Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / CompMod / System / ComponentModel / ReadOnlyAttribute.cs / 1 / ReadOnlyAttribute.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel { using System; using System.Diagnostics; using System.Security.Permissions; ////// [AttributeUsage(AttributeTargets.All)] public sealed class ReadOnlyAttribute : Attribute { private bool isReadOnly = false; ///Specifies whether the property this attribute is bound to /// is read-only or read/write. ////// public static readonly ReadOnlyAttribute Yes = new ReadOnlyAttribute(true); ////// Specifies that the property this attribute is bound to is read-only and /// cannot be modified in the server explorer. This ///field is /// read-only. /// /// public static readonly ReadOnlyAttribute No = new ReadOnlyAttribute(false); ////// Specifies that the property this attribute is bound to is read/write and can /// be modified at design time. This ///field is read-only. /// /// public static readonly ReadOnlyAttribute Default = No; ////// Specifies the default value for the ///, which is , that is, /// the property this attribute is bound to is read/write. This field is read-only. /// /// public ReadOnlyAttribute(bool isReadOnly) { this.isReadOnly = isReadOnly; } ////// Initializes a new instance of the ///class. /// /// public bool IsReadOnly { get { return isReadOnly; } } ////// Gets a value indicating whether the property this attribute is bound to is /// read-only. /// ////// /// public override bool Equals(object value) { if (this == value) { return true; } ReadOnlyAttribute other = value as ReadOnlyAttribute; return other != null && other.IsReadOnly == IsReadOnly; } ////// public override int GetHashCode() { return base.GetHashCode(); } ////// Returns the hashcode for this object. /// ////// /// public override bool IsDefaultAttribute() { return (this.IsReadOnly == Default.IsReadOnly); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Determines if this attribute is the default. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel { using System; using System.Diagnostics; using System.Security.Permissions; ////// [AttributeUsage(AttributeTargets.All)] public sealed class ReadOnlyAttribute : Attribute { private bool isReadOnly = false; ///Specifies whether the property this attribute is bound to /// is read-only or read/write. ////// public static readonly ReadOnlyAttribute Yes = new ReadOnlyAttribute(true); ////// Specifies that the property this attribute is bound to is read-only and /// cannot be modified in the server explorer. This ///field is /// read-only. /// /// public static readonly ReadOnlyAttribute No = new ReadOnlyAttribute(false); ////// Specifies that the property this attribute is bound to is read/write and can /// be modified at design time. This ///field is read-only. /// /// public static readonly ReadOnlyAttribute Default = No; ////// Specifies the default value for the ///, which is , that is, /// the property this attribute is bound to is read/write. This field is read-only. /// /// public ReadOnlyAttribute(bool isReadOnly) { this.isReadOnly = isReadOnly; } ////// Initializes a new instance of the ///class. /// /// public bool IsReadOnly { get { return isReadOnly; } } ////// Gets a value indicating whether the property this attribute is bound to is /// read-only. /// ////// /// public override bool Equals(object value) { if (this == value) { return true; } ReadOnlyAttribute other = value as ReadOnlyAttribute; return other != null && other.IsReadOnly == IsReadOnly; } ////// public override int GetHashCode() { return base.GetHashCode(); } ////// Returns the hashcode for this object. /// ////// /// public override bool IsDefaultAttribute() { return (this.IsReadOnly == Default.IsReadOnly); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Determines if this attribute is the default. /// ///
Link Menu
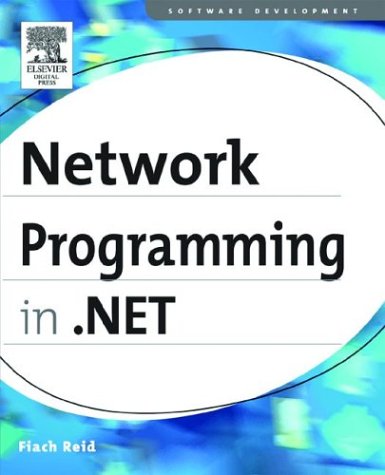
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Stacktrace.cs
- NamedElement.cs
- AppAction.cs
- TableAutomationPeer.cs
- bidPrivateBase.cs
- DataBinding.cs
- __Filters.cs
- HostSecurityManager.cs
- XmlSignatureManifest.cs
- TemplateDefinition.cs
- Span.cs
- DataTableCollection.cs
- RawKeyboardInputReport.cs
- SupportedAddressingMode.cs
- CleanUpVirtualizedItemEventArgs.cs
- ThreadStartException.cs
- WebConfigurationFileMap.cs
- ExpressionValueEditor.cs
- Container.cs
- UniqueIdentifierService.cs
- PathGeometry.cs
- SHA256.cs
- KeyGestureValueSerializer.cs
- SrgsRule.cs
- WsiProfilesElementCollection.cs
- WebFormDesignerActionService.cs
- ValueConversionAttribute.cs
- AcceleratedTokenAuthenticator.cs
- webclient.cs
- DataGridViewDataConnection.cs
- securestring.cs
- CultureSpecificStringDictionary.cs
- UrlMapping.cs
- DesignerEventService.cs
- UiaCoreProviderApi.cs
- TrustLevel.cs
- SystemIPGlobalProperties.cs
- XmlSchemaCompilationSettings.cs
- DurableEnlistmentState.cs
- ExtentCqlBlock.cs
- OdbcDataAdapter.cs
- ISAPIApplicationHost.cs
- CryptoStream.cs
- TrackingParameters.cs
- SamlAttribute.cs
- TemplateKeyConverter.cs
- ArraySubsetEnumerator.cs
- ToolBar.cs
- _NTAuthentication.cs
- StringArrayEditor.cs
- _StreamFramer.cs
- SqlLiftWhereClauses.cs
- MenuBindingsEditorForm.cs
- SR.cs
- DtdParser.cs
- ReceiveActivityValidator.cs
- MessageOperationFormatter.cs
- MetadataWorkspace.cs
- DataGridTemplateColumn.cs
- EventLogPermissionEntryCollection.cs
- Container.cs
- AnnotationService.cs
- EpmSyndicationContentSerializer.cs
- DiagnosticTrace.cs
- MexNamedPipeBindingElement.cs
- ExtensionElement.cs
- LabelExpression.cs
- ServiceDescription.cs
- IsolationInterop.cs
- OperatorExpressions.cs
- XmlSerializer.cs
- GenericEnumerator.cs
- DirtyTextRange.cs
- DynamicResourceExtensionConverter.cs
- COM2ExtendedBrowsingHandler.cs
- VariantWrapper.cs
- WebDisplayNameAttribute.cs
- CounterCreationData.cs
- RequestCachePolicy.cs
- tooltip.cs
- MetadataSource.cs
- RijndaelManagedTransform.cs
- ScrollProperties.cs
- RuntimeHandles.cs
- SortQuery.cs
- DoubleCollection.cs
- DoubleLink.cs
- ALinqExpressionVisitor.cs
- MappingModelBuildProvider.cs
- ColorAnimationUsingKeyFrames.cs
- XmlNodeReader.cs
- DataBoundControl.cs
- EncryptedType.cs
- ConstantSlot.cs
- WebPartCatalogAddVerb.cs
- EmptyEnumerator.cs
- DrawingImage.cs
- ActiveXContainer.cs
- LocationChangedEventArgs.cs
- PerformanceCounterTraceRecord.cs