Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / clr / src / BCL / System / Security / Permissions / HostProtectionPermission.cs / 1 / HostProtectionPermission.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // HostProtectionPermission.cs // namespace System.Security.Permissions { using System; using System.IO; using System.Security.Util; using System.Text; using System.Threading; using System.Runtime.Remoting; using System.Security; using System.Runtime.Serialization; using System.Reflection; using System.Globalization; // Keep this enum in [....] with tools\ngen\ngen.cpp and inc\mscoree.idl [Flags, Serializable] [System.Runtime.InteropServices.ComVisible(true)] public enum HostProtectionResource { None = 0x0, //-------------------------------- Synchronization = 0x1, SharedState = 0x2, ExternalProcessMgmt = 0x4, SelfAffectingProcessMgmt = 0x8, ExternalThreading = 0x10, SelfAffectingThreading = 0x20, SecurityInfrastructure = 0x40, UI = 0x80, MayLeakOnAbort = 0x100, //--------------------------------- All = 0x1ff, } [AttributeUsage(AttributeTargets.Method | AttributeTargets.Constructor | AttributeTargets.Class | AttributeTargets.Struct | AttributeTargets.Assembly | AttributeTargets.Delegate, AllowMultiple = true, Inherited = false )] [System.Runtime.InteropServices.ComVisible(true)] [Serializable()] sealed public class HostProtectionAttribute : CodeAccessSecurityAttribute { private HostProtectionResource m_resources = HostProtectionResource.None; public HostProtectionAttribute() : base( SecurityAction.LinkDemand ) { } public HostProtectionAttribute( SecurityAction action ) : base( action ) { if (action != SecurityAction.LinkDemand) throw new ArgumentException(Environment.GetResourceString("Argument_InvalidFlag")); } public HostProtectionResource Resources { get { return m_resources; } set { m_resources = value; } } public bool Synchronization { get { return (m_resources & HostProtectionResource.Synchronization) != 0; } set { m_resources = (value ? m_resources | HostProtectionResource.Synchronization : m_resources & ~HostProtectionResource.Synchronization); } } public bool SharedState { get { return (m_resources & HostProtectionResource.SharedState) != 0; } set { m_resources = (value ? m_resources | HostProtectionResource.SharedState : m_resources & ~HostProtectionResource.SharedState); } } public bool ExternalProcessMgmt { get { return (m_resources & HostProtectionResource.ExternalProcessMgmt) != 0; } set { m_resources = (value ? m_resources | HostProtectionResource.ExternalProcessMgmt : m_resources & ~HostProtectionResource.ExternalProcessMgmt); } } public bool SelfAffectingProcessMgmt { get { return (m_resources & HostProtectionResource.SelfAffectingProcessMgmt) != 0; } set { m_resources = (value ? m_resources | HostProtectionResource.SelfAffectingProcessMgmt : m_resources & ~HostProtectionResource.SelfAffectingProcessMgmt); } } public bool ExternalThreading { get { return (m_resources & HostProtectionResource.ExternalThreading) != 0; } set { m_resources = (value ? m_resources | HostProtectionResource.ExternalThreading : m_resources & ~HostProtectionResource.ExternalThreading); } } public bool SelfAffectingThreading { get { return (m_resources & HostProtectionResource.SelfAffectingThreading) != 0; } set { m_resources = (value ? m_resources | HostProtectionResource.SelfAffectingThreading : m_resources & ~HostProtectionResource.SelfAffectingThreading); } } [System.Runtime.InteropServices.ComVisible(true)] public bool SecurityInfrastructure { get { return (m_resources & HostProtectionResource.SecurityInfrastructure) != 0; } set { m_resources = (value ? m_resources | HostProtectionResource.SecurityInfrastructure : m_resources & ~HostProtectionResource.SecurityInfrastructure); } } public bool UI { get { return (m_resources & HostProtectionResource.UI) != 0; } set { m_resources = (value ? m_resources | HostProtectionResource.UI : m_resources & ~HostProtectionResource.UI); } } public bool MayLeakOnAbort { get { return (m_resources & HostProtectionResource.MayLeakOnAbort) != 0; } set { m_resources = (value ? m_resources | HostProtectionResource.MayLeakOnAbort : m_resources & ~HostProtectionResource.MayLeakOnAbort); } } public override IPermission CreatePermission() { if (m_unrestricted) { return new HostProtectionPermission( PermissionState.Unrestricted ); } else { return new HostProtectionPermission( m_resources ); } } } [Serializable()] sealed internal class HostProtectionPermission : CodeAccessPermission, IUnrestrictedPermission, IBuiltInPermission { //------------------------------------------------------ // // GLOBALS // //----------------------------------------------------- // This value is set by PermissionSet.FilterHostProtectionPermissions. It is only used for // constructing a HostProtectionException object. Changing it will not affect HostProtection. internal static HostProtectionResource protectedResources = HostProtectionResource.None; //----------------------------------------------------- // // MEMBERS // //----------------------------------------------------- private HostProtectionResource m_resources; //------------------------------------------------------ // // CONSTRUCTORS // //----------------------------------------------------- public HostProtectionPermission(PermissionState state) { if (state == PermissionState.Unrestricted) Resources = HostProtectionResource.All; else if (state == PermissionState.None) Resources = HostProtectionResource.None; else throw new ArgumentException(Environment.GetResourceString("Argument_InvalidPermissionState")); } public HostProtectionPermission(HostProtectionResource resources) { Resources = resources; } //------------------------------------------------------ // // IPermission interface implementation // //------------------------------------------------------ public bool IsUnrestricted() { return Resources == HostProtectionResource.All; } //----------------------------------------------------- // // Properties // //------------------------------------------------------ public HostProtectionResource Resources { set { if(value < HostProtectionResource.None || value > HostProtectionResource.All) throw new ArgumentException(String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("Arg_EnumIllegalVal"), (int)value)); m_resources = value; } get { return m_resources; } } //----------------------------------------------------- // // IPermission interface implementation // //----------------------------------------------------- public override bool IsSubsetOf(IPermission target) { if (target == null) return m_resources == HostProtectionResource.None; if(this.GetType() != target.GetType()) throw new ArgumentException( String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("Argument_WrongType"), this.GetType().FullName) ); return ((uint)this.m_resources & (uint)((HostProtectionPermission)target).m_resources) == (uint)this.m_resources; } public override IPermission Union(IPermission target) { if (target == null) return(this.Copy()); if(this.GetType() != target.GetType()) throw new ArgumentException( String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("Argument_WrongType"), this.GetType().FullName) ); HostProtectionResource newResources = (HostProtectionResource)((uint)this.m_resources | (uint)((HostProtectionPermission)target).m_resources); return new HostProtectionPermission(newResources); } public override IPermission Intersect(IPermission target) { if (target == null) return null; if(this.GetType() != target.GetType()) throw new ArgumentException( String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("Argument_WrongType"), this.GetType().FullName) ); HostProtectionResource newResources = (HostProtectionResource)((uint)this.m_resources & (uint)((HostProtectionPermission)target).m_resources); if(newResources == HostProtectionResource.None) return null; return new HostProtectionPermission(newResources); } public override IPermission Copy() { return new HostProtectionPermission(m_resources); } //----------------------------------------------------- // // XML // //------------------------------------------------------ public override SecurityElement ToXml() { SecurityElement esd = CodeAccessPermission.CreatePermissionElement( this, this.GetType().FullName ); if(IsUnrestricted()) esd.AddAttribute( "Unrestricted", "true" ); else esd.AddAttribute( "Resources", XMLUtil.BitFieldEnumToString( typeof( HostProtectionResource ), Resources ) ); return esd; } public override void FromXml(SecurityElement esd) { CodeAccessPermission.ValidateElement( esd, this ); if (XMLUtil.IsUnrestricted( esd )) Resources = HostProtectionResource.All; else { String resources = esd.Attribute( "Resources" ); if (resources == null) Resources = HostProtectionResource.None; else Resources = (HostProtectionResource)Enum.Parse( typeof( HostProtectionResource ), resources ); } } //----------------------------------------------------- // // OBJECT OVERRIDES // //------------------------------------------------------ ///int IBuiltInPermission.GetTokenIndex() { return HostProtectionPermission.GetTokenIndex(); } internal static int GetTokenIndex() { return BuiltInPermissionIndex.HostProtectionPermissionIndex; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // HostProtectionPermission.cs // namespace System.Security.Permissions { using System; using System.IO; using System.Security.Util; using System.Text; using System.Threading; using System.Runtime.Remoting; using System.Security; using System.Runtime.Serialization; using System.Reflection; using System.Globalization; // Keep this enum in [....] with tools\ngen\ngen.cpp and inc\mscoree.idl [Flags, Serializable] [System.Runtime.InteropServices.ComVisible(true)] public enum HostProtectionResource { None = 0x0, //-------------------------------- Synchronization = 0x1, SharedState = 0x2, ExternalProcessMgmt = 0x4, SelfAffectingProcessMgmt = 0x8, ExternalThreading = 0x10, SelfAffectingThreading = 0x20, SecurityInfrastructure = 0x40, UI = 0x80, MayLeakOnAbort = 0x100, //--------------------------------- All = 0x1ff, } [AttributeUsage(AttributeTargets.Method | AttributeTargets.Constructor | AttributeTargets.Class | AttributeTargets.Struct | AttributeTargets.Assembly | AttributeTargets.Delegate, AllowMultiple = true, Inherited = false )] [System.Runtime.InteropServices.ComVisible(true)] [Serializable()] sealed public class HostProtectionAttribute : CodeAccessSecurityAttribute { private HostProtectionResource m_resources = HostProtectionResource.None; public HostProtectionAttribute() : base( SecurityAction.LinkDemand ) { } public HostProtectionAttribute( SecurityAction action ) : base( action ) { if (action != SecurityAction.LinkDemand) throw new ArgumentException(Environment.GetResourceString("Argument_InvalidFlag")); } public HostProtectionResource Resources { get { return m_resources; } set { m_resources = value; } } public bool Synchronization { get { return (m_resources & HostProtectionResource.Synchronization) != 0; } set { m_resources = (value ? m_resources | HostProtectionResource.Synchronization : m_resources & ~HostProtectionResource.Synchronization); } } public bool SharedState { get { return (m_resources & HostProtectionResource.SharedState) != 0; } set { m_resources = (value ? m_resources | HostProtectionResource.SharedState : m_resources & ~HostProtectionResource.SharedState); } } public bool ExternalProcessMgmt { get { return (m_resources & HostProtectionResource.ExternalProcessMgmt) != 0; } set { m_resources = (value ? m_resources | HostProtectionResource.ExternalProcessMgmt : m_resources & ~HostProtectionResource.ExternalProcessMgmt); } } public bool SelfAffectingProcessMgmt { get { return (m_resources & HostProtectionResource.SelfAffectingProcessMgmt) != 0; } set { m_resources = (value ? m_resources | HostProtectionResource.SelfAffectingProcessMgmt : m_resources & ~HostProtectionResource.SelfAffectingProcessMgmt); } } public bool ExternalThreading { get { return (m_resources & HostProtectionResource.ExternalThreading) != 0; } set { m_resources = (value ? m_resources | HostProtectionResource.ExternalThreading : m_resources & ~HostProtectionResource.ExternalThreading); } } public bool SelfAffectingThreading { get { return (m_resources & HostProtectionResource.SelfAffectingThreading) != 0; } set { m_resources = (value ? m_resources | HostProtectionResource.SelfAffectingThreading : m_resources & ~HostProtectionResource.SelfAffectingThreading); } } [System.Runtime.InteropServices.ComVisible(true)] public bool SecurityInfrastructure { get { return (m_resources & HostProtectionResource.SecurityInfrastructure) != 0; } set { m_resources = (value ? m_resources | HostProtectionResource.SecurityInfrastructure : m_resources & ~HostProtectionResource.SecurityInfrastructure); } } public bool UI { get { return (m_resources & HostProtectionResource.UI) != 0; } set { m_resources = (value ? m_resources | HostProtectionResource.UI : m_resources & ~HostProtectionResource.UI); } } public bool MayLeakOnAbort { get { return (m_resources & HostProtectionResource.MayLeakOnAbort) != 0; } set { m_resources = (value ? m_resources | HostProtectionResource.MayLeakOnAbort : m_resources & ~HostProtectionResource.MayLeakOnAbort); } } public override IPermission CreatePermission() { if (m_unrestricted) { return new HostProtectionPermission( PermissionState.Unrestricted ); } else { return new HostProtectionPermission( m_resources ); } } } [Serializable()] sealed internal class HostProtectionPermission : CodeAccessPermission, IUnrestrictedPermission, IBuiltInPermission { //------------------------------------------------------ // // GLOBALS // //----------------------------------------------------- // This value is set by PermissionSet.FilterHostProtectionPermissions. It is only used for // constructing a HostProtectionException object. Changing it will not affect HostProtection. internal static HostProtectionResource protectedResources = HostProtectionResource.None; //----------------------------------------------------- // // MEMBERS // //----------------------------------------------------- private HostProtectionResource m_resources; //------------------------------------------------------ // // CONSTRUCTORS // //----------------------------------------------------- public HostProtectionPermission(PermissionState state) { if (state == PermissionState.Unrestricted) Resources = HostProtectionResource.All; else if (state == PermissionState.None) Resources = HostProtectionResource.None; else throw new ArgumentException(Environment.GetResourceString("Argument_InvalidPermissionState")); } public HostProtectionPermission(HostProtectionResource resources) { Resources = resources; } //------------------------------------------------------ // // IPermission interface implementation // //------------------------------------------------------ public bool IsUnrestricted() { return Resources == HostProtectionResource.All; } //----------------------------------------------------- // // Properties // //------------------------------------------------------ public HostProtectionResource Resources { set { if(value < HostProtectionResource.None || value > HostProtectionResource.All) throw new ArgumentException(String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("Arg_EnumIllegalVal"), (int)value)); m_resources = value; } get { return m_resources; } } //----------------------------------------------------- // // IPermission interface implementation // //----------------------------------------------------- public override bool IsSubsetOf(IPermission target) { if (target == null) return m_resources == HostProtectionResource.None; if(this.GetType() != target.GetType()) throw new ArgumentException( String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("Argument_WrongType"), this.GetType().FullName) ); return ((uint)this.m_resources & (uint)((HostProtectionPermission)target).m_resources) == (uint)this.m_resources; } public override IPermission Union(IPermission target) { if (target == null) return(this.Copy()); if(this.GetType() != target.GetType()) throw new ArgumentException( String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("Argument_WrongType"), this.GetType().FullName) ); HostProtectionResource newResources = (HostProtectionResource)((uint)this.m_resources | (uint)((HostProtectionPermission)target).m_resources); return new HostProtectionPermission(newResources); } public override IPermission Intersect(IPermission target) { if (target == null) return null; if(this.GetType() != target.GetType()) throw new ArgumentException( String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("Argument_WrongType"), this.GetType().FullName) ); HostProtectionResource newResources = (HostProtectionResource)((uint)this.m_resources & (uint)((HostProtectionPermission)target).m_resources); if(newResources == HostProtectionResource.None) return null; return new HostProtectionPermission(newResources); } public override IPermission Copy() { return new HostProtectionPermission(m_resources); } //----------------------------------------------------- // // XML // //------------------------------------------------------ public override SecurityElement ToXml() { SecurityElement esd = CodeAccessPermission.CreatePermissionElement( this, this.GetType().FullName ); if(IsUnrestricted()) esd.AddAttribute( "Unrestricted", "true" ); else esd.AddAttribute( "Resources", XMLUtil.BitFieldEnumToString( typeof( HostProtectionResource ), Resources ) ); return esd; } public override void FromXml(SecurityElement esd) { CodeAccessPermission.ValidateElement( esd, this ); if (XMLUtil.IsUnrestricted( esd )) Resources = HostProtectionResource.All; else { String resources = esd.Attribute( "Resources" ); if (resources == null) Resources = HostProtectionResource.None; else Resources = (HostProtectionResource)Enum.Parse( typeof( HostProtectionResource ), resources ); } } //----------------------------------------------------- // // OBJECT OVERRIDES // //------------------------------------------------------ /// int IBuiltInPermission.GetTokenIndex() { return HostProtectionPermission.GetTokenIndex(); } internal static int GetTokenIndex() { return BuiltInPermissionIndex.HostProtectionPermissionIndex; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
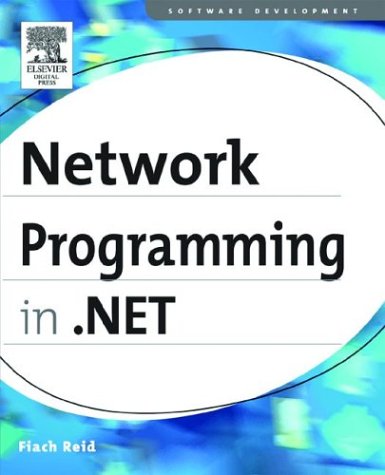
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StateWorkerRequest.cs
- ResXBuildProvider.cs
- FormClosedEvent.cs
- FrameworkContentElement.cs
- StringToken.cs
- MergeFilterQuery.cs
- CookieProtection.cs
- TemplatedMailWebEventProvider.cs
- EventProperty.cs
- DropShadowBitmapEffect.cs
- DependencyObjectProvider.cs
- XmlSchemas.cs
- UnsafeNativeMethods.cs
- XmlHelper.cs
- CodeTypeDeclaration.cs
- CharacterBufferReference.cs
- RegexNode.cs
- XmlTextEncoder.cs
- SoapMessage.cs
- ResourcesChangeInfo.cs
- RtfNavigator.cs
- ClientFormsIdentity.cs
- ParserExtension.cs
- DiscardableAttribute.cs
- ReturnType.cs
- TemplateManager.cs
- WindowsProgressbar.cs
- MD5.cs
- KeySpline.cs
- EqualityComparer.cs
- ConnectionConsumerAttribute.cs
- GroupBoxRenderer.cs
- SQLInt64Storage.cs
- DocumentEventArgs.cs
- WhileDesigner.cs
- SwitchLevelAttribute.cs
- ToolStripLabel.cs
- ApplicationInfo.cs
- entityreference_tresulttype.cs
- DeviceContexts.cs
- CustomValidator.cs
- VideoDrawing.cs
- PeerPresenceInfo.cs
- TemporaryBitmapFile.cs
- CardSpaceShim.cs
- PropertyReferenceSerializer.cs
- IntegrationExceptionEventArgs.cs
- ContentPlaceHolder.cs
- AnimatedTypeHelpers.cs
- FontInfo.cs
- WindowsListViewScroll.cs
- RangeBaseAutomationPeer.cs
- XmlSchemaValidator.cs
- XmlSchemaAttributeGroup.cs
- PageCatalogPart.cs
- SelectionRangeConverter.cs
- HttpCookie.cs
- SqlCachedBuffer.cs
- MembershipUser.cs
- InputEventArgs.cs
- Effect.cs
- RichListBox.cs
- ELinqQueryState.cs
- HijriCalendar.cs
- NavigationPropertyEmitter.cs
- FixedSOMTableRow.cs
- SimpleMailWebEventProvider.cs
- KeyGestureConverter.cs
- odbcmetadatafactory.cs
- InternalControlCollection.cs
- TextElementEnumerator.cs
- AppDomainManager.cs
- AttributeTableBuilder.cs
- Cell.cs
- AsyncPostBackErrorEventArgs.cs
- DesignTimeVisibleAttribute.cs
- PublishLicense.cs
- IndexOutOfRangeException.cs
- WebPartEditorOkVerb.cs
- LoadMessageLogger.cs
- Attributes.cs
- HttpModuleActionCollection.cs
- FontResourceCache.cs
- bindurihelper.cs
- MatrixTransform3D.cs
- ListBindableAttribute.cs
- TraceContextRecord.cs
- AccessibleObject.cs
- SmiEventStream.cs
- DesigntimeLicenseContextSerializer.cs
- EntityDataReader.cs
- PrincipalPermission.cs
- DropTarget.cs
- XPathDescendantIterator.cs
- SocketStream.cs
- ToolBarDesigner.cs
- DragDrop.cs
- TableDetailsCollection.cs
- mansign.cs
- SoapMessage.cs