Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / Abstractions / HttpSessionStateBase.cs / 1305376 / HttpSessionStateBase.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web { using System.Collections; using System.Collections.Specialized; using System.Diagnostics.CodeAnalysis; using System.Web.SessionState; using System.Runtime.CompilerServices; [TypeForwardedFrom("System.Web.Abstractions, Version=3.5.0.0, Culture=Neutral, PublicKeyToken=31bf3856ad364e35")] [SuppressMessage("Microsoft.Naming", "CA1710:IdentifiersShouldHaveCorrectSuffix", Justification = "This is consistent with the type it abstracts in System.Web.dll.")] public abstract class HttpSessionStateBase : ICollection, IEnumerable { public virtual int CodePage { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual HttpSessionStateBase Contents { get { throw new NotImplementedException(); } } public virtual HttpCookieMode CookieMode { get { throw new NotImplementedException(); } } public virtual bool IsCookieless { get { throw new NotImplementedException(); } } public virtual bool IsNewSession { get { throw new NotImplementedException(); } } public virtual bool IsReadOnly { get { throw new NotImplementedException(); } } public virtual NameObjectCollectionBase.KeysCollection Keys { get { throw new NotImplementedException(); } } [SuppressMessage("Microsoft.Naming", "CA1705:LongAcronymsShouldBePascalCased", Justification = "This is consistent with the type it abstracts in System.Web.dll.")] public virtual int LCID { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual SessionStateMode Mode { get { throw new NotImplementedException(); } } [SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "ID", Justification = "This is consistent with the type it abstracts in System.Web.dll.")] public virtual string SessionID { get { throw new NotImplementedException(); } } public virtual HttpStaticObjectsCollectionBase StaticObjects { get { throw new NotImplementedException(); } } public virtual int Timeout { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual object this[int index] { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual object this[string name] { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual void Abandon() { throw new NotImplementedException(); } public virtual void Add(string name, object value) { throw new NotImplementedException(); } public virtual void Clear() { throw new NotImplementedException(); } public virtual void Remove(string name) { throw new NotImplementedException(); } public virtual void RemoveAll() { throw new NotImplementedException(); } public virtual void RemoveAt(int index) { throw new NotImplementedException(); } #region ICollection Members [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public virtual void CopyTo(Array array, int index) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public virtual int Count { get { throw new NotImplementedException(); } } [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public virtual bool IsSynchronized { get { throw new NotImplementedException(); } } [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public virtual object SyncRoot { get { throw new NotImplementedException(); } } #endregion #region IEnumerable Members [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public virtual IEnumerator GetEnumerator() { throw new NotImplementedException(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web { using System.Collections; using System.Collections.Specialized; using System.Diagnostics.CodeAnalysis; using System.Web.SessionState; using System.Runtime.CompilerServices; [TypeForwardedFrom("System.Web.Abstractions, Version=3.5.0.0, Culture=Neutral, PublicKeyToken=31bf3856ad364e35")] [SuppressMessage("Microsoft.Naming", "CA1710:IdentifiersShouldHaveCorrectSuffix", Justification = "This is consistent with the type it abstracts in System.Web.dll.")] public abstract class HttpSessionStateBase : ICollection, IEnumerable { public virtual int CodePage { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual HttpSessionStateBase Contents { get { throw new NotImplementedException(); } } public virtual HttpCookieMode CookieMode { get { throw new NotImplementedException(); } } public virtual bool IsCookieless { get { throw new NotImplementedException(); } } public virtual bool IsNewSession { get { throw new NotImplementedException(); } } public virtual bool IsReadOnly { get { throw new NotImplementedException(); } } public virtual NameObjectCollectionBase.KeysCollection Keys { get { throw new NotImplementedException(); } } [SuppressMessage("Microsoft.Naming", "CA1705:LongAcronymsShouldBePascalCased", Justification = "This is consistent with the type it abstracts in System.Web.dll.")] public virtual int LCID { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual SessionStateMode Mode { get { throw new NotImplementedException(); } } [SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "ID", Justification = "This is consistent with the type it abstracts in System.Web.dll.")] public virtual string SessionID { get { throw new NotImplementedException(); } } public virtual HttpStaticObjectsCollectionBase StaticObjects { get { throw new NotImplementedException(); } } public virtual int Timeout { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual object this[int index] { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual object this[string name] { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual void Abandon() { throw new NotImplementedException(); } public virtual void Add(string name, object value) { throw new NotImplementedException(); } public virtual void Clear() { throw new NotImplementedException(); } public virtual void Remove(string name) { throw new NotImplementedException(); } public virtual void RemoveAll() { throw new NotImplementedException(); } public virtual void RemoveAt(int index) { throw new NotImplementedException(); } #region ICollection Members [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public virtual void CopyTo(Array array, int index) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public virtual int Count { get { throw new NotImplementedException(); } } [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public virtual bool IsSynchronized { get { throw new NotImplementedException(); } } [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public virtual object SyncRoot { get { throw new NotImplementedException(); } } #endregion #region IEnumerable Members [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public virtual IEnumerator GetEnumerator() { throw new NotImplementedException(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
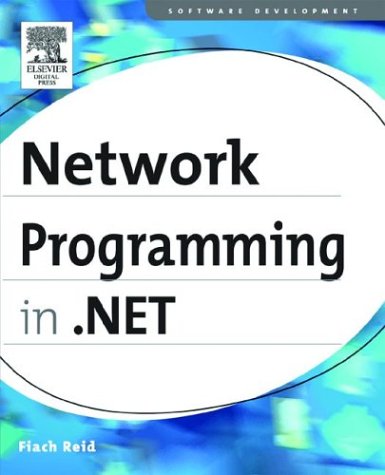
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LinkArea.cs
- Subset.cs
- StringTraceRecord.cs
- SEHException.cs
- BamlCollectionHolder.cs
- CodePrimitiveExpression.cs
- PropertyDescriptorCollection.cs
- ObjectDataSourceMethodEventArgs.cs
- SqlExpander.cs
- NavigatorInput.cs
- Query.cs
- Rectangle.cs
- WebPartVerbCollection.cs
- CodeIdentifiers.cs
- SecurityTokenAuthenticator.cs
- IDictionary.cs
- ToolBarButtonClickEvent.cs
- XmlCharCheckingWriter.cs
- WebResponse.cs
- DataGridViewImageColumn.cs
- RsaSecurityTokenAuthenticator.cs
- WebBrowserBase.cs
- HttpCookieCollection.cs
- SymbolTable.cs
- ToolBar.cs
- RecognizerBase.cs
- ServiceOperationViewControl.cs
- ComponentDispatcherThread.cs
- MetadataSerializer.cs
- NotifyCollectionChangedEventArgs.cs
- PackageFilter.cs
- GeometryGroup.cs
- DbConnectionStringBuilder.cs
- FileLogRecordEnumerator.cs
- BoolExpression.cs
- MimePart.cs
- ReadOnlyCollection.cs
- TransactedBatchingElement.cs
- CorrelationResolver.cs
- PolyQuadraticBezierSegment.cs
- ReadOnlyHierarchicalDataSourceView.cs
- ScriptHandlerFactory.cs
- Deflater.cs
- Matrix3DStack.cs
- ByteStreamMessageUtility.cs
- MemberExpression.cs
- CacheModeValueSerializer.cs
- CodeBlockBuilder.cs
- LinqMaximalSubtreeNominator.cs
- RequestNavigateEventArgs.cs
- CodeTypeConstructor.cs
- UserNameSecurityTokenProvider.cs
- FileUpload.cs
- StructuralObject.cs
- Rotation3DKeyFrameCollection.cs
- SiteMapHierarchicalDataSourceView.cs
- SharedStatics.cs
- WebPartPersonalization.cs
- LinqDataSourceContextEventArgs.cs
- WriteableBitmap.cs
- ThicknessConverter.cs
- TextSegment.cs
- TextBox.cs
- HotSpot.cs
- SiteIdentityPermission.cs
- SimpleBitVector32.cs
- IODescriptionAttribute.cs
- OdbcStatementHandle.cs
- FontResourceCache.cs
- ExceptionUtil.cs
- CrossAppDomainChannel.cs
- DBConnectionString.cs
- AudioDeviceOut.cs
- SafeHandles.cs
- SplitterPanel.cs
- StyleTypedPropertyAttribute.cs
- LogReserveAndAppendState.cs
- HTMLTagNameToTypeMapper.cs
- InvalidPipelineStoreException.cs
- DataGridTable.cs
- FullTrustAssembly.cs
- Comparer.cs
- Funcletizer.cs
- DefaultAssemblyResolver.cs
- Style.cs
- FirstMatchCodeGroup.cs
- XmlComplianceUtil.cs
- XPathAncestorIterator.cs
- Dump.cs
- EntityProxyFactory.cs
- DropShadowBitmapEffect.cs
- PreProcessor.cs
- ToolStripGripRenderEventArgs.cs
- ListSurrogate.cs
- XmlIgnoreAttribute.cs
- ProcessHostServerConfig.cs
- ScopeElementCollection.cs
- TemplatePartAttribute.cs
- StreamReader.cs
- SmiEventSink_DeferedProcessing.cs