Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / TrustUi / MS / Internal / documents / RMPermissions.cs / 1 / RMPermissions.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // RMPermissions. // // History: // 06/20/05 - [....] created // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.ComponentModel; using System.Drawing; using System.Text; using System.Windows.Forms; using System.Collections; using System.Windows.TrustUI; using System.Security; using System.Security.Permissions; using System.Security.RightsManagement; using MS.Internal.Documents.Application; namespace MS.Internal.Documents { ////// Class representing the Rights Management Permissions dialog. /// internal sealed partial class RMPermissionsDialog : DialogBaseForm { //------------------------------------------------------------------------- // Constructors //------------------------------------------------------------------------- #region Constructors ////// Constructor to use for non-owners /// /// The use license of the current user ////// Critical /// 1) A license object that contains critical information is passed /// in as an argument, but it is not saved except as strings to /// display in the UI. /// 2) Critical information about user objects is retrieved, but it is /// not saved except in the UI. /// 3) Calls critical function InitializeReferralInformation with the /// information passed in as a parameter. This function does not /// leak any critical information except again to show in the UI. /// [SecurityCritical] internal RMPermissionsDialog(RightsManagementLicense userLicense) { // Set up labels authenticatedAsLabel.Text = userLicense.LicensedUser.Name; expiresOnLabel.Text = GetUtcDateAsString(userLicense.ValidUntil); InitializeReferralInformation(userLicense); // Set up list of rights AddPermissions(GetRightsFromPermissions(userLicense)); } #endregion Constructors //-------------------------------------------------------------------------- // Private Methods //------------------------------------------------------------------------- #region Private Methods ////// Initialize the referral information (the name and the URI) to /// display in the UI. /// /// The license from which to retrieve the /// information to display ////// Critical /// 1) Sets UI based on object passed in as a parameter /// 2) Accesses critical properties of use license, but doesn't save /// or leak them except as strings to display in the UI or as /// critical member variables /// 3) Sets critical member _referralUri based on object passed in as /// a parameter /// [SecurityCritical] private void InitializeReferralInformation(RightsManagementLicense userLicense) { string referralName = userLicense.ReferralInfoName; Uri referralUri = userLicense.ReferralInfoUri; // The referral information displayed is: // - the referral URI. If that is not available, // - the referral name. If that is not available, // - the default text "Unknown" if (referralUri != null) { requestFromLabel.Text = referralUri.ToString(); } else if (!string.IsNullOrEmpty(referralName)) { requestFromLabel.Text = referralName; } // If the referral URI is a mailto URI, make the LinkLabel clickable if (referralUri != null && AddressUtility.IsMailtoUri(referralUri)) { // LinkLabels have one Link in the Links list by default requestFromLabel.Links[0].Description = referralName; // Set up the click handler; it uses _referralUri _referralUri = referralUri; requestFromLabel.LinkClicked += new LinkLabelLinkClickedEventHandler(requestFromLabel_LinkClicked); } else { // If there is no referral URI or it is not a mailto: link, the // label should not appear clickable. requestFromLabel.Links.Clear(); } } ////// Generates a string for each individual right granted to a user as represented /// in a RightsManagementLicense object. /// /// The license to convert ///An array of strings representing all rights granted private static string[] GetRightsFromPermissions(RightsManagementLicense license) { IListrightsStrings = new List (); if (license.HasPermission(RightsManagementPermissions.AllowOwner)) { rightsStrings.Add(SR.Get(SRID.RMPermissionsOwnerPermission)); } else { if (license.HasPermission(RightsManagementPermissions.AllowView)) { rightsStrings.Add(SR.Get(SRID.RMPermissionsViewPermission)); } if (license.HasPermission(RightsManagementPermissions.AllowPrint)) { rightsStrings.Add(SR.Get(SRID.RMPermissionsPrintPermission)); } if (license.HasPermission(RightsManagementPermissions.AllowCopy)) { rightsStrings.Add(SR.Get(SRID.RMPermissionsCopyPermission)); } if (license.HasPermission(RightsManagementPermissions.AllowSign)) { rightsStrings.Add(SR.Get(SRID.RMPermissionsSignPermission)); } if (license.HasPermission(RightsManagementPermissions.AllowAnnotate)) { rightsStrings.Add(SR.Get(SRID.RMPermissionsAnnotatePermission)); } } string[] stringArray = new string[rightsStrings.Count]; rightsStrings.CopyTo(stringArray, 0); return stringArray; } /// /// Returns a string that is the local representation (in the local /// time zone) of a UTC date /// /// The date to represent ///A string representing the date private static string GetUtcDateAsString(DateTime? date) { if (!date.HasValue || date.Value.Equals(DateTime.MaxValue)) { return SR.Get(SRID.RMPermissionsNoExpiration); } else { DateTime localDate = date.Value.ToLocalTime(); return localDate.ToShortDateString(); } } ////// The handler for when the "request permissions from" link is /// clicked. This navigates to the saved referral URI. /// /// Event sender (not used) /// Event arguments (not used) ///We save and use the cached referral URI for security /// reasons. This function is an event handler, so it cannot be marked /// critical and can be called by anything, so it is probably is not /// safe to read information from the argument. ////// Critical /// 1) Accesses critical data _referralUri /// 2) Calls critical function NavigationHelper.Navigate /// NotSafe /// 1) Could present a risk of a DoS attack because the function /// cannot confirm that this happened on user interaction /// [SecurityCritical] private void requestFromLabel_LinkClicked(object sender, LinkLabelLinkClickedEventArgs e) { // Navigate to the cached referral URI NavigationHelper.Navigate(new SecurityCriticalData(_referralUri)); } /// /// Adds the UI permission strings to the UI. /// /// private void AddPermissions(string[] uiStrings) { foreach (string permission in uiStrings) { if (!string.IsNullOrEmpty(permission)) { // Create a new label and add it to the permissionsFlowPanel Label permissionLabel = new Label(); permissionLabel.AutoSize = true; permissionLabel.Text = permission; permissionLabel.Margin = new Padding(13, 0, 3, 0); permissionsFlowPanel.Controls.Add(permissionLabel); } } } #endregion Private Methods //------------------------------------------------------ // Private Fields //------------------------------------------------------ #region Private Fields ////// The URI to contact for permissions. /// ////// Critical - Personally identifiable information /// [SecurityCritical] private Uri _referralUri; #endregion Private Fields //----------------------------------------------------- // Protected Methods //------------------------------------------------------ #region Protected Methods ////// ApplyResources override. Called to apply dialog resources. /// protected override void ApplyResources() { base.ApplyResources(); Text = SR.Get(SRID.RMPermissionsTitle); authenticatedAsTextLabel.Text = SR.Get(SRID.RMPermissionsAuthenticatedAs); permissionsHeldLabel.Text = SR.Get(SRID.RMPermissionsHavePermissions); requestFromTextLabel.Text = SR.Get(SRID.RMPermissionsRequestFrom); requestFromLabel.Text = SR.Get(SRID.RMPermissionsUnknownOwner); expiresOnTextLabel.Text = SR.Get(SRID.RMPermissionsExpiresOn); closeButton.Text = SR.Get(SRID.RMPermissionsCloseButton); } #endregion Protected Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
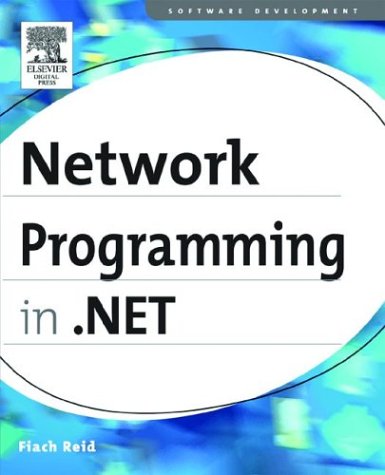
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- QuadraticBezierSegment.cs
- CryptoApi.cs
- ConfigsHelper.cs
- MappingMetadataHelper.cs
- SQLSingle.cs
- ZipArchive.cs
- DataSourceNameHandler.cs
- ResolveCriteriaCD1.cs
- StrokeNodeOperations.cs
- ExpressionPrefixAttribute.cs
- DiscardableAttribute.cs
- SecurityTokenException.cs
- CreateSequenceResponse.cs
- DataGridViewHitTestInfo.cs
- ManagementObject.cs
- FixUpCollection.cs
- ItemAutomationPeer.cs
- PngBitmapDecoder.cs
- CheckedPointers.cs
- StrokeSerializer.cs
- FontInfo.cs
- ConsumerConnectionPointCollection.cs
- BrushConverter.cs
- ValidationHelpers.cs
- TableSectionStyle.cs
- DoubleLink.cs
- VectorConverter.cs
- BinaryConverter.cs
- PerformanceCounterLib.cs
- ListViewContainer.cs
- PeerMaintainer.cs
- SchemaEntity.cs
- sortedlist.cs
- ThousandthOfEmRealDoubles.cs
- IConvertible.cs
- LocalizeDesigner.cs
- SelectionEditingBehavior.cs
- ExtentJoinTreeNode.cs
- XmlSchemaGroupRef.cs
- FilterUserControlBase.cs
- MD5Cng.cs
- ContentPosition.cs
- SetterBaseCollection.cs
- ProjectionCamera.cs
- BufferBuilder.cs
- TimelineGroup.cs
- UdpMessageProperty.cs
- GridViewHeaderRowPresenterAutomationPeer.cs
- XmlQueryStaticData.cs
- SpinLock.cs
- XmlNavigatorFilter.cs
- CodeDOMProvider.cs
- DataServiceHostFactory.cs
- JumpList.cs
- DescriptionAttribute.cs
- Point3DConverter.cs
- HandlerElement.cs
- SystemResourceHost.cs
- SponsorHelper.cs
- MatchAttribute.cs
- AttributeCollection.cs
- WindowsFormsHostPropertyMap.cs
- PropertyRef.cs
- IssuerInformation.cs
- HandlerMappingMemo.cs
- TimelineClockCollection.cs
- DetailsViewPageEventArgs.cs
- AnnotationService.cs
- ContractAdapter.cs
- AuthenticationManager.cs
- VersionedStream.cs
- XmlTextEncoder.cs
- SrgsDocument.cs
- httpapplicationstate.cs
- DateTimeStorage.cs
- MetadataItem_Static.cs
- Regex.cs
- ProcessHostServerConfig.cs
- Rijndael.cs
- ListMarkerSourceInfo.cs
- OperatorExpressions.cs
- RowToFieldTransformer.cs
- XamlSerializerUtil.cs
- MenuItemCollection.cs
- TextTrailingCharacterEllipsis.cs
- EUCJPEncoding.cs
- WorkerRequest.cs
- ObfuscateAssemblyAttribute.cs
- ComponentEditorForm.cs
- ProfileBuildProvider.cs
- WindowsEditBoxRange.cs
- KeyInterop.cs
- HttpWebRequest.cs
- HttpHandlerAction.cs
- columnmapkeybuilder.cs
- Membership.cs
- MessageBox.cs
- CollectionView.cs
- EntityFrameworkVersions.cs
- Delegate.cs