Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / AddIn / AddIn / System / Addin / Pipeline / ContractListAdapter.cs / 1305376 / ContractListAdapter.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: ContractListAdapter ** ** ===========================================================*/ using System; using System.Collections.Generic; using System.AddIn.Contract; using System.Runtime.Remoting; using System.Diagnostics.Contracts; namespace System.AddIn.Pipeline { internal class ContractListAdapter: IList { private IListContract m_listContract; private Converter m_wrapper; private Converter m_unwrapper; private ContractHandle m_contractHandle; public ContractListAdapter(IListContract source, Converter wrapper, Converter unwrapper) { if (source == null) throw new ArgumentNullException("source"); if (wrapper == null) throw new ArgumentNullException("wrapper"); if (unwrapper == null) throw new ArgumentNullException("unwrapper"); System.Diagnostics.Contracts.Contract.EndContractBlock(); m_listContract = source; m_wrapper = wrapper; m_unwrapper = unwrapper; m_contractHandle = new ContractHandle(m_listContract); } public void Add(U item) { m_listContract.Add(m_unwrapper(item)); } public void Clear() { m_listContract.Clear(); } public U this[int index] { get { return m_wrapper(m_listContract.GetItem(index)); } set { m_listContract.SetItem(index, m_unwrapper(value)); } } public bool Contains(U item) { return m_listContract.Contains(m_unwrapper(item)); } public void CopyTo(U[] destination, int index) { // need to check Count >= destination.Length then copy wrapped (U) instances to the destination array if (destination == null) throw new ArgumentNullException("destination"); if (index < 0) throw new ArgumentOutOfRangeException("index"); System.Diagnostics.Contracts.Contract.EndContractBlock(); int listContractCount = m_listContract.GetCount(); if (index > destination.Length - listContractCount) { throw new ArgumentOutOfRangeException("index"); } for (int i = 0; i < listContractCount; i++) { destination[index++]=m_wrapper(m_listContract.GetItem(i)); } } public int IndexOf(U item) { return m_listContract.IndexOf(m_unwrapper(item)); } public void Insert(int index, U item) { m_listContract.Insert(index, m_unwrapper(item)); } public bool Remove(U item) { return m_listContract.Remove(m_unwrapper(item)); } public void RemoveAt(int index) { m_listContract.RemoveAt(index); } public U GetItem(int index) { T item = m_listContract.GetItem(index); return m_wrapper(item); } public void SetItem(int index, U item) { m_listContract.SetItem(index, m_unwrapper(item)); } public int Count { get { return m_listContract.GetCount(); } } public bool IsReadOnly { get { return m_listContract.GetIsReadOnly(); } } System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { return this.GetEnumerator(); } public IEnumerator GetEnumerator() { return new ContractEnumeratorAdapter (m_listContract.GetEnumeratorContract(), m_wrapper); } } internal class ContractEnumeratorAdapter : IEnumerator { private IEnumeratorContract m_enumerator; private Converter m_wrapper; private ContractHandle m_contractHandle; public ContractEnumeratorAdapter(IEnumeratorContract enumerator, Converter wrapper) { if (enumerator == null) throw new ArgumentNullException("enumerator"); if (wrapper == null) throw new ArgumentNullException("wrapper"); System.Diagnostics.Contracts.Contract.EndContractBlock(); m_enumerator = enumerator; m_wrapper = wrapper; m_contractHandle = new ContractHandle((IContract)m_enumerator); } public U Current { get { T val = m_enumerator.GetCurrent(); return m_wrapper(val); } } object System.Collections.IEnumerator.Current { get { return Current; } } public bool MoveNext() { return m_enumerator.MoveNext(); } public void Reset() { m_enumerator.Reset(); } public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } // Dispose(bool disposing) executes in two distinct scenarios. // If disposing equals true, the method has been called directly // or indirectly by a user's code. Managed and unmanaged resources // can be disposed. // If disposing equals false, the method has been called by the // runtime from inside the finalizer and you should not reference // other objects. Only unmanaged resources can be disposed. void Dispose(bool disposing) { if (m_contractHandle != null ) { if (disposing) { try { m_contractHandle.Dispose(); if (m_enumerator != null) m_enumerator.Dispose(); } catch (AppDomainUnloadedException) { } catch (RemotingException) { } } m_contractHandle = null; } } // Dispose pattern - here for completeness ~ContractEnumeratorAdapter() { Dispose(false); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: ContractListAdapter ** ** ===========================================================*/ using System; using System.Collections.Generic; using System.AddIn.Contract; using System.Runtime.Remoting; using System.Diagnostics.Contracts; namespace System.AddIn.Pipeline { internal class ContractListAdapter : IList { private IListContract m_listContract; private Converter m_wrapper; private Converter m_unwrapper; private ContractHandle m_contractHandle; public ContractListAdapter(IListContract source, Converter wrapper, Converter unwrapper) { if (source == null) throw new ArgumentNullException("source"); if (wrapper == null) throw new ArgumentNullException("wrapper"); if (unwrapper == null) throw new ArgumentNullException("unwrapper"); System.Diagnostics.Contracts.Contract.EndContractBlock(); m_listContract = source; m_wrapper = wrapper; m_unwrapper = unwrapper; m_contractHandle = new ContractHandle(m_listContract); } public void Add(U item) { m_listContract.Add(m_unwrapper(item)); } public void Clear() { m_listContract.Clear(); } public U this[int index] { get { return m_wrapper(m_listContract.GetItem(index)); } set { m_listContract.SetItem(index, m_unwrapper(value)); } } public bool Contains(U item) { return m_listContract.Contains(m_unwrapper(item)); } public void CopyTo(U[] destination, int index) { // need to check Count >= destination.Length then copy wrapped (U) instances to the destination array if (destination == null) throw new ArgumentNullException("destination"); if (index < 0) throw new ArgumentOutOfRangeException("index"); System.Diagnostics.Contracts.Contract.EndContractBlock(); int listContractCount = m_listContract.GetCount(); if (index > destination.Length - listContractCount) { throw new ArgumentOutOfRangeException("index"); } for (int i = 0; i < listContractCount; i++) { destination[index++]=m_wrapper(m_listContract.GetItem(i)); } } public int IndexOf(U item) { return m_listContract.IndexOf(m_unwrapper(item)); } public void Insert(int index, U item) { m_listContract.Insert(index, m_unwrapper(item)); } public bool Remove(U item) { return m_listContract.Remove(m_unwrapper(item)); } public void RemoveAt(int index) { m_listContract.RemoveAt(index); } public U GetItem(int index) { T item = m_listContract.GetItem(index); return m_wrapper(item); } public void SetItem(int index, U item) { m_listContract.SetItem(index, m_unwrapper(item)); } public int Count { get { return m_listContract.GetCount(); } } public bool IsReadOnly { get { return m_listContract.GetIsReadOnly(); } } System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { return this.GetEnumerator(); } public IEnumerator GetEnumerator() { return new ContractEnumeratorAdapter (m_listContract.GetEnumeratorContract(), m_wrapper); } } internal class ContractEnumeratorAdapter : IEnumerator { private IEnumeratorContract m_enumerator; private Converter m_wrapper; private ContractHandle m_contractHandle; public ContractEnumeratorAdapter(IEnumeratorContract enumerator, Converter wrapper) { if (enumerator == null) throw new ArgumentNullException("enumerator"); if (wrapper == null) throw new ArgumentNullException("wrapper"); System.Diagnostics.Contracts.Contract.EndContractBlock(); m_enumerator = enumerator; m_wrapper = wrapper; m_contractHandle = new ContractHandle((IContract)m_enumerator); } public U Current { get { T val = m_enumerator.GetCurrent(); return m_wrapper(val); } } object System.Collections.IEnumerator.Current { get { return Current; } } public bool MoveNext() { return m_enumerator.MoveNext(); } public void Reset() { m_enumerator.Reset(); } public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } // Dispose(bool disposing) executes in two distinct scenarios. // If disposing equals true, the method has been called directly // or indirectly by a user's code. Managed and unmanaged resources // can be disposed. // If disposing equals false, the method has been called by the // runtime from inside the finalizer and you should not reference // other objects. Only unmanaged resources can be disposed. void Dispose(bool disposing) { if (m_contractHandle != null ) { if (disposing) { try { m_contractHandle.Dispose(); if (m_enumerator != null) m_enumerator.Dispose(); } catch (AppDomainUnloadedException) { } catch (RemotingException) { } } m_contractHandle = null; } } // Dispose pattern - here for completeness ~ContractEnumeratorAdapter() { Dispose(false); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
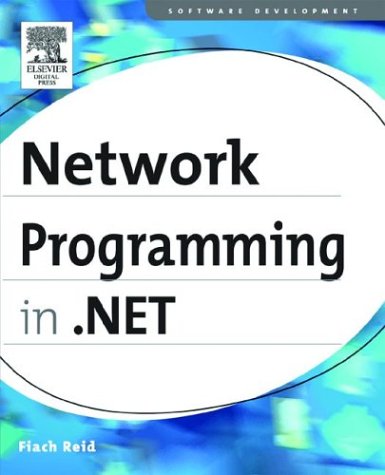
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IPEndPoint.cs
- ZeroOpNode.cs
- TagPrefixInfo.cs
- InputEventArgs.cs
- StringFormat.cs
- CookielessHelper.cs
- HostingEnvironmentWrapper.cs
- GPPOINT.cs
- WebPartTransformerAttribute.cs
- XsltOutput.cs
- PriorityChain.cs
- ProtocolsSection.cs
- CommonDialog.cs
- ADConnectionHelper.cs
- NotifyCollectionChangedEventArgs.cs
- AuthStoreRoleProvider.cs
- XmlSchemaInfo.cs
- GeneralTransform3DCollection.cs
- UnderstoodHeaders.cs
- DbMetaDataCollectionNames.cs
- DateTimeValueSerializer.cs
- SelectionList.cs
- SiteMapDesignerDataSourceView.cs
- IpcManager.cs
- ZipFileInfoCollection.cs
- CodeExpressionCollection.cs
- FixedPageProcessor.cs
- DictionarySectionHandler.cs
- RegisteredScript.cs
- TextContainerHelper.cs
- PostBackTrigger.cs
- ItemChangedEventArgs.cs
- odbcmetadatacolumnnames.cs
- RequestNavigateEventArgs.cs
- XmlSchemaNotation.cs
- TimersDescriptionAttribute.cs
- DetailsViewModeEventArgs.cs
- BuiltInExpr.cs
- DataRowChangeEvent.cs
- HelpProvider.cs
- PopupEventArgs.cs
- SuppressMessageAttribute.cs
- XmlArrayItemAttributes.cs
- DrawListViewItemEventArgs.cs
- PluralizationService.cs
- DeobfuscatingStream.cs
- DoubleStorage.cs
- FieldNameLookup.cs
- TransactionTraceIdentifier.cs
- Random.cs
- XmlNullResolver.cs
- MessageDescriptionCollection.cs
- ButtonFlatAdapter.cs
- DeobfuscatingStream.cs
- ModelPerspective.cs
- XmlElementAttribute.cs
- RequestStatusBarUpdateEventArgs.cs
- HttpApplicationFactory.cs
- Utility.cs
- ToolStripArrowRenderEventArgs.cs
- DefaultValueTypeConverter.cs
- CellConstantDomain.cs
- AssemblyCollection.cs
- LiteralSubsegment.cs
- UmAlQuraCalendar.cs
- DBSqlParserTableCollection.cs
- ServiceBehaviorAttribute.cs
- View.cs
- MouseButton.cs
- ClientScriptManager.cs
- TextRangeSerialization.cs
- ChangeTracker.cs
- ProtocolsConfiguration.cs
- SnapLine.cs
- OleDbConnection.cs
- HttpVersion.cs
- XmlAttributeProperties.cs
- PointAnimationBase.cs
- SemaphoreFullException.cs
- DiscoveryDocumentLinksPattern.cs
- CommonObjectSecurity.cs
- PopupRoot.cs
- SqlDesignerDataSourceView.cs
- RequiredAttributeAttribute.cs
- ImplicitInputBrush.cs
- ValueChangedEventManager.cs
- CatalogPartCollection.cs
- TreeViewDesigner.cs
- OptionUsage.cs
- CaretElement.cs
- IsolatedStorageFile.cs
- DataIdProcessor.cs
- WhereQueryOperator.cs
- ByteViewer.cs
- ChangesetResponse.cs
- DetailsViewRowCollection.cs
- DiscoveryClientReferences.cs
- CodeParameterDeclarationExpression.cs
- JobDuplex.cs
- RSAPKCS1SignatureDeformatter.cs