Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / SystemNet / Net / UnsafeMethods.cs / 2 / UnsafeMethods.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System; using System.Collections.Generic; using System.Text; using Microsoft.Win32.SafeHandles; using System.Security; using System.Runtime.InteropServices; using System.Runtime.ConstrainedExecution; using System.Threading; using System.Net.Sockets; [Flags] internal enum FormatMessageFlags : uint { FORMAT_MESSAGE_ALLOCATE_BUFFER = 0x00000100, //FORMAT_MESSAGE_IGNORE_INSERTS = 0x00000200, //FORMAT_MESSAGE_FROM_STRING = 0x00000400, FORMAT_MESSAGE_FROM_HMODULE = 0x00000800, FORMAT_MESSAGE_FROM_SYSTEM = 0x00001000, FORMAT_MESSAGE_ARGUMENT_ARRAY = 0x00002000 } [System.Security.SuppressUnmanagedCodeSecurityAttribute()] internal static class UnsafeSystemNativeMethods { private const string KERNEL32 = "KERNEL32.dll"; [System.Security.SecurityCritical] [DllImport(KERNEL32, ExactSpelling = true, CharSet = CharSet.Unicode, SetLastError = true)] internal static extern unsafe SafeLoadLibrary LoadLibraryExW( string lpwLibFileName, [In] void* hFile, uint dwFlags); [DllImport(KERNEL32, ExactSpelling = true, SetLastError = true)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [return: MarshalAs(UnmanagedType.Bool)] internal static extern unsafe bool FreeLibrary(IntPtr hModule); [System.Security.SecurityCritical] [DllImport(KERNEL32, EntryPoint = "GetProcAddress", SetLastError = true, BestFitMapping = false)] internal extern static IntPtr GetProcAddress(SafeLoadLibrary hModule, string entryPoint); [DllImport(KERNEL32, CharSet = CharSet.Unicode)] internal extern static uint FormatMessage( FormatMessageFlags dwFlags, IntPtr lpSource, UInt32 dwMessageId, UInt32 dwLanguageId, ref IntPtr lpBuffer, UInt32 nSize, IntPtr vaArguments ); [DllImport(KERNEL32, CharSet = CharSet.Unicode)] internal extern static uint LocalFree(IntPtr lpMem); } //// [System.Security.SecurityCritical(System.Security.SecurityCriticalScope.Everything)] [System.Security.SuppressUnmanagedCodeSecurityAttribute()] internal sealed class SafeLoadLibrary : SafeHandleZeroOrMinusOneIsInvalid { private const string KERNEL32 = "kernel32.dll"; private SafeLoadLibrary() : base(true) { } //private SafeLoadLibrary(bool ownsHandle) : base(ownsHandle) { } //internal static readonly SafeLoadLibrary Zero = new SafeLoadLibrary(false); internal unsafe static SafeLoadLibrary LoadLibraryEx(string library) { SafeLoadLibrary result = UnsafeSystemNativeMethods.LoadLibraryExW(library, null, 0); if (result.IsInvalid) { //NOTE: //IsInvalid tests the numeric value of the handle. //SetHandleAsInvalid sets the handle as closed, so that further closing //does not have to take place in the critical finalizer thread. // //You would think that when you assign 0 or -1 to an instance of //SafeHandleZeroOrMinusoneIsInvalid, the handle will not be closed, since after all it is invalid //It turns out that the SafeHandleZetoOrMinusOneIsInvalid overrides only the IsInvalid() method //It does not do anything to automatically close it. //So we have to SetHandleAsInvalid --> Which means mark it closed -- so that //we will not eventually call CloseHandle on 0 or -1 result.SetHandleAsInvalid(); } return result; } protected override bool ReleaseHandle() { return UnsafeSystemNativeMethods.FreeLibrary(handle); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System; using System.Collections.Generic; using System.Text; using Microsoft.Win32.SafeHandles; using System.Security; using System.Runtime.InteropServices; using System.Runtime.ConstrainedExecution; using System.Threading; using System.Net.Sockets; [Flags] internal enum FormatMessageFlags : uint { FORMAT_MESSAGE_ALLOCATE_BUFFER = 0x00000100, //FORMAT_MESSAGE_IGNORE_INSERTS = 0x00000200, //FORMAT_MESSAGE_FROM_STRING = 0x00000400, FORMAT_MESSAGE_FROM_HMODULE = 0x00000800, FORMAT_MESSAGE_FROM_SYSTEM = 0x00001000, FORMAT_MESSAGE_ARGUMENT_ARRAY = 0x00002000 } [System.Security.SuppressUnmanagedCodeSecurityAttribute()] internal static class UnsafeSystemNativeMethods { private const string KERNEL32 = "KERNEL32.dll"; [System.Security.SecurityCritical] [DllImport(KERNEL32, ExactSpelling = true, CharSet = CharSet.Unicode, SetLastError = true)] internal static extern unsafe SafeLoadLibrary LoadLibraryExW( string lpwLibFileName, [In] void* hFile, uint dwFlags); [DllImport(KERNEL32, ExactSpelling = true, SetLastError = true)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [return: MarshalAs(UnmanagedType.Bool)] internal static extern unsafe bool FreeLibrary(IntPtr hModule); [System.Security.SecurityCritical] [DllImport(KERNEL32, EntryPoint = "GetProcAddress", SetLastError = true, BestFitMapping = false)] internal extern static IntPtr GetProcAddress(SafeLoadLibrary hModule, string entryPoint); [DllImport(KERNEL32, CharSet = CharSet.Unicode)] internal extern static uint FormatMessage( FormatMessageFlags dwFlags, IntPtr lpSource, UInt32 dwMessageId, UInt32 dwLanguageId, ref IntPtr lpBuffer, UInt32 nSize, IntPtr vaArguments ); [DllImport(KERNEL32, CharSet = CharSet.Unicode)] internal extern static uint LocalFree(IntPtr lpMem); } //// [System.Security.SecurityCritical(System.Security.SecurityCriticalScope.Everything)] [System.Security.SuppressUnmanagedCodeSecurityAttribute()] internal sealed class SafeLoadLibrary : SafeHandleZeroOrMinusOneIsInvalid { private const string KERNEL32 = "kernel32.dll"; private SafeLoadLibrary() : base(true) { } //private SafeLoadLibrary(bool ownsHandle) : base(ownsHandle) { } //internal static readonly SafeLoadLibrary Zero = new SafeLoadLibrary(false); internal unsafe static SafeLoadLibrary LoadLibraryEx(string library) { SafeLoadLibrary result = UnsafeSystemNativeMethods.LoadLibraryExW(library, null, 0); if (result.IsInvalid) { //NOTE: //IsInvalid tests the numeric value of the handle. //SetHandleAsInvalid sets the handle as closed, so that further closing //does not have to take place in the critical finalizer thread. // //You would think that when you assign 0 or -1 to an instance of //SafeHandleZeroOrMinusoneIsInvalid, the handle will not be closed, since after all it is invalid //It turns out that the SafeHandleZetoOrMinusOneIsInvalid overrides only the IsInvalid() method //It does not do anything to automatically close it. //So we have to SetHandleAsInvalid --> Which means mark it closed -- so that //we will not eventually call CloseHandle on 0 or -1 result.SetHandleAsInvalid(); } return result; } protected override bool ReleaseHandle() { return UnsafeSystemNativeMethods.FreeLibrary(handle); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.//
Link Menu
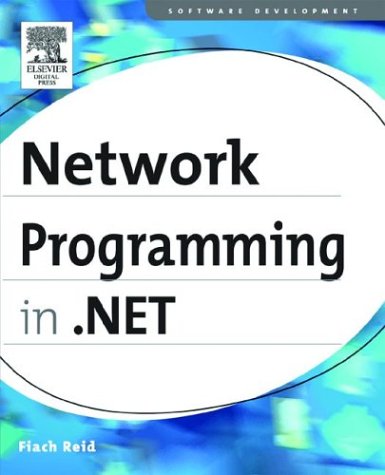
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ErrorFormatterPage.cs
- InternalException.cs
- GACMembershipCondition.cs
- OleDbParameterCollection.cs
- InstanceStore.cs
- ByteStream.cs
- CurrentChangedEventManager.cs
- Bidi.cs
- MessageSecurityException.cs
- DiscoveryCallbackBehavior.cs
- ConfigurationValidatorBase.cs
- SR.cs
- WindowsSecurityToken.cs
- CalloutQueueItem.cs
- Decoder.cs
- BypassElementCollection.cs
- NamespaceDecl.cs
- Operators.cs
- FontWeight.cs
- XmlCountingReader.cs
- HtmlInputImage.cs
- WebPageTraceListener.cs
- FileUpload.cs
- StaticFileHandler.cs
- WebPartEditorApplyVerb.cs
- AssociationSetMetadata.cs
- ParameterEditorUserControl.cs
- StyleCollection.cs
- VBIdentifierNameEditor.cs
- MSHTMLHost.cs
- QueryContinueDragEventArgs.cs
- ByteKeyFrameCollection.cs
- SystemInfo.cs
- ToolBarButtonDesigner.cs
- RootBrowserWindowProxy.cs
- RawStylusInputCustomData.cs
- ParameterModifier.cs
- SpeechUI.cs
- MailAddressCollection.cs
- SmiEventSink_DeferedProcessing.cs
- Triplet.cs
- CodeAttributeArgumentCollection.cs
- OracleDateTime.cs
- SignedInfo.cs
- DbDeleteCommandTree.cs
- UriTemplateDispatchFormatter.cs
- HtmlSelect.cs
- MouseDevice.cs
- TextParagraph.cs
- StylusPointPropertyUnit.cs
- JsonUriDataContract.cs
- XmlDataProvider.cs
- ComplexBindingPropertiesAttribute.cs
- HttpPostServerProtocol.cs
- TabControlEvent.cs
- RoamingStoreFile.cs
- DisplayNameAttribute.cs
- DefaultObjectMappingItemCollection.cs
- AdjustableArrowCap.cs
- SplitterCancelEvent.cs
- Pens.cs
- TitleStyle.cs
- DataTrigger.cs
- ClientBuildManager.cs
- TextBoxBase.cs
- RtfControls.cs
- SelectionWordBreaker.cs
- LinkLabel.cs
- TransportChannelListener.cs
- SimpleNameService.cs
- GeneralTransform2DTo3DTo2D.cs
- XmlSchemaGroupRef.cs
- SystemUnicastIPAddressInformation.cs
- TargetControlTypeAttribute.cs
- FontSourceCollection.cs
- StickyNote.cs
- ModuleBuilderData.cs
- DBSchemaRow.cs
- MetadataProperty.cs
- EditorPart.cs
- HostedNamedPipeTransportManager.cs
- SqlServer2KCompatibilityAnnotation.cs
- XPathPatternBuilder.cs
- InvokeHandlers.cs
- ThreadLocal.cs
- ZoomingMessageFilter.cs
- WaveHeader.cs
- StorageComplexPropertyMapping.cs
- PublisherIdentityPermission.cs
- DrawingBrush.cs
- InfiniteTimeSpanConverter.cs
- DataGridViewDataConnection.cs
- EntityParameterCollection.cs
- ItemContainerProviderWrapper.cs
- XmlSerializerAssemblyAttribute.cs
- FormViewUpdatedEventArgs.cs
- SmiContextFactory.cs
- rsa.cs
- DeobfuscatingStream.cs
- FloatUtil.cs