Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / VerifyHashRequest.cs / 1 / VerifyHashRequest.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Collections; using System.Diagnostics; using System.Threading; //ManualResetEvent using System.ComponentModel; //Win32Exception using System.IO; //Stream using System.Text; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; using System.Security.Principal; // // Summary: // Wraps a request to verify a given signature against an existing hash. // class VerifyHashRequest : ClientRequest { // // The cryptosession id we are attaching to. // int m_cryptoSession; // // The hash we are trying to verify against. // byte[] m_hash; // // The hash algorithm in use. // string m_hashAlgorithmOid; // // The signature we are verifying // byte[] m_signature; // // Has the signature been successfully validated.. // private bool m_verified; // // Sumamry: // Construct a VerifyHashRequest object // // Arguments: // callingProcess - The process in which the caller originated. // callingIdentity - The WindowsIdentity of the caller // rpcHandle - The handle of the native RPC request // inArgs - The stream to read input data from // outArgs - The stream to write output data to // public VerifyHashRequest( Process callingProcess, WindowsIdentity callingIdentity, IntPtr rpcHandle, Stream inArgs, Stream outArgs ) : base( callingProcess, callingIdentity, rpcHandle, inArgs, outArgs ) { IDT.TraceDebug( "Intiating a verifyHash request" ); m_cryptoSession = 0; m_hash = null; m_hashAlgorithmOid = null; m_signature = null; m_verified = false; } protected override void OnMarshalInArgs() { IDT.DebugAssert( null != InArgs, "null inargs" ); BinaryReader reader = new InfoCardBinaryReader( InArgs, Encoding.Unicode ); // // Reader should have data in the order: // crytpsession ( int32 ) // hash length ( int32 ) // hash bytes // sig length ( int 32 ) // sig bytes // algid len // algorithm id ( string ) // m_cryptoSession = reader.ReadInt32(); int count = reader.ReadInt32(); m_hash = reader.ReadBytes( count ); count = reader.ReadInt32(); m_signature = reader.ReadBytes( count ); m_hashAlgorithmOid = Utility.DeserializeString( reader ); IDT.ThrowInvalidArgumentConditional( 0 == m_cryptoSession, "cryptoSession" ); IDT.ThrowInvalidArgumentConditional( null == m_hash || 0 == m_hash.Length, "hash" ); IDT.ThrowInvalidArgumentConditional( null == m_signature || 0 == m_signature.Length, "signature" ); IDT.ThrowInvalidArgumentConditional( null == m_hashAlgorithmOid, "hashAlgorithmOid" ); } // // Summary: // Attach to the appropriate cryptosession and verify the signature. // protected override void OnProcess() { IDT.DebugAssert( 0 != m_cryptoSession, "null crypto session" ); IDT.DebugAssert( null != m_hash && 0 != m_hash.Length, "null hash" ); IDT.DebugAssert( null != m_signature && 0 != m_signature.Length, "null signature" ); IDT.DebugAssert( null != m_hashAlgorithmOid, "null hash algorithm "); AsymmetricCryptoSession session = ( AsymmetricCryptoSession )CryptoSession.Find( m_cryptoSession, CallerPid, RequestorIdentity.User ); m_verified = session.VerifyHash( m_hash, m_hashAlgorithmOid, m_signature ); } // // Summary: // Return our verified status in the output stream. // protected override void OnMarshalOutArgs() { IDT.DebugAssert( null != OutArgs, "Null out args" ); BinaryWriter writer = new BinaryWriter( OutArgs, Encoding.Unicode ); writer.Write( m_verified ); writer.Flush(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
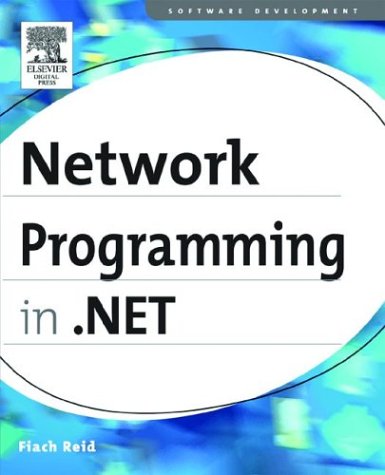
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DictionaryContent.cs
- WmlControlAdapter.cs
- UrlMappingsModule.cs
- UrlPath.cs
- CookieParameter.cs
- Environment.cs
- TypeExtension.cs
- BindingContext.cs
- ImageInfo.cs
- basecomparevalidator.cs
- ExtenderControl.cs
- ObjectListGeneralPage.cs
- AnnotationHighlightLayer.cs
- MembershipValidatePasswordEventArgs.cs
- x509utils.cs
- CodeIdentifier.cs
- WindowsListViewGroup.cs
- UserCancellationException.cs
- SqlDelegatedTransaction.cs
- BevelBitmapEffect.cs
- PkcsUtils.cs
- MessageFault.cs
- IssuedTokenServiceElement.cs
- HyperLinkColumn.cs
- SQLMoneyStorage.cs
- ZoneIdentityPermission.cs
- WorkflowApplication.cs
- HtmlTextArea.cs
- ToolTip.cs
- ActivityFunc.cs
- Timeline.cs
- RequestCacheValidator.cs
- CacheDict.cs
- CodeTypeConstructor.cs
- Point3DCollectionValueSerializer.cs
- Freezable.cs
- IndexExpression.cs
- WebHttpBehavior.cs
- WmlCommandAdapter.cs
- StylusDevice.cs
- TraceSection.cs
- PrintDialogException.cs
- XmlSecureResolver.cs
- SubpageParagraph.cs
- DbProviderFactoriesConfigurationHandler.cs
- ResourceManager.cs
- StackOverflowException.cs
- BamlRecordReader.cs
- CustomActivityDesigner.cs
- XmlReflectionMember.cs
- HttpRuntimeSection.cs
- Image.cs
- TargetException.cs
- DbConnectionClosed.cs
- WebPartChrome.cs
- SeekableReadStream.cs
- TdsRecordBufferSetter.cs
- Point3DAnimationBase.cs
- UniqueEventHelper.cs
- Brushes.cs
- InfocardChannelParameter.cs
- TransactionalPackage.cs
- MasterPageCodeDomTreeGenerator.cs
- lengthconverter.cs
- DataErrorValidationRule.cs
- ConfigXmlElement.cs
- WSSecurityTokenSerializer.cs
- Constants.cs
- Clause.cs
- RemoteHelper.cs
- MultiBinding.cs
- NotCondition.cs
- _ConnectionGroup.cs
- UnmanagedMemoryStream.cs
- MultiPropertyDescriptorGridEntry.cs
- ImpersonateTokenRef.cs
- TwoPhaseCommit.cs
- EllipseGeometry.cs
- AdPostCacheSubstitution.cs
- AsyncOperationManager.cs
- MulticastOption.cs
- PeerMaintainer.cs
- Hex.cs
- TraceListener.cs
- BamlLocalizableResource.cs
- StrongBox.cs
- WebControlAdapter.cs
- RootBuilder.cs
- DbParameterHelper.cs
- XsltException.cs
- ThumbButtonInfo.cs
- SqlClientFactory.cs
- Expressions.cs
- WriteTimeStream.cs
- CqlQuery.cs
- IOException.cs
- MobileListItemCollection.cs
- ContentControl.cs
- RemotingConfigParser.cs
- MetadataUtil.cs