Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / DrawListViewItemEventArgs.cs / 1 / DrawListViewItemEventArgs.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.ComponentModel; using System.Drawing; using System.Windows.Forms.Internal; using Microsoft.Win32; using System.Windows.Forms.VisualStyles; ////// /// This class contains the information a user needs to paint ListView items. /// public class DrawListViewItemEventArgs : EventArgs { private readonly Graphics graphics; private readonly ListViewItem item; private readonly Rectangle bounds; private readonly int itemIndex; private readonly ListViewItemStates state; private bool drawDefault; ////// /// Creates a new DrawListViewItemEventArgs with the given parameters. /// public DrawListViewItemEventArgs(Graphics graphics, ListViewItem item, Rectangle bounds, int itemIndex, ListViewItemStates state) { this.graphics = graphics; this.item = item; this.bounds = bounds; this.itemIndex = itemIndex; this.state = state; this.drawDefault = false; } ////// /// Causes the item do be drawn by the system instead of owner drawn. /// public bool DrawDefault { get { return drawDefault; } set { drawDefault = value; } } ////// /// Graphics object with which painting should be done. /// public Graphics Graphics { get { return graphics; } } ////// /// The item to be painted. /// public ListViewItem Item { get { return item; } } ////// /// The rectangle outlining the area in which the painting should be done. /// public Rectangle Bounds { get { return bounds; } } ////// /// The index of the item that should be painted. /// public int ItemIndex { get { return itemIndex; } } ////// /// Miscellaneous state information. /// public ListViewItemStates State { get { return state; } } ////// /// Draws the item's background. /// public void DrawBackground() { Brush backBrush = new SolidBrush(item.BackColor); Graphics.FillRectangle(backBrush, bounds); backBrush.Dispose(); } ////// /// Draws a focus rectangle in the given bounds, if the item is focused. In Details View, if FullRowSelect is /// true, the rectangle is drawn around the whole item, else around the first sub-item's text area. /// public void DrawFocusRectangle() { if ((state & ListViewItemStates.Focused) == ListViewItemStates.Focused) { Rectangle focusBounds = bounds; ControlPaint.DrawFocusRectangle(graphics, UpdateBounds(focusBounds, false /*drawText*/), item.ForeColor, item.BackColor); } } ////// /// Draws the item's text (overloaded) - useful only when View != View.Details /// public void DrawText() { DrawText(TextFormatFlags.Left); } ////// /// Draws the item's text (overloaded) - useful only when View != View.Details - takes a TextFormatFlags argument. /// public void DrawText(TextFormatFlags flags) { TextRenderer.DrawText(graphics, item.Text, item.Font, UpdateBounds(bounds, true /*drawText*/), item.ForeColor, flags); } private Rectangle UpdateBounds(Rectangle originalBounds, bool drawText) { Rectangle resultBounds = originalBounds; if (item.ListView.View == View.Details) { // Note: this logic will compute the bounds so they align w/ the system drawn bounds only // for the default font. if (!item.ListView.FullRowSelect && item.SubItems.Count > 0) { ListViewItem.ListViewSubItem subItem = item.SubItems[0]; Size textSize = TextRenderer.MeasureText(subItem.Text, subItem.Font); resultBounds = new Rectangle(originalBounds.X, originalBounds.Y, textSize.Width, textSize.Height); // Add some padding so we paint like the system control paints. resultBounds.X += 4; resultBounds.Width ++; } else { resultBounds.X +=4; resultBounds.Width -= 4; } if (drawText) { resultBounds.X --; } } return resultBounds; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
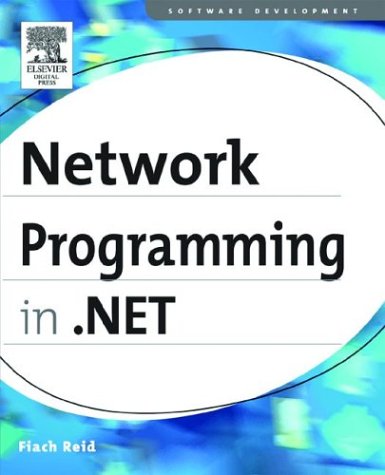
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DirectoryNotFoundException.cs
- _UriTypeConverter.cs
- Int64AnimationUsingKeyFrames.cs
- ConfigXmlText.cs
- ProtocolsConfiguration.cs
- PersonalizationProviderHelper.cs
- ClipboardData.cs
- _ChunkParse.cs
- COM2IDispatchConverter.cs
- DateTimeFormat.cs
- OLEDB_Util.cs
- DefaultHttpHandler.cs
- DataSourceCacheDurationConverter.cs
- RsaKeyIdentifierClause.cs
- InputReport.cs
- ListSortDescriptionCollection.cs
- TableLayoutPanel.cs
- ItemCheckEvent.cs
- XhtmlTextWriter.cs
- TableRow.cs
- RowBinding.cs
- ConnectorRouter.cs
- _HeaderInfo.cs
- EntitySetDataBindingList.cs
- StartUpEventArgs.cs
- WSHttpBindingBaseElement.cs
- InternalBase.cs
- AppSettingsReader.cs
- UnsupportedPolicyOptionsException.cs
- RouteValueExpressionBuilder.cs
- ValidatingPropertiesEventArgs.cs
- ViewKeyConstraint.cs
- ObjectDataSource.cs
- PeerNameResolver.cs
- AdPostCacheSubstitution.cs
- Shared.cs
- Rfc4050KeyFormatter.cs
- InputScope.cs
- RectKeyFrameCollection.cs
- DocumentViewer.cs
- AccessibleObject.cs
- UxThemeWrapper.cs
- OneWayBindingElement.cs
- QilLiteral.cs
- ReadOnlyAttribute.cs
- ADConnectionHelper.cs
- StandardOleMarshalObject.cs
- SqlInternalConnectionTds.cs
- LogManagementAsyncResult.cs
- DBPropSet.cs
- WebPartConnectionsCancelVerb.cs
- EventProviderWriter.cs
- ArrayExtension.cs
- DocumentXmlWriter.cs
- IdentityReference.cs
- ClipboardProcessor.cs
- WebHttpDispatchOperationSelectorData.cs
- ContractBase.cs
- CodeDomDesignerLoader.cs
- _BaseOverlappedAsyncResult.cs
- InputBinding.cs
- XmlSerializerFactory.cs
- Form.cs
- ContextStack.cs
- MarginCollapsingState.cs
- InvalidWorkflowException.cs
- RootBuilder.cs
- XpsFixedPageReaderWriter.cs
- SafeRegistryHandle.cs
- QueryActivatableWorkflowsCommand.cs
- BulletedListEventArgs.cs
- WinFormsSecurity.cs
- CollectionExtensions.cs
- GeneralTransform2DTo3D.cs
- InstanceDescriptor.cs
- TransformCryptoHandle.cs
- ClickablePoint.cs
- BuilderInfo.cs
- CodeLabeledStatement.cs
- BinaryMethodMessage.cs
- XPathParser.cs
- TimeSpanOrInfiniteConverter.cs
- WeakRefEnumerator.cs
- _KerberosClient.cs
- DelayedRegex.cs
- messageonlyhwndwrapper.cs
- SmiContextFactory.cs
- SlotInfo.cs
- LinkConverter.cs
- ClosableStream.cs
- RemotingAttributes.cs
- ReliableSessionBindingElementImporter.cs
- JulianCalendar.cs
- ConsoleCancelEventArgs.cs
- HttpsTransportElement.cs
- RegexNode.cs
- TypeTypeConverter.cs
- XmlWriterSettings.cs
- StackBuilderSink.cs
- XamlTypeMapper.cs