Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / UI / WebControls / ChildTable.cs / 3 / ChildTable.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.ComponentModel; using System.Web.UI; using System.Web.Util; [ ToolboxItem(false), SupportsEventValidation, ] ////// /// Used by composite controls that are based on a table, that only render /// their contents. /// Used to render out an ID attribute representing the parent composite control /// if an ID is not actually set on this table. /// internal class ChildTable : Table { private int _parentLevel; private string _parentID; private bool _parentIDSet; ///internal ChildTable() : this(1) { } /// internal ChildTable(int parentLevel) { Debug.Assert(parentLevel >= 1); _parentLevel = parentLevel; _parentIDSet = false; } internal ChildTable(string parentID) { _parentID = parentID; _parentIDSet = true; } /// protected override void AddAttributesToRender(HtmlTextWriter writer) { base.AddAttributesToRender(writer); string parentID = _parentID; if (!_parentIDSet) { parentID = GetParentID(); } if (parentID != null) { writer.AddAttribute(HtmlTextWriterAttribute.Id, parentID); } } /// /// Gets the ClientID of the parent whose ID is supposed to be used in the rendering. /// private string GetParentID() { if (ID != null) { return null; } Control parent = this; for (int i = 0; i < _parentLevel; i++) { parent = parent.Parent; if (parent == null) { break; } } Debug.Assert(parent != null); if (parent != null) { string id = parent.ID; if (!String.IsNullOrEmpty(id)) { return parent.ClientID; } } return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.ComponentModel; using System.Web.UI; using System.Web.Util; [ ToolboxItem(false), SupportsEventValidation, ] ////// /// Used by composite controls that are based on a table, that only render /// their contents. /// Used to render out an ID attribute representing the parent composite control /// if an ID is not actually set on this table. /// internal class ChildTable : Table { private int _parentLevel; private string _parentID; private bool _parentIDSet; ///internal ChildTable() : this(1) { } /// internal ChildTable(int parentLevel) { Debug.Assert(parentLevel >= 1); _parentLevel = parentLevel; _parentIDSet = false; } internal ChildTable(string parentID) { _parentID = parentID; _parentIDSet = true; } /// protected override void AddAttributesToRender(HtmlTextWriter writer) { base.AddAttributesToRender(writer); string parentID = _parentID; if (!_parentIDSet) { parentID = GetParentID(); } if (parentID != null) { writer.AddAttribute(HtmlTextWriterAttribute.Id, parentID); } } /// /// Gets the ClientID of the parent whose ID is supposed to be used in the rendering. /// private string GetParentID() { if (ID != null) { return null; } Control parent = this; for (int i = 0; i < _parentLevel; i++) { parent = parent.Parent; if (parent == null) { break; } } Debug.Assert(parent != null); if (parent != null) { string id = parent.ID; if (!String.IsNullOrEmpty(id)) { return parent.ClientID; } } return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
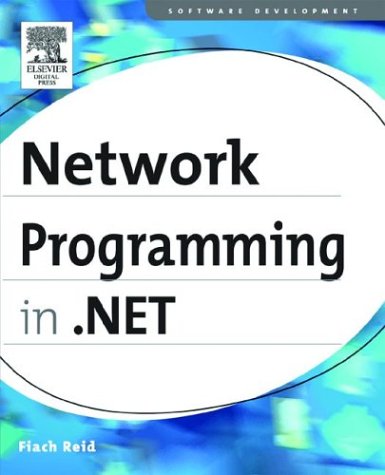
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EffectiveValueEntry.cs
- ArglessEventHandlerProxy.cs
- StrokeNodeOperations.cs
- ConnectorEditor.cs
- AttachedAnnotation.cs
- ForeignKeyConstraint.cs
- SortedDictionary.cs
- RichTextBoxAutomationPeer.cs
- DispatcherHookEventArgs.cs
- IndependentlyAnimatedPropertyMetadata.cs
- DesignerActionKeyboardBehavior.cs
- Encoder.cs
- RangeContentEnumerator.cs
- TimeStampChecker.cs
- StreamGeometryContext.cs
- WindowsFormsSynchronizationContext.cs
- UserControlCodeDomTreeGenerator.cs
- JsonWriterDelegator.cs
- ActiveDocumentEvent.cs
- EventLogRecord.cs
- DbConnectionStringBuilder.cs
- LoginDesignerUtil.cs
- TextRangeBase.cs
- DirtyTextRange.cs
- UnsettableComboBox.cs
- ButtonField.cs
- XmlValidatingReaderImpl.cs
- _SslState.cs
- DocumentPaginator.cs
- DataGridCellEditEndingEventArgs.cs
- ToolStripLabel.cs
- Compiler.cs
- XmlElement.cs
- OdbcConnectionOpen.cs
- StringOutput.cs
- LeaseManager.cs
- UnaryNode.cs
- Size3D.cs
- EdmType.cs
- CLRBindingWorker.cs
- PermissionListSet.cs
- ColumnHeaderConverter.cs
- ServiceBuildProvider.cs
- AutoResetEvent.cs
- PasswordPropertyTextAttribute.cs
- panel.cs
- LinqDataView.cs
- DockPanel.cs
- RSAPKCS1SignatureFormatter.cs
- MemberJoinTreeNode.cs
- CrossAppDomainChannel.cs
- DefaultTraceListener.cs
- Profiler.cs
- FileUpload.cs
- DataPointer.cs
- JumpTask.cs
- XmlQueryOutput.cs
- QilStrConcat.cs
- BamlCollectionHolder.cs
- InheritanceContextHelper.cs
- Substitution.cs
- XmlEntity.cs
- WebDisplayNameAttribute.cs
- DataKey.cs
- VirtualPathProvider.cs
- LineInfo.cs
- ExpressionNode.cs
- InputScopeManager.cs
- CFStream.cs
- HttpPostedFile.cs
- EncodingFallbackAwareXmlTextWriter.cs
- HttpRuntimeSection.cs
- TCPClient.cs
- Rotation3DAnimation.cs
- ReachIDocumentPaginatorSerializerAsync.cs
- BaseTemplateBuildProvider.cs
- MSAAEventDispatcher.cs
- SchemaInfo.cs
- X509ChainElement.cs
- PowerStatus.cs
- Shape.cs
- HtmlFormWrapper.cs
- XPathQueryGenerator.cs
- DeviceSpecificDialogCachedState.cs
- XmlSortKey.cs
- EmptyReadOnlyDictionaryInternal.cs
- BrowserDefinition.cs
- XmlWriter.cs
- TreeNodeMouseHoverEvent.cs
- SoapTypeAttribute.cs
- TypeDelegator.cs
- ResourceDescriptionAttribute.cs
- EditorAttribute.cs
- VirtualDirectoryMapping.cs
- UriTemplateDispatchFormatter.cs
- XslTransformFileEditor.cs
- IndexOutOfRangeException.cs
- OrderedDictionary.cs
- ExpressionBindingCollection.cs
- ApplicationBuildProvider.cs