Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CompMod / System / ComponentModel / Design / Serialization / MemberRelationshipService.cs / 1305376 / MemberRelationshipService.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel.Design.Serialization { using System.Collections.Generic; using System.Diagnostics.CodeAnalysis; using System.Security.Permissions; ////// A member relationship service is used by a serializer to announce that one /// property is related to a property on another object. Consider a code /// based serialization scheme where code is of the following form: /// /// object1.Property1 = object2.Property2 /// /// Upon interpretation of this code, Property1 on object1 will be /// set to the return value of object2.Property2. But the relationship /// between these two objects is lost. Serialization schemes that /// wish to maintain this relationship may install a MemberRelationshipService /// into the serialization manager. When an object is deserialized /// this serivce will be notified of these relationships. It is up to the service /// to act on these notifications if it wishes. During serialization, the /// service is also consulted. If a relationship exists the same /// relationship is maintained by the serializer. /// [HostProtection(SharedState = true)] public abstract class MemberRelationshipService { private Dictionary_relationships = new Dictionary (); /// /// Returns the the current relationship associated with the source, or MemberRelationship.Empty if /// there is no relationship. Also sets a relationship between two objects. Empty /// can also be passed as the property value, in which case the relationship will /// be cleared. /// [SuppressMessage("Microsoft.Design", "CA1043:UseIntegralOrStringArgumentForIndexers")] public MemberRelationship this[MemberRelationship source] { get { if (source.Owner == null) throw new ArgumentNullException("Owner"); if (source.Member== null) throw new ArgumentNullException("Member"); return GetRelationship(source); } set { if (source.Owner == null) throw new ArgumentNullException("Owner"); if (source.Member == null) throw new ArgumentNullException("Member"); SetRelationship(source, value); } } ////// Returns the the current relationship associated with the source, or null if /// there is no relationship. Also sets a relationship between two objects. Null /// can be passed as the property value, in which case the relationship will /// be cleared. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1023:IndexersShouldNotBeMultidimensional")] public MemberRelationship this[object sourceOwner, MemberDescriptor sourceMember] { get { if (sourceOwner == null) throw new ArgumentNullException("sourceOwner"); if (sourceMember == null) throw new ArgumentNullException("sourceMember"); return GetRelationship(new MemberRelationship(sourceOwner, sourceMember)); } set { if (sourceOwner == null) throw new ArgumentNullException("sourceOwner"); if (sourceMember == null) throw new ArgumentNullException("sourceMember"); SetRelationship(new MemberRelationship(sourceOwner, sourceMember), value); } } ////// This is the implementation API for returning relationships. The default implementation stores the /// relationship in a table. Relationships are stored weakly, so they do not keep an object alive. /// protected virtual MemberRelationship GetRelationship(MemberRelationship source) { RelationshipEntry retVal; if (_relationships != null && _relationships.TryGetValue(new RelationshipEntry(source), out retVal) && retVal.Owner.IsAlive) { return new MemberRelationship(retVal.Owner.Target, retVal.Member); } return MemberRelationship.Empty; } ////// This is the implementation API for returning relationships. The default implementation stores the /// relationship in a table. Relationships are stored weakly, so they do not keep an object alive. Empty can be /// passed in for relationship to remove the relationship. /// protected virtual void SetRelationship(MemberRelationship source, MemberRelationship relationship) { if (!relationship.IsEmpty && !SupportsRelationship(source, relationship)) { string sourceName = TypeDescriptor.GetComponentName(source.Owner); string relName = TypeDescriptor.GetComponentName(relationship.Owner); if (sourceName == null) { sourceName = source.Owner.ToString(); } if (relName == null) { relName = relationship.Owner.ToString(); } throw new ArgumentException(SR.GetString(SR.MemberRelationshipService_RelationshipNotSupported, sourceName, source.Member.Name, relName, relationship.Member.Name)); } if (_relationships == null) { _relationships = new Dictionary(); } _relationships[new RelationshipEntry(source)] = new RelationshipEntry(relationship); } /// /// Returns true if the provided relatinoship is supported. /// public abstract bool SupportsRelationship(MemberRelationship source, MemberRelationship relationship); ////// Used as storage in our relationship table /// private struct RelationshipEntry { internal WeakReference Owner; internal MemberDescriptor Member; private int hashCode; internal RelationshipEntry(MemberRelationship rel) { Owner = new WeakReference(rel.Owner); Member = rel.Member; hashCode = rel.Owner == null ? 0 : rel.Owner.GetHashCode(); } public override bool Equals(object o) { if (o is RelationshipEntry) { RelationshipEntry e = (RelationshipEntry)o; return this == e; } return false; } public static bool operator==(RelationshipEntry re1, RelationshipEntry re2){ object owner1 = (re1.Owner.IsAlive ? re1.Owner.Target : null); object owner2 = (re2.Owner.IsAlive ? re2.Owner.Target : null); return owner1 == owner2 && re1.Member.Equals(re2.Member); } public static bool operator!=(RelationshipEntry re1, RelationshipEntry re2){ return !(re1 == re2); } public override int GetHashCode() { return hashCode; } } } ////// This class represents a single relationship between an object and a member. /// public struct MemberRelationship { private object _owner; private MemberDescriptor _member; public static readonly MemberRelationship Empty = new MemberRelationship(); ////// Creates a new member relationship. /// public MemberRelationship(object owner, MemberDescriptor member) { if (owner == null) throw new ArgumentNullException("owner"); if (member == null) throw new ArgumentNullException("member"); _owner = owner; _member = member; } ////// Returns true if this relationship is empty. /// public bool IsEmpty { get { return _owner == null; } } ////// The member in this relationship. /// public MemberDescriptor Member { get { return _member; } } ////// The object owning the member. /// public object Owner { get { return _owner; } } ////// Infrastructure support to make this a first class struct /// public override bool Equals(object obj) { if (!(obj is MemberRelationship)) return false; MemberRelationship rel = (MemberRelationship)obj; return rel.Owner == Owner && rel.Member == Member; } ////// Infrastructure support to make this a first class struct /// public override int GetHashCode() { if (_owner == null) return base.GetHashCode(); return _owner.GetHashCode() ^ _member.GetHashCode(); } ////// Infrastructure support to make this a first class struct /// public static bool operator ==(MemberRelationship left, MemberRelationship right) { return left.Owner == right.Owner && left.Member == right.Member; } ////// Infrastructure support to make this a first class struct /// public static bool operator !=(MemberRelationship left, MemberRelationship right) { return !(left == right); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel.Design.Serialization { using System.Collections.Generic; using System.Diagnostics.CodeAnalysis; using System.Security.Permissions; ////// A member relationship service is used by a serializer to announce that one /// property is related to a property on another object. Consider a code /// based serialization scheme where code is of the following form: /// /// object1.Property1 = object2.Property2 /// /// Upon interpretation of this code, Property1 on object1 will be /// set to the return value of object2.Property2. But the relationship /// between these two objects is lost. Serialization schemes that /// wish to maintain this relationship may install a MemberRelationshipService /// into the serialization manager. When an object is deserialized /// this serivce will be notified of these relationships. It is up to the service /// to act on these notifications if it wishes. During serialization, the /// service is also consulted. If a relationship exists the same /// relationship is maintained by the serializer. /// [HostProtection(SharedState = true)] public abstract class MemberRelationshipService { private Dictionary_relationships = new Dictionary (); /// /// Returns the the current relationship associated with the source, or MemberRelationship.Empty if /// there is no relationship. Also sets a relationship between two objects. Empty /// can also be passed as the property value, in which case the relationship will /// be cleared. /// [SuppressMessage("Microsoft.Design", "CA1043:UseIntegralOrStringArgumentForIndexers")] public MemberRelationship this[MemberRelationship source] { get { if (source.Owner == null) throw new ArgumentNullException("Owner"); if (source.Member== null) throw new ArgumentNullException("Member"); return GetRelationship(source); } set { if (source.Owner == null) throw new ArgumentNullException("Owner"); if (source.Member == null) throw new ArgumentNullException("Member"); SetRelationship(source, value); } } ////// Returns the the current relationship associated with the source, or null if /// there is no relationship. Also sets a relationship between two objects. Null /// can be passed as the property value, in which case the relationship will /// be cleared. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1023:IndexersShouldNotBeMultidimensional")] public MemberRelationship this[object sourceOwner, MemberDescriptor sourceMember] { get { if (sourceOwner == null) throw new ArgumentNullException("sourceOwner"); if (sourceMember == null) throw new ArgumentNullException("sourceMember"); return GetRelationship(new MemberRelationship(sourceOwner, sourceMember)); } set { if (sourceOwner == null) throw new ArgumentNullException("sourceOwner"); if (sourceMember == null) throw new ArgumentNullException("sourceMember"); SetRelationship(new MemberRelationship(sourceOwner, sourceMember), value); } } ////// This is the implementation API for returning relationships. The default implementation stores the /// relationship in a table. Relationships are stored weakly, so they do not keep an object alive. /// protected virtual MemberRelationship GetRelationship(MemberRelationship source) { RelationshipEntry retVal; if (_relationships != null && _relationships.TryGetValue(new RelationshipEntry(source), out retVal) && retVal.Owner.IsAlive) { return new MemberRelationship(retVal.Owner.Target, retVal.Member); } return MemberRelationship.Empty; } ////// This is the implementation API for returning relationships. The default implementation stores the /// relationship in a table. Relationships are stored weakly, so they do not keep an object alive. Empty can be /// passed in for relationship to remove the relationship. /// protected virtual void SetRelationship(MemberRelationship source, MemberRelationship relationship) { if (!relationship.IsEmpty && !SupportsRelationship(source, relationship)) { string sourceName = TypeDescriptor.GetComponentName(source.Owner); string relName = TypeDescriptor.GetComponentName(relationship.Owner); if (sourceName == null) { sourceName = source.Owner.ToString(); } if (relName == null) { relName = relationship.Owner.ToString(); } throw new ArgumentException(SR.GetString(SR.MemberRelationshipService_RelationshipNotSupported, sourceName, source.Member.Name, relName, relationship.Member.Name)); } if (_relationships == null) { _relationships = new Dictionary(); } _relationships[new RelationshipEntry(source)] = new RelationshipEntry(relationship); } /// /// Returns true if the provided relatinoship is supported. /// public abstract bool SupportsRelationship(MemberRelationship source, MemberRelationship relationship); ////// Used as storage in our relationship table /// private struct RelationshipEntry { internal WeakReference Owner; internal MemberDescriptor Member; private int hashCode; internal RelationshipEntry(MemberRelationship rel) { Owner = new WeakReference(rel.Owner); Member = rel.Member; hashCode = rel.Owner == null ? 0 : rel.Owner.GetHashCode(); } public override bool Equals(object o) { if (o is RelationshipEntry) { RelationshipEntry e = (RelationshipEntry)o; return this == e; } return false; } public static bool operator==(RelationshipEntry re1, RelationshipEntry re2){ object owner1 = (re1.Owner.IsAlive ? re1.Owner.Target : null); object owner2 = (re2.Owner.IsAlive ? re2.Owner.Target : null); return owner1 == owner2 && re1.Member.Equals(re2.Member); } public static bool operator!=(RelationshipEntry re1, RelationshipEntry re2){ return !(re1 == re2); } public override int GetHashCode() { return hashCode; } } } ////// This class represents a single relationship between an object and a member. /// public struct MemberRelationship { private object _owner; private MemberDescriptor _member; public static readonly MemberRelationship Empty = new MemberRelationship(); ////// Creates a new member relationship. /// public MemberRelationship(object owner, MemberDescriptor member) { if (owner == null) throw new ArgumentNullException("owner"); if (member == null) throw new ArgumentNullException("member"); _owner = owner; _member = member; } ////// Returns true if this relationship is empty. /// public bool IsEmpty { get { return _owner == null; } } ////// The member in this relationship. /// public MemberDescriptor Member { get { return _member; } } ////// The object owning the member. /// public object Owner { get { return _owner; } } ////// Infrastructure support to make this a first class struct /// public override bool Equals(object obj) { if (!(obj is MemberRelationship)) return false; MemberRelationship rel = (MemberRelationship)obj; return rel.Owner == Owner && rel.Member == Member; } ////// Infrastructure support to make this a first class struct /// public override int GetHashCode() { if (_owner == null) return base.GetHashCode(); return _owner.GetHashCode() ^ _member.GetHashCode(); } ////// Infrastructure support to make this a first class struct /// public static bool operator ==(MemberRelationship left, MemberRelationship right) { return left.Owner == right.Owner && left.Member == right.Member; } ////// Infrastructure support to make this a first class struct /// public static bool operator !=(MemberRelationship left, MemberRelationship right) { return !(left == right); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
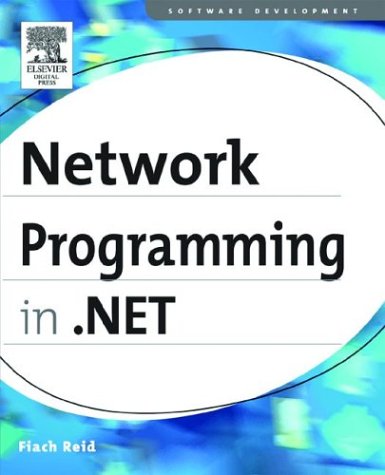
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DesignTimeResourceProviderFactoryAttribute.cs
- SamlAction.cs
- Graphics.cs
- HttpCacheVaryByContentEncodings.cs
- ObjectDataSourceMethodEventArgs.cs
- DataTemplateSelector.cs
- InfoCardRSAPKCS1SignatureDeformatter.cs
- AccessedThroughPropertyAttribute.cs
- WindowsRegion.cs
- Trace.cs
- ProtocolViolationException.cs
- RenamedEventArgs.cs
- RegistrySecurity.cs
- NameSpaceExtractor.cs
- CustomValidator.cs
- ExpressionVisitorHelpers.cs
- OutputCacheProfile.cs
- ISSmlParser.cs
- TimeSpanMinutesConverter.cs
- TabPanel.cs
- SecuritySessionSecurityTokenAuthenticator.cs
- OutputCacheSettingsSection.cs
- ColorConverter.cs
- ProcessInfo.cs
- FamilyCollection.cs
- StructuredTypeInfo.cs
- GlobalizationAssembly.cs
- DefaultSerializationProviderAttribute.cs
- DirectoryObjectSecurity.cs
- RightNameExpirationInfoPair.cs
- ZipIOModeEnforcingStream.cs
- SqlBooleanMismatchVisitor.cs
- CustomCredentialPolicy.cs
- Item.cs
- DbConnectionPoolGroup.cs
- Misc.cs
- TargetControlTypeCache.cs
- ScrollProperties.cs
- HttpHandlerAction.cs
- ConfigurationErrorsException.cs
- DataSourceHelper.cs
- Selector.cs
- DebuggerAttributes.cs
- PromptBuilder.cs
- TaskScheduler.cs
- IdentityNotMappedException.cs
- HeaderedItemsControl.cs
- Size.cs
- ServiceModelSectionGroup.cs
- MessageOperationFormatter.cs
- PlanCompiler.cs
- ModifierKeysConverter.cs
- ReferenceEqualityComparer.cs
- DtdParser.cs
- WebFaultClientMessageInspector.cs
- SqlTriggerContext.cs
- RegistryKey.cs
- AspNetHostingPermission.cs
- UInt32.cs
- RegexWorker.cs
- Clock.cs
- ManualWorkflowSchedulerService.cs
- DataSysAttribute.cs
- DataControlImageButton.cs
- CacheForPrimitiveTypes.cs
- ContextStack.cs
- DataControlImageButton.cs
- SynchronizedInputPattern.cs
- GeometryGroup.cs
- TypeContext.cs
- BuilderPropertyEntry.cs
- ResourceSet.cs
- SerializationInfo.cs
- FragmentNavigationEventArgs.cs
- SafeSecurityHandles.cs
- Constants.cs
- WindowsGrip.cs
- TabRenderer.cs
- ToolZone.cs
- ByteConverter.cs
- _SecureChannel.cs
- BaseResourcesBuildProvider.cs
- UnsafeNativeMethods.cs
- FormsAuthenticationUserCollection.cs
- SharedPerformanceCounter.cs
- ConvertEvent.cs
- ChangeToolStripParentVerb.cs
- RayMeshGeometry3DHitTestResult.cs
- ContourSegment.cs
- DirectionalLight.cs
- MenuItemStyleCollection.cs
- DESCryptoServiceProvider.cs
- ColumnTypeConverter.cs
- FastPropertyAccessor.cs
- ActivityExecutor.cs
- MenuItemAutomationPeer.cs
- ChildTable.cs
- ArraySegment.cs
- DbProviderServices.cs
- AnnotationComponentManager.cs