Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntityDesign / Design / System / Data / Entity / Design / SSDLGenerator / TableDetailsCollection.cs / 4 / TableDetailsCollection.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Xml; using System.Data.Common; using System.Globalization; using System.Data; namespace System.Data.Entity.Design.SsdlGenerator { ////// Strongly typed DataTable for TableDetails /// [Serializable] internal sealed class TableDetailsCollection : System.Data.DataTable, System.Collections.IEnumerable { [NonSerialized] private System.Data.DataColumn _columnCatalog; [NonSerialized] private System.Data.DataColumn _columnSchema; [NonSerialized] private System.Data.DataColumn _columnTable; [NonSerialized] private System.Data.DataColumn _columnFieldColumn; [NonSerialized] private System.Data.DataColumn _columnIsNullable; [NonSerialized] private System.Data.DataColumn _columnDataType; [NonSerialized] private System.Data.DataColumn _columnMaximumLength; [NonSerialized] private System.Data.DataColumn _columnDateTimePrecision; [NonSerialized] private System.Data.DataColumn _columnPrecision; [NonSerialized] private System.Data.DataColumn _columnScale; [NonSerialized] private System.Data.DataColumn _columnIsIdentity; [NonSerialized] private System.Data.DataColumn _columnIsServerGenerated; [NonSerialized] private System.Data.DataColumn _columnIsPrimaryKey; ////// Constructs a TableDetailsDataTable /// public TableDetailsCollection() { this.TableName = "TableDetails"; // had to remove these because of an fxcop violation //BeginInit(); InitClass(); //EndInit(); } ////// Constructs a new instance TableDetailsDataTable with a given SerializationInfo and StreamingContext /// /// /// internal TableDetailsCollection(System.Runtime.Serialization.SerializationInfo serializationInfo, System.Runtime.Serialization.StreamingContext streamingContext) : base(serializationInfo, streamingContext) { UpdateMemberFieldsAfterDeserialization(); } ////// Gets the Catalog column /// public System.Data.DataColumn CatalogColumn { get { return this._columnCatalog; } } ////// Gets the Schema column /// public System.Data.DataColumn SchemaColumn { get { return this._columnSchema; } } ////// Gets the TableName column /// public System.Data.DataColumn TableNameColumn { get { return this._columnTable; } } ////// Gets the ColumnName column /// public System.Data.DataColumn ColumnNameColumn { get { return this._columnFieldColumn; } } ////// Gets the IsNullable column /// public System.Data.DataColumn IsNullableColumn { get { return this._columnIsNullable; } } ////// Gets the DataType column /// public System.Data.DataColumn DataTypeColumn { get { return this._columnDataType; } } ////// Gets the MaximumLength column /// public System.Data.DataColumn MaximumLengthColumn { get { return this._columnMaximumLength; } } ////// Gets the Precision column /// public System.Data.DataColumn PrecisionColumn { get { return this._columnPrecision; } } ////// Gets the Precision column /// public System.Data.DataColumn DateTimePrecisionColumn { get { return this._columnDateTimePrecision; } } ////// Gets the Scale column /// public System.Data.DataColumn ScaleColumn { get { return this._columnScale; } } ////// Gets the IsIdentityColumn column /// public System.Data.DataColumn IsIdentityColumn { get { return this._columnIsIdentity; } } ////// Gets the IsIdentityColumn column /// public System.Data.DataColumn IsServerGeneratedColumn { get { return this._columnIsServerGenerated; } } ////// Gets the IsPrimaryKey column /// public System.Data.DataColumn IsPrimaryKeyColumn { get { return this._columnIsPrimaryKey; } } ////// Gets an enumerator over the rows. /// ///The row enumerator public System.Collections.IEnumerator GetEnumerator() { return this.Rows.GetEnumerator(); } ////// Creates an instance of this table /// ///The newly created instance. protected override System.Data.DataTable CreateInstance() { return new TableDetailsCollection(); } private const string CATALOG = "CatalogName"; private const string SCHEMA = "SchemaName"; private const string TABLE = "TableName"; private const string COLUMN = "ColumnName"; private const string ORDINAL = "Ordinal"; private const string IS_NULLABLE = "IsNullable"; private const string DATA_TYPE = "DataType"; private const string MAX_LENGTH = "MaximumLength"; private const string PRECISION = "Precision"; private const string DATETIMEPRECISION = "DateTimePrecision"; private const string SCALE = "Scale"; private const string IS_IDENTITY = "IsIdentity"; private const string IS_SERVERGENERATED = "IsServerGenerated"; private const string IS_PRIMARYKEY = "IsPrimaryKey"; private void InitClass() { this._columnCatalog = new System.Data.DataColumn(CATALOG, typeof(string), null, System.Data.MappingType.Element); base.Columns.Add(this._columnCatalog); this._columnSchema = new System.Data.DataColumn(SCHEMA, typeof(string), null, System.Data.MappingType.Element); base.Columns.Add(this._columnSchema); this._columnTable = new System.Data.DataColumn(TABLE, typeof(string), null, System.Data.MappingType.Element); base.Columns.Add(this._columnTable); this._columnFieldColumn = new System.Data.DataColumn(COLUMN, typeof(string), null, System.Data.MappingType.Element); base.Columns.Add(this._columnFieldColumn); this._columnIsNullable = new System.Data.DataColumn(IS_NULLABLE, typeof(bool), null, System.Data.MappingType.Element); base.Columns.Add(this._columnIsNullable); this._columnDataType = new System.Data.DataColumn(DATA_TYPE, typeof(string), null, System.Data.MappingType.Element); base.Columns.Add(this._columnDataType); this._columnMaximumLength = new System.Data.DataColumn(MAX_LENGTH, typeof(int), null, System.Data.MappingType.Element); base.Columns.Add(this._columnMaximumLength); this._columnPrecision = new System.Data.DataColumn(PRECISION, typeof(int), null, System.Data.MappingType.Element); base.Columns.Add(this._columnPrecision); this._columnDateTimePrecision = new System.Data.DataColumn(DATETIMEPRECISION, typeof(int), null, System.Data.MappingType.Element); base.Columns.Add(this._columnDateTimePrecision); this._columnScale = new System.Data.DataColumn(SCALE, typeof(int), null, System.Data.MappingType.Element); base.Columns.Add(this._columnScale); this._columnIsIdentity = new System.Data.DataColumn(IS_IDENTITY, typeof(bool), null, System.Data.MappingType.Element); base.Columns.Add(this._columnIsIdentity); this._columnIsServerGenerated = new System.Data.DataColumn(IS_SERVERGENERATED, typeof(bool), null, System.Data.MappingType.Element); base.Columns.Add(this._columnIsServerGenerated); this._columnIsPrimaryKey = new System.Data.DataColumn(IS_PRIMARYKEY, typeof(bool), null, System.Data.MappingType.Element); base.Columns.Add(this._columnIsPrimaryKey); } private void UpdateMemberFieldsAfterDeserialization() { this._columnCatalog = base.Columns[CATALOG]; this._columnSchema = base.Columns[SCHEMA]; this._columnTable = base.Columns[TABLE]; this._columnFieldColumn = base.Columns[COLUMN]; this._columnIsNullable = base.Columns[IS_NULLABLE]; this._columnDataType = base.Columns[DATA_TYPE]; this._columnMaximumLength = base.Columns[MAX_LENGTH]; this._columnPrecision = base.Columns[PRECISION]; this._columnDateTimePrecision = base.Columns[DATETIMEPRECISION]; this._columnScale = base.Columns[SCALE]; this._columnIsIdentity = base.Columns[IS_IDENTITY]; this._columnIsServerGenerated = base.Columns[IS_SERVERGENERATED]; this._columnIsPrimaryKey = base.Columns[IS_PRIMARYKEY]; } ////// Create a new row from a DataRowBuilder object. /// /// The builder to create the row from. ///The row that was created. protected override System.Data.DataRow NewRowFromBuilder(System.Data.DataRowBuilder builder) { return new TableDetailsRow(builder); } ////// Gets the Type that this row is. /// ///The type of this row. protected override System.Type GetRowType() { return typeof(TableDetailsRow); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Xml; using System.Data.Common; using System.Globalization; using System.Data; namespace System.Data.Entity.Design.SsdlGenerator { ////// Strongly typed DataTable for TableDetails /// [Serializable] internal sealed class TableDetailsCollection : System.Data.DataTable, System.Collections.IEnumerable { [NonSerialized] private System.Data.DataColumn _columnCatalog; [NonSerialized] private System.Data.DataColumn _columnSchema; [NonSerialized] private System.Data.DataColumn _columnTable; [NonSerialized] private System.Data.DataColumn _columnFieldColumn; [NonSerialized] private System.Data.DataColumn _columnIsNullable; [NonSerialized] private System.Data.DataColumn _columnDataType; [NonSerialized] private System.Data.DataColumn _columnMaximumLength; [NonSerialized] private System.Data.DataColumn _columnDateTimePrecision; [NonSerialized] private System.Data.DataColumn _columnPrecision; [NonSerialized] private System.Data.DataColumn _columnScale; [NonSerialized] private System.Data.DataColumn _columnIsIdentity; [NonSerialized] private System.Data.DataColumn _columnIsServerGenerated; [NonSerialized] private System.Data.DataColumn _columnIsPrimaryKey; ////// Constructs a TableDetailsDataTable /// public TableDetailsCollection() { this.TableName = "TableDetails"; // had to remove these because of an fxcop violation //BeginInit(); InitClass(); //EndInit(); } ////// Constructs a new instance TableDetailsDataTable with a given SerializationInfo and StreamingContext /// /// /// internal TableDetailsCollection(System.Runtime.Serialization.SerializationInfo serializationInfo, System.Runtime.Serialization.StreamingContext streamingContext) : base(serializationInfo, streamingContext) { UpdateMemberFieldsAfterDeserialization(); } ////// Gets the Catalog column /// public System.Data.DataColumn CatalogColumn { get { return this._columnCatalog; } } ////// Gets the Schema column /// public System.Data.DataColumn SchemaColumn { get { return this._columnSchema; } } ////// Gets the TableName column /// public System.Data.DataColumn TableNameColumn { get { return this._columnTable; } } ////// Gets the ColumnName column /// public System.Data.DataColumn ColumnNameColumn { get { return this._columnFieldColumn; } } ////// Gets the IsNullable column /// public System.Data.DataColumn IsNullableColumn { get { return this._columnIsNullable; } } ////// Gets the DataType column /// public System.Data.DataColumn DataTypeColumn { get { return this._columnDataType; } } ////// Gets the MaximumLength column /// public System.Data.DataColumn MaximumLengthColumn { get { return this._columnMaximumLength; } } ////// Gets the Precision column /// public System.Data.DataColumn PrecisionColumn { get { return this._columnPrecision; } } ////// Gets the Precision column /// public System.Data.DataColumn DateTimePrecisionColumn { get { return this._columnDateTimePrecision; } } ////// Gets the Scale column /// public System.Data.DataColumn ScaleColumn { get { return this._columnScale; } } ////// Gets the IsIdentityColumn column /// public System.Data.DataColumn IsIdentityColumn { get { return this._columnIsIdentity; } } ////// Gets the IsIdentityColumn column /// public System.Data.DataColumn IsServerGeneratedColumn { get { return this._columnIsServerGenerated; } } ////// Gets the IsPrimaryKey column /// public System.Data.DataColumn IsPrimaryKeyColumn { get { return this._columnIsPrimaryKey; } } ////// Gets an enumerator over the rows. /// ///The row enumerator public System.Collections.IEnumerator GetEnumerator() { return this.Rows.GetEnumerator(); } ////// Creates an instance of this table /// ///The newly created instance. protected override System.Data.DataTable CreateInstance() { return new TableDetailsCollection(); } private const string CATALOG = "CatalogName"; private const string SCHEMA = "SchemaName"; private const string TABLE = "TableName"; private const string COLUMN = "ColumnName"; private const string ORDINAL = "Ordinal"; private const string IS_NULLABLE = "IsNullable"; private const string DATA_TYPE = "DataType"; private const string MAX_LENGTH = "MaximumLength"; private const string PRECISION = "Precision"; private const string DATETIMEPRECISION = "DateTimePrecision"; private const string SCALE = "Scale"; private const string IS_IDENTITY = "IsIdentity"; private const string IS_SERVERGENERATED = "IsServerGenerated"; private const string IS_PRIMARYKEY = "IsPrimaryKey"; private void InitClass() { this._columnCatalog = new System.Data.DataColumn(CATALOG, typeof(string), null, System.Data.MappingType.Element); base.Columns.Add(this._columnCatalog); this._columnSchema = new System.Data.DataColumn(SCHEMA, typeof(string), null, System.Data.MappingType.Element); base.Columns.Add(this._columnSchema); this._columnTable = new System.Data.DataColumn(TABLE, typeof(string), null, System.Data.MappingType.Element); base.Columns.Add(this._columnTable); this._columnFieldColumn = new System.Data.DataColumn(COLUMN, typeof(string), null, System.Data.MappingType.Element); base.Columns.Add(this._columnFieldColumn); this._columnIsNullable = new System.Data.DataColumn(IS_NULLABLE, typeof(bool), null, System.Data.MappingType.Element); base.Columns.Add(this._columnIsNullable); this._columnDataType = new System.Data.DataColumn(DATA_TYPE, typeof(string), null, System.Data.MappingType.Element); base.Columns.Add(this._columnDataType); this._columnMaximumLength = new System.Data.DataColumn(MAX_LENGTH, typeof(int), null, System.Data.MappingType.Element); base.Columns.Add(this._columnMaximumLength); this._columnPrecision = new System.Data.DataColumn(PRECISION, typeof(int), null, System.Data.MappingType.Element); base.Columns.Add(this._columnPrecision); this._columnDateTimePrecision = new System.Data.DataColumn(DATETIMEPRECISION, typeof(int), null, System.Data.MappingType.Element); base.Columns.Add(this._columnDateTimePrecision); this._columnScale = new System.Data.DataColumn(SCALE, typeof(int), null, System.Data.MappingType.Element); base.Columns.Add(this._columnScale); this._columnIsIdentity = new System.Data.DataColumn(IS_IDENTITY, typeof(bool), null, System.Data.MappingType.Element); base.Columns.Add(this._columnIsIdentity); this._columnIsServerGenerated = new System.Data.DataColumn(IS_SERVERGENERATED, typeof(bool), null, System.Data.MappingType.Element); base.Columns.Add(this._columnIsServerGenerated); this._columnIsPrimaryKey = new System.Data.DataColumn(IS_PRIMARYKEY, typeof(bool), null, System.Data.MappingType.Element); base.Columns.Add(this._columnIsPrimaryKey); } private void UpdateMemberFieldsAfterDeserialization() { this._columnCatalog = base.Columns[CATALOG]; this._columnSchema = base.Columns[SCHEMA]; this._columnTable = base.Columns[TABLE]; this._columnFieldColumn = base.Columns[COLUMN]; this._columnIsNullable = base.Columns[IS_NULLABLE]; this._columnDataType = base.Columns[DATA_TYPE]; this._columnMaximumLength = base.Columns[MAX_LENGTH]; this._columnPrecision = base.Columns[PRECISION]; this._columnDateTimePrecision = base.Columns[DATETIMEPRECISION]; this._columnScale = base.Columns[SCALE]; this._columnIsIdentity = base.Columns[IS_IDENTITY]; this._columnIsServerGenerated = base.Columns[IS_SERVERGENERATED]; this._columnIsPrimaryKey = base.Columns[IS_PRIMARYKEY]; } ////// Create a new row from a DataRowBuilder object. /// /// The builder to create the row from. ///The row that was created. protected override System.Data.DataRow NewRowFromBuilder(System.Data.DataRowBuilder builder) { return new TableDetailsRow(builder); } ////// Gets the Type that this row is. /// ///The type of this row. protected override System.Type GetRowType() { return typeof(TableDetailsRow); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
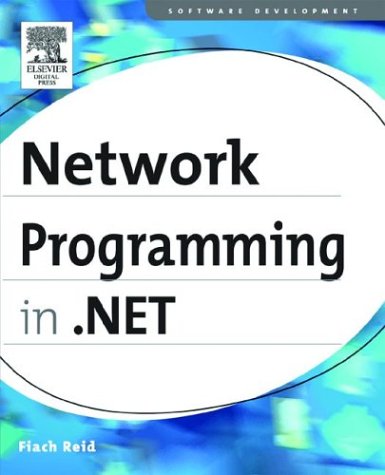
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FrameAutomationPeer.cs
- SynchronizationLockException.cs
- GeometryHitTestResult.cs
- TrackingWorkflowEventArgs.cs
- Missing.cs
- OdbcStatementHandle.cs
- IndexedGlyphRun.cs
- SchemaNames.cs
- BaseTypeViewSchema.cs
- UniformGrid.cs
- ObjectHandle.cs
- ActivityExecutorOperation.cs
- UriGenerator.cs
- DataGridViewCellMouseEventArgs.cs
- CompositeActivityDesigner.cs
- EmptyImpersonationContext.cs
- AspNetHostingPermission.cs
- MonthChangedEventArgs.cs
- SafeLibraryHandle.cs
- ALinqExpressionVisitor.cs
- DispatchChannelSink.cs
- EnterpriseServicesHelper.cs
- TypeGeneratedEventArgs.cs
- BitmapCodecInfoInternal.cs
- RightNameExpirationInfoPair.cs
- TeredoHelper.cs
- MonitoringDescriptionAttribute.cs
- _NestedMultipleAsyncResult.cs
- NotFiniteNumberException.cs
- NullableConverter.cs
- PowerModeChangedEventArgs.cs
- ConditionCollection.cs
- XPathDocumentNavigator.cs
- ButtonColumn.cs
- Executor.cs
- DocumentOrderQuery.cs
- RemoteWebConfigurationHostServer.cs
- wmiutil.cs
- EndPoint.cs
- SqlDataSourceFilteringEventArgs.cs
- RealProxy.cs
- RadioButtonList.cs
- LinearQuaternionKeyFrame.cs
- EntityProviderServices.cs
- VirtualizingStackPanel.cs
- Vector3DIndependentAnimationStorage.cs
- ListMarkerLine.cs
- InlineUIContainer.cs
- ExtendedPropertyDescriptor.cs
- SoapAttributeAttribute.cs
- TreeViewBindingsEditor.cs
- HotSpotCollectionEditor.cs
- WorkflowTerminatedException.cs
- DynamicQueryableWrapper.cs
- WindowsAuthenticationEventArgs.cs
- MemberPath.cs
- SaveFileDialog.cs
- SingleObjectCollection.cs
- WebPartManager.cs
- ObjectStateManagerMetadata.cs
- ImageListImage.cs
- Graphics.cs
- XamlTypeMapper.cs
- DbConnectionFactory.cs
- ControlCachePolicy.cs
- OperatorExpressions.cs
- InkPresenterAutomationPeer.cs
- RoutedEventHandlerInfo.cs
- WindowAutomationPeer.cs
- TemplateControlBuildProvider.cs
- PersonalizationStateQuery.cs
- GraphicsContainer.cs
- BindingGraph.cs
- XmlSchemaAnnotated.cs
- Operand.cs
- ToolStripOverflow.cs
- BulletDecorator.cs
- AuthorizationRuleCollection.cs
- Exceptions.cs
- NotificationContext.cs
- Win32.cs
- DataGridViewCellMouseEventArgs.cs
- FlagsAttribute.cs
- OdbcParameterCollection.cs
- GridViewHeaderRowPresenterAutomationPeer.cs
- WebColorConverter.cs
- Package.cs
- SchemaEntity.cs
- NetPeerTcpBindingElement.cs
- DateTimeUtil.cs
- _Connection.cs
- TagPrefixCollection.cs
- TextRenderer.cs
- ToolStripSystemRenderer.cs
- HighlightComponent.cs
- printdlgexmarshaler.cs
- ScriptMethodAttribute.cs
- HMACRIPEMD160.cs
- HwndSource.cs
- DelegateBodyWriter.cs