Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CompMod / System / ComponentModel / Win32Exception.cs / 1305376 / Win32Exception.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel { using Microsoft.Win32; using System; using System.Diagnostics; using System.Runtime.InteropServices; using System.Runtime.Remoting; using System.Runtime.Serialization; using System.Security; using System.Security.Permissions; using System.Text; ////// // Code already shipped - safe to place link demand on derived class constructor when base doesn't have it - Suppress message. [HostProtection(SharedState = true)] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] [Serializable] [SuppressUnmanagedCodeSecurity] public class Win32Exception : ExternalException, ISerializable { ///The exception that is thrown for a Win32 error code. ////// private readonly int nativeErrorCode; ///Represents the Win32 error code associated with this exception. This /// field is read-only. ////// [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] public Win32Exception() : this(Marshal.GetLastWin32Error()) { } ///Initializes a new instance of the ///class with the last Win32 error /// that occured. /// [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] public Win32Exception(int error) : this(error, GetErrorMessage(error)) { } ///Initializes a new instance of the ///class with the specified error. /// [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] public Win32Exception(int error, string message) : base(message) { nativeErrorCode = error; } ///Initializes a new instance of the ///class with the specified error and the /// specified detailed description. /// Initializes a new instance of the Exception class with a specified error message. /// FxCop CA1032: Multiple constructors are required to correctly implement a custom exception. /// [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] public Win32Exception( string message ) : this(Marshal.GetLastWin32Error(), message) { } ////// Initializes a new instance of the Exception class with a specified error message and a /// reference to the inner exception that is the cause of this exception. /// FxCop CA1032: Multiple constructors are required to correctly implement a custom exception. /// [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] public Win32Exception( string message, Exception innerException ) : base(message, innerException) { nativeErrorCode = Marshal.GetLastWin32Error(); } protected Win32Exception(SerializationInfo info, StreamingContext context) : base (info, context) { IntSecurity.UnmanagedCode.Demand(); nativeErrorCode = info.GetInt32("NativeErrorCode"); } ////// public int NativeErrorCode { get { return nativeErrorCode; } } private static string GetErrorMessage(int error) { //get the system error message... string errorMsg = ""; StringBuilder sb = new StringBuilder(256); int result = SafeNativeMethods.FormatMessage( SafeNativeMethods.FORMAT_MESSAGE_IGNORE_INSERTS | SafeNativeMethods.FORMAT_MESSAGE_FROM_SYSTEM | SafeNativeMethods.FORMAT_MESSAGE_ARGUMENT_ARRAY, NativeMethods.NullHandleRef, error, 0, sb, sb.Capacity + 1, IntPtr.Zero); if (result != 0) { int i = sb.Length; while (i > 0) { char ch = sb[i - 1]; if (ch > 32 && ch != '.') break; i--; } errorMsg = sb.ToString(0, i); } else { errorMsg ="Unknown error (0x" + Convert.ToString(error, 16) + ")"; } return errorMsg; } // Even though all we're exposing is the nativeErrorCode (which is also available via public property) // it's not a bad idea to have this in place. Later, if more fields are added to this exception, // we won't need to worry about accidentaly exposing them through this interface. [SecurityPermissionAttribute(SecurityAction.Demand,SerializationFormatter=true)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { if (info==null) { throw new ArgumentNullException("info"); } info.AddValue("NativeErrorCode", nativeErrorCode); base.GetObjectData(info, context); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //Represents the Win32 error code associated with this exception. This /// field is read-only. ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel { using Microsoft.Win32; using System; using System.Diagnostics; using System.Runtime.InteropServices; using System.Runtime.Remoting; using System.Runtime.Serialization; using System.Security; using System.Security.Permissions; using System.Text; ////// // Code already shipped - safe to place link demand on derived class constructor when base doesn't have it - Suppress message. [HostProtection(SharedState = true)] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] [Serializable] [SuppressUnmanagedCodeSecurity] public class Win32Exception : ExternalException, ISerializable { ///The exception that is thrown for a Win32 error code. ////// private readonly int nativeErrorCode; ///Represents the Win32 error code associated with this exception. This /// field is read-only. ////// [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] public Win32Exception() : this(Marshal.GetLastWin32Error()) { } ///Initializes a new instance of the ///class with the last Win32 error /// that occured. /// [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] public Win32Exception(int error) : this(error, GetErrorMessage(error)) { } ///Initializes a new instance of the ///class with the specified error. /// [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] public Win32Exception(int error, string message) : base(message) { nativeErrorCode = error; } ///Initializes a new instance of the ///class with the specified error and the /// specified detailed description. /// Initializes a new instance of the Exception class with a specified error message. /// FxCop CA1032: Multiple constructors are required to correctly implement a custom exception. /// [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] public Win32Exception( string message ) : this(Marshal.GetLastWin32Error(), message) { } ////// Initializes a new instance of the Exception class with a specified error message and a /// reference to the inner exception that is the cause of this exception. /// FxCop CA1032: Multiple constructors are required to correctly implement a custom exception. /// [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] public Win32Exception( string message, Exception innerException ) : base(message, innerException) { nativeErrorCode = Marshal.GetLastWin32Error(); } protected Win32Exception(SerializationInfo info, StreamingContext context) : base (info, context) { IntSecurity.UnmanagedCode.Demand(); nativeErrorCode = info.GetInt32("NativeErrorCode"); } ////// public int NativeErrorCode { get { return nativeErrorCode; } } private static string GetErrorMessage(int error) { //get the system error message... string errorMsg = ""; StringBuilder sb = new StringBuilder(256); int result = SafeNativeMethods.FormatMessage( SafeNativeMethods.FORMAT_MESSAGE_IGNORE_INSERTS | SafeNativeMethods.FORMAT_MESSAGE_FROM_SYSTEM | SafeNativeMethods.FORMAT_MESSAGE_ARGUMENT_ARRAY, NativeMethods.NullHandleRef, error, 0, sb, sb.Capacity + 1, IntPtr.Zero); if (result != 0) { int i = sb.Length; while (i > 0) { char ch = sb[i - 1]; if (ch > 32 && ch != '.') break; i--; } errorMsg = sb.ToString(0, i); } else { errorMsg ="Unknown error (0x" + Convert.ToString(error, 16) + ")"; } return errorMsg; } // Even though all we're exposing is the nativeErrorCode (which is also available via public property) // it's not a bad idea to have this in place. Later, if more fields are added to this exception, // we won't need to worry about accidentaly exposing them through this interface. [SecurityPermissionAttribute(SecurityAction.Demand,SerializationFormatter=true)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { if (info==null) { throw new ArgumentNullException("info"); } info.AddValue("NativeErrorCode", nativeErrorCode); base.GetObjectData(info, context); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Represents the Win32 error code associated with this exception. This /// field is read-only. ///
Link Menu
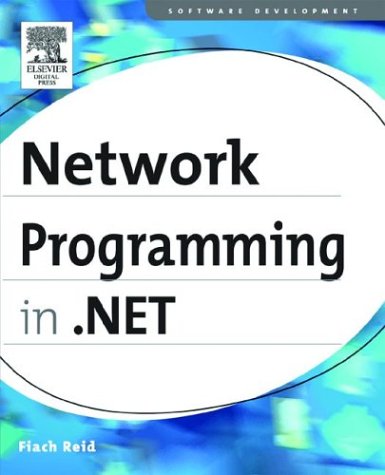
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PageTheme.cs
- ForEachAction.cs
- LogPolicy.cs
- SiteMapDataSourceView.cs
- OutputCacheSettings.cs
- XmlValidatingReaderImpl.cs
- OdbcParameter.cs
- ForeignKeyFactory.cs
- MessageFault.cs
- AlternateViewCollection.cs
- GridErrorDlg.cs
- __FastResourceComparer.cs
- TraceContextEventArgs.cs
- AdapterUtil.cs
- TagPrefixAttribute.cs
- AssociationSet.cs
- FacetValues.cs
- DebugHandleTracker.cs
- CorrelationQuery.cs
- OdbcPermission.cs
- WriteLine.cs
- AvTrace.cs
- GenericUI.cs
- OleDbConnectionPoolGroupProviderInfo.cs
- DrawListViewSubItemEventArgs.cs
- ResourceContainer.cs
- BufferedReadStream.cs
- CollectionViewGroup.cs
- ErrorWebPart.cs
- DataGridViewColumnStateChangedEventArgs.cs
- FramingEncoders.cs
- DataSetUtil.cs
- ContentPlaceHolder.cs
- Base64Stream.cs
- CriticalExceptions.cs
- XmlParserContext.cs
- Ref.cs
- ContainerVisual.cs
- SubqueryTrackingVisitor.cs
- XmlILOptimizerVisitor.cs
- RichTextBox.cs
- Highlights.cs
- RowToParametersTransformer.cs
- XamlReader.cs
- SourceFileBuildProvider.cs
- CodeMethodInvokeExpression.cs
- LexicalChunk.cs
- PropertyTab.cs
- BlobPersonalizationState.cs
- ComponentGuaranteesAttribute.cs
- TableLayoutPanel.cs
- CalendarAutomationPeer.cs
- CollectionConverter.cs
- Int64Animation.cs
- WebSysDescriptionAttribute.cs
- MatrixAnimationUsingPath.cs
- TrackingServices.cs
- MetadataProperty.cs
- X509Certificate2Collection.cs
- Script.cs
- ComponentEditorPage.cs
- AttachedPropertyInfo.cs
- InputScopeConverter.cs
- System.Data_BID.cs
- InstanceDescriptor.cs
- GridViewCellAutomationPeer.cs
- InvalidTimeZoneException.cs
- CodeTypeMember.cs
- Assembly.cs
- QueryExpression.cs
- Constants.cs
- XmlNodeReader.cs
- SaveLedgerEntryRequest.cs
- ValidationManager.cs
- PropertyStore.cs
- ShaderEffect.cs
- ServiceContractGenerationContext.cs
- ValidationRuleCollection.cs
- SqlDataRecord.cs
- DataTableMappingCollection.cs
- WebRequestModulesSection.cs
- HtmlTableRowCollection.cs
- ParallelEnumerable.cs
- DataGridHeaderBorder.cs
- InplaceBitmapMetadataWriter.cs
- WebResourceUtil.cs
- NotImplementedException.cs
- EventlogProvider.cs
- dtdvalidator.cs
- CustomAttributeSerializer.cs
- SpeechRecognizer.cs
- SizeAnimationUsingKeyFrames.cs
- PrivilegedConfigurationManager.cs
- Choices.cs
- Inline.cs
- SafeArrayRankMismatchException.cs
- DateTimeValueSerializer.cs
- XamlFilter.cs
- CommandBinding.cs
- RadioButtonList.cs