Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / Microsoft / Scripting / Compiler / Set.cs / 1305376 / Set.cs
/* **************************************************************************** * * Copyright (c) Microsoft Corporation. * * This source code is subject to terms and conditions of the Microsoft Public License. A * copy of the license can be found in the License.html file at the root of this distribution. If * you cannot locate the Microsoft Public License, please send an email to * dlr@microsoft.com. By using this source code in any fashion, you are agreeing to be bound * by the terms of the Microsoft Public License. * * You must not remove this notice, or any other, from this software. * * * ***************************************************************************/ using System.Collections; using System.Collections.Generic; // Note: can't move to Utils because name conflicts with System.Linq.Set namespace System.Linq.Expressions { ////// A simple hashset, built on Dictionary{K, V} /// internal sealed class Set: ICollection { private readonly Dictionary _data; internal Set() { _data = new Dictionary (); } internal Set(IEqualityComparer comparer) { _data = new Dictionary (comparer); } internal Set(IList list) { _data = new Dictionary (list.Count); foreach (T t in list) { Add(t); } } internal Set(IEnumerable list) { _data = new Dictionary (); foreach (T t in list) { Add(t); } } internal Set(int capacity) { _data = new Dictionary (capacity); } public void Add(T item) { _data[item] = null; } public void Clear() { _data.Clear(); } public bool Contains(T item) { return _data.ContainsKey(item); } public void CopyTo(T[] array, int arrayIndex) { _data.Keys.CopyTo(array, arrayIndex); } public int Count { get { return _data.Count; } } public bool IsReadOnly { get { return false; } } public bool Remove(T item) { return _data.Remove(item); } public IEnumerator GetEnumerator() { return _data.Keys.GetEnumerator(); } System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { return _data.Keys.GetEnumerator(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /* **************************************************************************** * * Copyright (c) Microsoft Corporation. * * This source code is subject to terms and conditions of the Microsoft Public License. A * copy of the license can be found in the License.html file at the root of this distribution. If * you cannot locate the Microsoft Public License, please send an email to * dlr@microsoft.com. By using this source code in any fashion, you are agreeing to be bound * by the terms of the Microsoft Public License. * * You must not remove this notice, or any other, from this software. * * * ***************************************************************************/ using System.Collections; using System.Collections.Generic; // Note: can't move to Utils because name conflicts with System.Linq.Set namespace System.Linq.Expressions { /// /// A simple hashset, built on Dictionary{K, V} /// internal sealed class Set: ICollection { private readonly Dictionary _data; internal Set() { _data = new Dictionary (); } internal Set(IEqualityComparer comparer) { _data = new Dictionary (comparer); } internal Set(IList list) { _data = new Dictionary (list.Count); foreach (T t in list) { Add(t); } } internal Set(IEnumerable list) { _data = new Dictionary (); foreach (T t in list) { Add(t); } } internal Set(int capacity) { _data = new Dictionary (capacity); } public void Add(T item) { _data[item] = null; } public void Clear() { _data.Clear(); } public bool Contains(T item) { return _data.ContainsKey(item); } public void CopyTo(T[] array, int arrayIndex) { _data.Keys.CopyTo(array, arrayIndex); } public int Count { get { return _data.Count; } } public bool IsReadOnly { get { return false; } } public bool Remove(T item) { return _data.Remove(item); } public IEnumerator GetEnumerator() { return _data.Keys.GetEnumerator(); } System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { return _data.Keys.GetEnumerator(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
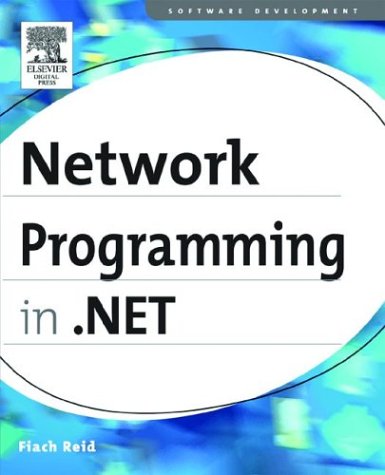
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Logging.cs
- SqlServices.cs
- HtmlPageAdapter.cs
- WebConfigurationManager.cs
- WindowsSysHeader.cs
- BindingOperations.cs
- CapabilitiesRule.cs
- DataFormats.cs
- TriggerActionCollection.cs
- SspiNegotiationTokenProvider.cs
- HyperlinkAutomationPeer.cs
- DataControlImageButton.cs
- SingleStorage.cs
- SqlOuterApplyReducer.cs
- ToolStripDropDownItem.cs
- SpecialFolderEnumConverter.cs
- ProfileGroupSettings.cs
- MergeFailedEvent.cs
- TextTreeFixupNode.cs
- AnnotationHelper.cs
- GenericNameHandler.cs
- EndpointAddress.cs
- EmbossBitmapEffect.cs
- Assert.cs
- BookmarkOptionsHelper.cs
- ModelItemExtensions.cs
- DataGridViewColumnConverter.cs
- IntSecurity.cs
- RequestCachePolicy.cs
- DataViewSetting.cs
- CollectionEditVerbManager.cs
- StringSource.cs
- SqlCaseSimplifier.cs
- CookieParameter.cs
- BindingFormattingDialog.cs
- GacUtil.cs
- DBConnectionString.cs
- QilNode.cs
- ProtocolsSection.cs
- StyleModeStack.cs
- XmlSchemaAny.cs
- HtmlGenericControl.cs
- TypeContext.cs
- ValidatorAttribute.cs
- DataGridViewColumnEventArgs.cs
- SortQuery.cs
- RuleAttributes.cs
- StylusDownEventArgs.cs
- SQLInt16Storage.cs
- GridViewUpdatedEventArgs.cs
- OpacityConverter.cs
- ObjectViewFactory.cs
- _NTAuthentication.cs
- WebPermission.cs
- FrameworkContentElement.cs
- BufferedGraphics.cs
- ListArgumentProvider.cs
- Utility.cs
- DataBindingCollectionConverter.cs
- Rect.cs
- ComboBoxRenderer.cs
- TouchesCapturedWithinProperty.cs
- SoapSchemaImporter.cs
- StateMachineSubscription.cs
- AsyncResult.cs
- HashCodeCombiner.cs
- DataGridViewButtonCell.cs
- StandardOleMarshalObject.cs
- XmlUnspecifiedAttribute.cs
- NativeMethods.cs
- WrapperSecurityCommunicationObject.cs
- LayoutTable.cs
- ListViewInsertEventArgs.cs
- BitmapEffectGroup.cs
- StateDesignerConnector.cs
- DataGridAddNewRow.cs
- SplashScreenNativeMethods.cs
- HandledMouseEvent.cs
- WebPart.cs
- TimelineCollection.cs
- autovalidator.cs
- SamlAuthorizationDecisionStatement.cs
- IInstanceTable.cs
- PerformanceCounterPermission.cs
- StickyNoteHelper.cs
- ObjectItemAssemblyLoader.cs
- _PooledStream.cs
- FrameworkElement.cs
- ContentPosition.cs
- ExecutionEngineException.cs
- ContractListAdapter.cs
- IndependentlyAnimatedPropertyMetadata.cs
- ObjectSet.cs
- DispatcherHookEventArgs.cs
- PathGradientBrush.cs
- invalidudtexception.cs
- ScrollPatternIdentifiers.cs
- ADConnectionHelper.cs
- SoapAttributeOverrides.cs
- TemplateXamlParser.cs