Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / NetFx35 / System.ServiceModel.Web / System / ServiceModel / Dispatcher / SingleBodyParameterMessageFormatter.cs / 1 / SingleBodyParameterMessageFormatter.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Dispatcher { using System; using System.ServiceModel; using System.ServiceModel.Channels; using System.ServiceModel.Description; using System.Collections.Generic; using System.Xml; using System.Runtime.Serialization; using DiagnosticUtility = System.ServiceModel.DiagnosticUtility; using System.ServiceModel.Web; abstract class SingleBodyParameterMessageFormatter : IDispatchMessageFormatter, IClientMessageFormatter { string contractName; string contractNs; bool isRequestFormatter; string operationName; string serializerType; protected SingleBodyParameterMessageFormatter(OperationDescription operation, bool isRequestFormatter, string serializerType) { if (operation == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("operation"); } this.contractName = operation.DeclaringContract.Name; this.contractNs = operation.DeclaringContract.Namespace; this.operationName = operation.Name; this.isRequestFormatter = isRequestFormatter; this.serializerType = serializerType; } protected string ContractName { get { return this.contractName; } } protected string ContractNs { get { return this.contractNs; } } protected string OperationName { get { return this.operationName; } } public static IClientMessageFormatter CreateXmlAndJsonClientFormatter(OperationDescription operation, Type type, bool isRequestFormatter, UnwrappedTypesXmlSerializerManager xmlSerializerManager) { IClientMessageFormatter xmlFormatter = CreateClientFormatter(operation, type, isRequestFormatter, false, xmlSerializerManager); if (!WebHttpBehavior.SupportsJsonFormat(operation)) { return xmlFormatter; } IClientMessageFormatter jsonFormatter = CreateClientFormatter(operation, type, isRequestFormatter, true, xmlSerializerManager); Dictionarymap = new Dictionary (); map.Add(WebContentFormat.Xml, xmlFormatter); map.Add(WebContentFormat.Json, jsonFormatter); return new DemultiplexingClientMessageFormatter(map, xmlFormatter); } public static IDispatchMessageFormatter CreateXmlAndJsonDispatchFormatter(OperationDescription operation, Type type, bool isRequestFormatter, UnwrappedTypesXmlSerializerManager xmlSerializerManager) { IDispatchMessageFormatter xmlFormatter = CreateDispatchFormatter(operation, type, isRequestFormatter, false, xmlSerializerManager); if (!WebHttpBehavior.SupportsJsonFormat(operation)) { return xmlFormatter; } IDispatchMessageFormatter jsonFormatter = CreateDispatchFormatter(operation, type, isRequestFormatter, true, xmlSerializerManager); Dictionary map = new Dictionary (); map.Add(WebContentFormat.Xml, xmlFormatter); map.Add(WebContentFormat.Json, jsonFormatter); return new DemultiplexingDispatchMessageFormatter(map, xmlFormatter); } public object DeserializeReply(Message message, object[] parameters) { if (isRequestFormatter) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR2.GetString(SR2.FormatterCannotBeUsedForReplyMessages))); } return ReadObject(message); } public void DeserializeRequest(Message message, object[] parameters) { if (!isRequestFormatter) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR2.GetString(SR2.FormatterCannotBeUsedForRequestMessages))); } parameters[0] = ReadObject(message); } public Message SerializeReply(MessageVersion messageVersion, object[] parameters, object result) { if (isRequestFormatter) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR2.GetString(SR2.FormatterCannotBeUsedForReplyMessages))); } Message message = Message.CreateMessage(messageVersion, (string) null, CreateBodyWriter(result)); if (result == null) { SuppressReplyEntityBody(message); } AttachMessageProperties(message, false); return message; } public Message SerializeRequest(MessageVersion messageVersion, object[] parameters) { if (!isRequestFormatter) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR2.GetString(SR2.FormatterCannotBeUsedForRequestMessages))); } Message message = Message.CreateMessage(messageVersion, (string) null, CreateBodyWriter(parameters[0])); if (parameters[0] == null) { SuppressRequestEntityBody(message); } AttachMessageProperties(message, true); return message; } internal static IClientMessageFormatter CreateClientFormatter(OperationDescription operation, Type type, bool isRequestFormatter, bool useJson, UnwrappedTypesXmlSerializerManager xmlSerializerManager) { if (type == null) { return new NullMessageFormatter(); } else { return CreateFormatter(operation, type, isRequestFormatter, useJson, xmlSerializerManager); } } internal static IDispatchMessageFormatter CreateDispatchFormatter(OperationDescription operation, Type type, bool isRequestFormatter, bool useJson, UnwrappedTypesXmlSerializerManager xmlSerializerManager) { if (type == null) { return new NullMessageFormatter(); } else { return CreateFormatter(operation, type, isRequestFormatter, useJson, xmlSerializerManager); } } internal static void SuppressReplyEntityBody(Message message) { if (WebOperationContext.Current != null) { WebOperationContext.Current.OutgoingResponse.SuppressEntityBody = true; } else { object untypedProp; message.Properties.TryGetValue(HttpResponseMessageProperty.Name, out untypedProp); HttpResponseMessageProperty prop = untypedProp as HttpResponseMessageProperty; if (prop == null) { prop = new HttpResponseMessageProperty(); message.Properties[HttpResponseMessageProperty.Name] = prop; } prop.SuppressEntityBody = true; } } internal static void SuppressRequestEntityBody(Message message) { if (WebOperationContext.Current != null) { WebOperationContext.Current.OutgoingRequest.SuppressEntityBody = true; } else { object untypedProp; message.Properties.TryGetValue(HttpRequestMessageProperty.Name, out untypedProp); HttpRequestMessageProperty prop = untypedProp as HttpRequestMessageProperty; if (prop == null) { prop = new HttpRequestMessageProperty(); message.Properties[HttpRequestMessageProperty.Name] = prop; } prop.SuppressEntityBody = true; } } protected virtual void AttachMessageProperties(Message message, bool isRequest) { } protected abstract XmlObjectSerializer[] GetInputSerializers(); protected abstract XmlObjectSerializer GetOutputSerializer(Type type); protected virtual void ValidateMessageFormatProperty(Message message) { } protected void ValidateOutputType(Type type, Type parameterType, IList knownTypes) { // ensure that the type is the parameter type or is in the list of known types bool isValidType = false; if (type == parameterType) { isValidType = true; } else if (knownTypes != null) { for (int i = 0; i < knownTypes.Count; ++i) { if (type == knownTypes[i]) { isValidType = true; break; } } } if (!isValidType) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SerializationException(SR2.GetString(SR2.TypeIsNotParameterTypeAndIsNotPresentInKnownTypes, type, this.OperationName, this.ContractName, parameterType))); } } static SingleBodyParameterMessageFormatter CreateFormatter(OperationDescription operation, Type type, bool isRequestFormatter, bool useJson, UnwrappedTypesXmlSerializerManager xmlSerializerManager) { IOperationBehavior behavior = operation.Behaviors.Find (); if (behavior == null) { behavior = operation.Behaviors.Find (); } return CreateFormatter(behavior, operation, type, isRequestFormatter, useJson, xmlSerializerManager); } static SingleBodyParameterMessageFormatter CreateFormatter(IOperationBehavior behavior, OperationDescription operation, Type type, bool isRequestFormatter, bool useJson, UnwrappedTypesXmlSerializerManager xmlSerializerManager) { DataContractSerializerOperationBehavior dcsob = behavior as DataContractSerializerOperationBehavior; if (useJson) { if (dcsob == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR2.GetString(SR2.JsonFormatRequiresDataContract, operation.Name, operation.DeclaringContract.Name, operation.DeclaringContract.Namespace))); } } if (dcsob != null) { return new SingleBodyParameterDataContractMessageFormatter(operation, type, isRequestFormatter, useJson, dcsob); } XmlSerializerOperationBehavior xsob = behavior as XmlSerializerOperationBehavior; if (xsob != null) { return new SingleBodyParameterXmlSerializerMessageFormatter(operation, type, isRequestFormatter, xsob, xmlSerializerManager); } throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException(SR2.GetString(SR2.OnlyDataContractAndXmlSerializerTypesInUnWrappedMode, operation.Name))); } BodyWriter CreateBodyWriter(object body) { XmlObjectSerializer serializer; if (body != null) { serializer = GetOutputSerializer(body.GetType()); if (serializer == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException(SR2.GetString(SR2.CannotSerializeType, body.GetType(), this.operationName, this.contractName, this.contractNs, this.serializerType))); } } else { serializer = null; } return new SingleParameterBodyWriter(body, serializer); } object ReadObject(Message message) { if (HttpStreamFormatter.IsEmptyMessage(message)) { return null; } XmlObjectSerializer[] inputSerializers = GetInputSerializers(); XmlDictionaryReader reader = message.GetReaderAtBodyContents(); if (inputSerializers != null) { for (int i = 0; i < inputSerializers.Length; ++i) { if (inputSerializers[i].IsStartObject(reader)) { return inputSerializers[i].ReadObject(reader, false); } } } throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SerializationException(SR2.GetString(SR2.CannotDeserializeBody, reader.LocalName, reader.NamespaceURI, operationName, contractName, contractNs, this.serializerType))); } class NullMessageFormatter : IClientMessageFormatter, IDispatchMessageFormatter { public object DeserializeReply(Message message, object[] parameters) { return null; } public void DeserializeRequest(Message message, object[] parameters) { } public Message SerializeReply(MessageVersion messageVersion, object[] parameters, object result) { Message reply = Message.CreateMessage(messageVersion, (string) null); SuppressReplyEntityBody(reply); return reply; } public Message SerializeRequest(MessageVersion messageVersion, object[] parameters) { Message request = Message.CreateMessage(messageVersion, (string) null); SuppressRequestEntityBody(request); return request; } } class SingleParameterBodyWriter : BodyWriter { object body; XmlObjectSerializer serializer; public SingleParameterBodyWriter(object body, XmlObjectSerializer serializer) : base(false) { if (body != null && serializer == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("serializer"); } this.body = body; this.serializer = serializer; } protected override void OnWriteBodyContents(XmlDictionaryWriter writer) { if (body != null) { this.serializer.WriteObject(writer, body); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
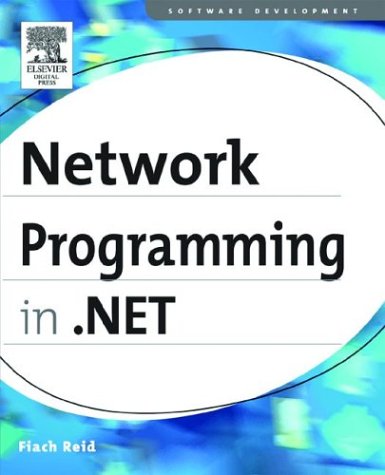
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UInt16Converter.cs
- UIAgentAsyncParams.cs
- MsmqChannelFactoryBase.cs
- DisplayClaim.cs
- BamlTreeNode.cs
- IssuedTokenClientBehaviorsElementCollection.cs
- DataGridViewSelectedColumnCollection.cs
- ImmComposition.cs
- DataAccessor.cs
- TreeBuilder.cs
- DisplayMemberTemplateSelector.cs
- ListViewInsertEventArgs.cs
- ProviderUtil.cs
- ByteAnimationBase.cs
- InternalDispatchObject.cs
- SchemaComplexType.cs
- ColorConvertedBitmapExtension.cs
- AddressUtility.cs
- HtmlMeta.cs
- SmtpClient.cs
- PartManifestEntry.cs
- RemotingService.cs
- ViewgenGatekeeper.cs
- SoapReflectionImporter.cs
- XamlStream.cs
- ExtractorMetadata.cs
- PermissionSet.cs
- HostProtectionPermission.cs
- DateTimeValueSerializerContext.cs
- IListConverters.cs
- EventLogPropertySelector.cs
- FunctionQuery.cs
- ServiceContractDetailViewControl.cs
- FileInfo.cs
- GuidConverter.cs
- ProcessProtocolHandler.cs
- PositiveTimeSpanValidatorAttribute.cs
- TableLayoutRowStyleCollection.cs
- DeferredElementTreeState.cs
- DetailsViewDeletedEventArgs.cs
- ExecutionProperties.cs
- NonBatchDirectoryCompiler.cs
- CharStorage.cs
- HitTestParameters3D.cs
- XmlSchemaCollection.cs
- SizeLimitedCache.cs
- TextEditorLists.cs
- PipeStream.cs
- DaylightTime.cs
- ISAPIApplicationHost.cs
- UshortList2.cs
- AppDomainShutdownMonitor.cs
- MDIWindowDialog.cs
- AuthStoreRoleProvider.cs
- SocketPermission.cs
- ListView.cs
- CollectionViewGroupInternal.cs
- SqlDataSourceView.cs
- ReferenceEqualityComparer.cs
- FunctionImportElement.cs
- RuntimeConfigurationRecord.cs
- HMACSHA1.cs
- FileCodeGroup.cs
- AttributedMetaModel.cs
- Stopwatch.cs
- RuleSetBrowserDialog.cs
- SoapCodeExporter.cs
- TableSectionStyle.cs
- ColumnMapTranslator.cs
- XmlDocumentFragment.cs
- ValidationUtility.cs
- CodeGenerator.cs
- GridViewRow.cs
- bidPrivateBase.cs
- ModelTypeConverter.cs
- ExitEventArgs.cs
- PowerStatus.cs
- CheckBoxBaseAdapter.cs
- ErrorLog.cs
- InitializingNewItemEventArgs.cs
- Itemizer.cs
- AstNode.cs
- SemanticResultKey.cs
- TextContainerChangeEventArgs.cs
- VerificationException.cs
- ScrollProviderWrapper.cs
- CollectionDataContractAttribute.cs
- HttpVersion.cs
- EdmEntityTypeAttribute.cs
- UIntPtr.cs
- DataGridComponentEditor.cs
- IPAddress.cs
- AttachmentService.cs
- LookupBindingPropertiesAttribute.cs
- SubpageParaClient.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- SystemTcpStatistics.cs
- XmlDataSourceView.cs
- SponsorHelper.cs
- TreeNodeEventArgs.cs