Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Documents / Inline.cs / 1 / Inline.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Inline element. // // History: // 06/06/2002 : MikeOrr - Created. // 07/10/2002 : MikeOrr - Renamed element/class 'Phrase' -> 'Inline'. // 06/25/2003 : ZhenbinX - Ported to /Rewrote for WCP tree // 10/28/2004 : grzegorz - ContentElements refactoring. // //--------------------------------------------------------------------------- using MS.Internal; namespace System.Windows.Documents { ////// Inline element. /// [TextElementEditingBehaviorAttribute(IsMergeable = true, IsTypographicOnly = true)] public abstract class Inline : TextElement { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Public constructor. /// protected Inline() : base() { } #endregion Constructors //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// A collection of Inlines containing this one in its sequential tree. /// May return null if an element is not inserted into any tree. /// public InlineCollection SiblingInlines { get { if (this.Parent == null) { return null; } return new InlineCollection(this, /*isOwnerParent*/false); } } ////// Returns an Inline immediately following this one /// on the same level of siblings /// public Inline NextInline { get { return this.NextElement as Inline; } } ////// Returns an Inline immediately preceding this one /// on the same level of siblings /// public Inline PreviousInline { get { return this.PreviousElement as Inline; } } ////// DependencyProperty for public static readonly DependencyProperty BaselineAlignmentProperty = DependencyProperty.Register( "BaselineAlignment", typeof(BaselineAlignment), typeof(Inline), new FrameworkPropertyMetadata( BaselineAlignment.Baseline, FrameworkPropertyMetadataOptions.AffectsParentMeasure), new ValidateValueCallback(IsValidBaselineAlignment)); ///property. /// /// /// public BaselineAlignment BaselineAlignment { get { return (BaselineAlignment) GetValue(BaselineAlignmentProperty); } set { SetValue(BaselineAlignmentProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty TextDecorationsProperty = DependencyProperty.Register( "TextDecorations", typeof(TextDecorationCollection), typeof(Inline), new FrameworkPropertyMetadata( new FreezableDefaultValueFactory(TextDecorationCollection.Empty), FrameworkPropertyMetadataOptions.AffectsRender )); ///property. /// /// The TextDecorations property specifies decorations that are added to the text of an element. /// public TextDecorationCollection TextDecorations { get { return (TextDecorationCollection) GetValue(TextDecorationsProperty); } set { SetValue(TextDecorationsProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty FlowDirectionProperty = FrameworkElement.FlowDirectionProperty.AddOwner(typeof(Inline)); ///property. /// /// The FlowDirection property specifies the flow direction of the element. /// public FlowDirection FlowDirection { get { return (FlowDirection)GetValue(FlowDirectionProperty); } set { SetValue(FlowDirectionProperty, value); } } #endregion Public Properties //-------------------------------------------------------------------- // // Internal Methods // //-------------------------------------------------------------------- #region Internal Methods internal static Run CreateImplicitRun(DependencyObject parent) { return new Run(); } internal static InlineUIContainer CreateImplicitInlineUIContainer(DependencyObject parent) { return new InlineUIContainer(); } #endregion Internal Methods //------------------------------------------------------------------- // // Private Methods // //-------------------------------------------------------------------- #region Private Methods private static bool IsValidBaselineAlignment(object o) { BaselineAlignment value = (BaselineAlignment)o; return value == BaselineAlignment.Baseline || value == BaselineAlignment.Bottom || value == BaselineAlignment.Center || value == BaselineAlignment.Subscript || value == BaselineAlignment.Superscript || value == BaselineAlignment.TextBottom || value == BaselineAlignment.TextTop || value == BaselineAlignment.Top; } #endregion Private Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Inline element. // // History: // 06/06/2002 : MikeOrr - Created. // 07/10/2002 : MikeOrr - Renamed element/class 'Phrase' -> 'Inline'. // 06/25/2003 : ZhenbinX - Ported to /Rewrote for WCP tree // 10/28/2004 : grzegorz - ContentElements refactoring. // //--------------------------------------------------------------------------- using MS.Internal; namespace System.Windows.Documents { ////// Inline element. /// [TextElementEditingBehaviorAttribute(IsMergeable = true, IsTypographicOnly = true)] public abstract class Inline : TextElement { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Public constructor. /// protected Inline() : base() { } #endregion Constructors //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// A collection of Inlines containing this one in its sequential tree. /// May return null if an element is not inserted into any tree. /// public InlineCollection SiblingInlines { get { if (this.Parent == null) { return null; } return new InlineCollection(this, /*isOwnerParent*/false); } } ////// Returns an Inline immediately following this one /// on the same level of siblings /// public Inline NextInline { get { return this.NextElement as Inline; } } ////// Returns an Inline immediately preceding this one /// on the same level of siblings /// public Inline PreviousInline { get { return this.PreviousElement as Inline; } } ////// DependencyProperty for public static readonly DependencyProperty BaselineAlignmentProperty = DependencyProperty.Register( "BaselineAlignment", typeof(BaselineAlignment), typeof(Inline), new FrameworkPropertyMetadata( BaselineAlignment.Baseline, FrameworkPropertyMetadataOptions.AffectsParentMeasure), new ValidateValueCallback(IsValidBaselineAlignment)); ///property. /// /// /// public BaselineAlignment BaselineAlignment { get { return (BaselineAlignment) GetValue(BaselineAlignmentProperty); } set { SetValue(BaselineAlignmentProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty TextDecorationsProperty = DependencyProperty.Register( "TextDecorations", typeof(TextDecorationCollection), typeof(Inline), new FrameworkPropertyMetadata( new FreezableDefaultValueFactory(TextDecorationCollection.Empty), FrameworkPropertyMetadataOptions.AffectsRender )); ///property. /// /// The TextDecorations property specifies decorations that are added to the text of an element. /// public TextDecorationCollection TextDecorations { get { return (TextDecorationCollection) GetValue(TextDecorationsProperty); } set { SetValue(TextDecorationsProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty FlowDirectionProperty = FrameworkElement.FlowDirectionProperty.AddOwner(typeof(Inline)); ///property. /// /// The FlowDirection property specifies the flow direction of the element. /// public FlowDirection FlowDirection { get { return (FlowDirection)GetValue(FlowDirectionProperty); } set { SetValue(FlowDirectionProperty, value); } } #endregion Public Properties //-------------------------------------------------------------------- // // Internal Methods // //-------------------------------------------------------------------- #region Internal Methods internal static Run CreateImplicitRun(DependencyObject parent) { return new Run(); } internal static InlineUIContainer CreateImplicitInlineUIContainer(DependencyObject parent) { return new InlineUIContainer(); } #endregion Internal Methods //------------------------------------------------------------------- // // Private Methods // //-------------------------------------------------------------------- #region Private Methods private static bool IsValidBaselineAlignment(object o) { BaselineAlignment value = (BaselineAlignment)o; return value == BaselineAlignment.Baseline || value == BaselineAlignment.Bottom || value == BaselineAlignment.Center || value == BaselineAlignment.Subscript || value == BaselineAlignment.Superscript || value == BaselineAlignment.TextBottom || value == BaselineAlignment.TextTop || value == BaselineAlignment.Top; } #endregion Private Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
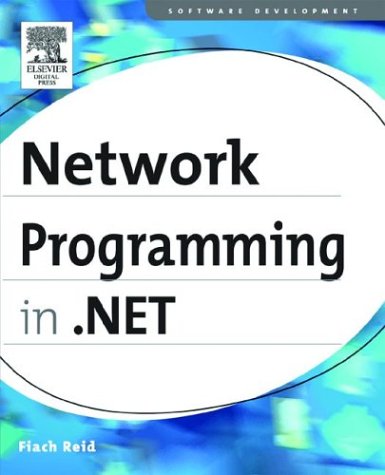
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Types.cs
- ServiceDescriptionSerializer.cs
- NamespaceTable.cs
- TablePattern.cs
- UpdatePanel.cs
- ToolStripItem.cs
- DefaultPropertiesToSend.cs
- InputProcessorProfilesLoader.cs
- CardSpaceException.cs
- ControlHelper.cs
- StringFormat.cs
- LocatorManager.cs
- PerfCounters.cs
- DataPagerFieldCommandEventArgs.cs
- FilteredSchemaElementLookUpTable.cs
- TriggerBase.cs
- Operand.cs
- SoapAttributes.cs
- WsatServiceAddress.cs
- ReadOnlyHierarchicalDataSourceView.cs
- EndpointAddress.cs
- EventLogInformation.cs
- ReadOnlyAttribute.cs
- PersistencePipeline.cs
- TransformedBitmap.cs
- BinaryUtilClasses.cs
- MouseGestureConverter.cs
- CompiledQueryCacheKey.cs
- GeneralTransform3DGroup.cs
- WebConfigurationManager.cs
- SQLBinary.cs
- EntityDataSourceContextCreatingEventArgs.cs
- PeerNameRecordCollection.cs
- ConnectionManagementSection.cs
- FixedDocumentSequencePaginator.cs
- FlatButtonAppearance.cs
- MdiWindowListItemConverter.cs
- ColumnClickEvent.cs
- DBSqlParserColumn.cs
- Splitter.cs
- MinimizableAttributeTypeConverter.cs
- DesignTableCollection.cs
- WindowsPrincipal.cs
- StateChangeEvent.cs
- IFlowDocumentViewer.cs
- TextDecoration.cs
- Set.cs
- MappingException.cs
- Axis.cs
- WorkflowElementDialog.cs
- TransformedBitmap.cs
- TemplateApplicationHelper.cs
- XmlSerializer.cs
- GeneralTransformGroup.cs
- BlockingCollection.cs
- GeneralTransform3DGroup.cs
- DeviceContext.cs
- WebPartTracker.cs
- SoapHelper.cs
- ConfigXmlComment.cs
- AuthenticateEventArgs.cs
- BaseInfoTable.cs
- PathFigure.cs
- ContextProperty.cs
- CompilationUtil.cs
- BindingCollection.cs
- SimpleHandlerBuildProvider.cs
- precedingsibling.cs
- EdmToObjectNamespaceMap.cs
- TypeLoadException.cs
- ObjectPropertyMapping.cs
- ThaiBuddhistCalendar.cs
- MemoryResponseElement.cs
- PreservationFileReader.cs
- ColumnMap.cs
- Rfc2898DeriveBytes.cs
- XmlAttributeOverrides.cs
- XmlIgnoreAttribute.cs
- securitycriticaldataClass.cs
- X509LogoTypeExtension.cs
- Queue.cs
- TextEditorMouse.cs
- DataColumnPropertyDescriptor.cs
- XPathParser.cs
- Int16AnimationBase.cs
- Operators.cs
- DataGrid.cs
- SafeEventLogWriteHandle.cs
- Int32CAMarshaler.cs
- SecurityTokenException.cs
- EventLogEntry.cs
- SafeNativeMethods.cs
- ClientType.cs
- TextParaClient.cs
- BaseAsyncResult.cs
- DataContractSerializerFaultFormatter.cs
- XmlSerializerFactory.cs
- TextSearch.cs
- HtmlWindowCollection.cs
- ConfigXmlText.cs