Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Configuration / NetPeerTcpBindingElement.cs / 1 / NetPeerTcpBindingElement.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Configuration { using System.Net; using System.Collections.Generic; using System.ServiceModel; using System.Configuration; using System.Globalization; using System.ServiceModel.Channels; public partial class NetPeerTcpBindingElement : StandardBindingElement { public NetPeerTcpBindingElement(string name) : base(name) { } public NetPeerTcpBindingElement() : this(null) { } protected override Type BindingElementType { get { return typeof(NetPeerTcpBinding); } } [ConfigurationProperty(ConfigurationStrings.ListenIPAddress, DefaultValue = PeerTransportDefaults.ListenIPAddress)] [System.ComponentModel.TypeConverter(typeof(PeerTransportListenAddressConverter))] [PeerTransportListenAddressValidator()] public IPAddress ListenIPAddress { get { return (IPAddress)base[ConfigurationStrings.ListenIPAddress]; } set { base[ConfigurationStrings.ListenIPAddress] = value; } } [ConfigurationProperty(ConfigurationStrings.MaxBufferPoolSize, DefaultValue = TransportDefaults.MaxBufferPoolSize)] [LongValidator(MinValue = 0)] public long MaxBufferPoolSize { get {return (long) base[ConfigurationStrings.MaxBufferPoolSize]; } set {base[ConfigurationStrings.MaxBufferPoolSize] = value; } } [ConfigurationProperty(ConfigurationStrings.MaxReceivedMessageSize, DefaultValue = TransportDefaults.MaxReceivedMessageSize)] [LongValidator(MinValue = PeerTransportConstants.MinMessageSize)] public long MaxReceivedMessageSize { get { return (long)base[ConfigurationStrings.MaxReceivedMessageSize]; } set { base[ConfigurationStrings.MaxReceivedMessageSize] = value; } } [ConfigurationProperty(ConfigurationStrings.Port, DefaultValue = PeerTransportDefaults.Port)] [IntegerValidator(MinValue = PeerTransportConstants.MinPort, MaxValue = PeerTransportConstants.MaxPort)] public int Port { get { return (int)base[ConfigurationStrings.Port]; } set { base[ConfigurationStrings.Port] = value; } } [ConfigurationProperty(ConfigurationStrings.ReaderQuotas)] public XmlDictionaryReaderQuotasElement ReaderQuotas { get { return (XmlDictionaryReaderQuotasElement) base[ConfigurationStrings.ReaderQuotas]; } } [ConfigurationProperty(ConfigurationStrings.PeerResolver, DefaultValue = null)] public PeerResolverElement Resolver { get { return (PeerResolverElement)base[ConfigurationStrings.PeerResolver]; } } [ConfigurationProperty(ConfigurationStrings.Security)] public PeerSecurityElement Security { get { return (PeerSecurityElement)base[ConfigurationStrings.Security]; } } protected internal override void InitializeFrom(Binding binding) { base.InitializeFrom(binding); NetPeerTcpBinding peerBinding = (NetPeerTcpBinding) binding; this.ListenIPAddress= peerBinding.ListenIPAddress; this.MaxBufferPoolSize = peerBinding.MaxBufferPoolSize; this.MaxReceivedMessageSize = peerBinding.MaxReceivedMessageSize; this.Port = peerBinding.Port; this.Security.InitializeFrom(peerBinding.Security); this.Resolver.InitializeFrom(peerBinding.Resolver); this.ReaderQuotas.InitializeFrom(peerBinding.ReaderQuotas); } protected override void OnApplyConfiguration(Binding binding) { NetPeerTcpBinding peerBinding = (NetPeerTcpBinding)binding; peerBinding.ListenIPAddress = this.ListenIPAddress; peerBinding.MaxBufferPoolSize = this.MaxBufferPoolSize; peerBinding.MaxReceivedMessageSize = this.MaxReceivedMessageSize; peerBinding.Port = this.Port; peerBinding.Security = new PeerSecuritySettings(); this.ReaderQuotas.ApplyConfiguration(peerBinding.ReaderQuotas); this.Resolver.ApplyConfiguration(peerBinding.Resolver); this.Security.ApplyConfiguration(peerBinding.Security); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
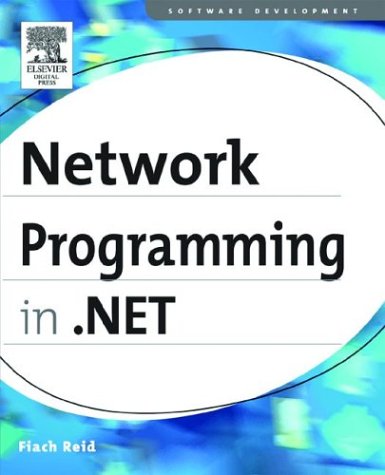
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TerminatorSinks.cs
- EnumMember.cs
- TextTreeObjectNode.cs
- PingReply.cs
- PageAsyncTaskManager.cs
- InkPresenter.cs
- TemplateInstanceAttribute.cs
- UInt32.cs
- LongValidatorAttribute.cs
- DSASignatureDeformatter.cs
- AQNBuilder.cs
- Roles.cs
- precedingquery.cs
- WindowsToolbarAsMenu.cs
- XmlDeclaration.cs
- TripleDES.cs
- EncodingDataItem.cs
- DesignerInterfaces.cs
- StatusBarPanelClickEvent.cs
- DiscoveryRequestHandler.cs
- DispatchChannelSink.cs
- DeviceContexts.cs
- HeaderCollection.cs
- OrderPreservingPipeliningMergeHelper.cs
- ExpressionDumper.cs
- PenCursorManager.cs
- FormatVersion.cs
- AppSettingsExpressionBuilder.cs
- PathNode.cs
- ExtendedProtectionPolicyTypeConverter.cs
- PrivilegedConfigurationManager.cs
- SqlDependency.cs
- Tile.cs
- StorageAssociationTypeMapping.cs
- HttpModulesSection.cs
- TempFiles.cs
- RuntimeDelegateArgument.cs
- DateRangeEvent.cs
- Point3DConverter.cs
- DataColumnPropertyDescriptor.cs
- MdiWindowListStrip.cs
- Int32AnimationBase.cs
- WmlCommandAdapter.cs
- FileResponseElement.cs
- CustomCategoryAttribute.cs
- WinCategoryAttribute.cs
- securestring.cs
- BaseCollection.cs
- StackBuilderSink.cs
- StrongNameIdentityPermission.cs
- Mapping.cs
- ListComponentEditorPage.cs
- LinkedResource.cs
- Accessible.cs
- HashAlgorithm.cs
- DataGridCommandEventArgs.cs
- SQLString.cs
- DateTimeFormatInfo.cs
- Point3DCollection.cs
- input.cs
- MissingMemberException.cs
- InvariantComparer.cs
- PickDesigner.xaml.cs
- cookiecollection.cs
- SynchronizationScope.cs
- DialogResultConverter.cs
- HttpRuntimeSection.cs
- entitydatasourceentitysetnameconverter.cs
- GridViewRowPresenter.cs
- WindowsAltTab.cs
- XmlStreamStore.cs
- _TLSstream.cs
- ScriptComponentDescriptor.cs
- DefaultTextStoreTextComposition.cs
- HtmlHead.cs
- CompilerErrorCollection.cs
- XmlCodeExporter.cs
- PseudoWebRequest.cs
- ShapeTypeface.cs
- ContentElementAutomationPeer.cs
- DesignerExtenders.cs
- LeaseManager.cs
- ServiceHttpHandlerFactory.cs
- OdbcPermission.cs
- TextRunProperties.cs
- SocketAddress.cs
- xml.cs
- DbMetaDataFactory.cs
- RoleManagerModule.cs
- WebPartDisplayMode.cs
- HWStack.cs
- DocumentsTrace.cs
- WindowsRichEdit.cs
- XmlILTrace.cs
- TabOrder.cs
- InvalidDataException.cs
- Switch.cs
- IImplicitResourceProvider.cs
- StructuralType.cs
- NetworkInformationPermission.cs