Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / WebParts / WebPartTracker.cs / 1 / WebPartTracker.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls.WebParts { using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Drawing.Design; using System.Security.Permissions; using System.Web; using System.Web.UI; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class WebPartTracker : IDisposable { private bool _disposed; private WebPart _webPart; private ProviderConnectionPoint _providerConnectionPoint; public WebPartTracker(WebPart webPart, ProviderConnectionPoint providerConnectionPoint) { if (webPart == null) { throw new ArgumentNullException("webPart"); } if (providerConnectionPoint == null) { throw new ArgumentNullException("providerConnectionPoint"); } if (providerConnectionPoint.ControlType != webPart.GetType()) { throw new ArgumentException(SR.GetString(SR.WebPartManager_InvalidConnectionPoint), "providerConnectionPoint"); } _webPart = webPart; _providerConnectionPoint = providerConnectionPoint; if (++Count > 1) { webPart.SetConnectErrorMessage(SR.GetString( SR.WebPartTracker_CircularConnection, _providerConnectionPoint.DisplayName)); } } public bool IsCircularConnection { get { return (Count > 1); } } private int Count { get { int count; _webPart.TrackerCounter.TryGetValue(_providerConnectionPoint, out count); return count; } set { _webPart.TrackerCounter[_providerConnectionPoint] = value; } } void IDisposable.Dispose() { if (!_disposed) { Debug.Assert(Count >= 1); Count--; _disposed = true; } } } }
Link Menu
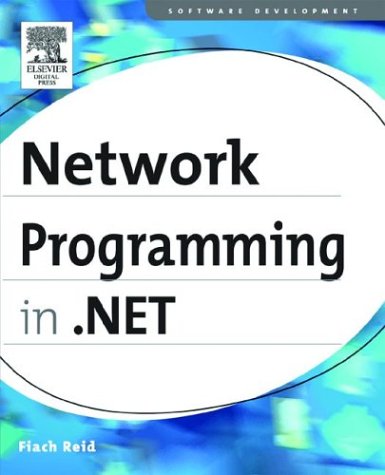
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- QueryLifecycle.cs
- SimpleType.cs
- BmpBitmapEncoder.cs
- AsyncResult.cs
- InitializerFacet.cs
- UserPreferenceChangingEventArgs.cs
- TypeToken.cs
- StructuredType.cs
- TypeBuilderInstantiation.cs
- BasicHttpSecurityMode.cs
- FixedSchema.cs
- ActivityPropertyReference.cs
- EntityContainer.cs
- PickBranch.cs
- IBuiltInEvidence.cs
- BufferedGraphicsManager.cs
- GenericParameterDataContract.cs
- NavigationService.cs
- AsmxEndpointPickerExtension.cs
- HtmlImage.cs
- FlatButtonAppearance.cs
- DynamicPropertyReader.cs
- FixedLineResult.cs
- ConfigurationValue.cs
- BuildProvidersCompiler.cs
- MinimizableAttributeTypeConverter.cs
- ProcessThreadDesigner.cs
- WindowsScroll.cs
- XmlCharacterData.cs
- PngBitmapDecoder.cs
- JsonWriter.cs
- ComNativeDescriptor.cs
- ContentElementAutomationPeer.cs
- IdentifierService.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- ScriptIgnoreAttribute.cs
- HwndKeyboardInputProvider.cs
- EdmProviderManifest.cs
- DataSysAttribute.cs
- CodeParameterDeclarationExpressionCollection.cs
- CapabilitiesPattern.cs
- QilXmlReader.cs
- InvalidCommandTreeException.cs
- Padding.cs
- ExecutionContext.cs
- DispatcherFrame.cs
- LocationUpdates.cs
- BitmapFrame.cs
- ActivityExecutorOperation.cs
- BitmapEffect.cs
- DataGridItemEventArgs.cs
- DataProtection.cs
- ProvidersHelper.cs
- AdRotator.cs
- TypeResolver.cs
- FontEditor.cs
- DataObject.cs
- ImageIndexConverter.cs
- HttpClientCertificate.cs
- GeometryDrawing.cs
- CatalogPartChrome.cs
- ListViewItem.cs
- XsdDateTime.cs
- SpnegoTokenProvider.cs
- Separator.cs
- mactripleDES.cs
- InstanceKeyView.cs
- BaseDataList.cs
- Funcletizer.cs
- Automation.cs
- LocalFileSettingsProvider.cs
- ImageFormat.cs
- COM2Enum.cs
- PeerCollaborationPermission.cs
- ControlEvent.cs
- _SpnDictionary.cs
- MarkupCompilePass1.cs
- DataPagerFieldCollection.cs
- Metadata.cs
- DetailsViewRow.cs
- SrgsNameValueTag.cs
- ReturnType.cs
- TypeCodeDomSerializer.cs
- SqlXml.cs
- FileReservationCollection.cs
- TypeForwardedToAttribute.cs
- ApplicationFileCodeDomTreeGenerator.cs
- SoapHeaderAttribute.cs
- WebBodyFormatMessageProperty.cs
- DataServiceRequestOfT.cs
- DesignerActionItemCollection.cs
- SrgsRulesCollection.cs
- SimpleNameService.cs
- LineGeometry.cs
- WindowVisualStateTracker.cs
- ObfuscationAttribute.cs
- EncryptedData.cs
- PolyQuadraticBezierSegment.cs
- userdatakeys.cs
- DialogResultConverter.cs