Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / CreateSequenceResponse.cs / 1 / CreateSequenceResponse.cs
//---------------------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------------------- namespace System.ServiceModel.Channels { using System.Xml; sealed class CreateSequenceResponse : BodyWriter { EndpointAddress acceptAcksTo; AddressingVersion addressingVersion; Nullableexpires; UniqueId identifier; bool ordered; ReliableMessagingVersion reliableMessagingVersion; CreateSequenceResponse() : base(true) { } public CreateSequenceResponse(AddressingVersion addressingVersion, ReliableMessagingVersion reliableMessagingVersion) : base(true) { this.addressingVersion = addressingVersion; this.reliableMessagingVersion = reliableMessagingVersion; } public EndpointAddress AcceptAcksTo { get { return this.acceptAcksTo; } set { this.acceptAcksTo = value; } } public Nullable Expires { get { return this.expires; } set { this.expires = value; } } public UniqueId Identifier { get { return this.identifier; } set { this.identifier = value; } } public bool Ordered { get { return this.ordered; } set { this.ordered = value; } } public static CreateSequenceResponseInfo Create(AddressingVersion addressingVersion, ReliableMessagingVersion reliableMessagingVersion, XmlDictionaryReader reader) { if (reader == null) { DiagnosticUtility.DebugAssert("Argument reader cannot be null."); } CreateSequenceResponseInfo createSequenceResponse = new CreateSequenceResponseInfo(); WsrmFeb2005Dictionary wsrmFeb2005Dictionary = XD.WsrmFeb2005Dictionary; XmlDictionaryString wsrmNs = WsrmIndex.GetNamespace(reliableMessagingVersion); reader.ReadStartElement(wsrmFeb2005Dictionary.CreateSequenceResponse, wsrmNs); reader.ReadStartElement(wsrmFeb2005Dictionary.Identifier, wsrmNs); createSequenceResponse.Identifier = reader.ReadContentAsUniqueId(); reader.ReadEndElement(); if (reader.IsStartElement(wsrmFeb2005Dictionary.Expires, wsrmNs)) { reader.ReadElementContentAsTimeSpan(); } if (reliableMessagingVersion == ReliableMessagingVersion.WSReliableMessaging11) { if (reader.IsStartElement(DXD.Wsrm11Dictionary.IncompleteSequenceBehavior, wsrmNs)) { string incompleteSequenceBehavior = reader.ReadElementContentAsString(); if ((incompleteSequenceBehavior != Wsrm11Strings.DiscardEntireSequence) && (incompleteSequenceBehavior != Wsrm11Strings.DiscardFollowingFirstGap) && (incompleteSequenceBehavior != Wsrm11Strings.NoDiscard)) { string reason = SR.GetString(SR.CSResponseWithInvalidIncompleteSequenceBehavior); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new XmlException(reason)); } // Otherwise ignore the value. } } if (reader.IsStartElement(wsrmFeb2005Dictionary.Accept, wsrmNs)) { reader.ReadStartElement(); createSequenceResponse.AcceptAcksTo = EndpointAddress.ReadFrom(addressingVersion, reader, wsrmFeb2005Dictionary.AcksTo, wsrmNs); while (reader.IsStartElement()) { reader.Skip(); } reader.ReadEndElement(); } while (reader.IsStartElement()) { reader.Skip(); } reader.ReadEndElement(); return createSequenceResponse; } protected override void OnWriteBodyContents(XmlDictionaryWriter writer) { WsrmFeb2005Dictionary wsrmFeb2005Dictionary = XD.WsrmFeb2005Dictionary; XmlDictionaryString wsrmNs = WsrmIndex.GetNamespace(this.reliableMessagingVersion); writer.WriteStartElement(wsrmFeb2005Dictionary.CreateSequenceResponse, wsrmNs); writer.WriteStartElement(wsrmFeb2005Dictionary.Identifier, wsrmNs); writer.WriteValue(this.identifier); writer.WriteEndElement(); if (this.expires.HasValue) { writer.WriteStartElement(wsrmFeb2005Dictionary.Expires, wsrmNs); writer.WriteValue(this.expires.Value); writer.WriteEndElement(); } if (this.reliableMessagingVersion == ReliableMessagingVersion.WSReliableMessaging11) { Wsrm11Dictionary wsrm11Dictionary = DXD.Wsrm11Dictionary; writer.WriteStartElement(wsrm11Dictionary.IncompleteSequenceBehavior, wsrmNs); writer.WriteValue( this.ordered ? wsrm11Dictionary.DiscardFollowingFirstGap : wsrm11Dictionary.NoDiscard); writer.WriteEndElement(); } if (this.acceptAcksTo != null) { writer.WriteStartElement(wsrmFeb2005Dictionary.Accept, wsrmNs); this.acceptAcksTo.WriteTo(this.addressingVersion, writer, wsrmFeb2005Dictionary.AcksTo, wsrmNs); writer.WriteEndElement(); } writer.WriteEndElement(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
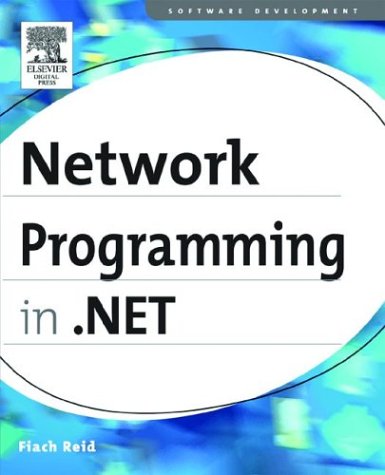
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- _ConnectionGroup.cs
- DataSourceBooleanViewSchemaConverter.cs
- UIElementIsland.cs
- TextStore.cs
- ThaiBuddhistCalendar.cs
- LabelEditEvent.cs
- Misc.cs
- ClientSideProviderDescription.cs
- Type.cs
- MessageAction.cs
- FormatStringEditor.cs
- RegionIterator.cs
- DataGridItemCollection.cs
- Helper.cs
- baseaxisquery.cs
- Win32MouseDevice.cs
- _TransmitFileOverlappedAsyncResult.cs
- SoapCodeExporter.cs
- HttpBrowserCapabilitiesWrapper.cs
- XamlStream.cs
- DecimalAnimationBase.cs
- XmlUtil.cs
- FormCollection.cs
- DataRow.cs
- LinqDataSourceContextEventArgs.cs
- MeasureItemEvent.cs
- XPathDescendantIterator.cs
- GlobalId.cs
- figurelengthconverter.cs
- WmpBitmapEncoder.cs
- X509Utils.cs
- CodeConditionStatement.cs
- Matrix3DConverter.cs
- OleDbEnumerator.cs
- ClientBuildManagerCallback.cs
- SEHException.cs
- EmbeddedMailObject.cs
- HttpHandlersSection.cs
- InputScope.cs
- PersonalizableAttribute.cs
- HelpInfo.cs
- CommandID.cs
- XmlCollation.cs
- XmlIgnoreAttribute.cs
- ElementHostPropertyMap.cs
- DataGridViewSortCompareEventArgs.cs
- RepeaterDesigner.cs
- SamlAdvice.cs
- TabOrder.cs
- PrintingPermissionAttribute.cs
- MimeReturn.cs
- FixedSOMTableRow.cs
- XsdBuilder.cs
- WebPartDisplayModeEventArgs.cs
- ExpressionBuilder.cs
- HelpPage.cs
- InputLanguageSource.cs
- CommandManager.cs
- columnmapfactory.cs
- DataStorage.cs
- PropertyFilterAttribute.cs
- XmlElementAttributes.cs
- ECDsaCng.cs
- AttributeCallbackBuilder.cs
- DaylightTime.cs
- altserialization.cs
- SocketPermission.cs
- DiffuseMaterial.cs
- Queue.cs
- RegexGroup.cs
- HtmlDocument.cs
- ErrorRuntimeConfig.cs
- MailDefinition.cs
- Guid.cs
- CodeTypeDeclarationCollection.cs
- InputMethod.cs
- GroupStyle.cs
- ConsumerConnectionPoint.cs
- TypefaceCollection.cs
- DataTransferEventArgs.cs
- PriorityQueue.cs
- BindingWorker.cs
- DictionaryItemsCollection.cs
- _LocalDataStore.cs
- FileSystemEnumerable.cs
- DataQuery.cs
- ExceptionHelpers.cs
- OutArgument.cs
- DataGridRelationshipRow.cs
- CreateParams.cs
- HttpRequestCacheValidator.cs
- MdImport.cs
- AutomationTextAttribute.cs
- InstanceDataCollection.cs
- AuthenticateEventArgs.cs
- Query.cs
- autovalidator.cs
- CodeNamespaceCollection.cs
- TransactionTable.cs
- PersianCalendar.cs