Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Base / System / ComponentModel / SortDescription.cs / 1 / SortDescription.cs
//---------------------------------------------------------------------------- // //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // // Description: Defines property and direction to sort. // // See spec at http://avalon/connecteddata/M5%20Specs/IDataCollection.mht // // History: // 06/02/2003 : [....] - Created // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Windows; // SR namespace System.ComponentModel { ////// Defines a property and direction to sort a list by. /// public struct SortDescription { //----------------------------------------------------- // // Public Constructors // //----------------------------------------------------- #region Public Constructors ////// Create a sort description. /// /// Property to sort by /// Specifies the direction of sort operation ///direction is not a valid value for ListSortDirection public SortDescription(string propertyName, ListSortDirection direction) { if (direction != ListSortDirection.Ascending && direction != ListSortDirection.Descending) throw new InvalidEnumArgumentException("direction", (int)direction, typeof(ListSortDirection)); _propertyName = propertyName; _direction = direction; _sealed = false; } #endregion Public Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// Property name to sort by. /// public string PropertyName { get { return _propertyName; } set { if (_sealed) throw new InvalidOperationException(SR.Get(SRID.CannotChangeAfterSealed, "SortDescription")); _propertyName = value; } } ////// Sort direction. /// public ListSortDirection Direction { get { return _direction; } set { if (_sealed) throw new InvalidOperationException(SR.Get(SRID.CannotChangeAfterSealed, "SortDescription")); if (value < ListSortDirection.Ascending || value > ListSortDirection.Descending) throw new InvalidEnumArgumentException("value", (int) value, typeof(ListSortDirection)); _direction = value; } } ////// Returns true if the SortDescription is in use (sealed). /// public bool IsSealed { get { return _sealed; } } #endregion Public Properties //------------------------------------------------------ // // Public methods // //------------------------------------------------------ #region Public Methods ///Override of Object.Equals public override bool Equals(object obj) { return (obj is SortDescription) ? (this == (SortDescription)obj) : false; } ///Equality operator for SortDescription. public static bool operator==(SortDescription sd1, SortDescription sd2) { return sd1.PropertyName == sd2.PropertyName && sd1.Direction == sd2.Direction; } ///Inequality operator for SortDescription. public static bool operator!=(SortDescription sd1, SortDescription sd2) { return !(sd1 == sd2); } ///Override of Object.GetHashCode public override int GetHashCode() { int result = Direction.GetHashCode(); if (PropertyName != null) { result = unchecked(PropertyName.GetHashCode() + result); } return result; } #endregion Public Methods //----------------------------------------------------- // // Internal methods // //------------------------------------------------------ #region Internal Methods internal void Seal() { _sealed = true; } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private string _propertyName; private ListSortDirection _direction; bool _sealed; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // // Description: Defines property and direction to sort. // // See spec at http://avalon/connecteddata/M5%20Specs/IDataCollection.mht // // History: // 06/02/2003 : [....] - Created // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Windows; // SR namespace System.ComponentModel { ////// Defines a property and direction to sort a list by. /// public struct SortDescription { //----------------------------------------------------- // // Public Constructors // //----------------------------------------------------- #region Public Constructors ////// Create a sort description. /// /// Property to sort by /// Specifies the direction of sort operation ///direction is not a valid value for ListSortDirection public SortDescription(string propertyName, ListSortDirection direction) { if (direction != ListSortDirection.Ascending && direction != ListSortDirection.Descending) throw new InvalidEnumArgumentException("direction", (int)direction, typeof(ListSortDirection)); _propertyName = propertyName; _direction = direction; _sealed = false; } #endregion Public Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// Property name to sort by. /// public string PropertyName { get { return _propertyName; } set { if (_sealed) throw new InvalidOperationException(SR.Get(SRID.CannotChangeAfterSealed, "SortDescription")); _propertyName = value; } } ////// Sort direction. /// public ListSortDirection Direction { get { return _direction; } set { if (_sealed) throw new InvalidOperationException(SR.Get(SRID.CannotChangeAfterSealed, "SortDescription")); if (value < ListSortDirection.Ascending || value > ListSortDirection.Descending) throw new InvalidEnumArgumentException("value", (int) value, typeof(ListSortDirection)); _direction = value; } } ////// Returns true if the SortDescription is in use (sealed). /// public bool IsSealed { get { return _sealed; } } #endregion Public Properties //------------------------------------------------------ // // Public methods // //------------------------------------------------------ #region Public Methods ///Override of Object.Equals public override bool Equals(object obj) { return (obj is SortDescription) ? (this == (SortDescription)obj) : false; } ///Equality operator for SortDescription. public static bool operator==(SortDescription sd1, SortDescription sd2) { return sd1.PropertyName == sd2.PropertyName && sd1.Direction == sd2.Direction; } ///Inequality operator for SortDescription. public static bool operator!=(SortDescription sd1, SortDescription sd2) { return !(sd1 == sd2); } ///Override of Object.GetHashCode public override int GetHashCode() { int result = Direction.GetHashCode(); if (PropertyName != null) { result = unchecked(PropertyName.GetHashCode() + result); } return result; } #endregion Public Methods //----------------------------------------------------- // // Internal methods // //------------------------------------------------------ #region Internal Methods internal void Seal() { _sealed = true; } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private string _propertyName; private ListSortDirection _direction; bool _sealed; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
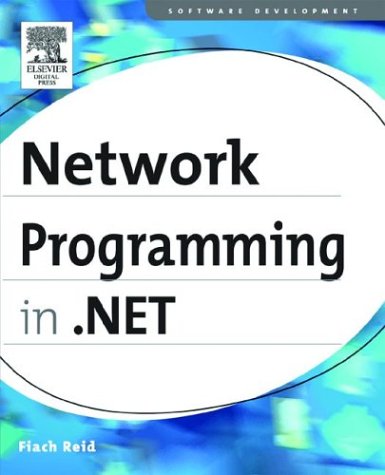
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSortKey.cs
- Comparer.cs
- TraceSource.cs
- ConfigurationValue.cs
- ADConnectionHelper.cs
- __Error.cs
- SendKeys.cs
- PaperSource.cs
- XmlSecureResolver.cs
- ParseChildrenAsPropertiesAttribute.cs
- ContextQuery.cs
- DBParameter.cs
- XmlUtf8RawTextWriter.cs
- XmlImplementation.cs
- SystemShuttingDownException.cs
- OdbcConnectionString.cs
- precedingsibling.cs
- HttpPostedFileBase.cs
- XmlParserContext.cs
- ResourceLoader.cs
- CssStyleCollection.cs
- MergablePropertyAttribute.cs
- ThrowHelper.cs
- FileStream.cs
- TransactionsSectionGroup.cs
- HostingEnvironmentSection.cs
- login.cs
- Point3DCollectionConverter.cs
- AuthenticationModuleElementCollection.cs
- TransformValueSerializer.cs
- AssertSection.cs
- ControlTemplate.cs
- QueryStatement.cs
- HostVisual.cs
- BufferCache.cs
- VirtualDirectoryMapping.cs
- DesignColumnCollection.cs
- ResourceBinder.cs
- BuilderPropertyEntry.cs
- AudioFormatConverter.cs
- DataKey.cs
- SelectQueryOperator.cs
- uribuilder.cs
- InArgument.cs
- PeerInvitationResponse.cs
- COM2ColorConverter.cs
- GPRECT.cs
- IProvider.cs
- XmlStringTable.cs
- ComponentCollection.cs
- Point4DValueSerializer.cs
- PropertyGridEditorPart.cs
- PrivilegeNotHeldException.cs
- ScriptReference.cs
- InkCanvas.cs
- XPathEmptyIterator.cs
- DownloadProgressEventArgs.cs
- HostProtectionPermission.cs
- DBConnection.cs
- ViewValidator.cs
- TrackingRecord.cs
- VBIdentifierTrimConverter.cs
- Helpers.cs
- RuleCache.cs
- DataGridBoolColumn.cs
- PageThemeBuildProvider.cs
- IndexedString.cs
- PrintDialogException.cs
- EntityContainerRelationshipSet.cs
- ParserHooks.cs
- AssemblyFilter.cs
- AvTraceDetails.cs
- TemplatePropertyEntry.cs
- Listen.cs
- AuthenticationModuleElementCollection.cs
- QueryPageSettingsEventArgs.cs
- SchemaNotation.cs
- ImageAutomationPeer.cs
- TextBox.cs
- SmiTypedGetterSetter.cs
- ObjectConverter.cs
- ValidationHelpers.cs
- IssuedTokenClientBehaviorsElementCollection.cs
- SqlTopReducer.cs
- WebPermission.cs
- QuaternionAnimation.cs
- MetadataArtifactLoaderCompositeFile.cs
- SqlCacheDependencyDatabaseCollection.cs
- ListBase.cs
- ProvidersHelper.cs
- DropTarget.cs
- EventLog.cs
- PageThemeBuildProvider.cs
- PreviewPageInfo.cs
- NameNode.cs
- Column.cs
- mactripleDES.cs
- CultureMapper.cs
- assemblycache.cs
- HMACSHA1.cs