Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / Documents / Serialization / SerializerProvider.cs / 1 / SerializerProvider.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Manages plug-in document serializers // // See spec at// // History: // 07/16/2005 : oliverfo - Created // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.IO; using System.Reflection; using System.Security; using System.Security.Permissions; using System.Windows.Xps.Serialization; using System.Diagnostics.CodeAnalysis; using Microsoft.Win32; using MS.Internal.PresentationFramework; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows.Documents.Serialization { /// /// SerializerProvider enumerates plug-in serializers /// public sealed class SerializerProvider { #region Constructors ////// creates a SerializerProvider /// The constructor accesses the registry to find all installed plug-ins /// ////// Create a SerializerProvider listing all installed serializers (from the registry) /// /// This method currently requires full trust to run. /// ////// The DemandPlugInSerializerPermissions() ensures that this method only works in full trust. /// Full trust is required, so that partial trust applications do not load or use potentially /// unsafe serializer plug ins /// public SerializerProvider() { SecurityHelper.DemandPlugInSerializerPermissions(); SerializerDescriptor sd = null; ListinstalledSerializers = new List (); sd = CreateSystemSerializerDescriptor(); if (sd != null) { installedSerializers.Add(sd); } RegistryKey plugIns = _rootKey.CreateSubKey(_registryPath); if ( plugIns != null ) { foreach ( string keyName in plugIns.GetSubKeyNames()) { sd = SerializerDescriptor.CreateFromRegistry(plugIns, keyName); if (sd != null) { installedSerializers.Add(sd); } } plugIns.Close(); } _installedSerializers = installedSerializers.AsReadOnly(); } #endregion #region Public Methods /// /// Registers the serializer plug-in identified by serializerDescriptor in the registry /// ////// The DemandPlugInSerializerPermissions() ensures that this method only works in full trust. /// Full trust is required, so that partial trust applications install /// potentially unsafe serializer plug ins /// public static void RegisterSerializer(SerializerDescriptor serializerDescriptor, bool overwrite) { SecurityHelper.DemandPlugInSerializerPermissions(); if (serializerDescriptor == null) { throw new ArgumentNullException("serializerDescriptor"); } RegistryKey plugIns = _rootKey.CreateSubKey(_registryPath); string serializerKey = serializerDescriptor.DisplayName + "/" + serializerDescriptor.AssemblyName + "/" + serializerDescriptor.AssemblyVersion + "/" + serializerDescriptor.WinFXVersion; if (!overwrite && plugIns.OpenSubKey(serializerKey) != null) { throw new ArgumentException(SR.Get(SRID.SerializerProviderAlreadyRegistered), serializerKey); } RegistryKey newPlugIn = plugIns.CreateSubKey(serializerKey); serializerDescriptor.WriteToRegistryKey(newPlugIn); newPlugIn.Close(); } ////// Un-Registers the serializer plug-in identified by serializerDescriptor in the registry /// ////// Removes a previously installed plug-n serialiazer from the registry /// /// This method currently requires full trust to run. /// ////// The DemandPlugInSerializerPermissions() ensures that this method only works in full trust. /// Full trust is required, so that partial trust applications do not uninstall /// serializer plug ins /// public static void UnregisterSerializer(SerializerDescriptor serializerDescriptor) { SecurityHelper.DemandPlugInSerializerPermissions(); if (serializerDescriptor == null) { throw new ArgumentNullException("serializerDescriptor"); } RegistryKey plugIns = _rootKey.CreateSubKey(_registryPath); string serializerKey = serializerDescriptor.DisplayName + "/" + serializerDescriptor.AssemblyName + "/" + serializerDescriptor.AssemblyVersion + "/" + serializerDescriptor.WinFXVersion; if (plugIns.OpenSubKey(serializerKey) == null) { throw new ArgumentException(SR.Get(SRID.SerializerProviderNotRegistered), serializerKey); } plugIns.DeleteSubKeyTree(serializerKey); } ////// Create a SerializerWriter identified by the passed in SerializerDescriptor on the passed in stream /// ////// With a SerializerProvider (which requires full trust to ctor) and a SerializerDescriptor (which requires /// full trust to obtain) create a SerializerWriter /// /// This method currently requires full trust to run. /// ////// The DemandPlugInSerializerPermissions() ensures that this method only works in full trust. /// Full trust is required, so that partial trust applications do not load or use potentially /// unsafe serializer plug ins /// public SerializerWriter CreateSerializerWriter(SerializerDescriptor serializerDescriptor, Stream stream) { SecurityHelper.DemandPlugInSerializerPermissions(); SerializerWriter serializerWriter = null; if (serializerDescriptor == null) { throw new ArgumentNullException("serializerDescriptor"); } string serializerKey = serializerDescriptor.DisplayName + "/" + serializerDescriptor.AssemblyName + "/" + serializerDescriptor.AssemblyVersion + "/" + serializerDescriptor.WinFXVersion; if (!serializerDescriptor.IsLoadable) { throw new ArgumentException(SR.Get(SRID.SerializerProviderWrongVersion), serializerKey); } if (stream == null) { throw new ArgumentNullException("stream"); } bool found = false; foreach (SerializerDescriptor sd in InstalledSerializers) { if (sd.Equals(serializerDescriptor)) { found = true; break; } } if (!found) { throw new ArgumentException(SR.Get(SRID.SerializerProviderUnknownSerializer), serializerKey); } try { ISerializerFactory factory = serializerDescriptor.CreateSerializerFactory(); serializerWriter = factory.CreateSerializerWriter(stream); } catch (FileNotFoundException) { throw new ArgumentException(SR.Get(SRID.SerializerProviderCannotLoad), serializerDescriptor.DisplayName); } catch (FileLoadException) { throw new ArgumentException(SR.Get(SRID.SerializerProviderCannotLoad), serializerDescriptor.DisplayName); } catch (BadImageFormatException) { throw new ArgumentException(SR.Get(SRID.SerializerProviderCannotLoad), serializerDescriptor.DisplayName); } catch (MissingMethodException) { throw new ArgumentException(SR.Get(SRID.SerializerProviderCannotLoad), serializerDescriptor.DisplayName); } return serializerWriter; } #endregion #region Private Methods ////// Uses reflection to create the XpsSerializer /// ////// Creates the Xps default serializer /// /// This method currently requires full trust to run. /// ////// Critical: XpsSerializerFactory..ctor is defined in a non-APTCA assembly. /// TreatAsSafe: demands appropriate permissions. /// The DemandPlugInSerializerPermissions() ensures that this method only works in full trust. /// Full trust is required, so that partial trust applications do not load the ReachFramework.dll /// which does not the Aptca attribute set /// ///SerializerDescriptor for new serializer [SuppressMessage("Microsoft.Security", "CA2116:AptcaMethodsShouldOnlyCallAptcaMethods")] [SecurityCritical, SecurityTreatAsSafe] private SerializerDescriptor CreateSystemSerializerDescriptor() { SecurityHelper.DemandPlugInSerializerPermissions(); SerializerDescriptor serializerDescriptor = null; // The XpsSerializer (our default document serializer) is defined in ReachFramework.dll // But callers can only get here if the above demand succeeds, so they are already fully trusted serializerDescriptor = SerializerDescriptor.CreateFromFactoryInstance( new XpsSerializerFactory() ); return serializerDescriptor; } #endregion #region Public Properties ////// Returns collection of installed serializers /// public ReadOnlyCollectionInstalledSerializers { get { return _installedSerializers; } } #endregion #region Data private const string _registryPath = @"SOFTWARE\Microsoft\WinFX Serializers"; private static readonly RegistryKey _rootKey = Registry.LocalMachine; private ReadOnlyCollection _installedSerializers; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Manages plug-in document serializers // // See spec at// // History: // 07/16/2005 : oliverfo - Created // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.IO; using System.Reflection; using System.Security; using System.Security.Permissions; using System.Windows.Xps.Serialization; using System.Diagnostics.CodeAnalysis; using Microsoft.Win32; using MS.Internal.PresentationFramework; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows.Documents.Serialization { /// /// SerializerProvider enumerates plug-in serializers /// public sealed class SerializerProvider { #region Constructors ////// creates a SerializerProvider /// The constructor accesses the registry to find all installed plug-ins /// ////// Create a SerializerProvider listing all installed serializers (from the registry) /// /// This method currently requires full trust to run. /// ////// The DemandPlugInSerializerPermissions() ensures that this method only works in full trust. /// Full trust is required, so that partial trust applications do not load or use potentially /// unsafe serializer plug ins /// public SerializerProvider() { SecurityHelper.DemandPlugInSerializerPermissions(); SerializerDescriptor sd = null; ListinstalledSerializers = new List (); sd = CreateSystemSerializerDescriptor(); if (sd != null) { installedSerializers.Add(sd); } RegistryKey plugIns = _rootKey.CreateSubKey(_registryPath); if ( plugIns != null ) { foreach ( string keyName in plugIns.GetSubKeyNames()) { sd = SerializerDescriptor.CreateFromRegistry(plugIns, keyName); if (sd != null) { installedSerializers.Add(sd); } } plugIns.Close(); } _installedSerializers = installedSerializers.AsReadOnly(); } #endregion #region Public Methods /// /// Registers the serializer plug-in identified by serializerDescriptor in the registry /// ////// The DemandPlugInSerializerPermissions() ensures that this method only works in full trust. /// Full trust is required, so that partial trust applications install /// potentially unsafe serializer plug ins /// public static void RegisterSerializer(SerializerDescriptor serializerDescriptor, bool overwrite) { SecurityHelper.DemandPlugInSerializerPermissions(); if (serializerDescriptor == null) { throw new ArgumentNullException("serializerDescriptor"); } RegistryKey plugIns = _rootKey.CreateSubKey(_registryPath); string serializerKey = serializerDescriptor.DisplayName + "/" + serializerDescriptor.AssemblyName + "/" + serializerDescriptor.AssemblyVersion + "/" + serializerDescriptor.WinFXVersion; if (!overwrite && plugIns.OpenSubKey(serializerKey) != null) { throw new ArgumentException(SR.Get(SRID.SerializerProviderAlreadyRegistered), serializerKey); } RegistryKey newPlugIn = plugIns.CreateSubKey(serializerKey); serializerDescriptor.WriteToRegistryKey(newPlugIn); newPlugIn.Close(); } ////// Un-Registers the serializer plug-in identified by serializerDescriptor in the registry /// ////// Removes a previously installed plug-n serialiazer from the registry /// /// This method currently requires full trust to run. /// ////// The DemandPlugInSerializerPermissions() ensures that this method only works in full trust. /// Full trust is required, so that partial trust applications do not uninstall /// serializer plug ins /// public static void UnregisterSerializer(SerializerDescriptor serializerDescriptor) { SecurityHelper.DemandPlugInSerializerPermissions(); if (serializerDescriptor == null) { throw new ArgumentNullException("serializerDescriptor"); } RegistryKey plugIns = _rootKey.CreateSubKey(_registryPath); string serializerKey = serializerDescriptor.DisplayName + "/" + serializerDescriptor.AssemblyName + "/" + serializerDescriptor.AssemblyVersion + "/" + serializerDescriptor.WinFXVersion; if (plugIns.OpenSubKey(serializerKey) == null) { throw new ArgumentException(SR.Get(SRID.SerializerProviderNotRegistered), serializerKey); } plugIns.DeleteSubKeyTree(serializerKey); } ////// Create a SerializerWriter identified by the passed in SerializerDescriptor on the passed in stream /// ////// With a SerializerProvider (which requires full trust to ctor) and a SerializerDescriptor (which requires /// full trust to obtain) create a SerializerWriter /// /// This method currently requires full trust to run. /// ////// The DemandPlugInSerializerPermissions() ensures that this method only works in full trust. /// Full trust is required, so that partial trust applications do not load or use potentially /// unsafe serializer plug ins /// public SerializerWriter CreateSerializerWriter(SerializerDescriptor serializerDescriptor, Stream stream) { SecurityHelper.DemandPlugInSerializerPermissions(); SerializerWriter serializerWriter = null; if (serializerDescriptor == null) { throw new ArgumentNullException("serializerDescriptor"); } string serializerKey = serializerDescriptor.DisplayName + "/" + serializerDescriptor.AssemblyName + "/" + serializerDescriptor.AssemblyVersion + "/" + serializerDescriptor.WinFXVersion; if (!serializerDescriptor.IsLoadable) { throw new ArgumentException(SR.Get(SRID.SerializerProviderWrongVersion), serializerKey); } if (stream == null) { throw new ArgumentNullException("stream"); } bool found = false; foreach (SerializerDescriptor sd in InstalledSerializers) { if (sd.Equals(serializerDescriptor)) { found = true; break; } } if (!found) { throw new ArgumentException(SR.Get(SRID.SerializerProviderUnknownSerializer), serializerKey); } try { ISerializerFactory factory = serializerDescriptor.CreateSerializerFactory(); serializerWriter = factory.CreateSerializerWriter(stream); } catch (FileNotFoundException) { throw new ArgumentException(SR.Get(SRID.SerializerProviderCannotLoad), serializerDescriptor.DisplayName); } catch (FileLoadException) { throw new ArgumentException(SR.Get(SRID.SerializerProviderCannotLoad), serializerDescriptor.DisplayName); } catch (BadImageFormatException) { throw new ArgumentException(SR.Get(SRID.SerializerProviderCannotLoad), serializerDescriptor.DisplayName); } catch (MissingMethodException) { throw new ArgumentException(SR.Get(SRID.SerializerProviderCannotLoad), serializerDescriptor.DisplayName); } return serializerWriter; } #endregion #region Private Methods ////// Uses reflection to create the XpsSerializer /// ////// Creates the Xps default serializer /// /// This method currently requires full trust to run. /// ////// Critical: XpsSerializerFactory..ctor is defined in a non-APTCA assembly. /// TreatAsSafe: demands appropriate permissions. /// The DemandPlugInSerializerPermissions() ensures that this method only works in full trust. /// Full trust is required, so that partial trust applications do not load the ReachFramework.dll /// which does not the Aptca attribute set /// ///SerializerDescriptor for new serializer [SuppressMessage("Microsoft.Security", "CA2116:AptcaMethodsShouldOnlyCallAptcaMethods")] [SecurityCritical, SecurityTreatAsSafe] private SerializerDescriptor CreateSystemSerializerDescriptor() { SecurityHelper.DemandPlugInSerializerPermissions(); SerializerDescriptor serializerDescriptor = null; // The XpsSerializer (our default document serializer) is defined in ReachFramework.dll // But callers can only get here if the above demand succeeds, so they are already fully trusted serializerDescriptor = SerializerDescriptor.CreateFromFactoryInstance( new XpsSerializerFactory() ); return serializerDescriptor; } #endregion #region Public Properties ////// Returns collection of installed serializers /// public ReadOnlyCollectionInstalledSerializers { get { return _installedSerializers; } } #endregion #region Data private const string _registryPath = @"SOFTWARE\Microsoft\WinFX Serializers"; private static readonly RegistryKey _rootKey = Registry.LocalMachine; private ReadOnlyCollection _installedSerializers; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
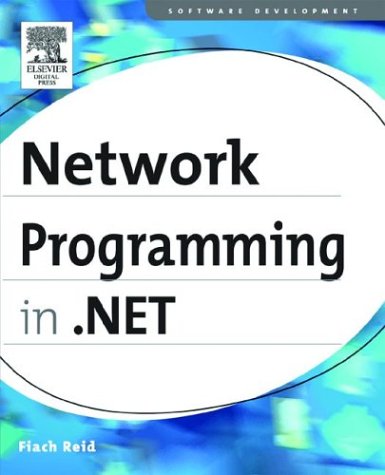
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CultureTableRecord.cs
- DropShadowBitmapEffect.cs
- IndexExpression.cs
- XmlBaseWriter.cs
- EastAsianLunisolarCalendar.cs
- PatternMatcher.cs
- SettingsPropertyCollection.cs
- DataSetMappper.cs
- BaseDataListActionList.cs
- DebuggerAttributes.cs
- RelationshipManager.cs
- HttpFileCollection.cs
- DSACryptoServiceProvider.cs
- StringReader.cs
- MatrixTransform3D.cs
- XmlSchemaDatatype.cs
- MimeBasePart.cs
- SiteOfOriginContainer.cs
- Normalization.cs
- ChildTable.cs
- WebPartTracker.cs
- DataGridViewImageColumn.cs
- OdbcConnectionString.cs
- ScriptServiceAttribute.cs
- SourceFileInfo.cs
- XmlReflectionMember.cs
- recordstate.cs
- VisualCollection.cs
- TlsnegoTokenProvider.cs
- ScriptingAuthenticationServiceSection.cs
- InvalidContentTypeException.cs
- XmlMemberMapping.cs
- TreeViewItem.cs
- TargetInvocationException.cs
- MissingFieldException.cs
- SafeIUnknown.cs
- DirectoryGroupQuery.cs
- XmlUnspecifiedAttribute.cs
- ItemsChangedEventArgs.cs
- ToolStripGrip.cs
- WorkflowTransactionService.cs
- BasicCommandTreeVisitor.cs
- CqlBlock.cs
- FixedSOMTable.cs
- HttpResponse.cs
- DoubleLinkList.cs
- RawKeyboardInputReport.cs
- ResourceDisplayNameAttribute.cs
- recordstatefactory.cs
- DesignTimeSiteMapProvider.cs
- TimerElapsedEvenArgs.cs
- BackEase.cs
- ParseHttpDate.cs
- ProcessHostConfigUtils.cs
- TraceUtility.cs
- MasterPageBuildProvider.cs
- DataView.cs
- Char.cs
- RegionData.cs
- XamlPoint3DCollectionSerializer.cs
- GridViewPageEventArgs.cs
- XmlSchemaFacet.cs
- MSAAWinEventWrap.cs
- MemberProjectionIndex.cs
- MarkerProperties.cs
- DatasetMethodGenerator.cs
- DayRenderEvent.cs
- StyleXamlTreeBuilder.cs
- VirtualPath.cs
- XmlCharCheckingReader.cs
- ConfigurationLocation.cs
- BasePattern.cs
- GridViewCellAutomationPeer.cs
- EraserBehavior.cs
- ExpressionValueEditor.cs
- HyperlinkAutomationPeer.cs
- Substitution.cs
- _HeaderInfo.cs
- FieldAccessException.cs
- DataGridColumnHeadersPresenterAutomationPeer.cs
- SoapAttributeAttribute.cs
- SyndicationDeserializer.cs
- SystemPens.cs
- AppDomainEvidenceFactory.cs
- ClientSettingsProvider.cs
- CodeAttachEventStatement.cs
- XmlUrlResolver.cs
- NavigateUrlConverter.cs
- HttpCookieCollection.cs
- HelpInfo.cs
- DeviceContexts.cs
- StylusEditingBehavior.cs
- EndpointConfigContainer.cs
- DynamicPropertyReader.cs
- DetailsViewPageEventArgs.cs
- ProjectionPathBuilder.cs
- SQLByte.cs
- FileEnumerator.cs
- FileAccessException.cs
- MenuCommands.cs