Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / HttpCookieCollection.cs / 4 / HttpCookieCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Collection of Http cookies for request and response intrinsics * * Copyright (c) 1998 Microsoft Corporation */ namespace System.Web { using System.Runtime.InteropServices; using System.Collections; using System.Collections.Specialized; using System.Security.Permissions; using System.Web.Util; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class HttpCookieCollection : NameObjectCollectionBase { // Response object to notify about changes in collection private HttpResponse _response; // cached All[] arrays private HttpCookie[] _all; private String[] _allKeys; private bool _changed; internal HttpCookieCollection(HttpResponse response, bool readOnly) : base(StringComparer.OrdinalIgnoreCase) { _response = response; IsReadOnly = readOnly; } ////// Provides a type-safe /// way to manipulate HTTP cookies. /// ////// public HttpCookieCollection(): base(StringComparer.OrdinalIgnoreCase) { } internal bool Changed { get { return _changed; } set { _changed = value; } } internal void AddCookie(HttpCookie cookie, bool append) { _all = null; _allKeys = null; if (append) { // mark cookie as new cookie.Added = true; BaseAdd(cookie.Name, cookie); } else { if (BaseGet(cookie.Name) != null) { // mark the cookie as changed because we are overriding the existing one cookie.Changed = true; } BaseSet(cookie.Name, cookie); } } internal void RemoveCookie(String name) { _all = null; _allKeys = null; BaseRemove(name); _changed = true; } internal void Reset() { _all = null; _allKeys = null; BaseClear(); _changed = true; } // // Public APIs to add / remove // ////// Initializes a new instance of the HttpCookieCollection /// class. /// ////// public void Add(HttpCookie cookie) { if (_response != null) _response.BeforeCookieCollectionChange(); AddCookie(cookie, true); if (_response != null) _response.OnCookieAdd(cookie); } ////// Adds a cookie to the collection. /// ////// public void CopyTo(Array dest, int index) { if (_all == null) { int n = Count; _all = new HttpCookie[n]; for (int i = 0; i < n; i++) _all[i] = Get(i); } _all.CopyTo(dest, index); } ///[To be supplied.] ////// public void Set(HttpCookie cookie) { if (_response != null) _response.BeforeCookieCollectionChange(); AddCookie(cookie, false); if (_response != null) _response.OnCookieCollectionChange(); } ///Updates the value of a cookie. ////// public void Remove(String name) { if (_response != null) _response.BeforeCookieCollectionChange(); RemoveCookie(name); if (_response != null) _response.OnCookieCollectionChange(); } ////// Removes a cookie from the collection. /// ////// public void Clear() { Reset(); } // // Access by name // ////// Clears all cookies from the collection. /// ////// public HttpCookie Get(String name) { HttpCookie cookie = (HttpCookie)BaseGet(name); if (cookie == null && _response != null) { // response cookies are created on demand cookie = new HttpCookie(name); AddCookie(cookie, true); _response.OnCookieAdd(cookie); } return cookie; } ///Returns an ///item from the collection. /// public HttpCookie this[String name] { get { return Get(name);} } // // Indexed access // ///Indexed value that enables access to a cookie in the collection. ////// public HttpCookie Get(int index) { return(HttpCookie)BaseGet(index); } ////// Returns an ////// item from the collection. /// /// public String GetKey(int index) { return BaseGetKey(index); } ////// Returns key name from collection. /// ////// public HttpCookie this[int index] { get { return Get(index);} } // // Access to keys and values as arrays // /* * All keys */ ////// Default property. /// Indexed property that enables access to a cookie in the collection. /// ////// public String[] AllKeys { get { if (_allKeys == null) _allKeys = BaseGetAllKeys(); return _allKeys; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Returns /// an array of all cookie keys in the cookie collection. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Collection of Http cookies for request and response intrinsics * * Copyright (c) 1998 Microsoft Corporation */ namespace System.Web { using System.Runtime.InteropServices; using System.Collections; using System.Collections.Specialized; using System.Security.Permissions; using System.Web.Util; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class HttpCookieCollection : NameObjectCollectionBase { // Response object to notify about changes in collection private HttpResponse _response; // cached All[] arrays private HttpCookie[] _all; private String[] _allKeys; private bool _changed; internal HttpCookieCollection(HttpResponse response, bool readOnly) : base(StringComparer.OrdinalIgnoreCase) { _response = response; IsReadOnly = readOnly; } ////// Provides a type-safe /// way to manipulate HTTP cookies. /// ////// public HttpCookieCollection(): base(StringComparer.OrdinalIgnoreCase) { } internal bool Changed { get { return _changed; } set { _changed = value; } } internal void AddCookie(HttpCookie cookie, bool append) { _all = null; _allKeys = null; if (append) { // mark cookie as new cookie.Added = true; BaseAdd(cookie.Name, cookie); } else { if (BaseGet(cookie.Name) != null) { // mark the cookie as changed because we are overriding the existing one cookie.Changed = true; } BaseSet(cookie.Name, cookie); } } internal void RemoveCookie(String name) { _all = null; _allKeys = null; BaseRemove(name); _changed = true; } internal void Reset() { _all = null; _allKeys = null; BaseClear(); _changed = true; } // // Public APIs to add / remove // ////// Initializes a new instance of the HttpCookieCollection /// class. /// ////// public void Add(HttpCookie cookie) { if (_response != null) _response.BeforeCookieCollectionChange(); AddCookie(cookie, true); if (_response != null) _response.OnCookieAdd(cookie); } ////// Adds a cookie to the collection. /// ////// public void CopyTo(Array dest, int index) { if (_all == null) { int n = Count; _all = new HttpCookie[n]; for (int i = 0; i < n; i++) _all[i] = Get(i); } _all.CopyTo(dest, index); } ///[To be supplied.] ////// public void Set(HttpCookie cookie) { if (_response != null) _response.BeforeCookieCollectionChange(); AddCookie(cookie, false); if (_response != null) _response.OnCookieCollectionChange(); } ///Updates the value of a cookie. ////// public void Remove(String name) { if (_response != null) _response.BeforeCookieCollectionChange(); RemoveCookie(name); if (_response != null) _response.OnCookieCollectionChange(); } ////// Removes a cookie from the collection. /// ////// public void Clear() { Reset(); } // // Access by name // ////// Clears all cookies from the collection. /// ////// public HttpCookie Get(String name) { HttpCookie cookie = (HttpCookie)BaseGet(name); if (cookie == null && _response != null) { // response cookies are created on demand cookie = new HttpCookie(name); AddCookie(cookie, true); _response.OnCookieAdd(cookie); } return cookie; } ///Returns an ///item from the collection. /// public HttpCookie this[String name] { get { return Get(name);} } // // Indexed access // ///Indexed value that enables access to a cookie in the collection. ////// public HttpCookie Get(int index) { return(HttpCookie)BaseGet(index); } ////// Returns an ////// item from the collection. /// /// public String GetKey(int index) { return BaseGetKey(index); } ////// Returns key name from collection. /// ////// public HttpCookie this[int index] { get { return Get(index);} } // // Access to keys and values as arrays // /* * All keys */ ////// Default property. /// Indexed property that enables access to a cookie in the collection. /// ////// public String[] AllKeys { get { if (_allKeys == null) _allKeys = BaseGetAllKeys(); return _allKeys; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Returns /// an array of all cookie keys in the cookie collection. /// ///
Link Menu
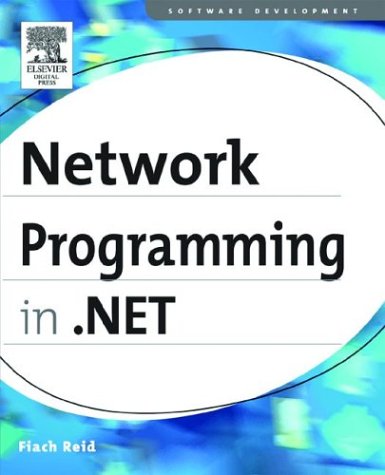
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ParamArrayAttribute.cs
- PathTooLongException.cs
- DesignerRegionCollection.cs
- CriticalHandle.cs
- Label.cs
- System.Data_BID.cs
- RankException.cs
- ResXResourceWriter.cs
- TextDecorationLocationValidation.cs
- FixedSOMImage.cs
- WorkflowMarkupElementEventArgs.cs
- PackageRelationshipCollection.cs
- AttributeCollection.cs
- TrackingAnnotationCollection.cs
- InstanceKeyCollisionException.cs
- StorageComplexPropertyMapping.cs
- ValueType.cs
- PointAnimationUsingKeyFrames.cs
- ObfuscateAssemblyAttribute.cs
- DataGridColumnEventArgs.cs
- PackWebRequest.cs
- DirectoryNotFoundException.cs
- ISAPIApplicationHost.cs
- BamlResourceContent.cs
- MailWebEventProvider.cs
- HttpEncoder.cs
- EmptyStringExpandableObjectConverter.cs
- TargetControlTypeAttribute.cs
- CodeTypeReferenceCollection.cs
- DataGridViewRowEventArgs.cs
- DatePickerDateValidationErrorEventArgs.cs
- XmlSchemaProviderAttribute.cs
- HtmlInputFile.cs
- OracleTimeSpan.cs
- ThreadPool.cs
- ISFClipboardData.cs
- ContextQuery.cs
- XmlUtf8RawTextWriter.cs
- ScrollEventArgs.cs
- Dynamic.cs
- RefreshEventArgs.cs
- Type.cs
- ThreadPool.cs
- DriveInfo.cs
- HoistedLocals.cs
- SqlLiftWhereClauses.cs
- StylusPointPropertyId.cs
- TextEffect.cs
- DelegateOutArgument.cs
- ActivityPropertyReference.cs
- MailDefinitionBodyFileNameEditor.cs
- InfoCardSymmetricCrypto.cs
- SecurityTokenValidationException.cs
- ConsumerConnectionPointCollection.cs
- TypeReference.cs
- RemotingService.cs
- WorkflowRuntimeSection.cs
- EditorZone.cs
- CodeCatchClauseCollection.cs
- InplaceBitmapMetadataWriter.cs
- IdnElement.cs
- TableLayoutStyleCollection.cs
- PreProcessInputEventArgs.cs
- ValidatingReaderNodeData.cs
- TransactionsSectionGroup.cs
- CompilerError.cs
- ErrorFormatter.cs
- LogicalExpr.cs
- ProtocolsConfiguration.cs
- TemplateBindingExtensionConverter.cs
- SQLDecimal.cs
- StringUtil.cs
- CopyOfAction.cs
- CommandField.cs
- Directory.cs
- SortedDictionary.cs
- NumberFunctions.cs
- SqlIdentifier.cs
- ComponentDispatcherThread.cs
- CompoundFileStorageReference.cs
- SafeNativeMethods.cs
- OLEDB_Util.cs
- ServiceDescriptionSerializer.cs
- BamlRecords.cs
- BuildProviderCollection.cs
- RuntimeConfig.cs
- PathTooLongException.cs
- XmlSchemaImport.cs
- CompilationUtil.cs
- WebPartHelpVerb.cs
- ImageInfo.cs
- FormsAuthenticationUser.cs
- Rotation3D.cs
- ControlIdConverter.cs
- WinEventQueueItem.cs
- SqlCrossApplyToCrossJoin.cs
- Membership.cs
- ScriptReference.cs
- rsa.cs
- SimpleRecyclingCache.cs