Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Mapping / ViewValidator.cs / 1 / ViewValidator.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System.Data.Metadata.Edm; using System.Globalization; using System.Data.Common.CommandTrees; using System.Collections.Generic; using System.Diagnostics; using System.Data.Entity; namespace System.Data.Mapping { ////// Verifies that only legal expressions exist in a user-defined query mapping view. /// /// - ViewExpr = (see ViewExpressionValidator) /// Project(Projection, ViewExpr) | /// Project(Value, ViewExpr) | /// Filter(ViewExpr, ViewExpr) | /// Join(ViewExpr, ViewExpr, ViewExpr) | /// UnionAll(ViewExpr, ViewExpr) | /// Scan(S-Space EntitySet) /// Constant | /// Property{StructuralProperty}(ViewExpr) | /// Null | /// VariableReference | /// Cast(ViewExpr) | /// Case([Predicate, ViewExpr]*, ViewExpr) | /// Comparison(ViewExpr, ViewExpr) | /// Not(ViewExpr) | /// And(ViewExpr, ViewExpr) | /// Or(ViewExpr, ViewExpr) | /// IsNull(ViewExpr) /// - Projection = (see ViewExpressionValidator.Visit(DbProjectExpression) /// NewInstance{TargetEntity}(ViewExpr*) | /// NewInstance{Row}(ViewExpr*) /// internal static class ViewValidator { ////// Determines whether the given view is valid. /// /// Query view to validate. /// Store item collection. /// Mapping in which view is declared. ///Errors in view definition. internal static IEnumerableValidateQueryView(DbQueryCommandTree view, StoreItemCollection storeItemCollection, StorageSetMapping setMapping, EntityTypeBase elementType, bool includeSubtypes) { ViewExpressionValidator validator = new ViewExpressionValidator(storeItemCollection, setMapping, elementType, includeSubtypes); validator.VisitExpression(view.Query); return validator.Errors; } private sealed class ViewExpressionValidator : BasicExpressionVisitor { private readonly StorageSetMapping _setMapping; private readonly StoreItemCollection _storeItemCollection; private readonly List _errors; private readonly EntityTypeBase _elementType; private readonly bool _includeSubtypes; internal ViewExpressionValidator(StoreItemCollection storeItemCollection, StorageSetMapping setMapping, EntityTypeBase elementType, bool includeSubtypes) { Debug.Assert(null != setMapping); Debug.Assert(null != storeItemCollection); _setMapping = setMapping; _storeItemCollection = storeItemCollection; _errors = new List (); _elementType = elementType; _includeSubtypes = includeSubtypes; } internal IEnumerable Errors { get { return _errors; } } public override void VisitExpression(DbExpression expression) { if (null != expression) { ValidateExpressionKind(expression.ExpressionKind); } base.VisitExpression(expression); } private void ValidateExpressionKind(DbExpressionKind expressionKind) { switch (expressionKind) { // Supported expression kinds case DbExpressionKind.Constant: case DbExpressionKind.Property: case DbExpressionKind.Null: case DbExpressionKind.VariableReference: case DbExpressionKind.Cast: case DbExpressionKind.Case: case DbExpressionKind.Not: case DbExpressionKind.Or: case DbExpressionKind.And: case DbExpressionKind.IsNull: case DbExpressionKind.Equals: case DbExpressionKind.NotEquals: case DbExpressionKind.LessThan: case DbExpressionKind.LessThanOrEquals: case DbExpressionKind.GreaterThan: case DbExpressionKind.GreaterThanOrEquals: case DbExpressionKind.Project: case DbExpressionKind.NewInstance: case DbExpressionKind.Filter: case DbExpressionKind.Ref: case DbExpressionKind.UnionAll: case DbExpressionKind.Scan: case DbExpressionKind.FullOuterJoin: case DbExpressionKind.LeftOuterJoin: case DbExpressionKind.InnerJoin: case DbExpressionKind.EntityRef: break; default: string elementString = (_includeSubtypes)?"IsTypeOf("+_elementType.ToString()+")":_elementType.ToString(); _errors.Add(new EdmSchemaError(System.Data.Entity.Strings.Mapping_UnsupportedExpressionKind_QueryView_2( _setMapping.Set.Name, elementString, expressionKind), (int)StorageMappingErrorCode.MappingUnsupportedExpressionKindQueryView, EdmSchemaErrorSeverity.Error, _setMapping.EntityContainerMapping.SourceLocation, _setMapping.StartLineNumber, _setMapping.StartLinePosition)); break; } } public override void Visit(DbPropertyExpression expression) { base.Visit(expression); if (expression.Property.BuiltInTypeKind != BuiltInTypeKind.EdmProperty) { _errors.Add(new EdmSchemaError(System.Data.Entity.Strings.Mapping_UnsupportedPropertyKind_QueryView_3( _setMapping.Set.Name, expression.Property.Name, expression.Property.BuiltInTypeKind), (int)StorageMappingErrorCode.MappingUnsupportedPropertyKindQueryView, EdmSchemaErrorSeverity.Error, _setMapping.EntityContainerMapping.SourceLocation, _setMapping.StartLineNumber, _setMapping.StartLinePosition)); } } public override void Visit(DbNewInstanceExpression expression) { base.Visit(expression); EdmType type = expression.ResultType.EdmType; if (type.BuiltInTypeKind != BuiltInTypeKind.RowType) { // restrict initialization of non-row types to the target of the view if (!_setMapping.Set.ElementType.IsAssignableFrom(type)) { _errors.Add(new EdmSchemaError(System.Data.Entity.Strings.Mapping_UnsupportedInitialization_QueryView_2( _setMapping.Set.Name, type.FullName), (int)StorageMappingErrorCode.MappingUnsupportedInitializationQueryView, EdmSchemaErrorSeverity.Error, _setMapping.EntityContainerMapping.SourceLocation, _setMapping.StartLineNumber, _setMapping.StartLinePosition)); } } } public override void Visit(DbScanExpression expression) { base.Visit(expression); Debug.Assert(null != expression.Target); // verify scan target is in S-space EntitySetBase target = expression.Target; EntityContainer targetContainer = target.EntityContainer; Debug.Assert(null != target.EntityContainer); if ((targetContainer.DataSpace != DataSpace.SSpace)) { _errors.Add(new EdmSchemaError(System.Data.Entity.Strings.Mapping_UnsupportedScanTarget_QueryView_2( _setMapping.Set.Name, target.Name), (int)StorageMappingErrorCode.MappingUnsupportedScanTargetQueryView, EdmSchemaErrorSeverity.Error, _setMapping.EntityContainerMapping.SourceLocation, _setMapping.StartLineNumber, _setMapping.StartLinePosition)); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System.Data.Metadata.Edm; using System.Globalization; using System.Data.Common.CommandTrees; using System.Collections.Generic; using System.Diagnostics; using System.Data.Entity; namespace System.Data.Mapping { ////// Verifies that only legal expressions exist in a user-defined query mapping view. /// /// - ViewExpr = (see ViewExpressionValidator) /// Project(Projection, ViewExpr) | /// Project(Value, ViewExpr) | /// Filter(ViewExpr, ViewExpr) | /// Join(ViewExpr, ViewExpr, ViewExpr) | /// UnionAll(ViewExpr, ViewExpr) | /// Scan(S-Space EntitySet) /// Constant | /// Property{StructuralProperty}(ViewExpr) | /// Null | /// VariableReference | /// Cast(ViewExpr) | /// Case([Predicate, ViewExpr]*, ViewExpr) | /// Comparison(ViewExpr, ViewExpr) | /// Not(ViewExpr) | /// And(ViewExpr, ViewExpr) | /// Or(ViewExpr, ViewExpr) | /// IsNull(ViewExpr) /// - Projection = (see ViewExpressionValidator.Visit(DbProjectExpression) /// NewInstance{TargetEntity}(ViewExpr*) | /// NewInstance{Row}(ViewExpr*) /// internal static class ViewValidator { ////// Determines whether the given view is valid. /// /// Query view to validate. /// Store item collection. /// Mapping in which view is declared. ///Errors in view definition. internal static IEnumerableValidateQueryView(DbQueryCommandTree view, StoreItemCollection storeItemCollection, StorageSetMapping setMapping, EntityTypeBase elementType, bool includeSubtypes) { ViewExpressionValidator validator = new ViewExpressionValidator(storeItemCollection, setMapping, elementType, includeSubtypes); validator.VisitExpression(view.Query); return validator.Errors; } private sealed class ViewExpressionValidator : BasicExpressionVisitor { private readonly StorageSetMapping _setMapping; private readonly StoreItemCollection _storeItemCollection; private readonly List _errors; private readonly EntityTypeBase _elementType; private readonly bool _includeSubtypes; internal ViewExpressionValidator(StoreItemCollection storeItemCollection, StorageSetMapping setMapping, EntityTypeBase elementType, bool includeSubtypes) { Debug.Assert(null != setMapping); Debug.Assert(null != storeItemCollection); _setMapping = setMapping; _storeItemCollection = storeItemCollection; _errors = new List (); _elementType = elementType; _includeSubtypes = includeSubtypes; } internal IEnumerable Errors { get { return _errors; } } public override void VisitExpression(DbExpression expression) { if (null != expression) { ValidateExpressionKind(expression.ExpressionKind); } base.VisitExpression(expression); } private void ValidateExpressionKind(DbExpressionKind expressionKind) { switch (expressionKind) { // Supported expression kinds case DbExpressionKind.Constant: case DbExpressionKind.Property: case DbExpressionKind.Null: case DbExpressionKind.VariableReference: case DbExpressionKind.Cast: case DbExpressionKind.Case: case DbExpressionKind.Not: case DbExpressionKind.Or: case DbExpressionKind.And: case DbExpressionKind.IsNull: case DbExpressionKind.Equals: case DbExpressionKind.NotEquals: case DbExpressionKind.LessThan: case DbExpressionKind.LessThanOrEquals: case DbExpressionKind.GreaterThan: case DbExpressionKind.GreaterThanOrEquals: case DbExpressionKind.Project: case DbExpressionKind.NewInstance: case DbExpressionKind.Filter: case DbExpressionKind.Ref: case DbExpressionKind.UnionAll: case DbExpressionKind.Scan: case DbExpressionKind.FullOuterJoin: case DbExpressionKind.LeftOuterJoin: case DbExpressionKind.InnerJoin: case DbExpressionKind.EntityRef: break; default: string elementString = (_includeSubtypes)?"IsTypeOf("+_elementType.ToString()+")":_elementType.ToString(); _errors.Add(new EdmSchemaError(System.Data.Entity.Strings.Mapping_UnsupportedExpressionKind_QueryView_2( _setMapping.Set.Name, elementString, expressionKind), (int)StorageMappingErrorCode.MappingUnsupportedExpressionKindQueryView, EdmSchemaErrorSeverity.Error, _setMapping.EntityContainerMapping.SourceLocation, _setMapping.StartLineNumber, _setMapping.StartLinePosition)); break; } } public override void Visit(DbPropertyExpression expression) { base.Visit(expression); if (expression.Property.BuiltInTypeKind != BuiltInTypeKind.EdmProperty) { _errors.Add(new EdmSchemaError(System.Data.Entity.Strings.Mapping_UnsupportedPropertyKind_QueryView_3( _setMapping.Set.Name, expression.Property.Name, expression.Property.BuiltInTypeKind), (int)StorageMappingErrorCode.MappingUnsupportedPropertyKindQueryView, EdmSchemaErrorSeverity.Error, _setMapping.EntityContainerMapping.SourceLocation, _setMapping.StartLineNumber, _setMapping.StartLinePosition)); } } public override void Visit(DbNewInstanceExpression expression) { base.Visit(expression); EdmType type = expression.ResultType.EdmType; if (type.BuiltInTypeKind != BuiltInTypeKind.RowType) { // restrict initialization of non-row types to the target of the view if (!_setMapping.Set.ElementType.IsAssignableFrom(type)) { _errors.Add(new EdmSchemaError(System.Data.Entity.Strings.Mapping_UnsupportedInitialization_QueryView_2( _setMapping.Set.Name, type.FullName), (int)StorageMappingErrorCode.MappingUnsupportedInitializationQueryView, EdmSchemaErrorSeverity.Error, _setMapping.EntityContainerMapping.SourceLocation, _setMapping.StartLineNumber, _setMapping.StartLinePosition)); } } } public override void Visit(DbScanExpression expression) { base.Visit(expression); Debug.Assert(null != expression.Target); // verify scan target is in S-space EntitySetBase target = expression.Target; EntityContainer targetContainer = target.EntityContainer; Debug.Assert(null != target.EntityContainer); if ((targetContainer.DataSpace != DataSpace.SSpace)) { _errors.Add(new EdmSchemaError(System.Data.Entity.Strings.Mapping_UnsupportedScanTarget_QueryView_2( _setMapping.Set.Name, target.Name), (int)StorageMappingErrorCode.MappingUnsupportedScanTargetQueryView, EdmSchemaErrorSeverity.Error, _setMapping.EntityContainerMapping.SourceLocation, _setMapping.StartLineNumber, _setMapping.StartLinePosition)); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
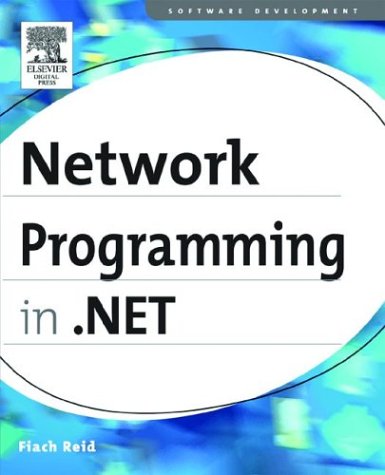
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TypeUsageBuilder.cs
- LayoutManager.cs
- ToolStrip.cs
- CodeEntryPointMethod.cs
- InvalidProgramException.cs
- PropertyEmitter.cs
- SafeSecurityHelper.cs
- HtmlTableRow.cs
- PriorityBindingExpression.cs
- DesignerDataView.cs
- CounterSetInstanceCounterDataSet.cs
- CodeMemberEvent.cs
- ObjectSpanRewriter.cs
- SQLBinary.cs
- EncoderFallback.cs
- SelectionRangeConverter.cs
- SafePEFileHandle.cs
- RoleBoolean.cs
- EncryptedPackageFilter.cs
- Tablet.cs
- DeferredReference.cs
- FlowDocumentScrollViewer.cs
- DataServiceConfiguration.cs
- CryptoHandle.cs
- SoapDocumentServiceAttribute.cs
- XmlMembersMapping.cs
- DataGridViewButtonColumn.cs
- GradientStop.cs
- GeometryCollection.cs
- SettingsPropertyCollection.cs
- XmlSyndicationContent.cs
- ExternalFile.cs
- DataBindingExpressionBuilder.cs
- DataGridCheckBoxColumn.cs
- KnowledgeBase.cs
- XmlnsCache.cs
- ObjectDataSourceChooseTypePanel.cs
- columnmapfactory.cs
- QuaternionRotation3D.cs
- ThemeableAttribute.cs
- WaitHandle.cs
- UserNameSecurityTokenProvider.cs
- BufferedReadStream.cs
- DomainConstraint.cs
- ExpressionParser.cs
- StringValueSerializer.cs
- TableDesigner.cs
- BindingOperations.cs
- Win32Exception.cs
- userdatakeys.cs
- XdrBuilder.cs
- ProfileModule.cs
- DataRecordInternal.cs
- RangeBaseAutomationPeer.cs
- GroupBox.cs
- UniqueConstraint.cs
- SqlInfoMessageEvent.cs
- SystemInfo.cs
- InputGestureCollection.cs
- TimeSpanSecondsOrInfiniteConverter.cs
- ForEach.cs
- IPEndPoint.cs
- DataGridViewMethods.cs
- DoubleAnimationClockResource.cs
- SqlClientMetaDataCollectionNames.cs
- DTCTransactionManager.cs
- DbgUtil.cs
- ToolStripRenderEventArgs.cs
- QueryRewriter.cs
- BufferedStream.cs
- GridPattern.cs
- System.Data.OracleClient_BID.cs
- PerformanceCounter.cs
- HostedElements.cs
- XomlCompilerParameters.cs
- ListViewDeleteEventArgs.cs
- SelectionRangeConverter.cs
- RunWorkerCompletedEventArgs.cs
- HttpServerVarsCollection.cs
- SQLInt32Storage.cs
- HasCopySemanticsAttribute.cs
- UnsafeNativeMethods.cs
- SessionStateModule.cs
- ValueTable.cs
- SafeNativeMethods.cs
- CompositeDuplexBindingElement.cs
- Line.cs
- CodeAccessPermission.cs
- ApplicationSecurityInfo.cs
- MediaElementAutomationPeer.cs
- RadioButtonStandardAdapter.cs
- RotateTransform3D.cs
- QueryGenerator.cs
- RoleManagerEventArgs.cs
- SoapFault.cs
- List.cs
- WorkflowOperationBehavior.cs
- WithParamAction.cs
- EncodingNLS.cs
- PreProcessInputEventArgs.cs