Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / Animation / AnimationTimeline.cs / 1 / AnimationTimeline.cs
// AnimationTimeline.cs using System; using System.Diagnostics; using System.Windows; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Animation { ////// /// public abstract class AnimationTimeline : Timeline { ////// /// protected AnimationTimeline() : base() { } #region Dependency Properties private static void AnimationTimeline_PropertyChangedFunction(DependencyObject d, DependencyPropertyChangedEventArgs e) { ((AnimationTimeline)d).PropertyChanged(e.Property); } ////// /// public static readonly DependencyProperty IsAdditiveProperty = DependencyProperty.Register( "IsAdditive", // Property Name typeof(bool), // Property Type typeof(AnimationTimeline), // Owner Class new PropertyMetadata(false, new PropertyChangedCallback(AnimationTimeline_PropertyChangedFunction))); ////// /// public static readonly DependencyProperty IsCumulativeProperty = DependencyProperty.Register( "IsCumulative", // Property Name typeof(bool), // Property Type typeof(AnimationTimeline), // Owner Class new PropertyMetadata(false, new PropertyChangedCallback(AnimationTimeline_PropertyChangedFunction))); #endregion #region Freezable ////// Creates a copy of this AnimationTimeline. /// ///The copy. public new AnimationTimeline Clone() { return (AnimationTimeline)base.Clone(); } #endregion #region Timeline ////// /// ///protected internal override Clock AllocateClock() { return new AnimationClock(this); } /// /// Creates a new AnimationClock using this AnimationTimeline. /// ///A new AnimationClock. new public AnimationClock CreateClock() { return (AnimationClock)base.CreateClock(); } #endregion ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is not the first in a composition /// chain this value will be the value returned by the previous /// animation in the chain with an animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. If this animation is not the first in a composition /// chain this value will be the value returned by the previous /// animation in the chain with an animationClock that is not Stopped. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// public virtual object GetCurrentValue(object defaultOriginValue, object defaultDestinationValue, AnimationClock animationClock) { ReadPreamble(); return defaultDestinationValue; } ////// Provide a custom natural Duration when the Duration property is set to Automatic. /// /// /// The Clock whose natural duration is desired. /// ////// A Duration quantity representing the natural duration. Default is 1 second for animations. /// protected override Duration GetNaturalDurationCore(Clock clock) { return new TimeSpan(0, 0, 1); } ////// Returns the type of the animation. /// ///public abstract Type TargetPropertyType { get; } /// /// This property is implemented by the animation to return true if the /// animation uses the defaultDestinationValue parameter to the /// GetCurrentValue method as its destination value. Specifically, if /// Progress is equal to 1.0, will this animation return the /// default destination value as its current value. /// public virtual bool IsDestinationDefault { get { ReadPreamble(); return false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. // AnimationTimeline.cs using System; using System.Diagnostics; using System.Windows; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Animation { ////// /// public abstract class AnimationTimeline : Timeline { ////// /// protected AnimationTimeline() : base() { } #region Dependency Properties private static void AnimationTimeline_PropertyChangedFunction(DependencyObject d, DependencyPropertyChangedEventArgs e) { ((AnimationTimeline)d).PropertyChanged(e.Property); } ////// /// public static readonly DependencyProperty IsAdditiveProperty = DependencyProperty.Register( "IsAdditive", // Property Name typeof(bool), // Property Type typeof(AnimationTimeline), // Owner Class new PropertyMetadata(false, new PropertyChangedCallback(AnimationTimeline_PropertyChangedFunction))); ////// /// public static readonly DependencyProperty IsCumulativeProperty = DependencyProperty.Register( "IsCumulative", // Property Name typeof(bool), // Property Type typeof(AnimationTimeline), // Owner Class new PropertyMetadata(false, new PropertyChangedCallback(AnimationTimeline_PropertyChangedFunction))); #endregion #region Freezable ////// Creates a copy of this AnimationTimeline. /// ///The copy. public new AnimationTimeline Clone() { return (AnimationTimeline)base.Clone(); } #endregion #region Timeline ////// /// ///protected internal override Clock AllocateClock() { return new AnimationClock(this); } /// /// Creates a new AnimationClock using this AnimationTimeline. /// ///A new AnimationClock. new public AnimationClock CreateClock() { return (AnimationClock)base.CreateClock(); } #endregion ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is not the first in a composition /// chain this value will be the value returned by the previous /// animation in the chain with an animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. If this animation is not the first in a composition /// chain this value will be the value returned by the previous /// animation in the chain with an animationClock that is not Stopped. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// public virtual object GetCurrentValue(object defaultOriginValue, object defaultDestinationValue, AnimationClock animationClock) { ReadPreamble(); return defaultDestinationValue; } ////// Provide a custom natural Duration when the Duration property is set to Automatic. /// /// /// The Clock whose natural duration is desired. /// ////// A Duration quantity representing the natural duration. Default is 1 second for animations. /// protected override Duration GetNaturalDurationCore(Clock clock) { return new TimeSpan(0, 0, 1); } ////// Returns the type of the animation. /// ///public abstract Type TargetPropertyType { get; } /// /// This property is implemented by the animation to return true if the /// animation uses the defaultDestinationValue parameter to the /// GetCurrentValue method as its destination value. Specifically, if /// Progress is equal to 1.0, will this animation return the /// default destination value as its current value. /// public virtual bool IsDestinationDefault { get { ReadPreamble(); return false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
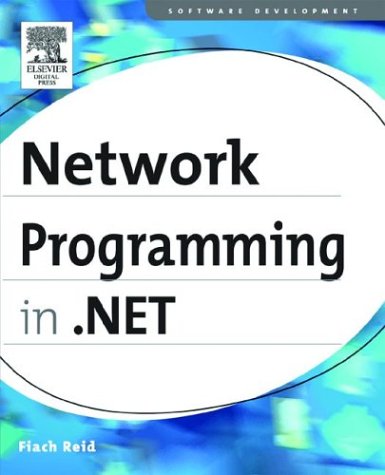
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IntegrationExceptionEventArgs.cs
- DataGridViewTextBoxEditingControl.cs
- StyleSelector.cs
- SqlFunctionAttribute.cs
- ObjectDataSourceView.cs
- FormsAuthenticationUserCollection.cs
- RSAPKCS1SignatureFormatter.cs
- EntityDataSourceDesigner.cs
- ISAPIWorkerRequest.cs
- DesignerDataSchemaClass.cs
- Package.cs
- filewebresponse.cs
- DiscoveryClientDuplexChannel.cs
- ArrayEditor.cs
- MdImport.cs
- BamlLocalizer.cs
- DragDeltaEventArgs.cs
- TextContainerChangedEventArgs.cs
- RPIdentityRequirement.cs
- LinqDataSourceInsertEventArgs.cs
- XmlSortKey.cs
- ValidationError.cs
- WebPartConnection.cs
- RegistryKey.cs
- CryptoApi.cs
- CacheAxisQuery.cs
- ProjectionCamera.cs
- ComEventsSink.cs
- ObjectAnimationBase.cs
- DocumentApplicationJournalEntry.cs
- LinkLabelLinkClickedEvent.cs
- IdentityNotMappedException.cs
- SqlCacheDependency.cs
- Rectangle.cs
- TakeOrSkipWhileQueryOperator.cs
- ViewBase.cs
- TabletDevice.cs
- SuspendDesigner.cs
- CacheChildrenQuery.cs
- FileDialog_Vista.cs
- X509SecurityTokenProvider.cs
- MediaContextNotificationWindow.cs
- SelectManyQueryOperator.cs
- Literal.cs
- SmtpException.cs
- MemberMaps.cs
- DataGridViewCheckBoxColumn.cs
- IsolatedStorageException.cs
- ConstraintManager.cs
- AssemblyCollection.cs
- PDBReader.cs
- XmlSchemaSequence.cs
- CurrentChangedEventManager.cs
- sqlcontext.cs
- NotifyParentPropertyAttribute.cs
- CommandBindingCollection.cs
- InstancePersistenceException.cs
- ApplicationHost.cs
- OutputCacheSettings.cs
- ScriptManager.cs
- FilteredXmlReader.cs
- LoginView.cs
- prompt.cs
- XmlParserContext.cs
- WizardForm.cs
- EventTrigger.cs
- GatewayDefinition.cs
- _WinHttpWebProxyDataBuilder.cs
- CrossSiteScriptingValidation.cs
- ValidationPropertyAttribute.cs
- AdornerPresentationContext.cs
- FormatException.cs
- ParameterBuilder.cs
- SqlStatistics.cs
- SqlBuilder.cs
- XPathNodeInfoAtom.cs
- TextBoxAutoCompleteSourceConverter.cs
- GeneralTransform3DGroup.cs
- TargetPerspective.cs
- DPCustomTypeDescriptor.cs
- SqlFunctionAttribute.cs
- TextEffectCollection.cs
- XmlSchemaIdentityConstraint.cs
- ArgumentsParser.cs
- XmlMemberMapping.cs
- RuleElement.cs
- OLEDB_Util.cs
- ObjectAnimationBase.cs
- Condition.cs
- XmlIgnoreAttribute.cs
- FileDialog.cs
- DisplayMemberTemplateSelector.cs
- ParagraphVisual.cs
- SQLInt64.cs
- LinkedList.cs
- AnnotationObservableCollection.cs
- FixedFlowMap.cs
- FacetValues.cs
- UIElement3D.cs
- ObjectMaterializedEventArgs.cs