Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / XmlUtils / System / Xml / Xsl / XsltOld / InputScopeManager.cs / 1 / InputScopeManager.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Xsl.XsltOld { using Res = System.Xml.Utils.Res; using System; using System.Diagnostics; using System.Xml; using System.Xml.XPath; internal class InputScopeManager { private InputScope scopeStack; private string defaultNS = string.Empty; private XPathNavigator navigator; // We need this nsvigator for document() function implementation public InputScopeManager(XPathNavigator navigator, InputScope rootScope) { this.navigator = navigator; this.scopeStack = rootScope; } internal InputScope CurrentScope { get { return this.scopeStack; } } internal InputScope VariableScope { get { Debug.Assert(this.scopeStack != null); Debug.Assert(this.scopeStack.Parent != null); return this.scopeStack.Parent; } } internal InputScopeManager Clone() { InputScopeManager manager = new InputScopeManager(this.navigator, null); manager.scopeStack = this.scopeStack; manager.defaultNS = this.defaultNS; return manager; } public XPathNavigator Navigator { get {return this.navigator;} } internal InputScope PushScope() { this.scopeStack = new InputScope(this.scopeStack); return this.scopeStack; } internal void PopScope() { Debug.Assert(this.scopeStack != null, "Push/Pop disbalance"); if (this.scopeStack == null) { return; } for (NamespaceDecl scope = this.scopeStack.Scopes; scope != null; scope = scope.Next) { this.defaultNS = scope.PrevDefaultNsUri; } this.scopeStack = this.scopeStack.Parent; } internal void PushNamespace(string prefix, string nspace) { Debug.Assert(this.scopeStack != null, "PushScope wasn't called"); Debug.Assert(prefix != null); Debug.Assert(nspace != null); this.scopeStack.AddNamespace(prefix, nspace, this.defaultNS); if (prefix == null || prefix.Length == 0) { this.defaultNS = nspace; } } // CompileContext public string DefaultNamespace { get { return this.defaultNS; } } private string ResolveNonEmptyPrefix(string prefix) { Debug.Assert(this.scopeStack != null, "PushScope wasn't called"); Debug.Assert(prefix != null || prefix.Length != 0); if (prefix == Keywords.s_Xml) { return Keywords.s_XmlNamespace; } else if (prefix == Keywords.s_Xmlns) { return Keywords.s_XmlnsNamespace; } for (InputScope inputScope = this.scopeStack; inputScope != null; inputScope = inputScope.Parent) { string nspace = inputScope.ResolveNonAtom(prefix); if (nspace != null) { return nspace; } } throw XsltException.Create(Res.Xslt_InvalidPrefix, prefix); } public string ResolveXmlNamespace(string prefix) { Debug.Assert(prefix != null); if (prefix.Length == 0) { return this.defaultNS; } return ResolveNonEmptyPrefix(prefix); } public string ResolveXPathNamespace(string prefix) { Debug.Assert(prefix != null); if (prefix.Length == 0) { return string.Empty; } return ResolveNonEmptyPrefix(prefix); } internal void InsertExtensionNamespaces(string[] nsList) { Debug.Assert(this.scopeStack != null, "PushScope wasn't called"); Debug.Assert(nsList != null); for (int idx = 0; idx < nsList.Length; idx++) { this.scopeStack.InsertExtensionNamespace(nsList[idx]); } } internal bool IsExtensionNamespace(String nspace) { Debug.Assert(this.scopeStack != null, "PushScope wasn't called"); for (InputScope inputScope = this.scopeStack; inputScope != null; inputScope = inputScope.Parent) { if (inputScope.IsExtensionNamespace( nspace )) { return true; } } return false; } internal void InsertExcludedNamespaces(string[] nsList) { Debug.Assert(this.scopeStack != null, "PushScope wasn't called"); Debug.Assert(nsList != null); for (int idx = 0; idx < nsList.Length; idx++) { this.scopeStack.InsertExcludedNamespace(nsList[idx]); } } internal bool IsExcludedNamespace(String nspace) { Debug.Assert(this.scopeStack != null, "PushScope wasn't called"); for (InputScope inputScope = this.scopeStack; inputScope != null; inputScope = inputScope.Parent) { if (inputScope.IsExcludedNamespace( nspace )) { return true; } } return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Xsl.XsltOld { using Res = System.Xml.Utils.Res; using System; using System.Diagnostics; using System.Xml; using System.Xml.XPath; internal class InputScopeManager { private InputScope scopeStack; private string defaultNS = string.Empty; private XPathNavigator navigator; // We need this nsvigator for document() function implementation public InputScopeManager(XPathNavigator navigator, InputScope rootScope) { this.navigator = navigator; this.scopeStack = rootScope; } internal InputScope CurrentScope { get { return this.scopeStack; } } internal InputScope VariableScope { get { Debug.Assert(this.scopeStack != null); Debug.Assert(this.scopeStack.Parent != null); return this.scopeStack.Parent; } } internal InputScopeManager Clone() { InputScopeManager manager = new InputScopeManager(this.navigator, null); manager.scopeStack = this.scopeStack; manager.defaultNS = this.defaultNS; return manager; } public XPathNavigator Navigator { get {return this.navigator;} } internal InputScope PushScope() { this.scopeStack = new InputScope(this.scopeStack); return this.scopeStack; } internal void PopScope() { Debug.Assert(this.scopeStack != null, "Push/Pop disbalance"); if (this.scopeStack == null) { return; } for (NamespaceDecl scope = this.scopeStack.Scopes; scope != null; scope = scope.Next) { this.defaultNS = scope.PrevDefaultNsUri; } this.scopeStack = this.scopeStack.Parent; } internal void PushNamespace(string prefix, string nspace) { Debug.Assert(this.scopeStack != null, "PushScope wasn't called"); Debug.Assert(prefix != null); Debug.Assert(nspace != null); this.scopeStack.AddNamespace(prefix, nspace, this.defaultNS); if (prefix == null || prefix.Length == 0) { this.defaultNS = nspace; } } // CompileContext public string DefaultNamespace { get { return this.defaultNS; } } private string ResolveNonEmptyPrefix(string prefix) { Debug.Assert(this.scopeStack != null, "PushScope wasn't called"); Debug.Assert(prefix != null || prefix.Length != 0); if (prefix == Keywords.s_Xml) { return Keywords.s_XmlNamespace; } else if (prefix == Keywords.s_Xmlns) { return Keywords.s_XmlnsNamespace; } for (InputScope inputScope = this.scopeStack; inputScope != null; inputScope = inputScope.Parent) { string nspace = inputScope.ResolveNonAtom(prefix); if (nspace != null) { return nspace; } } throw XsltException.Create(Res.Xslt_InvalidPrefix, prefix); } public string ResolveXmlNamespace(string prefix) { Debug.Assert(prefix != null); if (prefix.Length == 0) { return this.defaultNS; } return ResolveNonEmptyPrefix(prefix); } public string ResolveXPathNamespace(string prefix) { Debug.Assert(prefix != null); if (prefix.Length == 0) { return string.Empty; } return ResolveNonEmptyPrefix(prefix); } internal void InsertExtensionNamespaces(string[] nsList) { Debug.Assert(this.scopeStack != null, "PushScope wasn't called"); Debug.Assert(nsList != null); for (int idx = 0; idx < nsList.Length; idx++) { this.scopeStack.InsertExtensionNamespace(nsList[idx]); } } internal bool IsExtensionNamespace(String nspace) { Debug.Assert(this.scopeStack != null, "PushScope wasn't called"); for (InputScope inputScope = this.scopeStack; inputScope != null; inputScope = inputScope.Parent) { if (inputScope.IsExtensionNamespace( nspace )) { return true; } } return false; } internal void InsertExcludedNamespaces(string[] nsList) { Debug.Assert(this.scopeStack != null, "PushScope wasn't called"); Debug.Assert(nsList != null); for (int idx = 0; idx < nsList.Length; idx++) { this.scopeStack.InsertExcludedNamespace(nsList[idx]); } } internal bool IsExcludedNamespace(String nspace) { Debug.Assert(this.scopeStack != null, "PushScope wasn't called"); for (InputScope inputScope = this.scopeStack; inputScope != null; inputScope = inputScope.Parent) { if (inputScope.IsExcludedNamespace( nspace )) { return true; } } return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
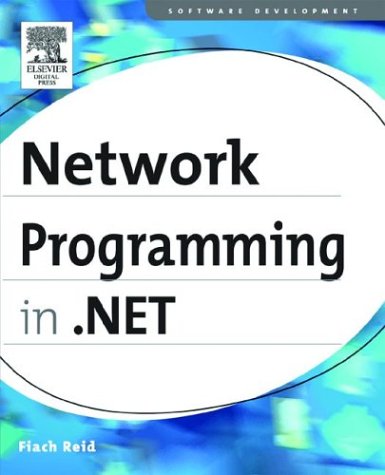
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Stream.cs
- Size.cs
- LoadedEvent.cs
- PeerObject.cs
- ACE.cs
- Monitor.cs
- KoreanLunisolarCalendar.cs
- UriSectionData.cs
- Transform3DGroup.cs
- IdentitySection.cs
- DbConnectionStringCommon.cs
- MarkedHighlightComponent.cs
- SemanticResolver.cs
- FieldAccessException.cs
- VariableQuery.cs
- ClassDataContract.cs
- EndpointInstanceProvider.cs
- OleDbConnectionFactory.cs
- HtmlControl.cs
- CleanUpVirtualizedItemEventArgs.cs
- StateWorkerRequest.cs
- ProviderBase.cs
- ListViewDeleteEventArgs.cs
- SharedStatics.cs
- Policy.cs
- SingleBodyParameterMessageFormatter.cs
- CombinedGeometry.cs
- CodeAssignStatement.cs
- HttpFileCollectionBase.cs
- PropertyOverridesTypeEditor.cs
- ViewSimplifier.cs
- NavigationEventArgs.cs
- HandlerFactoryCache.cs
- EventMappingSettingsCollection.cs
- SQLDoubleStorage.cs
- DelegateBodyWriter.cs
- ToolStripContentPanel.cs
- XmlBinaryReader.cs
- HttpMethodConstraint.cs
- _LoggingObject.cs
- CriticalFinalizerObject.cs
- NumberFormatInfo.cs
- Ipv6Element.cs
- EndpointAddress.cs
- Latin1Encoding.cs
- XmlDataSourceView.cs
- NumberFunctions.cs
- MSAANativeProvider.cs
- InfoCardBaseException.cs
- DrawTreeNodeEventArgs.cs
- ModelUIElement3D.cs
- TabControlAutomationPeer.cs
- SHA384Managed.cs
- SolidBrush.cs
- XmlSchemaSimpleContentExtension.cs
- CompressEmulationStream.cs
- NavigationService.cs
- DataViewSettingCollection.cs
- MemberRelationshipService.cs
- DispatchChannelSink.cs
- Hyperlink.cs
- CrossSiteScriptingValidation.cs
- SettingsPropertyValueCollection.cs
- sortedlist.cs
- ListSortDescriptionCollection.cs
- ResourceDescriptionAttribute.cs
- JournalEntry.cs
- XmlNodeChangedEventArgs.cs
- RSAPKCS1SignatureFormatter.cs
- StorageMappingFragment.cs
- XmlNamespaceDeclarationsAttribute.cs
- ConnectionsZone.cs
- CompilerErrorCollection.cs
- DeclarativeCatalogPart.cs
- TableLayoutStyleCollection.cs
- XPathMessageFilterElementCollection.cs
- SqlDataSourceSummaryPanel.cs
- SoapDocumentMethodAttribute.cs
- ProviderUtil.cs
- PolyBezierSegmentFigureLogic.cs
- Rijndael.cs
- HttpWriter.cs
- DrawingContextWalker.cs
- ExceptionWrapper.cs
- ObjectListCommandEventArgs.cs
- ButtonRenderer.cs
- Axis.cs
- ControlAdapter.cs
- TypeUtil.cs
- RtfToXamlLexer.cs
- FormattedText.cs
- Cloud.cs
- ToolboxService.cs
- HostExecutionContextManager.cs
- BindToObject.cs
- DebugView.cs
- TypeElement.cs
- InstancePersistenceContext.cs
- CodeTypeReference.cs
- XmlQualifiedName.cs