Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataWeb / Design / system / Data / Entity / Design / Common / UniqueIdentifierService.cs / 1 / UniqueIdentifierService.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Diagnostics; using System.Collections.Generic; using System.Globalization; namespace System.Data.Services.Design.Common { ////// Service making names within a scope unique. Initialize a new instance /// for every scope. /// /// internal sealed class UniqueIdentifierService { internal UniqueIdentifierService(bool caseSensitive) { _knownIdentifiers = new HashSet (caseSensitive ? StringComparer.Ordinal : StringComparer.OrdinalIgnoreCase); _identifierToAdjustedIdentifier = new Dictionary
Link Menu
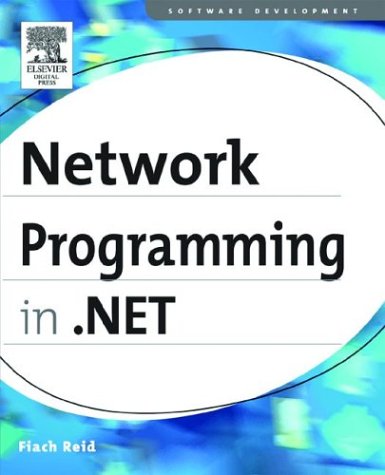
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MailSettingsSection.cs
- DockPatternIdentifiers.cs
- DocumentApplication.cs
- AnalyzedTree.cs
- Oid.cs
- Selection.cs
- ChtmlTextWriter.cs
- FixedSOMLineRanges.cs
- Matrix3D.cs
- ServiceParser.cs
- DashStyle.cs
- TableLayoutRowStyleCollection.cs
- RequestSecurityTokenResponse.cs
- GraphicsContainer.cs
- IssuanceLicense.cs
- DataBinding.cs
- DesignerProperties.cs
- QilLoop.cs
- CounterSetInstanceCounterDataSet.cs
- SecurityContextTokenValidationException.cs
- BoundField.cs
- SqlDataSourceSelectingEventArgs.cs
- OdbcHandle.cs
- ParenthesizePropertyNameAttribute.cs
- TreeChangeInfo.cs
- SaveFileDialogDesigner.cs
- RegexNode.cs
- IncrementalHitTester.cs
- SafeProcessHandle.cs
- TaskFormBase.cs
- DataGridViewCheckBoxCell.cs
- FileAccessException.cs
- SubMenuStyleCollection.cs
- Util.cs
- LinqDataSourceInsertEventArgs.cs
- UserControl.cs
- TypeSystem.cs
- AudioDeviceOut.cs
- HotSpot.cs
- DeleteMemberBinder.cs
- TargetFrameworkUtil.cs
- EntityCodeGenerator.cs
- TailCallAnalyzer.cs
- InstanceStore.cs
- ApplicationActivator.cs
- Point3DCollectionConverter.cs
- SafeSecurityHandles.cs
- ToolboxItemFilterAttribute.cs
- JoinGraph.cs
- ColumnWidthChangedEvent.cs
- ScaleTransform3D.cs
- XmlSerializerAssemblyAttribute.cs
- BaseDataBoundControl.cs
- RadioButtonAutomationPeer.cs
- PassportAuthentication.cs
- ConnectionStringsSection.cs
- Facet.cs
- DesignerOptionService.cs
- PublisherMembershipCondition.cs
- Evidence.cs
- CommandField.cs
- ImageSourceTypeConverter.cs
- HttpProtocolReflector.cs
- Events.cs
- Attributes.cs
- InputLanguageEventArgs.cs
- AssertFilter.cs
- Double.cs
- BindingSource.cs
- ViewgenGatekeeper.cs
- ProxyHelper.cs
- HScrollProperties.cs
- ISessionStateStore.cs
- DefaultClaimSet.cs
- FirstMatchCodeGroup.cs
- EventProvider.cs
- XmlEnumAttribute.cs
- Control.cs
- _TimerThread.cs
- DescendantOverDescendantQuery.cs
- PointCollectionConverter.cs
- OdbcTransaction.cs
- PriorityQueue.cs
- ListChangedEventArgs.cs
- DataGridItemCollection.cs
- __FastResourceComparer.cs
- TemplatePagerField.cs
- TraceEventCache.cs
- PointAnimationUsingKeyFrames.cs
- CommonObjectSecurity.cs
- EdmComplexTypeAttribute.cs
- WebPartsPersonalization.cs
- ProcessModelInfo.cs
- GeneralTransformCollection.cs
- ObjectContextServiceProvider.cs
- HorizontalAlignConverter.cs
- PublishLicense.cs
- DragAssistanceManager.cs
- DataBoundLiteralControl.cs
- OutputCacheSettings.cs