Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / FactoryMaker.cs / 1 / FactoryMaker.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2003 // // File: FactoryMaker.cs // //----------------------------------------------------------------------------- using System; using System.Security; using System.Security.Permissions; using System.Diagnostics; using MS.Internal; using MS.Win32; using System.Windows.Media.Composition; using Microsoft.Internal; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using UnsafeNativeMethods=MS.Win32.PresentationCore.UnsafeNativeMethods; namespace System.Windows.Media { ////// This is a internal class which is used by non context affinity objects /// to get access to a MIL factory object. /// internal class FactoryMaker: IDisposable { private bool _disposed = false; ////// Critical - Create unmanaged critical resource /// [SecurityCritical] internal FactoryMaker() { lock (s_factoryMakerLock) { // If we haven't have a factory, create one if (s_pFactory == IntPtr.Zero) { // Create the Core MIL factory. // Note: the call below might throw exception. The caller // should catch it. We won't add ref counter here if this // happens. HRESULT.Check(UnsafeNativeMethods.MILFactory2.CreateFactory(out s_pFactory, MS.Internal.Composition.Version.MilSdkVersion)); } s_cInstance++; _fValidObject = true; } } ~FactoryMaker() { Dispose(false); } ////// Dispose of any resources /// public void Dispose() { Dispose(true); } ////// Critical - performs an elevation to call MILUnknown.ReleaseInterface. /// TreatAsSafe - this function elevates to call release ( on an object we own). /// net effect is a shutdown of the FactoryMaker. Considered safe. /// /// Note that for now there is no SUC on ReleaseInterface. We may want to re-consider this. /// [SecurityCritical, SecurityTreatAsSafe] protected virtual void Dispose(bool fDisposing) { if (!_disposed) { if (_fValidObject == true) { lock (s_factoryMakerLock) { s_cInstance--; // Make sure we don't dispose twice _fValidObject = false; // If there is no FactoryMaker object out there, release // factory object if (s_cInstance == 0) { new SecurityPermission(SecurityPermissionFlag.UnmanagedCode).Assert(); // BlessedAssert: try { UnsafeNativeMethods.MILUnknown.ReleaseInterface(ref s_pFactory); if (s_pImagingFactory != IntPtr.Zero) { UnsafeNativeMethods.MILUnknown.ReleaseInterface(ref s_pImagingFactory); } } finally { CodeAccessPermission.RevertAssert(); s_pFactory = IntPtr.Zero; s_pImagingFactory = IntPtr.Zero; } } } } // Set the sentinel. _disposed = true; // Suppress finalization of this disposed instance. if (fDisposing) { GC.SuppressFinalize(this); } } } ////// Critical - returns critical resource created under an assert /// internal IntPtr FactoryPtr { [SecurityCritical] get { Debug.Assert(s_pFactory != IntPtr.Zero); return s_pFactory; } } ////// Critical - calls unmanaged code, returns unmanaged pointer /// internal IntPtr ImagingFactoryPtr { [SecurityCritical] get { if (s_pImagingFactory == IntPtr.Zero) { lock (s_factoryMakerLock) { HRESULT.Check(UnsafeNativeMethods.WICCodec.CreateImagingFactory(UnsafeNativeMethods.WICCodec.WINCODEC_SDK_VERSION, out s_pImagingFactory)); } } Debug.Assert(s_pImagingFactory != IntPtr.Zero); return s_pImagingFactory; } } ////// Critical - this is a pointer to an unmanaged object that methods are called directly on /// [SecurityCritical] private static IntPtr s_pFactory; ////// Critical - this is a pointer to an unmanaged object that methods are called directly on /// [SecurityCritical] private static IntPtr s_pImagingFactory; ////// Keeps track of how many instance of current object have been passed out /// private static int s_cInstance = 0; ////// "FactoryMaker" is free threaded. This lock is used to synchronize /// access to the FactoryMaker. /// private static object s_factoryMakerLock = new object(); private bool _fValidObject; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2003 // // File: FactoryMaker.cs // //----------------------------------------------------------------------------- using System; using System.Security; using System.Security.Permissions; using System.Diagnostics; using MS.Internal; using MS.Win32; using System.Windows.Media.Composition; using Microsoft.Internal; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using UnsafeNativeMethods=MS.Win32.PresentationCore.UnsafeNativeMethods; namespace System.Windows.Media { ////// This is a internal class which is used by non context affinity objects /// to get access to a MIL factory object. /// internal class FactoryMaker: IDisposable { private bool _disposed = false; ////// Critical - Create unmanaged critical resource /// [SecurityCritical] internal FactoryMaker() { lock (s_factoryMakerLock) { // If we haven't have a factory, create one if (s_pFactory == IntPtr.Zero) { // Create the Core MIL factory. // Note: the call below might throw exception. The caller // should catch it. We won't add ref counter here if this // happens. HRESULT.Check(UnsafeNativeMethods.MILFactory2.CreateFactory(out s_pFactory, MS.Internal.Composition.Version.MilSdkVersion)); } s_cInstance++; _fValidObject = true; } } ~FactoryMaker() { Dispose(false); } ////// Dispose of any resources /// public void Dispose() { Dispose(true); } ////// Critical - performs an elevation to call MILUnknown.ReleaseInterface. /// TreatAsSafe - this function elevates to call release ( on an object we own). /// net effect is a shutdown of the FactoryMaker. Considered safe. /// /// Note that for now there is no SUC on ReleaseInterface. We may want to re-consider this. /// [SecurityCritical, SecurityTreatAsSafe] protected virtual void Dispose(bool fDisposing) { if (!_disposed) { if (_fValidObject == true) { lock (s_factoryMakerLock) { s_cInstance--; // Make sure we don't dispose twice _fValidObject = false; // If there is no FactoryMaker object out there, release // factory object if (s_cInstance == 0) { new SecurityPermission(SecurityPermissionFlag.UnmanagedCode).Assert(); // BlessedAssert: try { UnsafeNativeMethods.MILUnknown.ReleaseInterface(ref s_pFactory); if (s_pImagingFactory != IntPtr.Zero) { UnsafeNativeMethods.MILUnknown.ReleaseInterface(ref s_pImagingFactory); } } finally { CodeAccessPermission.RevertAssert(); s_pFactory = IntPtr.Zero; s_pImagingFactory = IntPtr.Zero; } } } } // Set the sentinel. _disposed = true; // Suppress finalization of this disposed instance. if (fDisposing) { GC.SuppressFinalize(this); } } } ////// Critical - returns critical resource created under an assert /// internal IntPtr FactoryPtr { [SecurityCritical] get { Debug.Assert(s_pFactory != IntPtr.Zero); return s_pFactory; } } ////// Critical - calls unmanaged code, returns unmanaged pointer /// internal IntPtr ImagingFactoryPtr { [SecurityCritical] get { if (s_pImagingFactory == IntPtr.Zero) { lock (s_factoryMakerLock) { HRESULT.Check(UnsafeNativeMethods.WICCodec.CreateImagingFactory(UnsafeNativeMethods.WICCodec.WINCODEC_SDK_VERSION, out s_pImagingFactory)); } } Debug.Assert(s_pImagingFactory != IntPtr.Zero); return s_pImagingFactory; } } ////// Critical - this is a pointer to an unmanaged object that methods are called directly on /// [SecurityCritical] private static IntPtr s_pFactory; ////// Critical - this is a pointer to an unmanaged object that methods are called directly on /// [SecurityCritical] private static IntPtr s_pImagingFactory; ////// Keeps track of how many instance of current object have been passed out /// private static int s_cInstance = 0; ////// "FactoryMaker" is free threaded. This lock is used to synchronize /// access to the FactoryMaker. /// private static object s_factoryMakerLock = new object(); private bool _fValidObject; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
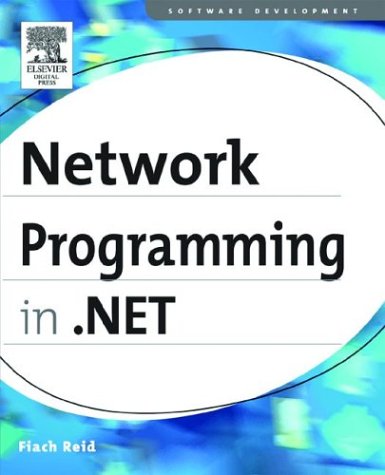
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UrlAuthFailedErrorFormatter.cs
- WorkflowInstance.cs
- EarlyBoundInfo.cs
- ServerIdentity.cs
- WebPartConnectionCollection.cs
- RangeValueProviderWrapper.cs
- RawStylusInputReport.cs
- GrammarBuilderPhrase.cs
- _NTAuthentication.cs
- ConfigurationSchemaErrors.cs
- ConnectionInterfaceCollection.cs
- OAVariantLib.cs
- SelectionEditor.cs
- PenThreadWorker.cs
- ConfigXmlElement.cs
- HtmlHistory.cs
- SettingsSavedEventArgs.cs
- DataGridViewTopRowAccessibleObject.cs
- DesignerAdRotatorAdapter.cs
- HashHelper.cs
- DataRowView.cs
- LogEntryHeaderv1Deserializer.cs
- HttpVersion.cs
- ListViewSortEventArgs.cs
- SecureConversationVersion.cs
- DynamicValidator.cs
- CustomErrorCollection.cs
- WebEvents.cs
- KerberosTicketHashIdentifierClause.cs
- GridViewItemAutomationPeer.cs
- SHA256Cng.cs
- FlowLayoutSettings.cs
- SqlExpressionNullability.cs
- RemoteAsymmetricSignatureFormatter.cs
- SoapFaultCodes.cs
- CanonicalizationDriver.cs
- PropertyMetadata.cs
- CapabilitiesSection.cs
- ConsoleTraceListener.cs
- EntitySetBaseCollection.cs
- DefinitionUpdate.cs
- LockRecoveryTask.cs
- XmlSchemaObjectCollection.cs
- WebException.cs
- DataServiceException.cs
- DetailsViewPagerRow.cs
- ActiveXHost.cs
- SamlAction.cs
- ObjectKeyFrameCollection.cs
- MimeAnyImporter.cs
- MobileTextWriter.cs
- RotationValidation.cs
- BlockUIContainer.cs
- PropertyStore.cs
- ParseChildrenAsPropertiesAttribute.cs
- RSAProtectedConfigurationProvider.cs
- PageEventArgs.cs
- WindowsImpersonationContext.cs
- PrePostDescendentsWalker.cs
- ServiceSecurityAuditElement.cs
- _LocalDataStoreMgr.cs
- TraceRecord.cs
- Accessible.cs
- SimpleParser.cs
- SegmentTree.cs
- TempFiles.cs
- XmlBinaryWriterSession.cs
- PropVariant.cs
- PathSegment.cs
- GlobalizationAssembly.cs
- ButtonBase.cs
- ConfigXmlElement.cs
- ServiceModelConfigurationElementCollection.cs
- CultureSpecificStringDictionary.cs
- ControlParameter.cs
- RuntimeHelpers.cs
- ServiceHostingEnvironment.cs
- NameScope.cs
- _RequestCacheProtocol.cs
- Header.cs
- RSAPKCS1SignatureFormatter.cs
- GPPOINT.cs
- RealProxy.cs
- Run.cs
- FullTextState.cs
- OleDbTransaction.cs
- XmlSortKeyAccumulator.cs
- HiddenFieldPageStatePersister.cs
- SplineKeyFrames.cs
- CodeGenHelper.cs
- Rules.cs
- XmlWellformedWriter.cs
- SimpleApplicationHost.cs
- WindowsButton.cs
- ResourcePropertyMemberCodeDomSerializer.cs
- BindingCollection.cs
- WebPartManager.cs
- PrintEvent.cs
- PriorityQueue.cs
- SynchronizedPool.cs