Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / CloseCryptoHandleRequest.cs / 1 / CloseCryptoHandleRequest.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Collections; using System.Diagnostics; using System.Threading; //ManualResetEvent using System.ComponentModel; //Win32Exception using System.IO; //Stream using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; using System.Security.Principal; // // Summary: // Handles a request to close a CryptoSession. // class CloseCryptoHandleRequest : ClientRequest { // // The cryptosession id we are attaching to. // int m_cryptoSession; // // Sumamry: // Construct an CloseCryptoHandleRequest object // // Arguments: // callingProcess - The process in which the caller originated. // callingIdentity - The WindowsIdentity of the caller // rpcHandle - The handle of the native RPC request // inArgs - The stream to read input data from // outArgs - The stream to write output data to // public CloseCryptoHandleRequest( Process callingProcess, WindowsIdentity callingIdentity, IntPtr rpcHandle, Stream inArgs, Stream outArgs ) : base( callingProcess, callingIdentity, rpcHandle, inArgs, outArgs ) { IDT.TraceDebug( "Intiating an CloseCryptoHandleRequest request" ); } protected override void OnMarshalInArgs() { IDT.DebugAssert( null != InArgs, "null inargs" ); BinaryReader reader = new InfoCardBinaryReader( InArgs ); m_cryptoSession = reader.ReadInt32(); IDT.ThrowInvalidArgumentConditional( 0 == m_cryptoSession, "cryptoSession" ); } protected override void OnMarshalOutArgs() { } // // Summary // Closes a cryptoSession. // protected override void OnProcess() { CryptoSession session = CryptoSession.Find( m_cryptoSession, CallerPid, RequestorIdentity.User ); session.Dispose(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
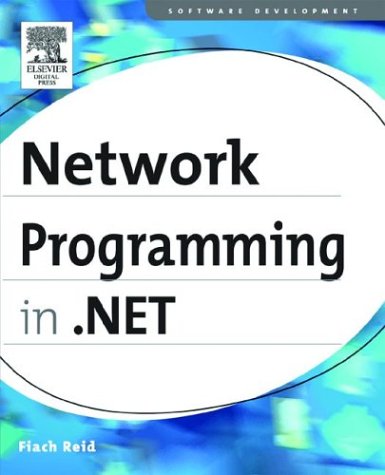
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NamedPermissionSet.cs
- WebPartExportVerb.cs
- ClientTarget.cs
- WebDescriptionAttribute.cs
- HtmlListAdapter.cs
- CollectionViewProxy.cs
- GcSettings.cs
- SmtpException.cs
- ListBoxItemWrapperAutomationPeer.cs
- PolyLineSegment.cs
- SortQueryOperator.cs
- FormViewInsertedEventArgs.cs
- XmlPreloadedResolver.cs
- Positioning.cs
- MsmqReceiveHelper.cs
- CheckBoxStandardAdapter.cs
- Part.cs
- WebPartDisplayModeCollection.cs
- ResourceProperty.cs
- UndirectedGraph.cs
- XMLUtil.cs
- GridEntryCollection.cs
- HtmlInputFile.cs
- InvalidEnumArgumentException.cs
- LogArchiveSnapshot.cs
- ApplyTemplatesAction.cs
- SafeCancelMibChangeNotify.cs
- RtfToXamlReader.cs
- NavigationExpr.cs
- LabelLiteral.cs
- XamlTemplateSerializer.cs
- BinaryFormatter.cs
- ColorConverter.cs
- SerializableAttribute.cs
- XmlQueryType.cs
- ProgressChangedEventArgs.cs
- UIElementParaClient.cs
- Aggregates.cs
- DocumentXmlWriter.cs
- StrongName.cs
- DbReferenceCollection.cs
- PageStatePersister.cs
- DBSqlParserColumnCollection.cs
- TypeCodeDomSerializer.cs
- TemplatedWizardStep.cs
- Inline.cs
- HttpBindingExtension.cs
- MultilineStringConverter.cs
- SolidBrush.cs
- BufferedReadStream.cs
- SoapClientMessage.cs
- SqlTypeConverter.cs
- ActivationServices.cs
- InternalControlCollection.cs
- smtpconnection.cs
- BitConverter.cs
- SchemaNamespaceManager.cs
- TreeNodeStyle.cs
- ToolStripSplitButton.cs
- BaseCAMarshaler.cs
- FontUnitConverter.cs
- TextChange.cs
- RegexNode.cs
- ExpressionBuilder.cs
- RsaKeyIdentifierClause.cs
- SimpleExpression.cs
- WorkflowValidationFailedException.cs
- ResourceDescriptionAttribute.cs
- DeviceSpecific.cs
- UserControl.cs
- ResourceExpression.cs
- ImageMap.cs
- OrderByQueryOptionExpression.cs
- odbcmetadatafactory.cs
- XmlSecureResolver.cs
- SchemaNames.cs
- LayeredChannelListener.cs
- Int16Storage.cs
- ComponentResourceKeyConverter.cs
- TextBoxBase.cs
- ConnectionConsumerAttribute.cs
- InstanceLockedException.cs
- TypeUtil.cs
- ObjectDataSourceView.cs
- RoleManagerModule.cs
- FaultContext.cs
- Oid.cs
- TemplatePartAttribute.cs
- ConfigXmlText.cs
- GPPOINT.cs
- Container.cs
- MissingManifestResourceException.cs
- RequestSecurityToken.cs
- CryptoProvider.cs
- ReflectPropertyDescriptor.cs
- IntSecurity.cs
- PointLight.cs
- TabControl.cs
- SelectedCellsCollection.cs
- Translator.cs