Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / MS / Internal / PtsHost / TextParaLineResult.cs / 1 / TextParaLineResult.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Access to calculated information of a line of text created // by TextParagraph. // // History: // 04/25/2003 : [....] - Moving from Avalon branch. // 06/25/2004 : [....] - Performance work. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Windows.Documents; using System.Windows; using System.Windows.Media; using MS.Internal.Documents; namespace MS.Internal.PtsHost { ////// Provides access to calculated information of a line of text created /// by TextParagraph /// internal sealed class TextParaLineResult : LineResult { //------------------------------------------------------------------- // // LineResult Methods // //------------------------------------------------------------------- #region LineResult Methods ////// Get text position corresponding to given distance from start of line /// /// /// Distance from start of line. /// internal override ITextPointer GetTextPositionFromDistance(double distance) { return _owner.GetTextPositionFromDistance(_dcp, distance); } ////// Returns true if given position is at a caret unit boundary and false if not. /// Not presently implemented. /// /// /// TextPointer representing position to check for unit boundary /// internal override bool IsAtCaretUnitBoundary(ITextPointer position) { Debug.Assert(false); return false; } ////// Return next caret unit position from the specified position in the given direction. /// Not presently implemented. /// /// /// TextPointer for the current position /// /// /// LogicalDirection in which next caret unit position is desired /// internal override ITextPointer GetNextCaretUnitPosition(ITextPointer position, LogicalDirection direction) { Debug.Assert(false); return null; } ////// Return next caret unit position from the specified position. /// Not presently implemented. /// /// /// TextPointer for the current position /// internal override ITextPointer GetBackspaceCaretUnitPosition(ITextPointer position) { Debug.Assert(false); return null; } ////// Return GlyphRun collection for the specified range of positions. /// Not presently implemented. /// /// /// ITextPointer marking start of range. /// /// /// ITextPointer marking end of range /// internal override ReadOnlyCollectionGetGlyphRuns(ITextPointer start, ITextPointer end) { Debug.Assert(false); return null; } /// /// Returns the position after last content character of the line, /// not including any line breaks. /// internal override ITextPointer GetContentEndPosition() { EnsureComplexData(); return _owner.GetTextPosition(_dcp + _cchContent, LogicalDirection.Backward); } ////// Returns the position in the line pointing to the beginning of content /// hidden by ellipses. /// internal override ITextPointer GetEllipsesPosition() { EnsureComplexData(); if (_cchEllipses != 0) { return _owner.GetTextPosition(_dcp + _cch - _cchEllipses, LogicalDirection.Forward); } return null; } ////// Retrieves the position after last content character of the line, /// not including any line breaks. /// ////// The position after last content character of the line, /// not including any line breaks. /// internal override int GetContentEndPositionCP() { EnsureComplexData(); return _dcp + _cchContent; } ////// Retrieves the position in the line pointing to the beginning of content /// hidden by ellipses. /// ////// The position in the line pointing to the beginning of content /// hidden by ellipses. /// internal override int GetEllipsesPositionCP() { EnsureComplexData(); return _dcp + _cch - _cchEllipses; } #endregion LineResult Methods //-------------------------------------------------------------------- // // LineResult Properties // //------------------------------------------------------------------- #region LineResult Properties ////// ITextPointer representing the beginning of the Line's contents. /// internal override ITextPointer StartPosition { get { if (_startPosition == null) { _startPosition = _owner.GetTextPosition(_dcp, LogicalDirection.Forward); } return _startPosition; } } ////// ITextPointer representing the end of the Line's contents. /// internal override ITextPointer EndPosition { get { if (_endPosition == null) { _endPosition = _owner.GetTextPosition(_dcp + _cch, LogicalDirection.Backward); } return _endPosition; } } ////// Character position representing the beginning of the Line's contents. /// internal override int StartPositionCP { get { return _dcp + _owner.Paragraph.ParagraphStartCharacterPosition; } } ////// Character position representing the end of the Line's contents. /// internal override int EndPositionCP { get { return _dcp + _cch + _owner.Paragraph.ParagraphStartCharacterPosition; } } ////// The bounding rectangle of the line. /// internal override Rect LayoutBox { get { return _layoutBox; } } ////// The dominant baseline of the line. /// Distance from the top of the line to the baseline. /// internal override double Baseline { get { return _baseline; } } #endregion LineResult Properties //-------------------------------------------------------------------- // // Constructors // //-------------------------------------------------------------------- #region Constructors ////// Constructor. /// /// Owner of the line. /// Index of the first character in the line. /// Number of all characters in the line. /// Rectangle of the line within a text paragraph. /// Distance from the top of the line to the baseline. internal TextParaLineResult(TextParaClient owner, int dcp, int cch, Rect layoutBox, double baseline) { _owner = owner; _dcp = dcp; _cch = cch; _layoutBox = layoutBox; _baseline = baseline; _cchContent = _cchEllipses = -1; } #endregion Constructors //------------------------------------------------------------------- // // Internal Properties // //-------------------------------------------------------------------- #region Internal Properties ////// Last character index in the line. /// internal int DcpLast { get { return _dcp + _cch; } set { _cch = value - _dcp; } } #endregion Internal Properties //------------------------------------------------------------------- // // Private Methods // //------------------------------------------------------------------- #region Private Methods ////// Ensure complex data in line /// private void EnsureComplexData() { if (_cchContent == -1) { _owner.GetLineDetails(_dcp, out _cchContent, out _cchEllipses); } } #endregion Private Methods //------------------------------------------------------------------- // // Private Fields // //-------------------------------------------------------------------- #region Private Fields ////// Owner of the line. /// private readonly TextParaClient _owner; ////// Index of the first character in the line. /// private int _dcp; ////// Number of all characters in the line. /// private int _cch; ////// Rectangle of the line within a text paragraph. /// private readonly Rect _layoutBox; ////// The dominant baseline of the line. Distance from the top of the /// line to the baseline. /// private readonly double _baseline; ////// ITextPointer representing the beginning of the Line's contents. /// private ITextPointer _startPosition; ////// ITextPointer representing the end of the Line's contents. /// private ITextPointer _endPosition; ////// Number of characters of content of the line, not including any line breaks. /// private int _cchContent; ////// Number of characters hidden by ellipses. /// private int _cchEllipses; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
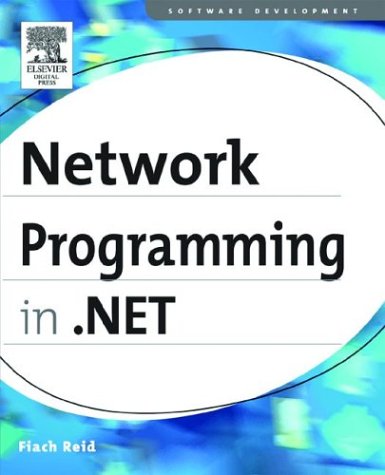
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MDIWindowDialog.cs
- ChameleonKey.cs
- TokenBasedSetEnumerator.cs
- ItemCollection.cs
- LassoSelectionBehavior.cs
- ClientScriptManagerWrapper.cs
- ADMembershipProvider.cs
- ErrorTolerantObjectWriter.cs
- SpeechUI.cs
- DBDataPermissionAttribute.cs
- CodeParameterDeclarationExpression.cs
- DataGridColumnCollection.cs
- IPHostEntry.cs
- ToolStripPanelSelectionBehavior.cs
- XmlWriter.cs
- XamlSerializerUtil.cs
- ListComponentEditor.cs
- ConfigurationManagerHelper.cs
- SQLChars.cs
- DataControlImageButton.cs
- Config.cs
- PolicyException.cs
- GenerateHelper.cs
- SafeMILHandle.cs
- ZipIOBlockManager.cs
- CaseInsensitiveHashCodeProvider.cs
- EncodingNLS.cs
- SetStoryboardSpeedRatio.cs
- RestClientProxyHandler.cs
- BamlRecordHelper.cs
- XmlSchemaSearchPattern.cs
- MsmqHostedTransportManager.cs
- CharEntityEncoderFallback.cs
- SQLDateTimeStorage.cs
- InvalidWorkflowException.cs
- PieceNameHelper.cs
- DeclarationUpdate.cs
- DataMisalignedException.cs
- AnimationTimeline.cs
- FrameworkElementAutomationPeer.cs
- Image.cs
- PenLineCapValidation.cs
- ByteStack.cs
- ContentTextAutomationPeer.cs
- ProtocolsConfigurationHandler.cs
- PassportPrincipal.cs
- BaseCodeDomTreeGenerator.cs
- OptimizedTemplateContentHelper.cs
- BaseAddressElementCollection.cs
- FormatVersion.cs
- ModuleBuilder.cs
- Table.cs
- Int64KeyFrameCollection.cs
- XmlDictionaryString.cs
- ObjectQueryProvider.cs
- GridSplitter.cs
- DesignerTransaction.cs
- RuntimeResourceSet.cs
- HMACMD5.cs
- XPathParser.cs
- ArrangedElement.cs
- ToolStripPanelCell.cs
- Internal.cs
- AppSettingsSection.cs
- FileSystemEventArgs.cs
- StylusPointCollection.cs
- InternalTypeHelper.cs
- Relationship.cs
- InfiniteIntConverter.cs
- TypeSystem.cs
- TableLayoutColumnStyleCollection.cs
- BitmapEffectDrawing.cs
- ControlCollection.cs
- XmlCharCheckingReader.cs
- DataGridViewSortCompareEventArgs.cs
- DataObjectEventArgs.cs
- AppDomainManager.cs
- InkCanvas.cs
- AppAction.cs
- DecimalAnimationUsingKeyFrames.cs
- BindingList.cs
- ColorAnimationBase.cs
- PolyLineSegment.cs
- SerialErrors.cs
- ProcessHostFactoryHelper.cs
- MD5.cs
- BinaryCommonClasses.cs
- KeyValueInternalCollection.cs
- CodeTryCatchFinallyStatement.cs
- MailDefinition.cs
- DateTimeFormatInfo.cs
- DataGrid.cs
- DoubleCollectionConverter.cs
- DataFormat.cs
- HostedElements.cs
- SafePEFileHandle.cs
- indexingfiltermarshaler.cs
- CalendarData.cs
- ConfigXmlReader.cs
- TextEditorTables.cs