Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / GlobalId.cs / 2 / GlobalId.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Security; using System.Security.Cryptography; using System.Text; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary: // "Guid" that is derived from a string. // [StructLayout( LayoutKind.Explicit, Size=StructSize )] unsafe internal struct GlobalId { public const int StructSize = 16; // // Summary: // Represents an empty GlobalId value // public static readonly GlobalId Empty = Guid.Empty; // // {7E55DB11-34DD-4696-A87B-0FFEC44D9BA8} // static readonly byte[] HASHPREFIX = { 0x7e, 0x55, 0xdb, 0x11, 0x34, 0xdd, 0x46, 0x96, 0xa8, 0x7b, 0x0f, 0xfe, 0xc4, 0x4d, 0x9b, 0xa8 }; // // Summary: // Creates a GlobalId from raw bytes // // Remarks: // Guid.ToByteArray() generates the same format. // // Parameters: // guidBytes: bytes to parse to create a guid // public GlobalId( byte[] guidBytes ) : this( new Guid( guidBytes ) ) { } // // Summary: // Creates a new GlobalId from the specified Guid // // Remarks: // Unsafe memcopy of src over current this. // // Parameters: // guid: Guid to copy. // public GlobalId( Guid guid ) { // // copy the guid over ourselves // // We must fix this, as the compiler doesn't // know if this is boxed or not, so we do it // just in case. // fixed( GlobalId* pValue = &this ) { *pValue = guid; } } // // Summary: // Get the raw bytes of the guid. // // Returns: // Raw bytes of the GlobalId // public byte[] ToByteArray() { return ((Guid)this).ToByteArray(); } // // Summary: // retuns the hashcode for the GlobalId // public override int GetHashCode() { return ((Guid)this).GetHashCode(); } // // Summary: // Determins if an object is the same as this object // // Parameters: // obj: object to test // // Returns: // boolean indicating the match. // public override bool Equals( object obj ) { if( obj is GlobalId || obj is Guid ) { return ((GlobalId)obj) == this; } return false; } // // Summary: // Returns the string form of the GlobalId // public override string ToString() { // // return delimited guid xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx // return ((Guid)this).ToString( "D" ); } // // Summary: // Not Equal override // public static bool operator != ( GlobalId a, GlobalId b ) { return !( a == b); } // // Summary: // Equals Override... Just do basic memcomp // public static bool operator == ( GlobalId a, GlobalId b ) { byte* pA = (byte*)&a; byte* pB = (byte*)&b; for( int i = 0; i < sizeof( GlobalId ); i++ ) { if( pA[ i ] != pB[ i ] ) { return false; } } return true; } // // Summary: // Implicit conversion from Guid to GlobalId. // // Remarks: // Becuase all structs are imutable stack items, this basically // performs a "memcpy" of this structure to the target guid // // Returns: // Writes bytes to return target Guid. // // Parameters: // id: The GlobalId to copy to the target return guid. // public static implicit operator Guid ( GlobalId id ) { return *((Guid*)&id); } // // Summary: // Implicit conversion from GlobalId to a Guid. // // Remarks: // Becuase all structs are imutable stack items, this basically // performs a "memcpy" of the Guid to the target GlobalId // // Returns: // Writes bytes to return target GlobalId. // // Parameters: // guid: The Guid to copy to the target return GlobalId. // public static implicit operator GlobalId ( Guid guid ) { return *((GlobalId*)&guid); } // // Summary: // Derives a GlobalId from a string value. // // Remarks: // Passing the same string in multiple times should always // create the same global ID. // // Returns: // A new GlobalId structure. // // Parameters: // value: The string value to derive the key from. // public static GlobalId DeriveFrom( string value ) { if( String.IsNullOrEmpty( value ) ) { throw IDT.ThrowHelperArgumentNull( "value" ); } //trim the whitespace //an canonicalize the value value = value.Trim(); value = value.ToLower( System.Globalization.CultureInfo.InvariantCulture ); using( SHA256Managed sha256 = new SHA256Managed() ) { int byteCount = Encoding.Unicode.GetByteCount( value ) + HASHPREFIX.Length; byte[] bytesToHash = new byte[ byteCount ]; Array.Copy( HASHPREFIX, 0, bytesToHash, 0, HASHPREFIX.Length ); Encoding.Unicode.GetBytes( value, 0, value.Length, bytesToHash, HASHPREFIX.Length); byte[] hashbytes = sha256.ComputeHash( bytesToHash ); // // The following 2 functions are designed to create a version 3 guid using a string. // This is done using an MD5 hash algorithm according the Leach document. // Some information from the leach document is placed in comment below. // // time_low // time_mid // time_hi, version // clock_seq_low, clock_seq_hi, reserved // node // ff5c0e9a-7cd2-4b8c-9c6e-cfa03ebc7238 // 0100, 1011, 1000, 1010 Version 4 guid // 1001, 1010, 0110, 1110 Reserved == 10 // // Field Data Type Octet Note // # // // time_low unsigned 32 0-3 The low field of the // bit integer timestamp. // // time_mid unsigned 16 4-5 The middle field of the // bit integer timestamp. // // time_hi_and_version unsigned 16 6-7 The high field of the // bit integer timestamp multiplexed // with the version number. // // clock_seq_hi_and_rese unsigned 8 8 The high field of the // rved bit integer clock sequence // multiplexed with the // variant. // // clock_seq_low unsigned 8 9 The low field of the // bit integer clock sequence. // // node unsigned 48 10-15 The spatially unique // bit integer node identifier. // // The version number is in the most significant 4 bits of the time // stamp (time_hi_and_version). // // The following table lists currently defined versions of the UUID. // // Msb0 Msb1 Msb2 Msb3 Version Description // // 0 0 0 1 1 The time-based version // specified in this // document. // // 0 0 1 0 2 Reserved for DCE // Security version, with // embedded POSIX UIDs. // // 0 0 1 1 3 The name-based version // specified in this // document // // 0 1 0 0 4 The randomly or pseudo- // randomly generated // version specified in // this document // // The following table lists the contents of the variant field. // // Msb0 Msb1 Msb2 Description // // 0 - - Reserved, NCS backward compatibility. // // 1 0 - The variant specified in this document. // // 1 1 0 Reserved, Microsoft Corporation backward // compatibility // // 1 1 1 Reserved for future definition. // byte[] newGuid = new byte[ 16 ]; newGuid[ 0 ] = hashbytes[ 3 ]; newGuid[ 1 ] = hashbytes[ 2 ]; newGuid[ 2 ] = hashbytes[ 1 ]; newGuid[ 3 ] = hashbytes[ 0 ]; newGuid[ 4 ] = hashbytes[ 5 ]; newGuid[ 5 ] = hashbytes[ 4 ]; newGuid[ 6 ] = hashbytes[ 7 ]; newGuid[ 7 ] = (byte)(( hashbytes[ 6 ] & 0x0f ) | 0x30); newGuid[ 8 ] = (byte)(( hashbytes[ 8 ] & 0x3F ) | 0x80); newGuid[ 9 ] = hashbytes[ 9 ]; newGuid[ 10 ] = hashbytes[ 10 ]; newGuid[ 11 ] = hashbytes[ 11 ]; newGuid[ 12 ] = hashbytes[ 12 ]; newGuid[ 13 ] = hashbytes[ 13 ]; newGuid[ 14 ] = hashbytes[ 14 ]; newGuid[ 15 ] = hashbytes[ 15 ]; return new GlobalId( newGuid ); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
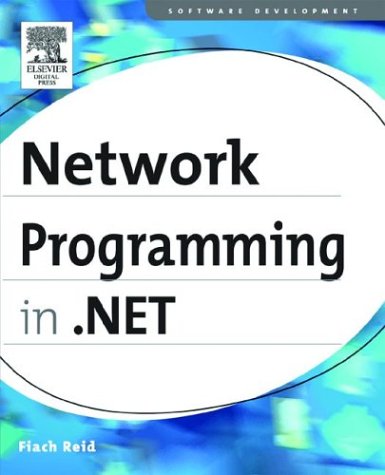
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StringStorage.cs
- BoundingRectTracker.cs
- DateTimeFormatInfoScanner.cs
- InvalidEnumArgumentException.cs
- AdornerDecorator.cs
- XmlMembersMapping.cs
- Button.cs
- CompoundFileIOPermission.cs
- XsdBuilder.cs
- Vector3DConverter.cs
- QueryAccessibilityHelpEvent.cs
- VerificationAttribute.cs
- Icon.cs
- Token.cs
- BufferedResponseStream.cs
- ImageField.cs
- SspiNegotiationTokenProvider.cs
- TextTreeTextBlock.cs
- ALinqExpressionVisitor.cs
- WorkflowExecutor.cs
- SqlBuffer.cs
- DelegatedStream.cs
- DbConnectionHelper.cs
- PermissionSetTriple.cs
- Point.cs
- HtmlTableRow.cs
- CompleteWizardStep.cs
- TypefaceMetricsCache.cs
- ConnectionConsumerAttribute.cs
- _Win32.cs
- SQLByteStorage.cs
- Button.cs
- XLinq.cs
- HtmlTableRow.cs
- Point3DCollectionValueSerializer.cs
- TextAnchor.cs
- DesignerObject.cs
- HierarchicalDataSourceControl.cs
- HttpBrowserCapabilitiesBase.cs
- AttachInfo.cs
- SchemaElement.cs
- SmiMetaDataProperty.cs
- OleDbCommand.cs
- DesignerExtenders.cs
- OptionalColumn.cs
- _NativeSSPI.cs
- PeerEndPoint.cs
- StringValidator.cs
- MultiAsyncResult.cs
- CacheChildrenQuery.cs
- HttpWebRequest.cs
- TextDpi.cs
- EndpointAddressProcessor.cs
- ListViewTableCell.cs
- ScriptResourceInfo.cs
- TextParaClient.cs
- RuntimeIdentifierPropertyAttribute.cs
- BaseDataBoundControl.cs
- StorageMappingItemCollection.cs
- Button.cs
- AxisAngleRotation3D.cs
- Converter.cs
- AttributeSetAction.cs
- DataGridState.cs
- FontStretches.cs
- SafeProcessHandle.cs
- NonSerializedAttribute.cs
- UInt16.cs
- ScrollItemPattern.cs
- ListViewEditEventArgs.cs
- TracePayload.cs
- DesignerDataConnection.cs
- ActivitySurrogateSelector.cs
- EntityClassGenerator.cs
- XmlReaderSettings.cs
- xsdvalidator.cs
- BookmarkManager.cs
- InteropAutomationProvider.cs
- DateTimeOffset.cs
- TextRunProperties.cs
- PeerApplication.cs
- Rect3D.cs
- StringUtil.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- ParentQuery.cs
- EtwProvider.cs
- DataGridViewSelectedCellCollection.cs
- TypographyProperties.cs
- NullReferenceException.cs
- BuildProvider.cs
- FileIOPermission.cs
- ToolStripCollectionEditor.cs
- TextBox.cs
- Int16KeyFrameCollection.cs
- EntityPropertyMappingAttribute.cs
- DesignerDataTable.cs
- ViewStateChangedEventArgs.cs
- SqlCacheDependencyDatabaseCollection.cs
- CustomLineCap.cs
- TCPListener.cs