Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / WebParts / WebPartTransformerCollection.cs / 1305376 / WebPartTransformerCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls.WebParts { using System; using System.Collections; public sealed class WebPartTransformerCollection : CollectionBase { private bool _readOnly; public bool IsReadOnly { get { return _readOnly; } } public WebPartTransformer this[int index] { get { return (WebPartTransformer) List[index]; } set { List[index] = value; } } public int Add(WebPartTransformer transformer) { return List.Add(transformer); } private void CheckReadOnly() { if (_readOnly) { throw new InvalidOperationException(SR.GetString(SR.WebPartTransformerCollection_ReadOnly)); } } public bool Contains(WebPartTransformer transformer) { return List.Contains(transformer); } public void CopyTo(WebPartTransformer[] array, int index) { List.CopyTo(array, index); } public int IndexOf(WebPartTransformer transformer) { return List.IndexOf(transformer); } public void Insert(int index, WebPartTransformer transformer) { List.Insert(index, transformer); } protected override void OnClear() { CheckReadOnly(); base.OnClear(); } protected override void OnInsert(int index, object value) { CheckReadOnly(); if (List.Count > 0) { throw new InvalidOperationException(SR.GetString(SR.WebPartTransformerCollection_NotEmpty)); } base.OnInsert(index, value); } protected override void OnRemove(int index, object value) { CheckReadOnly(); base.OnRemove(index, value); } protected override void OnSet(int index, object oldValue, object newValue) { CheckReadOnly(); base.OnSet(index, oldValue, newValue); } protected override void OnValidate(object value) { base.OnValidate(value); if (value == null) { throw new ArgumentNullException("value", SR.GetString(SR.Collection_CantAddNull)); } if (!(value is WebPartTransformer)) { throw new ArgumentException(SR.GetString(SR.Collection_InvalidType, "WebPartTransformer"), "value"); } } public void Remove(WebPartTransformer transformer) { List.Remove(transformer); } internal void SetReadOnly() { _readOnly = true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls.WebParts { using System; using System.Collections; public sealed class WebPartTransformerCollection : CollectionBase { private bool _readOnly; public bool IsReadOnly { get { return _readOnly; } } public WebPartTransformer this[int index] { get { return (WebPartTransformer) List[index]; } set { List[index] = value; } } public int Add(WebPartTransformer transformer) { return List.Add(transformer); } private void CheckReadOnly() { if (_readOnly) { throw new InvalidOperationException(SR.GetString(SR.WebPartTransformerCollection_ReadOnly)); } } public bool Contains(WebPartTransformer transformer) { return List.Contains(transformer); } public void CopyTo(WebPartTransformer[] array, int index) { List.CopyTo(array, index); } public int IndexOf(WebPartTransformer transformer) { return List.IndexOf(transformer); } public void Insert(int index, WebPartTransformer transformer) { List.Insert(index, transformer); } protected override void OnClear() { CheckReadOnly(); base.OnClear(); } protected override void OnInsert(int index, object value) { CheckReadOnly(); if (List.Count > 0) { throw new InvalidOperationException(SR.GetString(SR.WebPartTransformerCollection_NotEmpty)); } base.OnInsert(index, value); } protected override void OnRemove(int index, object value) { CheckReadOnly(); base.OnRemove(index, value); } protected override void OnSet(int index, object oldValue, object newValue) { CheckReadOnly(); base.OnSet(index, oldValue, newValue); } protected override void OnValidate(object value) { base.OnValidate(value); if (value == null) { throw new ArgumentNullException("value", SR.GetString(SR.Collection_CantAddNull)); } if (!(value is WebPartTransformer)) { throw new ArgumentException(SR.GetString(SR.Collection_InvalidType, "WebPartTransformer"), "value"); } } public void Remove(WebPartTransformer transformer) { List.Remove(transformer); } internal void SetReadOnly() { _readOnly = true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
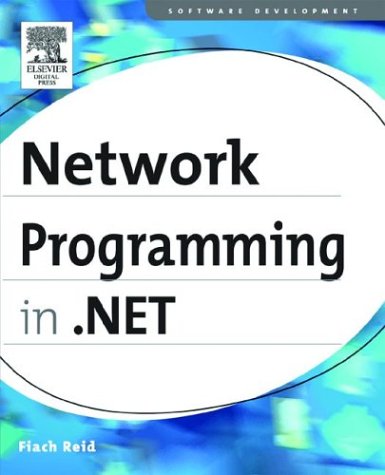
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InlineCollection.cs
- RequestCachePolicy.cs
- SevenBitStream.cs
- SessionEndingEventArgs.cs
- Point3DConverter.cs
- COM2ComponentEditor.cs
- ListBox.cs
- MetaChildrenColumn.cs
- DataListItem.cs
- SudsParser.cs
- LocalizableResourceBuilder.cs
- CodeDirectiveCollection.cs
- TranslateTransform3D.cs
- RelatedImageListAttribute.cs
- CalendarDesigner.cs
- CachedFontFamily.cs
- RsaSecurityKey.cs
- ManagementPath.cs
- DataStorage.cs
- DateTimePicker.cs
- SafeNativeMethodsCLR.cs
- ObjectDataSourceView.cs
- ImageAttributes.cs
- ProcessThreadCollection.cs
- ValidatorCollection.cs
- FontWeight.cs
- TimeSpan.cs
- MobileComponentEditorPage.cs
- ImageDrawing.cs
- ClaimSet.cs
- DirectionalLight.cs
- PhysicalOps.cs
- OdbcPermission.cs
- DurationConverter.cs
- InternalConfigConfigurationFactory.cs
- DocumentViewerBaseAutomationPeer.cs
- PageSettings.cs
- DataTemplateKey.cs
- ConfigurationException.cs
- UIElement3D.cs
- SortableBindingList.cs
- LicenseContext.cs
- HtmlString.cs
- CheckBoxAutomationPeer.cs
- XmlNamespaceDeclarationsAttribute.cs
- HistoryEventArgs.cs
- ConfigurationPropertyAttribute.cs
- ArraySegment.cs
- Input.cs
- FormParameter.cs
- KeyValueInternalCollection.cs
- UnsafeNativeMethodsCLR.cs
- UdpMessageProperty.cs
- Point4DValueSerializer.cs
- EventLogPermissionAttribute.cs
- AutoGeneratedField.cs
- QuerySelectOp.cs
- BufferModeSettings.cs
- _CookieModule.cs
- BaseConfigurationRecord.cs
- Vector3DValueSerializer.cs
- SplitterCancelEvent.cs
- Point3D.cs
- DependencyPropertyKey.cs
- MailMessageEventArgs.cs
- OleDbConnectionInternal.cs
- SupportsEventValidationAttribute.cs
- SchemaMerger.cs
- FamilyMapCollection.cs
- Scanner.cs
- SafeNativeMemoryHandle.cs
- WsatRegistrationHeader.cs
- DesignerAttribute.cs
- IntPtr.cs
- DynamicResourceExtension.cs
- ClientSettingsSection.cs
- UIAgentAsyncEndRequest.cs
- EdmFunctionAttribute.cs
- BlobPersonalizationState.cs
- Binding.cs
- DetailsViewInsertEventArgs.cs
- BookmarkScopeHandle.cs
- XmlSchemaType.cs
- CatalogPartCollection.cs
- EntityDataSourceWizardForm.cs
- ArithmeticLiteral.cs
- TypeReference.cs
- ErrorFormatterPage.cs
- XmlCustomFormatter.cs
- DataGridViewRowHeaderCell.cs
- SortDescription.cs
- HtmlElementErrorEventArgs.cs
- Encoder.cs
- HitTestParameters.cs
- ApplicationId.cs
- StringDictionaryCodeDomSerializer.cs
- TextTreeExtractElementUndoUnit.cs
- ReaderWriterLockWrapper.cs
- LiteralControl.cs
- DisplayMemberTemplateSelector.cs