Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / RoamingStoreFile.cs / 1 / RoamingStoreFile.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Collections.Generic; using System.Text; using System.Xml; using System.Xml.Serialization; using System.IO; using System.Security.Cryptography.X509Certificates; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary: // Reads and writes the Roaming store xml file format. // internal sealed class RoamingStoreFile { Listm_infocards; public RoamingStoreFile( ) { m_infocards = new List ( ); } // // Summary: // Get the list of cards that will be written or were read. // public IList Cards { get { return m_infocards; } } public void Clear() { foreach( InfoCard icard in m_infocards ) { icard.ClearSensitiveData(); } m_infocards.Clear(); } // // Summary: // Encrypts and writes the data to the specified xml writer. // public void WriteTo( string password, XmlWriter writer ) { byte[] cipherValue = null; byte[] newSalt = null; using( MemoryStream tempStream = new MemoryStream( ) ) { XmlWriterSettings settings = new XmlWriterSettings( ); settings.OmitXmlDeclaration = true; settings.CloseOutput = false; settings.Encoding = Encoding.UTF8; using( XmlWriter eltWriter = XmlWriter.Create( tempStream, settings ) ) { eltWriter.WriteStartElement( XmlNames.WSIdentity.RoamingStoreElement, XmlNames.WSIdentity.Namespace ); foreach( InfoCard serialzable in m_infocards ) { serialzable.WriteXml( eltWriter ); } eltWriter.WriteEndElement( ); eltWriter.Flush(); } tempStream.Flush( ); tempStream.Seek( 0, SeekOrigin.Begin ); cipherValue = new byte[ RoamingStoreFileUtility.CalculateEncryptedSize( Convert.ToInt32( tempStream.Length ) ) ]; using( MemoryStream encryptedStream = new MemoryStream( cipherValue ) ) { RoamingStoreFileUtility.Encrypt( tempStream, encryptedStream, password, out newSalt ); encryptedStream.Flush( ); } } writer.WriteStartElement( XmlNames.WSIdentity.EncryptedStoreElement, XmlNames.WSIdentity.Namespace ); writer.WriteStartElement( XmlNames.WSIdentity.StoreSaltElement, XmlNames.WSIdentity.Namespace ); writer.WriteBase64( newSalt, 0, newSalt.Length ); writer.WriteEndElement( ); writer.WriteStartElement( XmlNames.XmlEnc.EncryptedData,XmlNames.XmlEnc.Namespace ); writer.WriteStartElement( XmlNames.XmlEnc.CipherData,XmlNames.XmlEnc.Namespace ); writer.WriteStartElement( XmlNames.XmlEnc.CipherValue,XmlNames.XmlEnc.Namespace ); writer.WriteBase64( cipherValue, 0, cipherValue.Length ); writer.WriteEndElement( ); writer.WriteEndElement( ); writer.WriteEndElement( ); writer.WriteEndElement( ); } // // Summary: // Reads and decrypts the xml stream using the specified password. // public void ReadFrom( string password, XmlReader reader ) { if( !reader.IsStartElement( XmlNames.WSIdentity.EncryptedStoreElement, XmlNames.WSIdentity.Namespace ) ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.InvalidImportFile ) ) ); } if( !reader.ReadToDescendant( XmlNames.WSIdentity.StoreSaltElement, XmlNames.WSIdentity.Namespace ) ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.InvalidImportFile ) ) ); } byte[] salt = new byte[ RoamingStoreFileUtility.SaltLength ]; reader.ReadElementContentAsBase64( salt, 0, salt.Length ); if( 0 != reader.ReadElementContentAsBase64( new byte[ 1 ], 0, 1 ) ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.InvalidImportFile ) ) ); } if( !reader.IsStartElement( XmlNames.XmlEnc.EncryptedData,XmlNames.XmlEnc.Namespace ) ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.InvalidImportFile )) ); } if( !reader.ReadToDescendant( XmlNames.XmlEnc.CipherData,XmlNames.XmlEnc.Namespace ) ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.InvalidImportFile )) ); } if( !reader.ReadToDescendant( XmlNames.XmlEnc.CipherValue,XmlNames.XmlEnc.Namespace ) ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.InvalidImportFile ) ) ); } using( MemoryStream encrypted = new MemoryStream( 4096 ) ) { byte[] block = new byte[ 1024 ]; int bytesRead = 0; while( ( bytesRead = reader.ReadElementContentAsBase64( block, 0, block.Length ) ) > 0 ) { encrypted.Write( block, 0, bytesRead ); } encrypted.Flush( ); encrypted.Seek( 0, SeekOrigin.Begin ); using( MemoryStream decrypted = new MemoryStream( RoamingStoreFileUtility.CalculateDecryptedSize(Convert.ToInt32(encrypted.Length)) ) ) { RoamingStoreFileUtility.Decrypt( encrypted, decrypted, password, salt ); decrypted.Flush( ); decrypted.Seek( 0, SeekOrigin.Begin ); using( XmlReader decryptedReader = InfoCardSchemas.CreateReader( decrypted, reader.Settings ) ) { decryptedReader.Read( ); if( !decryptedReader.IsStartElement( XmlNames.WSIdentity.RoamingStoreElement, XmlNames.WSIdentity.Namespace ) ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.InvalidImportFile ) ) ); } if( decryptedReader.ReadToDescendant( XmlNames.WSIdentity.RoamingInfoCardElement, XmlNames.WSIdentity.Namespace ) ) { do { InfoCard card = new InfoCard(); card.ReadXml( decryptedReader ); m_infocards.Add(card); decryptedReader.ReadEndElement( ); } while( decryptedReader.IsStartElement( XmlNames.WSIdentity.RoamingInfoCardElement, XmlNames.WSIdentity.Namespace ) ); } decryptedReader.ReadEndElement( );//RoamingStore } } } reader.ReadEndElement( );//CipherData // // Skip optional encryption properties element. // if( reader.IsStartElement( XmlNames.XmlEnc.EncryptionProperties, XmlNames.XmlEnc.Namespace ) ) { reader.Skip(); } reader.ReadEndElement( );//EncryptedData reader.ReadEndElement( );//EncryptedStore } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
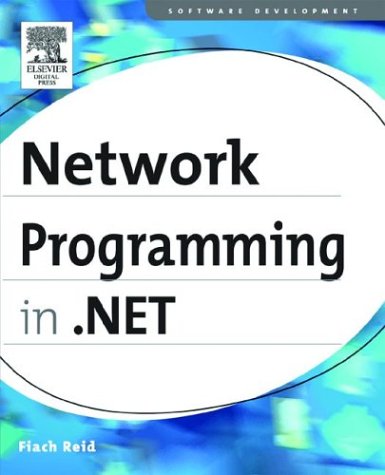
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Marshal.cs
- HtmlTernaryTree.cs
- FixedSchema.cs
- _DisconnectOverlappedAsyncResult.cs
- ViewSimplifier.cs
- _SafeNetHandles.cs
- SessionEndingEventArgs.cs
- PenContexts.cs
- DiscoveryDocumentLinksPattern.cs
- SqlDataSourceCustomCommandEditor.cs
- WebPartMinimizeVerb.cs
- WebMessageBodyStyleHelper.cs
- KnownTypeAttribute.cs
- CanonicalXml.cs
- EdgeProfileValidation.cs
- MarginsConverter.cs
- ToolboxItemWrapper.cs
- WorkflowMessageEventArgs.cs
- FrameSecurityDescriptor.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- SafeMILHandle.cs
- CapabilitiesRule.cs
- X509ClientCertificateCredentialsElement.cs
- HttpCookie.cs
- BrowserCapabilitiesCodeGenerator.cs
- GeneralTransform.cs
- SplitterDesigner.cs
- WindowsServiceCredential.cs
- DataGridViewBand.cs
- VBIdentifierNameEditor.cs
- ToolStripStatusLabel.cs
- DataServiceHostFactory.cs
- HashCodeCombiner.cs
- OutputChannel.cs
- PrintDialogDesigner.cs
- RowParagraph.cs
- TextFindEngine.cs
- EdmItemCollection.cs
- ExpressionBindingCollection.cs
- XmlAtomicValue.cs
- AttachedPropertyBrowsableAttribute.cs
- AssemblyLoader.cs
- BamlRecordReader.cs
- Condition.cs
- MonthCalendarDesigner.cs
- IxmlLineInfo.cs
- RecordManager.cs
- EarlyBoundInfo.cs
- IIS7UserPrincipal.cs
- ObjectDataSourceSelectingEventArgs.cs
- SqlSelectStatement.cs
- CheckBoxBaseAdapter.cs
- AuthorizationSection.cs
- UndoUnit.cs
- Html32TextWriter.cs
- PropertyDescriptor.cs
- HandlerBase.cs
- NumberFormatter.cs
- XsltException.cs
- DataGridViewCellCollection.cs
- CloseCryptoHandleRequest.cs
- DragCompletedEventArgs.cs
- MembershipUser.cs
- DoubleAverageAggregationOperator.cs
- Variable.cs
- MethodRental.cs
- SizeF.cs
- LayoutTable.cs
- NonClientArea.cs
- RegionInfo.cs
- ActivationServices.cs
- loginstatus.cs
- designeractionlistschangedeventargs.cs
- UInt32Storage.cs
- DocumentViewerBase.cs
- DragCompletedEventArgs.cs
- PkcsUtils.cs
- EntitySqlQueryCacheKey.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- FtpWebResponse.cs
- VisualStyleElement.cs
- DtdParser.cs
- Perspective.cs
- DataServiceQueryOfT.cs
- CreateUserErrorEventArgs.cs
- SecondaryIndexList.cs
- ValidationErrorCollection.cs
- GradientStopCollection.cs
- ISAPIWorkerRequest.cs
- ClientUrlResolverWrapper.cs
- LoginStatusDesigner.cs
- Mapping.cs
- TextProperties.cs
- ToolStripDropDownButton.cs
- PreviewKeyDownEventArgs.cs
- DataSourceView.cs
- ToolStripRenderer.cs
- BigInt.cs
- TickBar.cs
- PathNode.cs