTemplate.cs
- ExeContext.cs
- ReferencedType.cs
- InvalidWMPVersionException.cs
- MaterializeFromAtom.cs
- HandlerBase.cs
- FunctionMappingTranslator.cs
- ScriptReference.cs
- DrawingContextWalker.cs
- PenThreadPool.cs
- RequestCachePolicy.cs
- SafeThreadHandle.cs
- ZipPackage.cs
- WorkflowInstanceSuspendedRecord.cs
- OletxDependentTransaction.cs
- OleDbMetaDataFactory.cs
- Light.cs
- ApplicationSecurityManager.cs
- WebPartsPersonalization.cs
- CatalogPartCollection.cs
- MediaPlayerState.cs
- ConstraintCollection.cs
- EventLogger.cs
- UserPersonalizationStateInfo.cs
- XmlSchemaSimpleTypeRestriction.cs
- XmlCollation.cs
- SqlGenericUtil.cs
- XsltSettings.cs
- InvokeSchedule.cs
- ListChangedEventArgs.cs
- ExpressionPrinter.cs
- ToolStripRendererSwitcher.cs
- InheritanceContextHelper.cs
- Metafile.cs
- BrowserCapabilitiesFactoryBase.cs
- PersonalizableAttribute.cs
- HttpCapabilitiesEvaluator.cs
- XAMLParseException.cs
- CollaborationHelperFunctions.cs
- DataGridViewAutoSizeModeEventArgs.cs
- CollectionView.cs
- XmlUnspecifiedAttribute.cs
- Paragraph.cs
- AppendHelper.cs
- Array.cs
- NamespaceDecl.cs
- XsdValidatingReader.cs
- ChannelCacheDefaults.cs
- TabPage.cs
- ArglessEventHandlerProxy.cs
- AttachedPropertyBrowsableForChildrenAttribute.cs
- _WebProxyDataBuilder.cs
- Win32SafeHandles.cs
- WizardDesigner.cs
- MsmqInputChannel.cs
- EventLog.cs
- ArgumentsParser.cs
- QilUnary.cs
- SamlConditions.cs
- WebReferencesBuildProvider.cs
- FamilyCollection.cs
- XmlSchemaGroupRef.cs
- TreeNodeBindingCollection.cs
- MultipartIdentifier.cs
- CallSiteHelpers.cs
- TimeEnumHelper.cs
- StackBuilderSink.cs
- Pens.cs
- ItemContainerPattern.cs
- XXXInfos.cs
- relpropertyhelper.cs
- CoreSwitches.cs
- ExceptionHelpers.cs
- EditBehavior.cs
- MetadataUtilsSmi.cs
- TextChange.cs
- DmlSqlGenerator.cs
- MailWebEventProvider.cs
- Int16Converter.cs
- MultipartContentParser.cs
- GetPolicyDetailsRequest.cs
- CommonObjectSecurity.cs
- MulticastOption.cs
- CaseInsensitiveComparer.cs
- ParserExtension.cs
- ReadOnlyDataSource.cs
- ProxySimple.cs
- TypeInformation.cs
- ClientUrlResolverWrapper.cs
- ArithmeticException.cs
- RuleSetDialog.cs
- BooleanFacetDescriptionElement.cs
- LoginUtil.cs
- EmptyControlCollection.cs
- EnumCodeDomSerializer.cs