Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / QueryResponse.cs / 1 / QueryResponse.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Response to a batched query. // //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Collections; using System.Collections.Generic; using System.Reflection; ////// Response to a batched query. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1010", Justification = "required for this feature")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1710", Justification = "required for this feature")] public class QueryOperationResponse : OperationResponse, System.Collections.IEnumerable { ///Original query private DataServiceRequest query; ///Enumerable of objects in query private System.Collections.IEnumerable results; ////// constructor /// /// HTTP headers /// original query /// retrieved objects internal QueryOperationResponse(Dictionaryheaders, DataServiceRequest query, System.Collections.IEnumerable results) : base(headers) { this.query = query; this.results = results; } /// The query that executed within the batch. public DataServiceRequest Query { get { return this.query; } } ///get a non-null enumerable of the result internal System.Collections.IEnumerable Results { get { if (null != this.Error) { throw System.Data.Services.Client.Error.InvalidOperation(Strings.Context_BatchExecuteError, this.Error); } return this.results ?? Array.CreateInstance(this.query.ElementType, 0); } } ///Results from a query ///enumerator of objects in query public System.Collections.IEnumerator GetEnumerator() { return this.Results.GetEnumerator(); } ////// Creates a generic instance of the QueryOperationResponse and return it /// /// generic type for the QueryOperationResponse. /// constructor parameter1 /// constructor parameter2 /// constructor parameter3 ///returns a new strongly typed instance of QueryOperationResponse. internal static QueryOperationResponse GetInstance(Type elementType, Dictionaryheaders, DataServiceRequest query, IEnumerable results) { Type genericType = typeof(QueryOperationResponse<>).MakeGenericType(elementType); return (QueryOperationResponse)Activator.CreateInstance( genericType, BindingFlags.CreateInstance | BindingFlags.NonPublic | BindingFlags.Instance, null, new object[] { headers, query, results }, System.Globalization.CultureInfo.InvariantCulture); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // //// Response to a batched query. // //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Collections; using System.Collections.Generic; using System.Reflection; ////// Response to a batched query. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1010", Justification = "required for this feature")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1710", Justification = "required for this feature")] public class QueryOperationResponse : OperationResponse, System.Collections.IEnumerable { ///Original query private DataServiceRequest query; ///Enumerable of objects in query private System.Collections.IEnumerable results; ////// constructor /// /// HTTP headers /// original query /// retrieved objects internal QueryOperationResponse(Dictionaryheaders, DataServiceRequest query, System.Collections.IEnumerable results) : base(headers) { this.query = query; this.results = results; } /// The query that executed within the batch. public DataServiceRequest Query { get { return this.query; } } ///get a non-null enumerable of the result internal System.Collections.IEnumerable Results { get { if (null != this.Error) { throw System.Data.Services.Client.Error.InvalidOperation(Strings.Context_BatchExecuteError, this.Error); } return this.results ?? Array.CreateInstance(this.query.ElementType, 0); } } ///Results from a query ///enumerator of objects in query public System.Collections.IEnumerator GetEnumerator() { return this.Results.GetEnumerator(); } ////// Creates a generic instance of the QueryOperationResponse and return it /// /// generic type for the QueryOperationResponse. /// constructor parameter1 /// constructor parameter2 /// constructor parameter3 ///returns a new strongly typed instance of QueryOperationResponse. internal static QueryOperationResponse GetInstance(Type elementType, Dictionaryheaders, DataServiceRequest query, IEnumerable results) { Type genericType = typeof(QueryOperationResponse<>).MakeGenericType(elementType); return (QueryOperationResponse)Activator.CreateInstance( genericType, BindingFlags.CreateInstance | BindingFlags.NonPublic | BindingFlags.Instance, null, new object[] { headers, query, results }, System.Globalization.CultureInfo.InvariantCulture); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
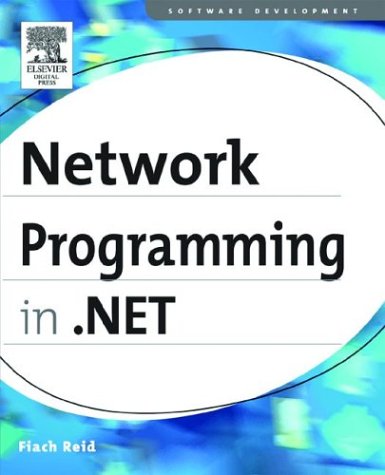
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MessageQueueTransaction.cs
- FileLoadException.cs
- IconBitmapDecoder.cs
- PersonalizationProviderHelper.cs
- DataGridCellAutomationPeer.cs
- SQLBytes.cs
- Vector3DKeyFrameCollection.cs
- RuntimeArgumentHandle.cs
- FormViewPagerRow.cs
- ObjectParameter.cs
- Compiler.cs
- XPathMultyIterator.cs
- SessionEndingEventArgs.cs
- CodeNamespace.cs
- TagNameToTypeMapper.cs
- WindowsSecurityTokenAuthenticator.cs
- ReliabilityContractAttribute.cs
- SystemInformation.cs
- PasswordTextNavigator.cs
- CircleEase.cs
- XslNumber.cs
- InvalidateEvent.cs
- AppDomainProtocolHandler.cs
- ProcessModuleCollection.cs
- DataSourceCache.cs
- EditorPartCollection.cs
- WebConvert.cs
- XmlWhitespace.cs
- Aes.cs
- StylusPointPropertyInfo.cs
- MouseBinding.cs
- SettingsPropertyCollection.cs
- EntityClientCacheKey.cs
- Point3DAnimationBase.cs
- DataKeyArray.cs
- ChannelCredentials.cs
- HashCryptoHandle.cs
- UInt32Storage.cs
- WebScriptEnablingElement.cs
- PropertyValueUIItem.cs
- mongolianshape.cs
- InstanceStoreQueryResult.cs
- DesignerDataTableBase.cs
- TargetInvocationException.cs
- XmlLanguageConverter.cs
- ModelUIElement3D.cs
- InvokeHandlers.cs
- UnconditionalPolicy.cs
- SecurityPolicySection.cs
- ISAPIApplicationHost.cs
- ColumnHeader.cs
- TabControlDesigner.cs
- DataTemplateSelector.cs
- RedistVersionInfo.cs
- SBCSCodePageEncoding.cs
- SiteMapNodeCollection.cs
- TextElementEditingBehaviorAttribute.cs
- SmtpNegotiateAuthenticationModule.cs
- ParseHttpDate.cs
- Quaternion.cs
- glyphs.cs
- CardSpaceException.cs
- ChildrenQuery.cs
- Schema.cs
- StyleSheet.cs
- ClassHandlersStore.cs
- SpotLight.cs
- TraceUtility.cs
- HealthMonitoringSection.cs
- ReadOnlyTernaryTree.cs
- WebPartPersonalization.cs
- PtsHelper.cs
- WmlSelectionListAdapter.cs
- SmtpSection.cs
- ParameterCollection.cs
- EncryptedKey.cs
- connectionpool.cs
- XmlDataSourceView.cs
- StateChangeEvent.cs
- DocumentsTrace.cs
- IndicCharClassifier.cs
- DataServiceExpressionVisitor.cs
- RegisteredArrayDeclaration.cs
- GradientSpreadMethodValidation.cs
- HttpModule.cs
- MenuEventArgs.cs
- RuntimeConfigLKG.cs
- WpfKnownTypeInvoker.cs
- DesignerDataTableBase.cs
- Converter.cs
- SqlExpander.cs
- DataGridViewCellLinkedList.cs
- CheckoutException.cs
- ObjectListShowCommandsEventArgs.cs
- CodeSnippetStatement.cs
- SerializerWriterEventHandlers.cs
- HtmlElementCollection.cs
- Compiler.cs
- ManagementEventArgs.cs
- X509CertificateValidator.cs