Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / WebControls / CookieParameter.cs / 1305376 / CookieParameter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.ComponentModel; using System.Data; ////// Represents a Parameter that gets its value from the application's request parameters. /// [ DefaultProperty("CookieName"), ] public class CookieParameter : Parameter { ////// Creates an instance of the CookieParameter class. /// public CookieParameter() { } ////// Creates an instance of the CookieParameter class with the specified parameter name and request field. /// public CookieParameter(string name, string cookieName) : base(name) { CookieName = cookieName; } ////// Creates an instance of the CookieParameter class with the specified parameter name, database type, and /// request field. /// public CookieParameter(string name, DbType dbType, string cookieName) : base(name, dbType) { CookieName = cookieName; } ////// Creates an instance of the CookieParameter class with the specified parameter name, type, and request field. /// public CookieParameter(string name, TypeCode type, string cookieName) : base(name, type) { CookieName = cookieName; } ////// Used to clone a parameter. /// protected CookieParameter(CookieParameter original) : base(original) { CookieName = original.CookieName; } ////// The name of the request parameter to get the value from. /// [ DefaultValue(""), WebCategory("Parameter"), WebSysDescription(SR.CookieParameter_CookieName), ] public string CookieName { get { object o = ViewState["CookieName"]; if (o == null) return String.Empty; return (string)o; } set { if (CookieName != value) { ViewState["CookieName"] = value; OnParameterChanged(); } } } ////// Creates a new CookieParameter that is a copy of this CookieParameter. /// protected override Parameter Clone() { return new CookieParameter(this); } ////// Returns the updated value of the parameter. /// protected internal override object Evaluate(HttpContext context, Control control) { if (context == null || context.Request == null) { return null; } HttpCookie cookie = context.Request.Cookies[CookieName]; if (cookie == null) { return null; } return cookie.Value; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
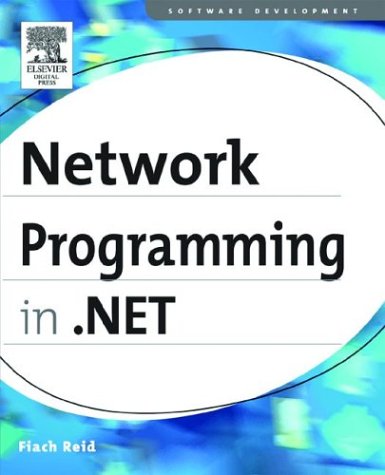
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NonParentingControl.cs
- StringPropertyBuilder.cs
- PropertiesTab.cs
- InvalidComObjectException.cs
- PolicyUtility.cs
- DataControlCommands.cs
- AutoGeneratedFieldProperties.cs
- TreeView.cs
- DrawTreeNodeEventArgs.cs
- MemoryFailPoint.cs
- BitmapSource.cs
- EncodingNLS.cs
- SqlDataSourceView.cs
- Ipv6Element.cs
- DataRowChangeEvent.cs
- InvalidEnumArgumentException.cs
- TextBlock.cs
- WebPartDisplayModeEventArgs.cs
- RbTree.cs
- mil_sdk_version.cs
- CriticalHandle.cs
- CodeAssignStatement.cs
- SqlRewriteScalarSubqueries.cs
- CreatingCookieEventArgs.cs
- Compress.cs
- ProcessProtocolHandler.cs
- BaseAppDomainProtocolHandler.cs
- TagMapInfo.cs
- ListItemConverter.cs
- WaitHandleCannotBeOpenedException.cs
- ConfigurationStrings.cs
- TransformValueSerializer.cs
- PropertyTabChangedEvent.cs
- WebPartVerb.cs
- DetailsViewCommandEventArgs.cs
- JsonByteArrayDataContract.cs
- PeerInvitationResponse.cs
- DataGridColumn.cs
- ExtractorMetadata.cs
- PrivilegeNotHeldException.cs
- PackageRelationshipSelector.cs
- AuthenticationService.cs
- XmlAnyAttributeAttribute.cs
- PropertyPathConverter.cs
- SelectionProcessor.cs
- Label.cs
- PrintPreviewControl.cs
- SynchronizationContext.cs
- HMACSHA512.cs
- smtpconnection.cs
- ExpandableObjectConverter.cs
- DesignerObject.cs
- XPathBinder.cs
- ScriptRef.cs
- WindowsPen.cs
- namescope.cs
- DynamicRenderer.cs
- StoreContentChangedEventArgs.cs
- EllipticalNodeOperations.cs
- HttpSessionStateBase.cs
- SelectionListComponentEditor.cs
- _Rfc2616CacheValidators.cs
- BamlResourceDeserializer.cs
- SafeFileMapViewHandle.cs
- ListManagerBindingsCollection.cs
- translator.cs
- ArrangedElement.cs
- ReachSerializableProperties.cs
- StorageMappingItemCollection.cs
- DataKey.cs
- BinaryCommonClasses.cs
- Native.cs
- CallTemplateAction.cs
- CqlGenerator.cs
- PersonalizationState.cs
- TableStyle.cs
- TreePrinter.cs
- CredentialCache.cs
- XmlValidatingReader.cs
- SizeKeyFrameCollection.cs
- CacheVirtualItemsEvent.cs
- OperandQuery.cs
- ScalarType.cs
- _UriSyntax.cs
- ProfileModule.cs
- NetNamedPipeSecurity.cs
- EnglishPluralizationService.cs
- PreparingEnlistment.cs
- ConcurrentStack.cs
- RC2CryptoServiceProvider.cs
- MatrixValueSerializer.cs
- ListViewGroupItemCollection.cs
- FrugalList.cs
- Vector3D.cs
- CanExecuteRoutedEventArgs.cs
- SecurityTokenValidationException.cs
- AudienceUriMode.cs
- PageThemeParser.cs
- TransactionInterop.cs
- SqlClientWrapperSmiStream.cs