Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / xsp / System / Web / Security / RoleManagerModule.cs / 1 / RoleManagerModule.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * RoleManagerModule class * * Copyright (c) 1999 Microsoft Corporation */ namespace System.Web.Security { using System.Collections; using System.Security.Principal; using System.Security.Permissions; using System.Text; using System.Threading; using System.Web; using System.Web.Configuration; using System.Web.Caching; using System.Web.Util; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class RoleManagerModule : IHttpModule { private const int MAX_COOKIE_LENGTH = 4096; private RoleManagerEventHandler _eventHandler; ///[To be supplied.] ////// [SecurityPermission(SecurityAction.Demand, Unrestricted=true)] public RoleManagerModule() { } public event RoleManagerEventHandler GetRoles { add { HttpRuntime.CheckAspNetHostingPermission(AspNetHostingPermissionLevel.Low, SR.Feature_not_supported_at_this_level); _eventHandler += value; } remove { _eventHandler -= value; } } ////// Initializes a new instance of the ////// class. /// /// public void Dispose() { } ///[To be supplied.] ////// public void Init(HttpApplication app) { // for IIS 7, skip wireup of these delegates altogether unless the // feature is enabled for this application // this avoids the initial OnEnter transition unless it's needed if (Roles.Enabled) { app.PostAuthenticateRequest += new EventHandler(this.OnEnter); app.EndRequest += new EventHandler(this.OnLeave); } } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// ///[To be supplied.] ////// private void OnEnter(Object source, EventArgs eventArgs) { if (!Roles.Enabled) { if (HttpRuntime.UseIntegratedPipeline) { ((HttpApplication)source).Context.DisableNotifications(RequestNotification.EndRequest, 0); } return; } HttpApplication app = (HttpApplication)source; HttpContext context = app.Context; if (_eventHandler != null) { RoleManagerEventArgs e = new RoleManagerEventArgs(context); _eventHandler(this, e); if (e.RolesPopulated) return; } Debug.Assert(null != context.User, "null != context.User"); if (Roles.CacheRolesInCookie) { if (context.User.Identity.IsAuthenticated && (!Roles.CookieRequireSSL || context.Request.IsSecureConnection)) { // Try to create from cookie try { HttpCookie cookie = context.Request.Cookies[Roles.CookieName]; if (cookie != null) { string cookieValue = cookie.Value; // Ignore cookies that are too long if (cookieValue != null && cookieValue.Length > MAX_COOKIE_LENGTH) { Roles.DeleteCookie(); } else { if (!String.IsNullOrEmpty(Roles.CookiePath) && Roles.CookiePath != "/") { cookie.Path = Roles.CookiePath; } cookie.Domain = Roles.Domain; context.User = new RolePrincipal(context.User.Identity, cookieValue); } } } catch { } // skip exceptions } else { if (context.Request.Cookies[Roles.CookieName] != null) Roles.DeleteCookie(); // if we're not using cookie caching, we don't need the EndRequest // event and can suppress it if (HttpRuntime.UseIntegratedPipeline) { context.DisableNotifications(RequestNotification.EndRequest, 0); } } } if (!(context.User is RolePrincipal)) context.User = new RolePrincipal(context.User.Identity); Thread.CurrentPrincipal = context.User; } private void OnLeave(Object source, EventArgs eventArgs) { HttpApplication app; HttpContext context; app = (HttpApplication)source; context = app.Context; if (!Roles.Enabled || !Roles.CacheRolesInCookie || context.Response.HeadersWritten) return; if (context.User == null || !(context.User is RolePrincipal) || !context.User.Identity.IsAuthenticated) return; if (Roles.CookieRequireSSL && !context.Request.IsSecureConnection) { // if cookie is sent, then clear it if (context.Request.Cookies[Roles.CookieName] != null) Roles.DeleteCookie(); return; } RolePrincipal rp = (RolePrincipal) context.User; if (rp.CachedListChanged && context.Request.Browser.Cookies) { string s = rp.ToEncryptedTicket(); if (string.IsNullOrEmpty(s) || s.Length > MAX_COOKIE_LENGTH) { Roles.DeleteCookie(); } else { HttpCookie cookie = new HttpCookie(Roles.CookieName, s); cookie.HttpOnly = true; cookie.Path = Roles.CookiePath; cookie.Domain = Roles.Domain; if (Roles.CreatePersistentCookie) cookie.Expires = rp.ExpireDate; cookie.Secure = Roles.CookieRequireSSL; context.Response.Cookies.Add(cookie); } } } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * RoleManagerModule class * * Copyright (c) 1999 Microsoft Corporation */ namespace System.Web.Security { using System.Collections; using System.Security.Principal; using System.Security.Permissions; using System.Text; using System.Threading; using System.Web; using System.Web.Configuration; using System.Web.Caching; using System.Web.Util; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class RoleManagerModule : IHttpModule { private const int MAX_COOKIE_LENGTH = 4096; private RoleManagerEventHandler _eventHandler; ///[To be supplied.] ////// [SecurityPermission(SecurityAction.Demand, Unrestricted=true)] public RoleManagerModule() { } public event RoleManagerEventHandler GetRoles { add { HttpRuntime.CheckAspNetHostingPermission(AspNetHostingPermissionLevel.Low, SR.Feature_not_supported_at_this_level); _eventHandler += value; } remove { _eventHandler -= value; } } ////// Initializes a new instance of the ////// class. /// /// public void Dispose() { } ///[To be supplied.] ////// public void Init(HttpApplication app) { // for IIS 7, skip wireup of these delegates altogether unless the // feature is enabled for this application // this avoids the initial OnEnter transition unless it's needed if (Roles.Enabled) { app.PostAuthenticateRequest += new EventHandler(this.OnEnter); app.EndRequest += new EventHandler(this.OnLeave); } } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// ///[To be supplied.] ////// private void OnEnter(Object source, EventArgs eventArgs) { if (!Roles.Enabled) { if (HttpRuntime.UseIntegratedPipeline) { ((HttpApplication)source).Context.DisableNotifications(RequestNotification.EndRequest, 0); } return; } HttpApplication app = (HttpApplication)source; HttpContext context = app.Context; if (_eventHandler != null) { RoleManagerEventArgs e = new RoleManagerEventArgs(context); _eventHandler(this, e); if (e.RolesPopulated) return; } Debug.Assert(null != context.User, "null != context.User"); if (Roles.CacheRolesInCookie) { if (context.User.Identity.IsAuthenticated && (!Roles.CookieRequireSSL || context.Request.IsSecureConnection)) { // Try to create from cookie try { HttpCookie cookie = context.Request.Cookies[Roles.CookieName]; if (cookie != null) { string cookieValue = cookie.Value; // Ignore cookies that are too long if (cookieValue != null && cookieValue.Length > MAX_COOKIE_LENGTH) { Roles.DeleteCookie(); } else { if (!String.IsNullOrEmpty(Roles.CookiePath) && Roles.CookiePath != "/") { cookie.Path = Roles.CookiePath; } cookie.Domain = Roles.Domain; context.User = new RolePrincipal(context.User.Identity, cookieValue); } } } catch { } // skip exceptions } else { if (context.Request.Cookies[Roles.CookieName] != null) Roles.DeleteCookie(); // if we're not using cookie caching, we don't need the EndRequest // event and can suppress it if (HttpRuntime.UseIntegratedPipeline) { context.DisableNotifications(RequestNotification.EndRequest, 0); } } } if (!(context.User is RolePrincipal)) context.User = new RolePrincipal(context.User.Identity); Thread.CurrentPrincipal = context.User; } private void OnLeave(Object source, EventArgs eventArgs) { HttpApplication app; HttpContext context; app = (HttpApplication)source; context = app.Context; if (!Roles.Enabled || !Roles.CacheRolesInCookie || context.Response.HeadersWritten) return; if (context.User == null || !(context.User is RolePrincipal) || !context.User.Identity.IsAuthenticated) return; if (Roles.CookieRequireSSL && !context.Request.IsSecureConnection) { // if cookie is sent, then clear it if (context.Request.Cookies[Roles.CookieName] != null) Roles.DeleteCookie(); return; } RolePrincipal rp = (RolePrincipal) context.User; if (rp.CachedListChanged && context.Request.Browser.Cookies) { string s = rp.ToEncryptedTicket(); if (string.IsNullOrEmpty(s) || s.Length > MAX_COOKIE_LENGTH) { Roles.DeleteCookie(); } else { HttpCookie cookie = new HttpCookie(Roles.CookieName, s); cookie.HttpOnly = true; cookie.Path = Roles.CookiePath; cookie.Domain = Roles.Domain; if (Roles.CreatePersistentCookie) cookie.Expires = rp.ExpireDate; cookie.Secure = Roles.CookieRequireSSL; context.Response.Cookies.Add(cookie); } } } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
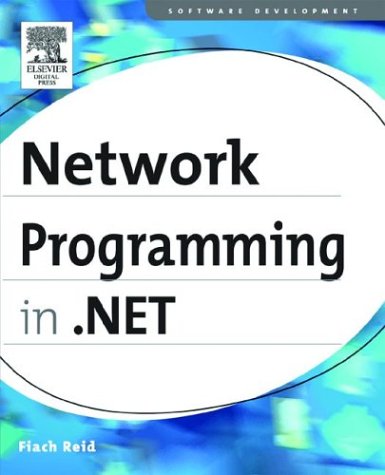
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DoubleLinkListEnumerator.cs
- ItemsControlAutomationPeer.cs
- ToolBarButton.cs
- PtsCache.cs
- DataGridParentRows.cs
- AstNode.cs
- TextBox.cs
- Inflater.cs
- WindowPatternIdentifiers.cs
- SimpleExpression.cs
- ToolStripButton.cs
- MimeTextImporter.cs
- SoapClientProtocol.cs
- IndentedTextWriter.cs
- ArithmeticException.cs
- WebPartCatalogAddVerb.cs
- PeerNameResolver.cs
- Quaternion.cs
- TextStore.cs
- CustomTypeDescriptor.cs
- ClickablePoint.cs
- CompositeDuplexBindingElementImporter.cs
- TextTreeText.cs
- CheckedListBox.cs
- PartialTrustVisibleAssembliesSection.cs
- HtmlTable.cs
- BitmapSource.cs
- NamedElement.cs
- ListViewGroupConverter.cs
- StringCollection.cs
- DateTimeSerializationSection.cs
- MailSettingsSection.cs
- MonthChangedEventArgs.cs
- CodeChecksumPragma.cs
- PropertyEmitter.cs
- HttpWriter.cs
- FontSource.cs
- TreeViewHitTestInfo.cs
- SecurityUtils.cs
- Pen.cs
- DetailsViewInsertEventArgs.cs
- Cursors.cs
- VectorCollectionConverter.cs
- DataGridViewBindingCompleteEventArgs.cs
- ActivityCodeGenerator.cs
- ReferencedType.cs
- ApplicationDirectory.cs
- CroppedBitmap.cs
- TitleStyle.cs
- StrongName.cs
- TableStyle.cs
- StylusShape.cs
- XmlSchemaSubstitutionGroup.cs
- StubHelpers.cs
- ProxyAttribute.cs
- ProtocolsSection.cs
- NullableIntAverageAggregationOperator.cs
- RootBrowserWindowAutomationPeer.cs
- UrlPath.cs
- GridItemProviderWrapper.cs
- CapabilitiesSection.cs
- CompositeCollectionView.cs
- TrustLevel.cs
- DataGridViewColumnCollection.cs
- EventProxy.cs
- TextEffect.cs
- GifBitmapEncoder.cs
- SingleSelectRootGridEntry.cs
- XamlWrappingReader.cs
- FieldBuilder.cs
- EntityChangedParams.cs
- X509ThumbprintKeyIdentifierClause.cs
- PixelFormatConverter.cs
- __TransparentProxy.cs
- MemberMemberBinding.cs
- ColumnTypeConverter.cs
- PathSegment.cs
- XamlFigureLengthSerializer.cs
- MemberRelationshipService.cs
- SignedPkcs7.cs
- DbSetClause.cs
- HtmlTableCell.cs
- RootProfilePropertySettingsCollection.cs
- AddToCollection.cs
- DataGridViewCellLinkedList.cs
- MulticastNotSupportedException.cs
- Configuration.cs
- StorageConditionPropertyMapping.cs
- ReflectionServiceProvider.cs
- SchemaInfo.cs
- DtrList.cs
- SoapSchemaImporter.cs
- ExpressionQuoter.cs
- VisualTreeHelper.cs
- HttpApplicationFactory.cs
- ExpressionBuilderCollection.cs
- MetadataImporterQuotas.cs
- TransactionScope.cs
- NotifyParentPropertyAttribute.cs
- DefaultWorkflowLoaderService.cs