Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / CompMod / System / Runtime / InteropServices / StandardOleMarshalObject.cs / 1 / StandardOleMarshalObject.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Runtime.InteropServices { using System.Diagnostics; using System; using Microsoft.Win32; using System.Security; using System.Security.Permissions; ////// /// /// Replaces the standard CLR free-threaded marshaler with the standard OLE STA one. This prevents the calls made into /// our hosting object by OLE from coming in on threads other than the UI thread. /// /// [ComVisible(true)] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Interoperability", "CA1403:AutoLayoutTypesShouldNotBeComVisible")] public class StandardOleMarshalObject : MarshalByRefObject, UnsafeNativeMethods.IMarshal { protected StandardOleMarshalObject() { } [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] private IntPtr GetStdMarshaller(ref Guid riid, int dwDestContext, int mshlflags) { IntPtr pStdMarshal = IntPtr.Zero; IntPtr pUnk = Marshal.GetIUnknownForObject(this); if (pUnk != IntPtr.Zero) { try { if (NativeMethods.S_OK == UnsafeNativeMethods.CoGetStandardMarshal(ref riid, pUnk, dwDestContext, IntPtr.Zero, mshlflags, out pStdMarshal)) { Debug.Assert(pStdMarshal != null, "Failed to get marshaller for interface '" + riid.ToString() + "', CoGetStandardMarshal returned S_OK"); return pStdMarshal; } } finally { Marshal.Release(pUnk); } } throw new InvalidOperationException(SR.GetString(SR.StandardOleMarshalObjectGetMarshalerFailed, riid.ToString())); } ////// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] int UnsafeNativeMethods.IMarshal.GetUnmarshalClass(ref Guid riid, IntPtr pv, int dwDestContext, IntPtr pvDestContext, int mshlflags, out Guid pCid){ pCid = typeof(UnsafeNativeMethods.IStdMarshal).GUID; return NativeMethods.S_OK; } /// /// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] int UnsafeNativeMethods.IMarshal.GetMarshalSizeMax(ref Guid riid, IntPtr pv, int dwDestContext, IntPtr pvDestContext, int mshlflags, out int pSize) { // 98830 - GUID marshaling in StandardOleMarshalObject AVs on 64-bit Guid riid_copy = riid; IntPtr pStandardMarshal = GetStdMarshaller(ref riid_copy, dwDestContext, mshlflags); try { return UnsafeNativeMethods.CoGetMarshalSizeMax(out pSize, ref riid_copy, pStandardMarshal, dwDestContext, pvDestContext, mshlflags); } finally { Marshal.Release(pStandardMarshal); } } /// /// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] int UnsafeNativeMethods.IMarshal.MarshalInterface(object pStm, ref Guid riid, IntPtr pv, int dwDestContext, IntPtr pvDestContext, int mshlflags) { IntPtr pStandardMarshal = GetStdMarshaller(ref riid, dwDestContext, mshlflags); try { return UnsafeNativeMethods.CoMarshalInterface(pStm, ref riid, pStandardMarshal, dwDestContext, pvDestContext, mshlflags); } finally { Marshal.Release(pStandardMarshal); if (pStm != null) { Marshal.ReleaseComObject(pStm); } } } /// /// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] int UnsafeNativeMethods.IMarshal.UnmarshalInterface(object pStm, ref Guid riid, out IntPtr ppv) { // this should never be called on this interface, but on the standard one handed back by the previous calls. Debug.Fail("IMarshal::UnmarshalInterface should not be called."); ppv = IntPtr.Zero; if (pStm != null) { Marshal.ReleaseComObject(pStm); } return NativeMethods.E_NOTIMPL; } /// /// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] int UnsafeNativeMethods.IMarshal.ReleaseMarshalData(object pStm) { // this should never be called on this interface, but on the standard one handed back by the previous calls. Debug.Fail("IMarshal::ReleaseMarshalData should not be called."); if (pStm != null) { Marshal.ReleaseComObject(pStm); } return NativeMethods.E_NOTIMPL; } /// /// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] int UnsafeNativeMethods.IMarshal.DisconnectObject(int dwReserved) { // this should never be called on this interface, but on the standard one handed back by the previous calls. Debug.Fail("IMarshal::DisconnectObject should not be called."); return NativeMethods.E_NOTIMPL; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Runtime.InteropServices { using System.Diagnostics; using System; using Microsoft.Win32; using System.Security; using System.Security.Permissions; ////// /// /// Replaces the standard CLR free-threaded marshaler with the standard OLE STA one. This prevents the calls made into /// our hosting object by OLE from coming in on threads other than the UI thread. /// /// [ComVisible(true)] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Interoperability", "CA1403:AutoLayoutTypesShouldNotBeComVisible")] public class StandardOleMarshalObject : MarshalByRefObject, UnsafeNativeMethods.IMarshal { protected StandardOleMarshalObject() { } [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] private IntPtr GetStdMarshaller(ref Guid riid, int dwDestContext, int mshlflags) { IntPtr pStdMarshal = IntPtr.Zero; IntPtr pUnk = Marshal.GetIUnknownForObject(this); if (pUnk != IntPtr.Zero) { try { if (NativeMethods.S_OK == UnsafeNativeMethods.CoGetStandardMarshal(ref riid, pUnk, dwDestContext, IntPtr.Zero, mshlflags, out pStdMarshal)) { Debug.Assert(pStdMarshal != null, "Failed to get marshaller for interface '" + riid.ToString() + "', CoGetStandardMarshal returned S_OK"); return pStdMarshal; } } finally { Marshal.Release(pUnk); } } throw new InvalidOperationException(SR.GetString(SR.StandardOleMarshalObjectGetMarshalerFailed, riid.ToString())); } ////// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] int UnsafeNativeMethods.IMarshal.GetUnmarshalClass(ref Guid riid, IntPtr pv, int dwDestContext, IntPtr pvDestContext, int mshlflags, out Guid pCid){ pCid = typeof(UnsafeNativeMethods.IStdMarshal).GUID; return NativeMethods.S_OK; } /// /// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] int UnsafeNativeMethods.IMarshal.GetMarshalSizeMax(ref Guid riid, IntPtr pv, int dwDestContext, IntPtr pvDestContext, int mshlflags, out int pSize) { // 98830 - GUID marshaling in StandardOleMarshalObject AVs on 64-bit Guid riid_copy = riid; IntPtr pStandardMarshal = GetStdMarshaller(ref riid_copy, dwDestContext, mshlflags); try { return UnsafeNativeMethods.CoGetMarshalSizeMax(out pSize, ref riid_copy, pStandardMarshal, dwDestContext, pvDestContext, mshlflags); } finally { Marshal.Release(pStandardMarshal); } } /// /// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] int UnsafeNativeMethods.IMarshal.MarshalInterface(object pStm, ref Guid riid, IntPtr pv, int dwDestContext, IntPtr pvDestContext, int mshlflags) { IntPtr pStandardMarshal = GetStdMarshaller(ref riid, dwDestContext, mshlflags); try { return UnsafeNativeMethods.CoMarshalInterface(pStm, ref riid, pStandardMarshal, dwDestContext, pvDestContext, mshlflags); } finally { Marshal.Release(pStandardMarshal); if (pStm != null) { Marshal.ReleaseComObject(pStm); } } } /// /// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] int UnsafeNativeMethods.IMarshal.UnmarshalInterface(object pStm, ref Guid riid, out IntPtr ppv) { // this should never be called on this interface, but on the standard one handed back by the previous calls. Debug.Fail("IMarshal::UnmarshalInterface should not be called."); ppv = IntPtr.Zero; if (pStm != null) { Marshal.ReleaseComObject(pStm); } return NativeMethods.E_NOTIMPL; } /// /// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] int UnsafeNativeMethods.IMarshal.ReleaseMarshalData(object pStm) { // this should never be called on this interface, but on the standard one handed back by the previous calls. Debug.Fail("IMarshal::ReleaseMarshalData should not be called."); if (pStm != null) { Marshal.ReleaseComObject(pStm); } return NativeMethods.E_NOTIMPL; } /// /// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] int UnsafeNativeMethods.IMarshal.DisconnectObject(int dwReserved) { // this should never be called on this interface, but on the standard one handed back by the previous calls. Debug.Fail("IMarshal::DisconnectObject should not be called."); return NativeMethods.E_NOTIMPL; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
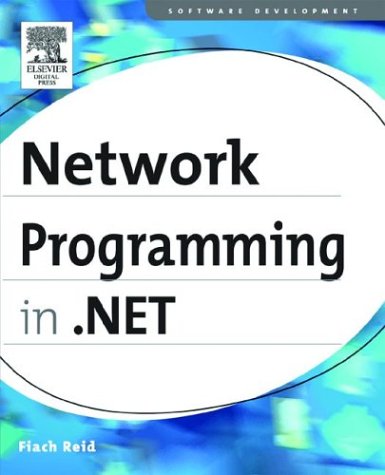
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SHA512Managed.cs
- ToolBarButton.cs
- InstanceOwner.cs
- DashStyle.cs
- WebPartConnectionsDisconnectVerb.cs
- StaticContext.cs
- DataGridViewCellValidatingEventArgs.cs
- BrowserCapabilitiesCompiler.cs
- Visual3D.cs
- LayoutEditorPart.cs
- VectorCollectionConverter.cs
- FontFamily.cs
- RequestDescription.cs
- FileSystemEventArgs.cs
- PlaceHolder.cs
- ShaperBuffers.cs
- SiteMapPath.cs
- TreeSet.cs
- DataRecord.cs
- HtmlControlPersistable.cs
- ObjectStateEntryOriginalDbUpdatableDataRecord.cs
- XmlNavigatorFilter.cs
- DatePicker.cs
- DocumentPage.cs
- CookieParameter.cs
- Configuration.cs
- GridLengthConverter.cs
- DrawingContextDrawingContextWalker.cs
- OptimalTextSource.cs
- CalendarKeyboardHelper.cs
- ImportOptions.cs
- FixedSOMPage.cs
- GPRECT.cs
- XmlParserContext.cs
- UnionExpr.cs
- SoapInteropTypes.cs
- ExpressionConverter.cs
- StateChangeEvent.cs
- CreateDataSourceDialog.cs
- PrinterUnitConvert.cs
- Funcletizer.cs
- ObjectDataSourceStatusEventArgs.cs
- JoinElimination.cs
- ItemsControl.cs
- dataprotectionpermission.cs
- DataPager.cs
- SmiContextFactory.cs
- ToolbarAUtomationPeer.cs
- HttpsHostedTransportConfiguration.cs
- BitmapEffectInputData.cs
- AccessText.cs
- DataGridViewComboBoxCell.cs
- LambdaCompiler.Expressions.cs
- ServiceInstanceProvider.cs
- IndexerNameAttribute.cs
- HealthMonitoringSectionHelper.cs
- DesignerWebPartChrome.cs
- Sql8ConformanceChecker.cs
- Vector3D.cs
- ListControl.cs
- HandlerBase.cs
- BindingListCollectionView.cs
- WindowsToolbarItemAsMenuItem.cs
- GlobalProxySelection.cs
- IPipelineRuntime.cs
- IResourceProvider.cs
- ScopedKnownTypes.cs
- DataColumnChangeEvent.cs
- LineInfo.cs
- SqlUserDefinedAggregateAttribute.cs
- KerberosRequestorSecurityToken.cs
- Setter.cs
- Funcletizer.cs
- FontFamily.cs
- SizeChangedInfo.cs
- DispatchChannelSink.cs
- IDataContractSurrogate.cs
- ObjectConverter.cs
- AppDomainProtocolHandler.cs
- EventManager.cs
- DocumentApplicationJournalEntry.cs
- Parser.cs
- CodeTypeDelegate.cs
- DependencyPropertyAttribute.cs
- SqlMethods.cs
- ProviderManager.cs
- DataObject.cs
- ObjectDisposedException.cs
- ClientSponsor.cs
- DoubleKeyFrameCollection.cs
- CellTreeNodeVisitors.cs
- TextFormatterImp.cs
- RuleSetReference.cs
- XmlQuerySequence.cs
- XmlMembersMapping.cs
- XmlComment.cs
- PointLight.cs
- WorkflowItemsPresenter.cs
- ExpandCollapsePattern.cs
- RadioButtonList.cs