Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / clr / src / BCL / System / SharedStatics.cs / 1 / SharedStatics.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================================== ** ** Class: SharedStatics ** ** ** Purpose: Container for statics that are shared across AppDomains. ** ** =============================================================================*/ namespace System { using System.Threading; using System.Runtime.Remoting; using System.Security; using System.Security.Util; using System.Runtime.CompilerServices; using System.Runtime.ConstrainedExecution; using StringMaker = System.Security.Util.Tokenizer.StringMaker; internal sealed class SharedStatics { // this is declared static but is actually forced to be the same object // for each AppDomain at AppDomain create time. internal static SharedStatics _sharedStatics; // when we create the single object we can construct anything we will need // here. If not too many, then just create them all in the constructor, otherwise // can have the property check & create. Need to be aware of threading issues // when do so though. // Note: This ctor is not called when we setup _sharedStatics via AppDomain::SetupSharedStatics SharedStatics() { _Remoting_Identity_IDGuid = null; _Remoting_Identity_IDSeqNum = 0x40; // Reserve initial numbers for well known objects. _maker = null; } private String _Remoting_Identity_IDGuid; public static String Remoting_Identity_IDGuid { get { if (_sharedStatics._Remoting_Identity_IDGuid == null) { bool tookLock = false; RuntimeHelpers.PrepareConstrainedRegions(); try { Monitor.ReliableEnter(_sharedStatics, ref tookLock); if (_sharedStatics._Remoting_Identity_IDGuid == null) { _sharedStatics._Remoting_Identity_IDGuid = Guid.NewGuid().ToString().Replace('-', '_'); } } finally { if (tookLock) Monitor.Exit(_sharedStatics); } } BCLDebug.Assert(_sharedStatics._Remoting_Identity_IDGuid != null, "_sharedStatics._Remoting_Identity_IDGuid != null"); return _sharedStatics._Remoting_Identity_IDGuid; } } private StringMaker _maker; static public StringMaker GetSharedStringMaker() { StringMaker maker = null; bool tookLock = false; RuntimeHelpers.PrepareConstrainedRegions(); try { Monitor.ReliableEnter(_sharedStatics, ref tookLock); if (_sharedStatics._maker != null) { maker = _sharedStatics._maker; _sharedStatics._maker = null; } } finally { if (tookLock) Monitor.Exit(_sharedStatics); } if (maker == null) { maker = new StringMaker(); } return maker; } static public void ReleaseSharedStringMaker(ref StringMaker maker) { // save this stringmaker so someone else can use it bool tookLock = false; RuntimeHelpers.PrepareConstrainedRegions(); try { Monitor.ReliableEnter(_sharedStatics, ref tookLock); _sharedStatics._maker = maker; maker = null; } finally { if (tookLock) Monitor.Exit(_sharedStatics); } } // Note this may not need to be process-wide. private int _Remoting_Identity_IDSeqNum; internal static int Remoting_Identity_GetNextSeqNum() { return Interlocked.Increment(ref _sharedStatics._Remoting_Identity_IDSeqNum); } // This is the total amount of memory currently "reserved" via // all MemoryFailPoints allocated within the process. // Stored as a long because we need to use Interlocked.Add. private long _memFailPointReservedMemory; [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static long AddMemoryFailPointReservation(long size) { // Size can legitimately be negative - see Dispose. return Interlocked.Add(ref _sharedStatics._memFailPointReservedMemory, (long) size); } internal static ulong MemoryFailPointReservedMemory { get { BCLDebug.Assert(_sharedStatics._memFailPointReservedMemory >= 0, "Process-wide MemoryFailPoint reserved memory was negative!"); return (ulong) _sharedStatics._memFailPointReservedMemory; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================================== ** ** Class: SharedStatics ** ** ** Purpose: Container for statics that are shared across AppDomains. ** ** =============================================================================*/ namespace System { using System.Threading; using System.Runtime.Remoting; using System.Security; using System.Security.Util; using System.Runtime.CompilerServices; using System.Runtime.ConstrainedExecution; using StringMaker = System.Security.Util.Tokenizer.StringMaker; internal sealed class SharedStatics { // this is declared static but is actually forced to be the same object // for each AppDomain at AppDomain create time. internal static SharedStatics _sharedStatics; // when we create the single object we can construct anything we will need // here. If not too many, then just create them all in the constructor, otherwise // can have the property check & create. Need to be aware of threading issues // when do so though. // Note: This ctor is not called when we setup _sharedStatics via AppDomain::SetupSharedStatics SharedStatics() { _Remoting_Identity_IDGuid = null; _Remoting_Identity_IDSeqNum = 0x40; // Reserve initial numbers for well known objects. _maker = null; } private String _Remoting_Identity_IDGuid; public static String Remoting_Identity_IDGuid { get { if (_sharedStatics._Remoting_Identity_IDGuid == null) { bool tookLock = false; RuntimeHelpers.PrepareConstrainedRegions(); try { Monitor.ReliableEnter(_sharedStatics, ref tookLock); if (_sharedStatics._Remoting_Identity_IDGuid == null) { _sharedStatics._Remoting_Identity_IDGuid = Guid.NewGuid().ToString().Replace('-', '_'); } } finally { if (tookLock) Monitor.Exit(_sharedStatics); } } BCLDebug.Assert(_sharedStatics._Remoting_Identity_IDGuid != null, "_sharedStatics._Remoting_Identity_IDGuid != null"); return _sharedStatics._Remoting_Identity_IDGuid; } } private StringMaker _maker; static public StringMaker GetSharedStringMaker() { StringMaker maker = null; bool tookLock = false; RuntimeHelpers.PrepareConstrainedRegions(); try { Monitor.ReliableEnter(_sharedStatics, ref tookLock); if (_sharedStatics._maker != null) { maker = _sharedStatics._maker; _sharedStatics._maker = null; } } finally { if (tookLock) Monitor.Exit(_sharedStatics); } if (maker == null) { maker = new StringMaker(); } return maker; } static public void ReleaseSharedStringMaker(ref StringMaker maker) { // save this stringmaker so someone else can use it bool tookLock = false; RuntimeHelpers.PrepareConstrainedRegions(); try { Monitor.ReliableEnter(_sharedStatics, ref tookLock); _sharedStatics._maker = maker; maker = null; } finally { if (tookLock) Monitor.Exit(_sharedStatics); } } // Note this may not need to be process-wide. private int _Remoting_Identity_IDSeqNum; internal static int Remoting_Identity_GetNextSeqNum() { return Interlocked.Increment(ref _sharedStatics._Remoting_Identity_IDSeqNum); } // This is the total amount of memory currently "reserved" via // all MemoryFailPoints allocated within the process. // Stored as a long because we need to use Interlocked.Add. private long _memFailPointReservedMemory; [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static long AddMemoryFailPointReservation(long size) { // Size can legitimately be negative - see Dispose. return Interlocked.Add(ref _sharedStatics._memFailPointReservedMemory, (long) size); } internal static ulong MemoryFailPointReservedMemory { get { BCLDebug.Assert(_sharedStatics._memFailPointReservedMemory >= 0, "Process-wide MemoryFailPoint reserved memory was negative!"); return (ulong) _sharedStatics._memFailPointReservedMemory; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
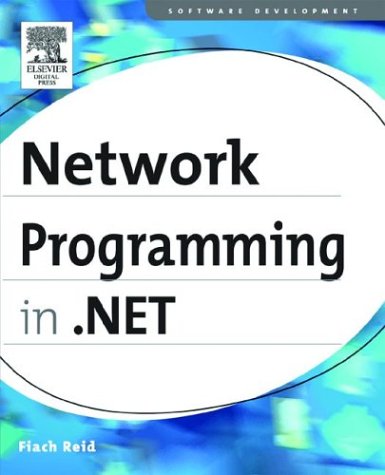
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HighlightComponent.cs
- EventQueueState.cs
- SinglePageViewer.cs
- Fx.cs
- SecureStringHasher.cs
- DbConnectionPool.cs
- ObjRef.cs
- ImageDrawing.cs
- StringResourceManager.cs
- StringToken.cs
- Function.cs
- LoginView.cs
- SymmetricKeyWrap.cs
- NavigationPropertyAccessor.cs
- FontFamily.cs
- XhtmlBasicSelectionListAdapter.cs
- WebScriptEnablingBehavior.cs
- XmlEventCache.cs
- AsymmetricAlgorithm.cs
- ImageListUtils.cs
- Conditional.cs
- LineServicesCallbacks.cs
- StatusBarAutomationPeer.cs
- RelationshipEndCollection.cs
- CheckPair.cs
- GridViewSortEventArgs.cs
- HostingEnvironmentWrapper.cs
- BlurEffect.cs
- PropertyConverter.cs
- NativeCppClassAttribute.cs
- TagMapInfo.cs
- LocalizableResourceBuilder.cs
- ContainsRowNumberChecker.cs
- CodeNamespace.cs
- StrongNameHelpers.cs
- RedirectionProxy.cs
- InfoCardKeyedHashAlgorithm.cs
- ObjectSecurity.cs
- TransportListener.cs
- ProjectedWrapper.cs
- NGCSerializationManager.cs
- ReadOnlyDataSource.cs
- WebPart.cs
- ListDataHelper.cs
- XmlnsCache.cs
- StylusPointPropertyInfo.cs
- XNodeNavigator.cs
- DragStartedEventArgs.cs
- MaterialGroup.cs
- WebPartTransformerCollection.cs
- CodeCommentStatementCollection.cs
- DocumentAutomationPeer.cs
- CodeMemberEvent.cs
- SendMessageRecord.cs
- GetPageNumberCompletedEventArgs.cs
- MenuCommand.cs
- StateWorkerRequest.cs
- MetadataUtil.cs
- ConfigXmlElement.cs
- CodeObject.cs
- linebase.cs
- EmptyStringExpandableObjectConverter.cs
- CannotUnloadAppDomainException.cs
- StringReader.cs
- PointLight.cs
- HitTestWithPointDrawingContextWalker.cs
- HandleRef.cs
- ListParagraph.cs
- ParseElementCollection.cs
- SafeFindHandle.cs
- ResponseBodyWriter.cs
- OracleInternalConnection.cs
- OrderedEnumerableRowCollection.cs
- SQLString.cs
- SiblingIterators.cs
- Light.cs
- log.cs
- LambdaExpression.cs
- LogConverter.cs
- RowBinding.cs
- MemberInfoSerializationHolder.cs
- TypeExtension.cs
- GradientStop.cs
- FactoryMaker.cs
- IdentityHolder.cs
- ContextStaticAttribute.cs
- BuilderInfo.cs
- COM2ExtendedUITypeEditor.cs
- DebugController.cs
- SqlConnectionPoolGroupProviderInfo.cs
- IResourceProvider.cs
- ArrangedElementCollection.cs
- securestring.cs
- NavigationProperty.cs
- HtmlTextArea.cs
- PartManifestEntry.cs
- EmbossBitmapEffect.cs
- TextWriter.cs
- MenuItemStyle.cs
- InstallerTypeAttribute.cs