Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Xml / System / Xml / XPath / Internal / XPathSelectionIterator.cs / 1 / XPathSelectionIterator.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace MS.Internal.Xml.XPath { using System; using System.Xml; using System.Xml.XPath; using System.Diagnostics; using System.Globalization; // We need this wrapper object to: // 1. Calculate position // 2. Protect internal query.Current from user who may call MoveNext(). internal class XPathSelectionIterator : ResetableIterator { private XPathNavigator nav; private Query query; private int position; internal XPathSelectionIterator(XPathNavigator nav, Query query) { this.nav = nav.Clone(); this.query = query; } protected XPathSelectionIterator(XPathSelectionIterator it) { this.nav = it.nav.Clone(); this.query = (Query) it.query.Clone(); this.position = it.position; } public override void Reset() { this.query.Reset(); } public override bool MoveNext() { XPathNavigator n = query.Advance(); if( n != null ) { position++; if (!nav.MoveTo(n)) { nav = n.Clone(); } return true; } return false; } public override int Count { get { return query.Count; } } public override XPathNavigator Current { get { return nav; } } public override int CurrentPosition { get { return position; } } public override XPathNodeIterator Clone() { return new XPathSelectionIterator(this); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace MS.Internal.Xml.XPath { using System; using System.Xml; using System.Xml.XPath; using System.Diagnostics; using System.Globalization; // We need this wrapper object to: // 1. Calculate position // 2. Protect internal query.Current from user who may call MoveNext(). internal class XPathSelectionIterator : ResetableIterator { private XPathNavigator nav; private Query query; private int position; internal XPathSelectionIterator(XPathNavigator nav, Query query) { this.nav = nav.Clone(); this.query = query; } protected XPathSelectionIterator(XPathSelectionIterator it) { this.nav = it.nav.Clone(); this.query = (Query) it.query.Clone(); this.position = it.position; } public override void Reset() { this.query.Reset(); } public override bool MoveNext() { XPathNavigator n = query.Advance(); if( n != null ) { position++; if (!nav.MoveTo(n)) { nav = n.Clone(); } return true; } return false; } public override int Count { get { return query.Count; } } public override XPathNavigator Current { get { return nav; } } public override int CurrentPosition { get { return position; } } public override XPathNodeIterator Clone() { return new XPathSelectionIterator(this); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
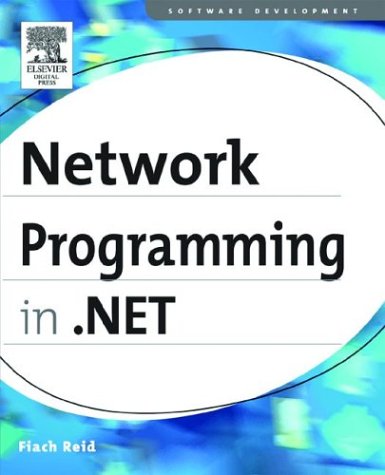
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ManagedCodeMarkers.cs
- CancelEventArgs.cs
- PrintingPermission.cs
- documentsequencetextview.cs
- path.cs
- CuspData.cs
- InsufficientExecutionStackException.cs
- DiscoveryClientRequestChannel.cs
- UIInitializationException.cs
- APCustomTypeDescriptor.cs
- SettingsAttributes.cs
- Globals.cs
- ExpressionEditor.cs
- CounterCreationData.cs
- ContextQuery.cs
- BinaryParser.cs
- AnimationTimeline.cs
- ObjectResult.cs
- ListenerChannelContext.cs
- CommonDialog.cs
- LookupBindingPropertiesAttribute.cs
- StringInfo.cs
- BitmapEffectvisualstate.cs
- TransformedBitmap.cs
- Attributes.cs
- MetricEntry.cs
- versioninfo.cs
- ResourcePermissionBaseEntry.cs
- ClientBuildManagerTypeDescriptionProviderBridge.cs
- Grant.cs
- CommunicationObjectAbortedException.cs
- PriorityBinding.cs
- ConfigurationStrings.cs
- ICollection.cs
- PolicyException.cs
- ReadOnlyHierarchicalDataSourceView.cs
- SignatureDescription.cs
- SingleStorage.cs
- HtmlInputSubmit.cs
- EmbeddedObject.cs
- UnsafeNativeMethods.cs
- HttpModuleCollection.cs
- RTTypeWrapper.cs
- HtmlSelect.cs
- XmlAnyElementAttribute.cs
- AspProxy.cs
- DataControlImageButton.cs
- GlobalizationSection.cs
- TextBoxLine.cs
- JournalNavigationScope.cs
- LoadGrammarCompletedEventArgs.cs
- XmlChildEnumerator.cs
- DataTableMappingCollection.cs
- ArgumentOutOfRangeException.cs
- BamlRecordWriter.cs
- UITypeEditor.cs
- TypedRowGenerator.cs
- HtmlListAdapter.cs
- DataGridViewCellCollection.cs
- DataErrorValidationRule.cs
- MsmqException.cs
- SafeRightsManagementQueryHandle.cs
- SizeFConverter.cs
- CrossAppDomainChannel.cs
- HttpFileCollectionBase.cs
- RegistryDataKey.cs
- Buffer.cs
- Bits.cs
- SerializerProvider.cs
- TrustLevelCollection.cs
- SpecialNameAttribute.cs
- GraphicsPath.cs
- CompositeScriptReference.cs
- IsolationInterop.cs
- ToolStripHighContrastRenderer.cs
- TypeSchema.cs
- SQLInt32Storage.cs
- EtwTrackingParticipant.cs
- TransformProviderWrapper.cs
- UIAgentRequest.cs
- TextBoxBase.cs
- MsmqChannelFactoryBase.cs
- DbMetaDataColumnNames.cs
- CharacterHit.cs
- BitmapSourceSafeMILHandle.cs
- ThreadExceptionEvent.cs
- MemberDomainMap.cs
- COM2DataTypeToManagedDataTypeConverter.cs
- ToolStripSeparatorRenderEventArgs.cs
- PathSegment.cs
- SamlSerializer.cs
- HtmlTableRow.cs
- MenuCommandService.cs
- TextSelectionHighlightLayer.cs
- ZoneButton.cs
- CatalogPartCollection.cs
- ScrollContentPresenter.cs
- regiisutil.cs
- DropTarget.cs
- ObjectDisposedException.cs