Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media / MILUtilities.cs / 1 / MILUtilities.cs
//------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Security; using System.Windows.Media; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using MS.Internal; using System.Runtime.InteropServices; using Microsoft.Internal; namespace System.Windows.Media { internal static class MILUtilities { internal static readonly D3DMATRIX D3DMATRIXIdentity = new D3DMATRIX(1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1); ////// Converts a System.Windows.Media.Matrix to a D3DMATRIX. /// /// Input Matrix to convert /// Output convered D3DMATRIX ////// Critical -- references and writes out to memory addresses. The /// caller is safe if the first pointer points to a /// constant Matrix value and the second points to a /// D3DMATRIX value. /// [SecurityCritical] internal static unsafe void ConvertToD3DMATRIX( /* in */ Matrix* matrix, /* out */ D3DMATRIX* d3dMatrix ) { *d3dMatrix = D3DMATRIXIdentity; float* pD3DMatrix = (float*)d3dMatrix; double* pMatrix = (double*)matrix; // m11 = m11 pD3DMatrix[0] = (float)pMatrix[0]; // m12 = m12 pD3DMatrix[1] = (float)pMatrix[1]; // m21 = m21 pD3DMatrix[4] = (float)pMatrix[2]; // m22 = m22 pD3DMatrix[5] = (float)pMatrix[3]; // m41 = offsetX pD3DMatrix[12] = (float)pMatrix[4]; // m42 = offsetY pD3DMatrix[13] = (float)pMatrix[5]; } ////// Converts a D3DMATRIX to a System.Windows.Media.Matrix. /// /// Input D3DMATRIX to convert /// Output converted Matrix ////// Critical -- references and writes out to memory addresses. The /// caller is safe if the first pointer points to a /// constant D3DMATRIX value and the second points to a /// Matrix value. /// [SecurityCritical] internal static unsafe void ConvertFromD3DMATRIX( /* in */ D3DMATRIX* d3dMatrix, /* out */ Matrix* matrix ) { float* pD3DMatrix = (float*)d3dMatrix; double* pMatrix = (double*)matrix; // // Convert first D3DMatrix Vector // pMatrix[0] = (double) pD3DMatrix[0]; // m11 = m11 pMatrix[1] = (double) pD3DMatrix[1]; // m12 = m12 // Assert that non-affine fields are identity or NaN // // Multiplication with an affine 2D matrix (i.e., a matrix // with only _11, _12, _21, _22, _41, & _42 set to non-identity // values) containing NaN's, can cause the NaN's to propagate to // all other fields. Thus, we allow NaN's in addition to // identity values. Debug.Assert(pD3DMatrix[2] == 0.0f || Single.IsNaN(pD3DMatrix[2])); Debug.Assert(pD3DMatrix[3] == 0.0f || Single.IsNaN(pD3DMatrix[3])); // // Convert second D3DMatrix Vector // pMatrix[2] = (double) pD3DMatrix[4]; // m21 = m21 pMatrix[3] = (double) pD3DMatrix[5]; // m22 = m22 Debug.Assert(pD3DMatrix[6] == 0.0f || Single.IsNaN(pD3DMatrix[6])); Debug.Assert(pD3DMatrix[7] == 0.0f || Single.IsNaN(pD3DMatrix[7])); // // Convert third D3DMatrix Vector // Debug.Assert(pD3DMatrix[8] == 0.0f || Single.IsNaN(pD3DMatrix[8])); Debug.Assert(pD3DMatrix[9] == 0.0f || Single.IsNaN(pD3DMatrix[9])); Debug.Assert(pD3DMatrix[10] == 1.0f || Single.IsNaN(pD3DMatrix[10])); Debug.Assert(pD3DMatrix[11] == 0.0f || Single.IsNaN(pD3DMatrix[11])); // // Convert fourth D3DMatrix Vector // pMatrix[4] = (double) pD3DMatrix[12]; // m41 = offsetX pMatrix[5] = (double) pD3DMatrix[13]; // m42 = offsetY Debug.Assert(pD3DMatrix[14] == 0.0f || Single.IsNaN(pD3DMatrix[14])); Debug.Assert(pD3DMatrix[15] == 1.0f || Single.IsNaN(pD3DMatrix[15])); *((MatrixTypes*)(pMatrix+6)) = MatrixTypes.TRANSFORM_IS_UNKNOWN; } [StructLayout(LayoutKind.Sequential)] internal struct MILRect3D { public MILRect3D(ref Rect3D rect) { X = (float)rect.X; Y = (float)rect.Y; Z = (float)rect.Z; LengthX = (float)rect.SizeX; LengthY = (float)rect.SizeY; LengthZ = (float)rect.SizeZ; } public float X; public float Y; public float Z; public float LengthX; public float LengthY; public float LengthZ; } [StructLayout(LayoutKind.Sequential)] internal struct MilRectF { public float Left; public float Top; public float Right; public float Bottom; }; ////// Critical as this code performs an elevation. /// /// It's safe because it's just doing matrix math. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(DllImport.MilCore)] private extern static /*HRESULT*/ int MIL3DCalcProjected2DBounds( ref D3DMATRIX pFullTransform3D, ref MILRect3D pboxBounds, out MilRectF prcDestRect); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(DllImport.MilCore)] private extern static /*HRESULT*/ int MIL3DCalcBrushToIdealSampleSpace( ref D3DMATRIX pDeviceTransform, ref MILRect3D mesh3DBox, ref MilRectF rcBrushSampleBounds, out D3DMATRIX matBrushSpaceToSampleSpace); [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(DllImport.MilCore, EntryPoint = "MilUtility_CopyPixelBuffer", PreserveSig = false)] internal extern static unsafe void MILCopyPixelBuffer( byte * pOutputBuffer, uint outputBufferSize, uint outputBufferStride, uint outputBufferOffsetInBits, byte * pInputBuffer, uint inputBufferSize, uint inputBufferStride, uint inputBufferOffsetInBits, uint height, uint copyWidthInBits ); ////// Critical - Calls a critical function - MIL3DCalcBrushToIdealSampleSpace. /// [SecurityCritical, SecurityTreatAsSafe] internal static void CalcMesh3DBrushAndSampleSpace( /* in */ ref Matrix3D deviceTransform, // ref for performance /* in */ ref Rect3D mesh3DBox, // ref for performance /* in */ ref Rect brushSampleBounds, // ref for performance out Matrix brushSpaceToIdealSampleSpace) { // Do a bunch of conversions to milcore types. D3DMATRIX world3D = CompositionResourceManager.Matrix3DToD3DMATRIX(deviceTransform); MILRect3D mb = new MILRect3D(ref mesh3DBox); MilRectF bsb = new MilRectF(); bsb.Left = (float)brushSampleBounds.X; bsb.Top = (float)brushSampleBounds.Y; bsb.Right = (float)(brushSampleBounds.X + brushSampleBounds.Width); bsb.Bottom = (float)(brushSampleBounds.Y + brushSampleBounds.Height); D3DMATRIX d3dbrushSpaceToIdealSampleSpace; HRESULT.Check( MIL3DCalcBrushToIdealSampleSpace( ref world3D, ref mb, ref bsb, out d3dbrushSpaceToIdealSampleSpace)); Debug.Assert( (d3dbrushSpaceToIdealSampleSpace._12 == 0) && (d3dbrushSpaceToIdealSampleSpace._13 == 0) && (d3dbrushSpaceToIdealSampleSpace._14 == 0) && (d3dbrushSpaceToIdealSampleSpace._21 == 0) && (d3dbrushSpaceToIdealSampleSpace._23 == 0) && (d3dbrushSpaceToIdealSampleSpace._24 == 0) && (d3dbrushSpaceToIdealSampleSpace._31 == 0) && (d3dbrushSpaceToIdealSampleSpace._32 == 0) && (d3dbrushSpaceToIdealSampleSpace._33 == 1.0f) && (d3dbrushSpaceToIdealSampleSpace._34 == 0) && (d3dbrushSpaceToIdealSampleSpace._41 == 0) && (d3dbrushSpaceToIdealSampleSpace._42 == 0) && (d3dbrushSpaceToIdealSampleSpace._43 == 0) && (d3dbrushSpaceToIdealSampleSpace._44 == 1.0f), "MIL3DCalcBrushToIdealSampleSpace must only return a 2D scale transform."); brushSpaceToIdealSampleSpace = Matrix.CreateScaling( d3dbrushSpaceToIdealSampleSpace._11, d3dbrushSpaceToIdealSampleSpace._22); } ////// Critical - Calls a critical function -- MilCalcProjectedBounds /// TreatAsSafe - It only does math on the given matrices. /// [SecurityCritical, SecurityTreatAsSafe] internal static Rect ProjectBounds( ref Matrix3D viewProjMatrix, ref Rect3D originalBox) { D3DMATRIX viewProjFloatMatrix = CompositionResourceManager.Matrix3DToD3DMATRIX(viewProjMatrix); MILRect3D originalBoxFloat = new MILRect3D(ref originalBox); MilRectF outRect = new MilRectF(); HRESULT.Check( MIL3DCalcProjected2DBounds( ref viewProjFloatMatrix, ref originalBoxFloat, out outRect)); if (outRect.Left == outRect.Right || outRect.Top == outRect.Bottom) { return Rect.Empty; } else { return new Rect( outRect.Left, outRect.Top, outRect.Right - outRect.Left, outRect.Bottom - outRect.Top ); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Security; using System.Windows.Media; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using MS.Internal; using System.Runtime.InteropServices; using Microsoft.Internal; namespace System.Windows.Media { internal static class MILUtilities { internal static readonly D3DMATRIX D3DMATRIXIdentity = new D3DMATRIX(1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1); ////// Converts a System.Windows.Media.Matrix to a D3DMATRIX. /// /// Input Matrix to convert /// Output convered D3DMATRIX ////// Critical -- references and writes out to memory addresses. The /// caller is safe if the first pointer points to a /// constant Matrix value and the second points to a /// D3DMATRIX value. /// [SecurityCritical] internal static unsafe void ConvertToD3DMATRIX( /* in */ Matrix* matrix, /* out */ D3DMATRIX* d3dMatrix ) { *d3dMatrix = D3DMATRIXIdentity; float* pD3DMatrix = (float*)d3dMatrix; double* pMatrix = (double*)matrix; // m11 = m11 pD3DMatrix[0] = (float)pMatrix[0]; // m12 = m12 pD3DMatrix[1] = (float)pMatrix[1]; // m21 = m21 pD3DMatrix[4] = (float)pMatrix[2]; // m22 = m22 pD3DMatrix[5] = (float)pMatrix[3]; // m41 = offsetX pD3DMatrix[12] = (float)pMatrix[4]; // m42 = offsetY pD3DMatrix[13] = (float)pMatrix[5]; } ////// Converts a D3DMATRIX to a System.Windows.Media.Matrix. /// /// Input D3DMATRIX to convert /// Output converted Matrix ////// Critical -- references and writes out to memory addresses. The /// caller is safe if the first pointer points to a /// constant D3DMATRIX value and the second points to a /// Matrix value. /// [SecurityCritical] internal static unsafe void ConvertFromD3DMATRIX( /* in */ D3DMATRIX* d3dMatrix, /* out */ Matrix* matrix ) { float* pD3DMatrix = (float*)d3dMatrix; double* pMatrix = (double*)matrix; // // Convert first D3DMatrix Vector // pMatrix[0] = (double) pD3DMatrix[0]; // m11 = m11 pMatrix[1] = (double) pD3DMatrix[1]; // m12 = m12 // Assert that non-affine fields are identity or NaN // // Multiplication with an affine 2D matrix (i.e., a matrix // with only _11, _12, _21, _22, _41, & _42 set to non-identity // values) containing NaN's, can cause the NaN's to propagate to // all other fields. Thus, we allow NaN's in addition to // identity values. Debug.Assert(pD3DMatrix[2] == 0.0f || Single.IsNaN(pD3DMatrix[2])); Debug.Assert(pD3DMatrix[3] == 0.0f || Single.IsNaN(pD3DMatrix[3])); // // Convert second D3DMatrix Vector // pMatrix[2] = (double) pD3DMatrix[4]; // m21 = m21 pMatrix[3] = (double) pD3DMatrix[5]; // m22 = m22 Debug.Assert(pD3DMatrix[6] == 0.0f || Single.IsNaN(pD3DMatrix[6])); Debug.Assert(pD3DMatrix[7] == 0.0f || Single.IsNaN(pD3DMatrix[7])); // // Convert third D3DMatrix Vector // Debug.Assert(pD3DMatrix[8] == 0.0f || Single.IsNaN(pD3DMatrix[8])); Debug.Assert(pD3DMatrix[9] == 0.0f || Single.IsNaN(pD3DMatrix[9])); Debug.Assert(pD3DMatrix[10] == 1.0f || Single.IsNaN(pD3DMatrix[10])); Debug.Assert(pD3DMatrix[11] == 0.0f || Single.IsNaN(pD3DMatrix[11])); // // Convert fourth D3DMatrix Vector // pMatrix[4] = (double) pD3DMatrix[12]; // m41 = offsetX pMatrix[5] = (double) pD3DMatrix[13]; // m42 = offsetY Debug.Assert(pD3DMatrix[14] == 0.0f || Single.IsNaN(pD3DMatrix[14])); Debug.Assert(pD3DMatrix[15] == 1.0f || Single.IsNaN(pD3DMatrix[15])); *((MatrixTypes*)(pMatrix+6)) = MatrixTypes.TRANSFORM_IS_UNKNOWN; } [StructLayout(LayoutKind.Sequential)] internal struct MILRect3D { public MILRect3D(ref Rect3D rect) { X = (float)rect.X; Y = (float)rect.Y; Z = (float)rect.Z; LengthX = (float)rect.SizeX; LengthY = (float)rect.SizeY; LengthZ = (float)rect.SizeZ; } public float X; public float Y; public float Z; public float LengthX; public float LengthY; public float LengthZ; } [StructLayout(LayoutKind.Sequential)] internal struct MilRectF { public float Left; public float Top; public float Right; public float Bottom; }; ////// Critical as this code performs an elevation. /// /// It's safe because it's just doing matrix math. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(DllImport.MilCore)] private extern static /*HRESULT*/ int MIL3DCalcProjected2DBounds( ref D3DMATRIX pFullTransform3D, ref MILRect3D pboxBounds, out MilRectF prcDestRect); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(DllImport.MilCore)] private extern static /*HRESULT*/ int MIL3DCalcBrushToIdealSampleSpace( ref D3DMATRIX pDeviceTransform, ref MILRect3D mesh3DBox, ref MilRectF rcBrushSampleBounds, out D3DMATRIX matBrushSpaceToSampleSpace); [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(DllImport.MilCore, EntryPoint = "MilUtility_CopyPixelBuffer", PreserveSig = false)] internal extern static unsafe void MILCopyPixelBuffer( byte * pOutputBuffer, uint outputBufferSize, uint outputBufferStride, uint outputBufferOffsetInBits, byte * pInputBuffer, uint inputBufferSize, uint inputBufferStride, uint inputBufferOffsetInBits, uint height, uint copyWidthInBits ); ////// Critical - Calls a critical function - MIL3DCalcBrushToIdealSampleSpace. /// [SecurityCritical, SecurityTreatAsSafe] internal static void CalcMesh3DBrushAndSampleSpace( /* in */ ref Matrix3D deviceTransform, // ref for performance /* in */ ref Rect3D mesh3DBox, // ref for performance /* in */ ref Rect brushSampleBounds, // ref for performance out Matrix brushSpaceToIdealSampleSpace) { // Do a bunch of conversions to milcore types. D3DMATRIX world3D = CompositionResourceManager.Matrix3DToD3DMATRIX(deviceTransform); MILRect3D mb = new MILRect3D(ref mesh3DBox); MilRectF bsb = new MilRectF(); bsb.Left = (float)brushSampleBounds.X; bsb.Top = (float)brushSampleBounds.Y; bsb.Right = (float)(brushSampleBounds.X + brushSampleBounds.Width); bsb.Bottom = (float)(brushSampleBounds.Y + brushSampleBounds.Height); D3DMATRIX d3dbrushSpaceToIdealSampleSpace; HRESULT.Check( MIL3DCalcBrushToIdealSampleSpace( ref world3D, ref mb, ref bsb, out d3dbrushSpaceToIdealSampleSpace)); Debug.Assert( (d3dbrushSpaceToIdealSampleSpace._12 == 0) && (d3dbrushSpaceToIdealSampleSpace._13 == 0) && (d3dbrushSpaceToIdealSampleSpace._14 == 0) && (d3dbrushSpaceToIdealSampleSpace._21 == 0) && (d3dbrushSpaceToIdealSampleSpace._23 == 0) && (d3dbrushSpaceToIdealSampleSpace._24 == 0) && (d3dbrushSpaceToIdealSampleSpace._31 == 0) && (d3dbrushSpaceToIdealSampleSpace._32 == 0) && (d3dbrushSpaceToIdealSampleSpace._33 == 1.0f) && (d3dbrushSpaceToIdealSampleSpace._34 == 0) && (d3dbrushSpaceToIdealSampleSpace._41 == 0) && (d3dbrushSpaceToIdealSampleSpace._42 == 0) && (d3dbrushSpaceToIdealSampleSpace._43 == 0) && (d3dbrushSpaceToIdealSampleSpace._44 == 1.0f), "MIL3DCalcBrushToIdealSampleSpace must only return a 2D scale transform."); brushSpaceToIdealSampleSpace = Matrix.CreateScaling( d3dbrushSpaceToIdealSampleSpace._11, d3dbrushSpaceToIdealSampleSpace._22); } ////// Critical - Calls a critical function -- MilCalcProjectedBounds /// TreatAsSafe - It only does math on the given matrices. /// [SecurityCritical, SecurityTreatAsSafe] internal static Rect ProjectBounds( ref Matrix3D viewProjMatrix, ref Rect3D originalBox) { D3DMATRIX viewProjFloatMatrix = CompositionResourceManager.Matrix3DToD3DMATRIX(viewProjMatrix); MILRect3D originalBoxFloat = new MILRect3D(ref originalBox); MilRectF outRect = new MilRectF(); HRESULT.Check( MIL3DCalcProjected2DBounds( ref viewProjFloatMatrix, ref originalBoxFloat, out outRect)); if (outRect.Left == outRect.Right || outRect.Top == outRect.Bottom) { return Rect.Empty; } else { return new Rect( outRect.Left, outRect.Top, outRect.Right - outRect.Left, outRect.Bottom - outRect.Top ); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
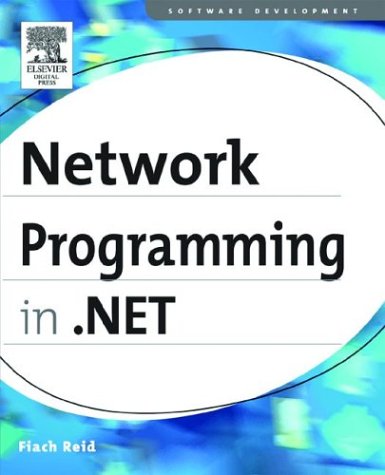
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ObjectRef.cs
- MobileControlsSection.cs
- SharedPersonalizationStateInfo.cs
- PagedDataSource.cs
- _ScatterGatherBuffers.cs
- LinkButton.cs
- ProfileServiceManager.cs
- MetadataUtilsSmi.cs
- SourceFilter.cs
- ToolStripDesignerAvailabilityAttribute.cs
- XmlSchemaIdentityConstraint.cs
- TableAdapterManagerHelper.cs
- TreeViewImageKeyConverter.cs
- UshortList2.cs
- NativeMethods.cs
- Visual3D.cs
- FontDriver.cs
- DataTableReaderListener.cs
- _CommandStream.cs
- _CacheStreams.cs
- CollectionExtensions.cs
- InputLanguageEventArgs.cs
- MsmqBindingElementBase.cs
- XmlNavigatorFilter.cs
- XsdDateTime.cs
- InternalConfigHost.cs
- UTF7Encoding.cs
- VariableQuery.cs
- LicenseException.cs
- EventDescriptor.cs
- DiagnosticTraceSource.cs
- ClientApiGenerator.cs
- JumpPath.cs
- SoapAttributeOverrides.cs
- TcpDuplicateContext.cs
- ToolBar.cs
- StorageComplexPropertyMapping.cs
- DataServices.cs
- FixedElement.cs
- EventToken.cs
- TextParaLineResult.cs
- NumericUpDownAcceleration.cs
- Set.cs
- MenuItem.cs
- TypedReference.cs
- ServiceModelDictionary.cs
- CodeMethodReturnStatement.cs
- ImageSourceTypeConverter.cs
- DbInsertCommandTree.cs
- XmlDataImplementation.cs
- DescendantOverDescendantQuery.cs
- TargetConverter.cs
- PropertiesTab.cs
- bindurihelper.cs
- CompositionDesigner.cs
- MouseGestureValueSerializer.cs
- Converter.cs
- QuotedPrintableStream.cs
- SvcMapFileLoader.cs
- datacache.cs
- OptimalBreakSession.cs
- PropertyDescriptorComparer.cs
- MarginsConverter.cs
- XpsTokenContext.cs
- FunctionImportMapping.cs
- WebExceptionStatus.cs
- ComponentSerializationService.cs
- ImageAutomationPeer.cs
- MenuItem.cs
- DragDeltaEventArgs.cs
- DbParameterCollectionHelper.cs
- BaseAsyncResult.cs
- TransactionContextManager.cs
- Accessors.cs
- Token.cs
- HitTestFilterBehavior.cs
- ParsedRoute.cs
- sqlser.cs
- IntranetCredentialPolicy.cs
- DecimalConverter.cs
- ToolTip.cs
- DashStyles.cs
- Tuple.cs
- ParserStreamGeometryContext.cs
- Pen.cs
- SerializationObjectManager.cs
- DbParameterHelper.cs
- EdmConstants.cs
- WindowProviderWrapper.cs
- XDeferredAxisSource.cs
- Expression.cs
- XmlStreamStore.cs
- NonParentingControl.cs
- DesignerMetadata.cs
- ReceiveActivity.cs
- ListViewDeletedEventArgs.cs
- BinarySecretKeyIdentifierClause.cs
- PolicyValidator.cs
- SafeTokenHandle.cs
- MatrixValueSerializer.cs